Common Errors When Inserting Content with Apache POI
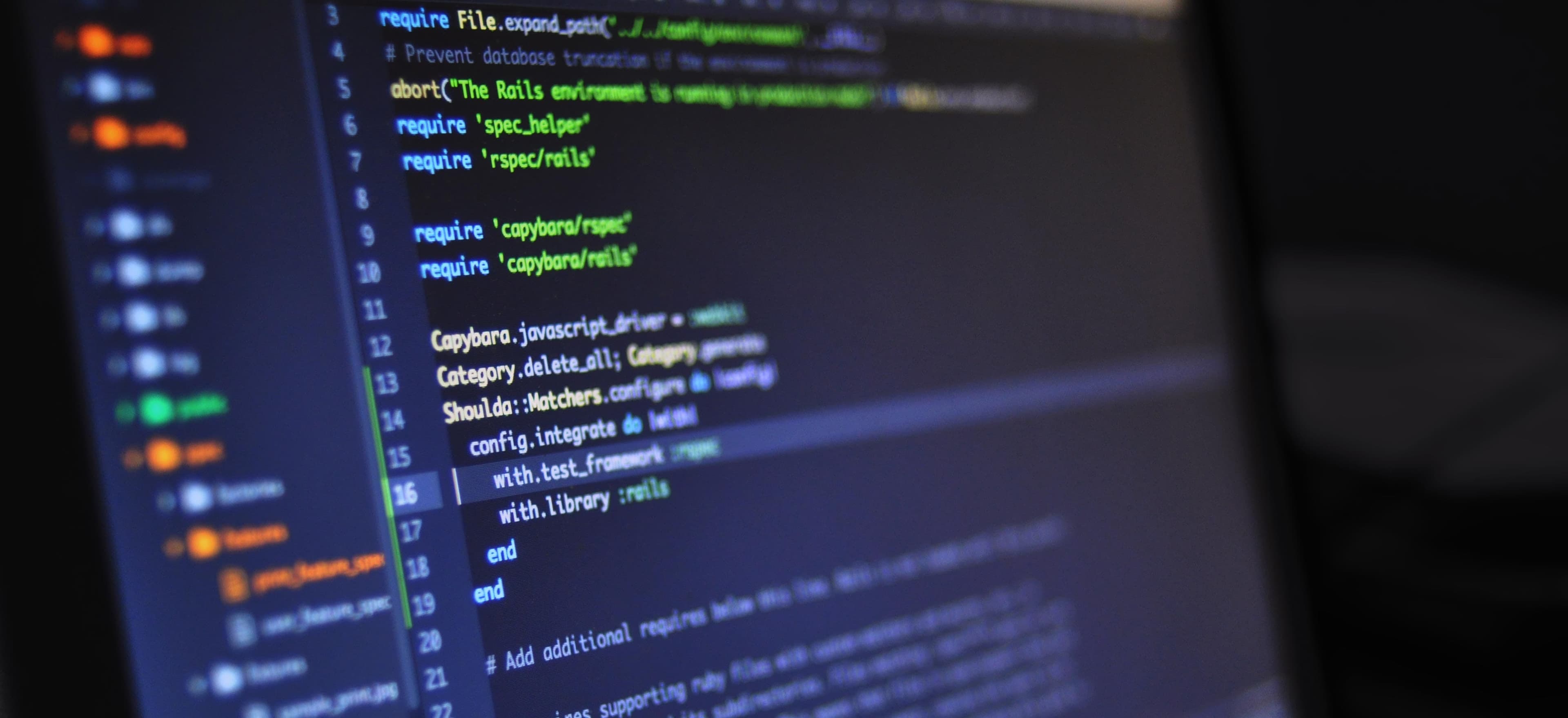
- Published on
Common Errors When Inserting Content with Apache POI
Apache POI is a powerful Java library that allows for the creation and manipulation of various file formats from the Microsoft Office suite, including Excel, Word, and PowerPoint formats. However, working with any library comes with its own set of challenges, particularly when it comes to handling errors. In this post, we’ll explore common errors encountered when inserting content with Apache POI and how to effectively troubleshoot and resolve them.
