How Optional Objects Prevent Null Pointer Turmoil
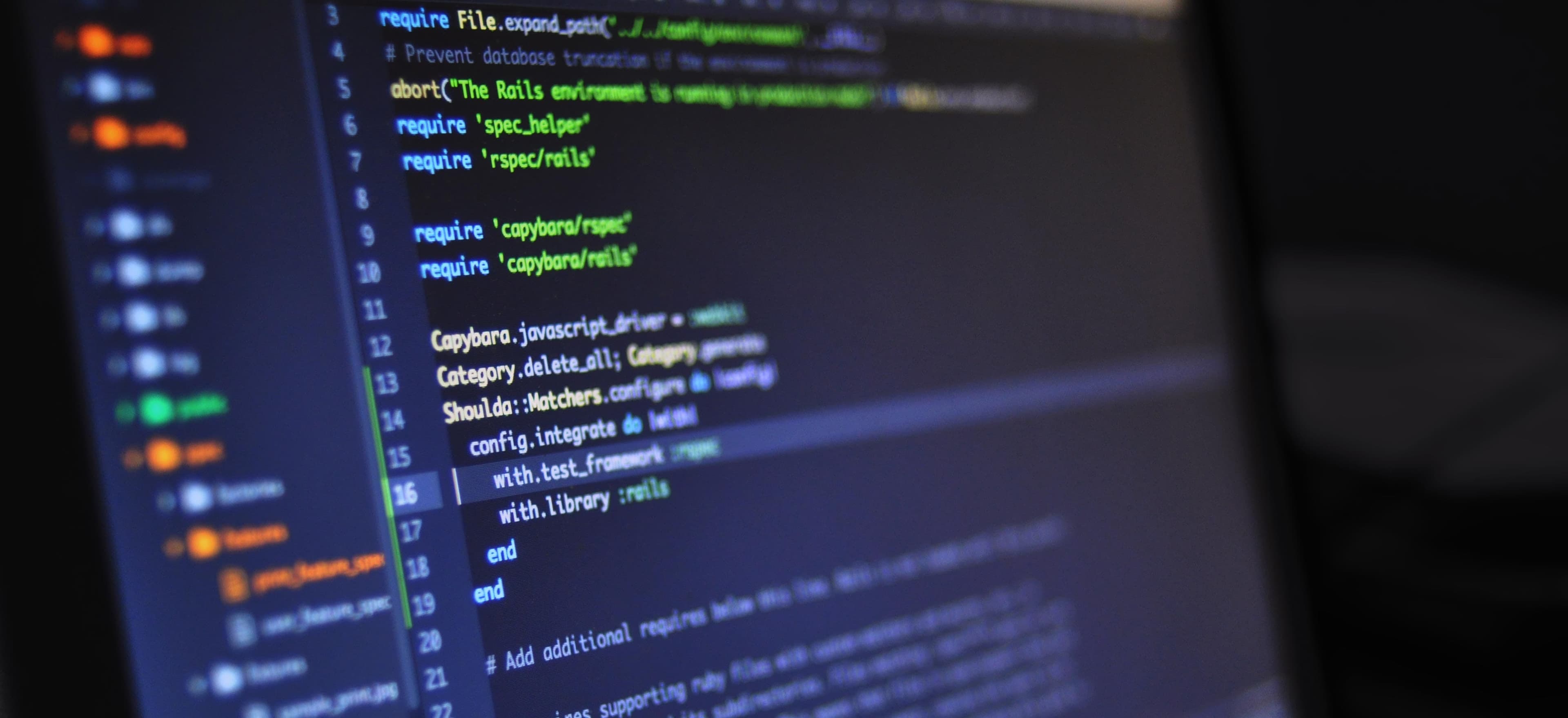
- Published on
How Optional Objects Prevent Null Pointer Turmoil in Java
Null pointer exceptions can lead to frustrating debugging sessions and unpredictable program behavior. In Java, developers often grapple with the infamous NullPointerException
(NPE), which can disrupt code execution when attempting to access an object reference that is null. Luckily, Java 8 introduced Optional
, a container object that helps to alleviate these issues. In this blog post, we'll dive into Optional
, examining its design, best practices, and how it can streamline your Java applications.
