Troubleshooting Common Async REST Call Issues
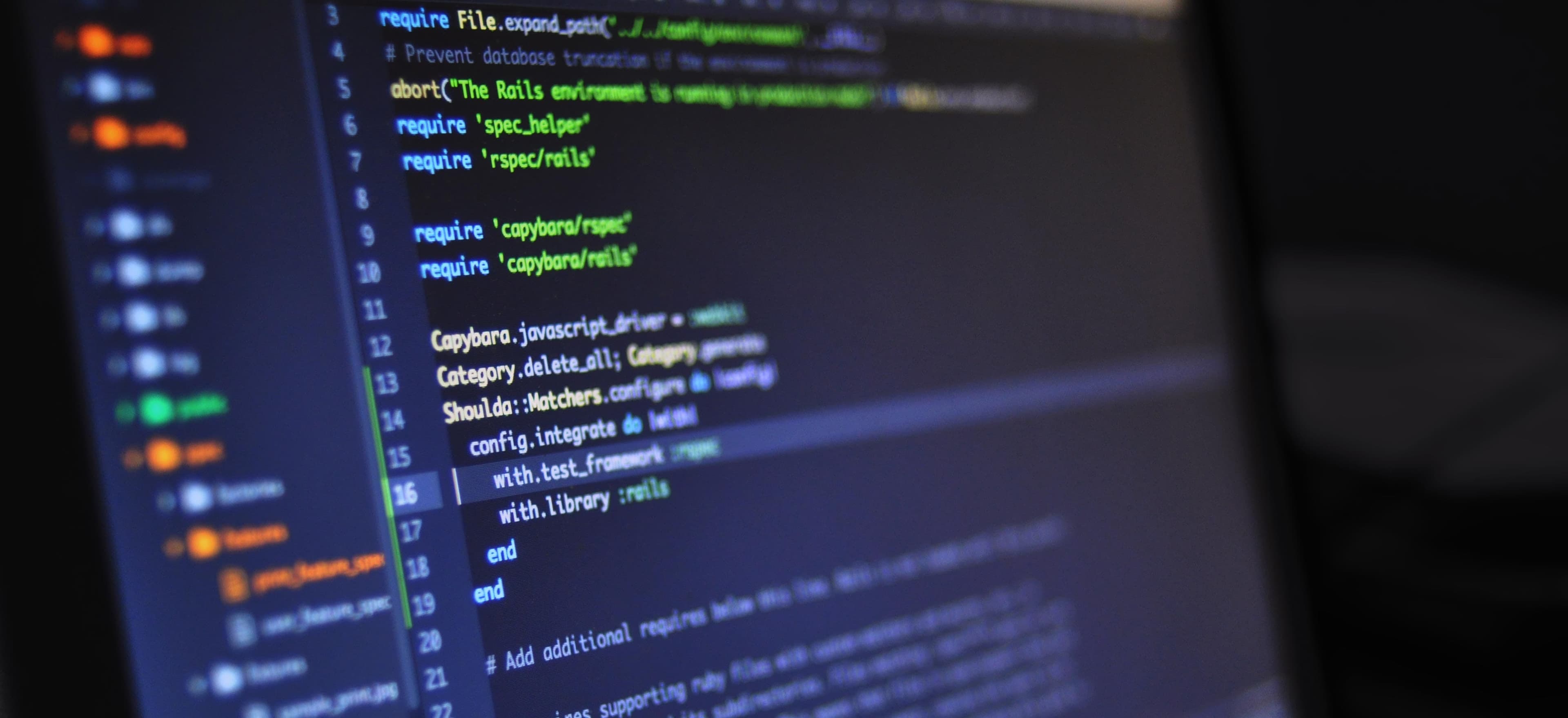
- Published on
Troubleshooting Common Async REST Call Issues in Java
When developing software applications, asynchronous programming is often a great approach. It improves responsiveness and allows for non-blocking I/O operations. However, making asynchronous REST calls in Java can present various challenges. This post will explore common issues developers face with async REST calls and provide troubleshooting tips.
Understanding Asynchronous REST Calls
Before we dive into troubleshooting, it's crucial to understand what an asynchronous REST call entails. Unlike synchronous calls, which block the executing thread until a response is received, asynchronous calls allow the thread to continue executing while waiting for a response. This is particularly beneficial in web services, where requests may take a while to process, such as fetching resources from a server.