Overview of Apache POI
Apache POI provides support for both the older binary formats (HSSF for Excel and HWPF for Word) as well as the newer XML-based formats (XSSF for Excel and XWPF for Word). This makes it an invaluable tool for any Java developer needing to process these types of files.
Getting Started
Before we dive into the specifics of error handling, let’s set up a basic Apache POI project.
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>5.2.3</version>
</dependency>
<dependency>
<groupId>org.apache.xmlbeans</groupId>
<artifactId>xmlbeans</artifactId>
<version>5.1.1</version>
</dependency>
These dependencies in your pom.xml
file will let you utilize Apache POI in your Java project.
Common Errors When Inserting Content
1. IOException: File Not Found
When working with Apache POI, one of the most common errors you'll encounter is IOException: File Not Found
. This usually occurs when the file path specified in your code does not point to an existing file.
Resolution:
Ensure the file path is correct and the file exists in that path. You can also use File
class methods to check if the file exists.
File file = new File("path/to/your/file.xlsx");
if (!file.exists()) {
System.out.println("File not found: " + file.getAbsolutePath());
}
2. NullPointerException when Accessing Workbook
Another error you might face is a NullPointerException
when trying to access a workbook. This may occur if the path provided does not lead to a valid Excel file or if the file could not be opened due to permissions.
Resolution: A basic check can prevent this error:
Workbook workbook = null;
try {
FileInputStream fis = new FileInputStream(file);
workbook = WorkbookFactory.create(fis);
} catch (IOException e) {
e.printStackTrace();
}
if (workbook == null) {
System.out.println("Workbook is null, check the file format or ensure it opens correctly.");
}
3. Incorrect Cell Type
When inserting data into cells, you may encounter an IllegalArgumentException
, particularly if you are trying to assign an incompatible type to a cell, such as attempting to insert a string into a numeric cell.
Resolution: Ensure that the data type you are inserting matches the cell's expected type:
Cell cell = row.createCell(0);
if (data instanceof String) {
cell.setCellValue((String) data);
} else if (data instanceof Double) {
cell.setCellValue((Double) data);
} else {
throw new IllegalArgumentException("Unsupported data type");
}
4. Formula Evaluation Errors
If you are working with formulas in Excel and encounter FormulaParseException
, this indicates that the formula you are trying to evaluate is incorrectly formatted or contains invalid references.
Resolution: Double-check the formula syntax. Additionally, you can validate the formula before evaluating it.
Cell formulaCell = row.createCell(1);
formulaCell.setCellFormula("SUM(A1:A10)"); // Example formula
FormulaEvaluator evaluator = workbook.getCreationHelper().createFormulaEvaluator();
CellValue cellValue = evaluator.evaluate(formulaCell);
if (cellValue.getCellType() == CellType.ERROR) {
System.out.println("Error in formula: " + cellValue.getErrorValue());
}
5. OutOfMemoryError
When manipulating large files or datasets, you might run into OutOfMemoryError
. This is particularly common when dealing with large Excel files, as they can take up a significant amount of memory.
Resolution:
If dealing with massive datasets, consider using the SXSSF
(Streaming Usermodel API) instead of XSSF
. SXSSF
allows for the writing of large Excel files while consuming less memory.
SXSSFWorkbook workbook = new SXSSFWorkbook();
Sheet sheet = workbook.createSheet("LargeData");
// Add data in a loop to the sheet
Best Practices When Using Apache POI
-
Always close your streams: Failure to do so can cause memory leaks.
try (FileInputStream fis = new FileInputStream(file); Workbook workbook = WorkbookFactory.create(fis)) { // Your code here } catch (IOException e) { e.printStackTrace(); }
-
Use a try-catch block appropriately: Handling exceptions properly helps you find issues more easily.
-
Read Documentation: The Apache POI Documentation provides a wealth of information on the capabilities and limitations of the library.
Final Considerations
Working with Apache POI can be incredibly rewarding, but it comes with its share of challenges. By understanding the common errors associated with it and implementing best practices, developers can streamline their workflow and improve their applications' robustness. Remember, like any other library, continuous learning and exploration are key to mastering Apache POI.
For further reading, consider checking out these resources:
- Apache POI official documentation
- Working with Excel in Java using Apache POI
Embrace coding with confidence, and may your Apache POI implementations be error-free!