Understanding Null Pointer Exceptions
Before we explore Optional
, let’s touch on why NPEs occur in the first place. A null pointer exception indicates that the program attempted to reference an object that is not initialized. For example:
public class User {
private String name;
public String getName() {
return name.length() > 0 ? name : null; // Potential NPE
}
}
If name
is null, name.length()
will throw a NullPointerException
. This situation is common and can often lead to widespread issues in larger systems.
Introducing Optional
What is Optional?
Optional
is a type introduced in Java 8 that serves as a container for objects that may or may not be present. The main advantage is that it explicitly defines the possibility of absence, encouraging you as a developer to think about the "no value" case.
Basic Usage
To initiate an Optional
, you can use the Optional.of()
, Optional.ofNullable()
, or Optional.empty()
methods:
Optional<String> optionalName1 = Optional.of("John Doe");
Optional<String> optionalName2 = Optional.ofNullable(null); // This won't throw NPE
Optional<String> optionalName3 = Optional.empty(); // No value
Optional.of()
: Throws an exception if the value is null.Optional.ofNullable()
: Allows for a nullable value.Optional.empty()
: Represents an absent value.
Checking for Presence
Once you have an Optional
object, you can check if a value is present:
if (optionalName1.isPresent()) {
System.out.println("Name: " + optionalName1.get());
} else {
System.out.println("No name present.");
}
While this works, there are better, more idiomatic approaches in Java.
Avoiding Null Pointer Exceptions
ifPresent
Method
You can use the ifPresent()
method as a cleaner alternative:
optionalName1.ifPresent(name -> System.out.println("Name: " + name));
This method takes a lambda expression, which executes if the value is present, thereby removing the need for explicit null checks.
Default Values with orElse
If you want to provide a default value when the Optional
is empty, use orElse()
:
String defaultName = optionalName2.orElse("Default Name");
System.out.println("Name: " + defaultName);
orElseGet
for Lazy Evaluation
If the default value involves a computation, you might prefer orElseGet()
:
String name = optionalName2.orElseGet(() -> "Default Name");
In this case, the lambda only executes if the Optional
is empty, making it more efficient.
Mapping Values with map
If you need to transform the contained value, you can utilize the map()
method:
Optional<String> upperCaseName = optionalName1.map(String::toUpperCase);
upperCaseName.ifPresent(System.out::println); // Prints: JOHN DOE
Here, if optionalName1
contains a value, map()
transforms it to upper case.
Chaining Operations with flatMap
To avoid nested Optional
instances, you can use flatMap()
. It's particularly useful when dealing with methods that return Optional
themselves.
Optional<User> userOptional = Optional.of(new User());
Optional<String> userName = userOptional
.flatMap(user -> user.getName())
.map(String::toUpperCase);
userName.ifPresent(System.out::println);
This eliminates unnecessary wrapping and provides you with a concise way to handle chains.
Best Practices with Optional
While Optional
is a powerful tool, here are some best practices to keep in mind:
Do Not Use Optional
for Fields
Utilizing Optional
as a class field is generally discouraged in favor of traditional null checks. Instead, opt to return Optional
from methods.
Favor Optional
for Return Types
When a method may not return a value, return an Optional
. This practice improves readability while clearly communicating potential absences.
Avoid Using Null in Optional
Defensively, you should avoid wrapping null inside an Optional
via Optional.of()
; use Optional.ofNullable()
instead. The intention is to prevent NPEs and make your code self-documenting.
Final Considerations
By embracing Optional
, Java developers can significantly reduce the frequency of null pointer exceptions and encourage cleaner, more maintainable code. The functionalities of Optional
– such as map()
, flatMap()
, ifPresent()
, orElse()
, and orElseGet()
– enhance your ability to handle potential absence gracefully.
With careful usage, Optional
not only clarifies intent but also leads to improved error handling and reduction of boilerplate code.
Additional Resources
To delve deeper into the subject, consider visiting these resources:
- Java Optional Documentation
- Effective Java by Joshua Bloch
- Handling Nullability in Java
With the strategies discussed above, you are well-equipped to take full advantage of Optional
in your projects, reducing the risk of null pointer exceptions and streamlining your Java development process.