Troubleshooting Hello World Portlet Issues in JBoss Portal
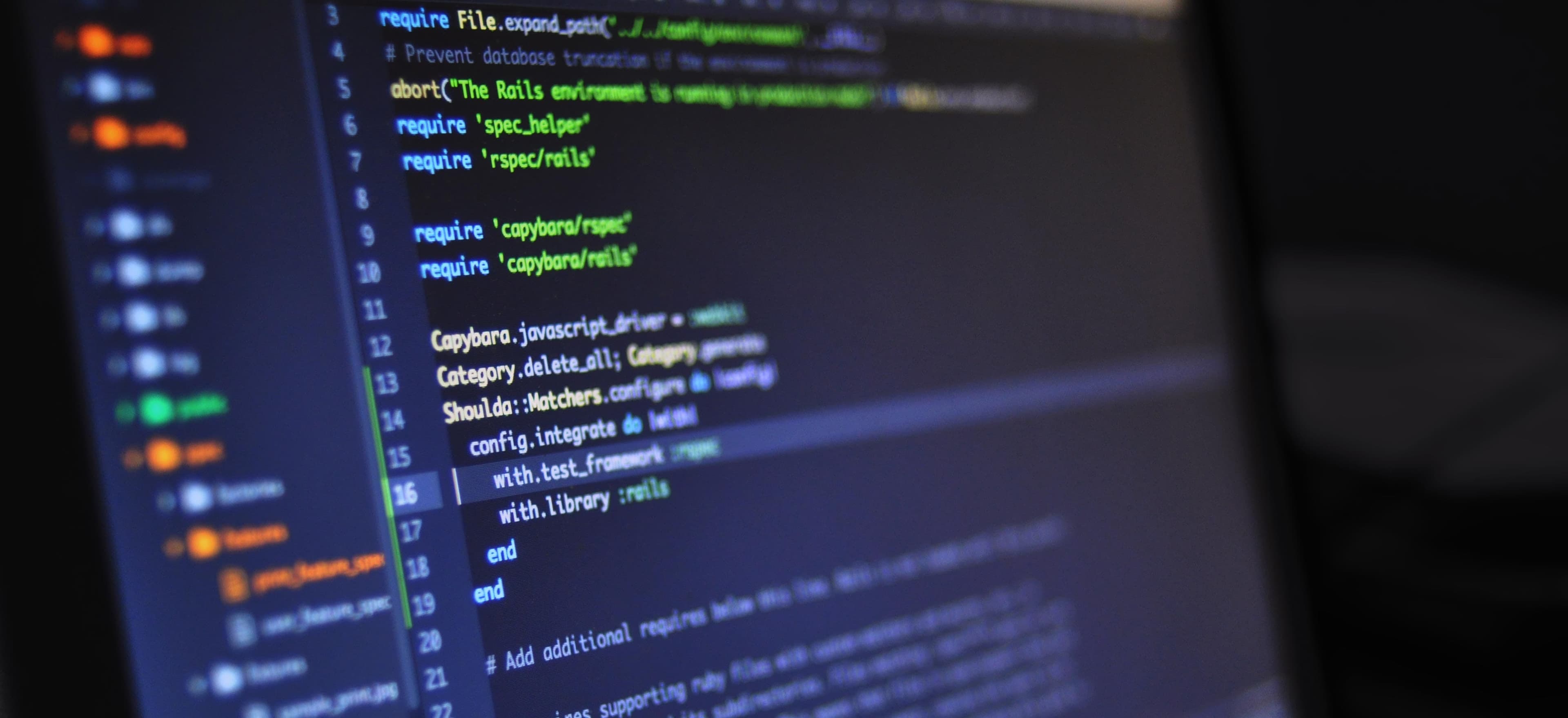
- Published on
Troubleshooting Hello World Portlet Issues in JBoss Portal
The JBoss Portal is a powerful tool for creating and managing web applications. Portlets are a significant component allowing for modular applications that can be integrated easily into any web portal. One of the most straightforward ways to get started with portlet development is by creating a "Hello World" portlet. However, as with any development environment, you may run into various issues. This blog post will guide you through common troubleshooting issues when deploying a Hello World portlet in JBoss Portal.