Here is a simple example of an asynchronous REST call using the CompletableFuture
class in Java:
import java.net.HttpURLConnection;
import java.net.URL;
import java.util.concurrent.CompletableFuture;
public class AsyncRestCall {
public CompletableFuture<String> fetchData(String urlStr) {
return CompletableFuture.supplyAsync(() -> {
try {
URL url = new URL(urlStr);
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("GET");
// Check if the response code is 200 (HTTP_OK)
if (conn.getResponseCode() != HttpURLConnection.HTTP_OK) {
throw new RuntimeException("Failed : HTTP error code : " + conn.getResponseCode());
}
// ... (Read response)
return "Data fetched successfully!";
} catch (Exception e) {
throw new RuntimeException("Exception in fetching data: " + e.getMessage());
}
});
}
}
Why Use CompletableFuture?
- Non-blocking: The
supplyAsync
method allows the code to run non-blocking. You can carry on with other tasks instead of waiting for a response. - Error handling: You can manage asynchronous exceptions effectively.
- Chaining: It can be easily chained with other asynchronous tasks.
Common Issues with Async REST Calls
1. Deadlocks
Deadlocks can occur when two or more threads are waiting for each other indefinitely. This is more common in applications that use shared resources. Here’s a scenario:
CompletableFuture<Void> future1 = CompletableFuture.runAsync(() -> {
// some task
});
CompletableFuture<Void> future2 = CompletableFuture.runAsync(() -> {
// waits for future1
future1.join();
});
// This can lead to a deadlock if future1 is waiting on future2.
Solution:
- Avoid waiting on futures that rely on each other. Use
thenApply
,thenAccept
, orthenCombine
, which allow for a non-blocking relational task execution.
2. Exception Handling
When using asynchronous calls, exceptions can be tricky. If an exception is thrown inside a CompletableFuture, it won’t be caught by traditional try-catch
blocks.
Handling with exceptionally:
fetchData("https://example.com")
.exceptionally(ex -> {
System.out.println("Error occurred: " + ex.getMessage());
return "Default value"; // return fallback value
});
3. Timeout Issues
Sometimes, requests may take longer than expected. In such cases, the application might hang or become unresponsive.
Solution:
Utilize the completeOnTimeout
method.
CompletableFuture<String> future = fetchData("https://example.com")
.completeOnTimeout("Fallback data", 5, TimeUnit.SECONDS);
4. Memory Leaks
Asynchronous calls can lead to memory issues if not handled correctly. For example, if references to objects are kept longer than necessary, it could create memory leaks.
Solution:
- Always clean up resources. Use
Runnable
orCallable
that does not retain outer references. - Implement proper cancellation mechanisms.
5. Not Handling Timeouts & Retries Properly
If a REST service fails to respond, it’s vital to handle such situations gracefully by implementing time-outs and retry mechanisms.
Example of a retry logic:
import java.util.function.Supplier;
public class RestClient {
public <T> T executeWithRetry(Supplier<T> supplier, int maxRetries) {
for (int i = 0; i < maxRetries; i++) {
try {
return supplier.get();
} catch (Exception e) {
// Log the exception and retry
System.out.println("Attempt " + (i + 1) + " failed: " + e.getMessage());
}
}
throw new RuntimeException("All retries failed.");
}
}
Best Practices
Now that we’ve addressed common issues, let’s outline some best practices for handling asynchronous REST calls in Java.
-
Use a Reliable Library: Consider libraries such as
Spring WebFlux
,Retrofit
, orOkHttp
, which simplify asynchronous handling and provide built-in error handling and timeouts. -
Implement Monitoring: Use metrics and logging to monitor the performance and failure rates of your asynchronous requests. This information can be invaluable when debugging.
-
Prioritize Task Management: Ensure that tasks are well-prioritized, and avoid starting too many threads at once, as this can lead to resource contention.
-
Test Thoroughly: Write extensive tests for asynchronous code. Use mocking frameworks to simulate various scenarios, including timeouts and failed requests.
-
Explore Reactive Programming: For advanced users, consider reactive programming paradigms that facilitate asynchronous programming using libraries like Project Reactor or RxJava, which can greatly simplify your async workflow.
Key Takeaways
Asynchronous REST calls in Java can offer significant performance improvements, but they come with their unique set of challenges. Understanding how to troubleshoot common issues like deadlocks, exceptions, timeouts, and memory leaks is crucial for building robust applications.
Remember to follow best practices and leverage libraries that help manage the complexities of asynchronous programming. By doing so, you’ll be better equipped to handle the unique challenges of async REST calls and build efficient, responsive applications.
For more in-depth resources, you might want to check the Java Concurrency documentation or explore Spring's reactive programming support. These resources can provide additional insight and guidance in managing async calls effectively.