Common Pitfalls When Bootstrapping Apache Camel in Java EE 7
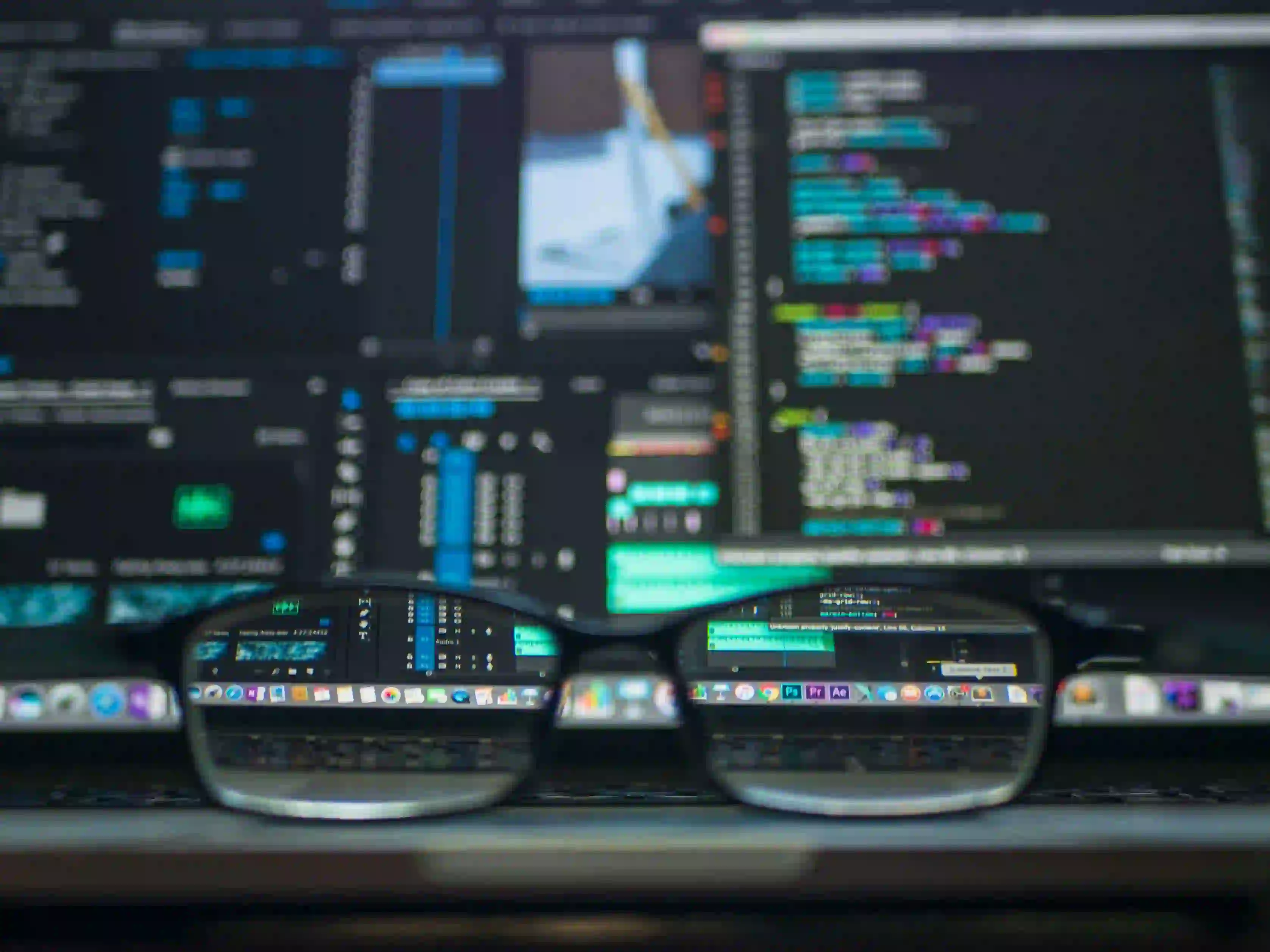
Common Pitfalls When Bootstrapping Apache Camel in Java EE 7
Apache Camel is a versatile integration framework that allows developers to connect various systems seamlessly. Bootstrapping Camel in a Java EE 7 environment introduces a fresh set of opportunities but also potential pitfalls. In this blog post, we will explore some common mistakes developers make while bootstrapping Apache Camel within their enterprise applications. By understanding these pitfalls, you can improve your integration projects and avoid headaches down the road.
Understanding Apache Camel
Before diving into common pitfalls, let’s briefly understand what Apache Camel is and its role in Java EE applications. Apache Camel provides a framework for rule-based routing and mediation of messages. It has a comprehensive list of components that allow integration with different transport protocols and data formats.
Utilizing the Enterprise Integration Patterns (EIPs), Camel offers a consistent API for various integration scenarios. This adaptability makes it the go-to solution for integrating disparate systems while following Java EE specifications.