Table of Contents
- Understanding JBoss Portal and Portlets
- Setting Up Your Hello World Portlet
- Common Hello World Portlet Issues
- Debugging Best Practices
- Conclusion
Understanding JBoss Portal and Portlets
JBoss Portal is a feature-rich, open-source portal server that offers support for various enterprise technologies. Portlets are reusable web components designed to be displayed in a web portal. They follow the Java Portlet Specification (JSR 168). Each portlet runs in its own context and can interact with other portlets within the same portal.
Setting Up Your Hello World Portlet
To begin, here is a simple example of a Hello World portlet:
- Create Your Portlet Class: Below is a simple portlet example.
package com.example;
import javax.portlet.*;
import java.io.IOException;
import java.io.PrintWriter;
public class HelloWorldPortlet extends GenericPortlet {
@Override
protected void doView(RenderRequest request, RenderResponse response) throws PortletException, IOException {
response.setContentType("text/html");
PrintWriter writer = response.getWriter();
writer.println("<h1>Hello, World!</h1>");
}
}
Why This Code? This is a simple Java class that extends GenericPortlet
. The doView
method is overridden to define what happens when the portlet is rendered. Using PrintWriter
, we're sending HTML output, which will be displayed in the portlet.
- Create Your Deployment Descriptor: Next, you need a file called
portlet.xml
:
<portlet-app xmlns="http://java.sun.com/xml/ns/portlet"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://java.sun.com/xml/ns/portlet http://java.sun.com/xml/ns/portlet/portlet-app_2_0.xsd"
version="2.0">
<portlet>
<portlet-name>HelloWorldPortlet</portlet-name>
<display-name>Hello World Portlet</display-name>
<portlet-class>com.example.HelloWorldPortlet</portlet-class>
<init-param>
<name>message</name>
<value>Hello from JBoss Portal!</value>
</init-param>
<portlet-info>
<title>Hello World</title>
<short-title>HW</short-title>
<keywords>hello, world, portlet</keywords>
</portlet-info>
</portlet>
</portlet-app>
Why This Code? This XML file serves as a deployment descriptor for the portlet. It specifies the name, class, initialization parameters, and additional info. This is a crucial part of a portlet's identity within the JBoss Portal.
Common Hello World Portlet Issues
Despite a seemingly simple setup, there are several issues that can arise during portlet development.
Portlets Not Displaying
Symptoms: You compile and deploy your portlet, but it doesn't show up in the portal.
Solutions:
- Check Deployment: Make sure your WAR file is correctly deployed in the JBoss deployment directory (usually
JBoss/server/default/deploy
). - Portlet Configuration: Verify that your portlet is correctly listed in
portlet.xml
. - Permissions: If you're using security roles, ensure your user has the necessary roles assigned to view the portlet. You can see more about JBoss security configurations here.
ClassNotFoundException Issues
Symptoms: You receive a ClassNotFoundException
during runtime.
Solutions:
- Verify Classpath: Make sure your
HelloWorldPortlet.class
is included in the WAR file. - Dependency Libraries: Ensure that required libraries are available in the
WEB-INF/lib
folder. Missing libraries likeportlet-api
versions can lead to runtime exceptions.
To verify, open the WEB-INF/lib
directory and check:
ls -l WEB-INF/lib
Deployment Descriptor Problems
Symptoms: Your portlet might not function as expected.
Solutions:
- XML Schema Version: Verify that you are using the correct XML schema version in your
portlet.xml
. Here is another reference to the Java Portlet Specification which may help clear confusion about valid namespaces: JSR 168 Portlet Specification. - Validate XML: Use an XML validator to ensure your
portlet.xml
is well-formed. Misconfigured elements can lead to issues that are hard to debug.
Debugging Best Practices
- Logging: Utilize logging to identify issues. Always log critical operations, especially within your portlet lifecycle methods (
doView
,doEdit
, anddoProcessAction
). - Error Handling: Implement proper error handling for all potential exceptions. This helps narrow down where things may be going wrong.
- Development Tools: Use IDEs like IntelliJ IDEA or Eclipse with JBoss tools for streamlined development and easier debugging.
The Closing Argument
Building and deploying a Hello World portlet in JBoss Portal can be an engaging introduction to portlet development. When running into common issues such as portlet display problems, classpath issues, or deployment descriptor configurations, following established troubleshooting steps can save a significant amount of time.
Additional Resources
- Getting Started with JBoss Portal
- Java Portlet Specification
By following the insights shared in this blog post, you should be equipped to troubleshoot portlet issues effectively, so you can focus on building amazing applications instead! Happy coding!
Checkout our other articles