Common Pitfalls of Bootstrapping Apache Camel
1. Skipping the Proper Dependency Injection
Mistake: Failing to utilize Java EE's built-in Dependency Injection (DI) for Camel context can lead to issues.
Explanation: Apache Camel integrates seamlessly with CDI (Contexts and Dependency Injection) and ensuring proper usage of DI simplifies access to beans defined in your application. A common pitfall occurs when developers create a new Camel context without leveraging the existing CDI context, which can lead to issues with component resolution.
Solution: Use CDI to manage the lifecycle of your Camel context.
@ApplicationScoped
public class MyCamelApplication {
@Inject
private CamelContext camelContext;
@PostConstruct
public void init() {
// Set up routes and other configurations here
camelContext.addRoutes(new MyRouteBuilder());
}
}
Why: This approach ensures that your Camel context is properly managed by the CDI container, facilitating the resolution of other beans.
2. Ignoring Transaction Management
Mistake: Neglecting to manage transactions can lead to data inconsistency and unpredictable application behavior.
Explanation: Java EE provides robust transaction management capabilities. When integrating with Camel, if your routes involve operations that modify the database, failing to employ transaction management can leave your data in an inconsistent state.
Solution: Use Camel's support for transactional routes.
from("jpa:com.example.MyEntity")
.to("someOtherComponent")
.transacted();
Why: This will ensure that if any part of your route fails, the transaction will roll back, preserving data integrity.
3. Not Leveraging the Camel Registry
Mistake: Accessing components directly rather than through the Camel registry can lead to tightly coupled code.
Explanation: When components are instantiated statically, it becomes challenging to make changes to configuration or swap components. By leveraging the Camel registry, you allow for more flexible designs.
Solution: Register your beans in the Camel registry context.
public void configure() throws Exception {
getContext().getRegistry().bind("myService", new MyServiceImplementation());
from("direct:start")
.to("bean:myService?method=doSomething");
}
Why: This enables loose coupling between your services and Camel routes, improving maintainability.
4. Failing to Properly Configure Error Handling
Mistake: Assuming the default error handling of Camel will suffice for all scenarios.
Explanation: While Camel has built-in error handling and retries, customizing these settings for specific routes can significantly enhance how your application handles failures.
Solution: Implement custom error handlers.
errorHandler(deadLetterChannel("jms:queue:deadLetter")
.maximumRedeliveries(3)
.redeliveryDelay(2000));
Why: This allows control over error management, enabling you to configure how many times a message should be retried and where it should go after it fails.
5. Focusing Solely on Routing
Mistake: Ignoring the importance of data format and transformation along with routing logic.
Explanation: Often, the focus can be narrowed down to just the routing of messages, neglecting the necessity of transforming data between various formats or protocols.
Solution: Use Camel's Transform and Processor capabilities to define how data is transformed along the route.
from("direct:input")
.process(new MyProcessor())
.marshal().json()
.to("someOtherComponent");
Why: Transforming the data ensures that it's prepared correctly for the next processing step or component, which is critical in integration scenarios.
6. Not Testing Routes and Components
Mistake: Considering the application integration complete once the routes have been configured without performing robust testing.
Explanation: Testing routes and components in isolation can uncover issues that are only visible during execution in a different environment.
Solution: Use the Camel Test Kit or custom unit tests with JUnit.
public class MyRouteTest extends CamelTestSupport {
@Override
protected RouteBuilder createRouteBuilder() {
return new MyRouteBuilder();
}
@Test
public void testRoute() throws Exception {
template.sendBody("direct:start", "Test Message");
// Assertion logic here
}
}
Why: Testing ensures that your routes behave as expected and reduces the likelihood of failures in production.
7. Neglecting Performance Tuning
Mistake: Failing to monitor and optimize Camel routes can lead to performance degradation as the application scales.
Explanation: Apache Camel provides several options for improving performance, including asynchronous processing, multithreading, and endpoint configurations.
Solution: Consider performance-tuning options:
from("direct:start")
.threads().executorService(myExecutorService).maxPoolSize(10)
.process(new MyProcessor());
Why: Implementing these optimizations can significantly improve the performance of your integrations.
Key Takeaways
Bootstrapping Apache Camel in Java EE 7 can unlock powerful integration possibilities. However, avoiding common pitfalls is crucial to ensure that your project runs smoothly and efficiently. Leveraging Dependency Injection, proper transaction management, the Camel registry, error handling, data transformation, testing, and performance tuning are key focuses for successful integration.
By keeping these pitfalls in mind and applying the recommended solutions, you will enhance your integration projects and significantly reduce the risk of encountering frustrating issues down the line.
For more information on Apache Camel and its integration capabilities, consult the official documentation and consider exploring Enterprise Integration Patterns for a deeper understanding of the fundamental patterns applied in integration design.