Common Pitfalls in Google App Engine JAX-RS REST Services
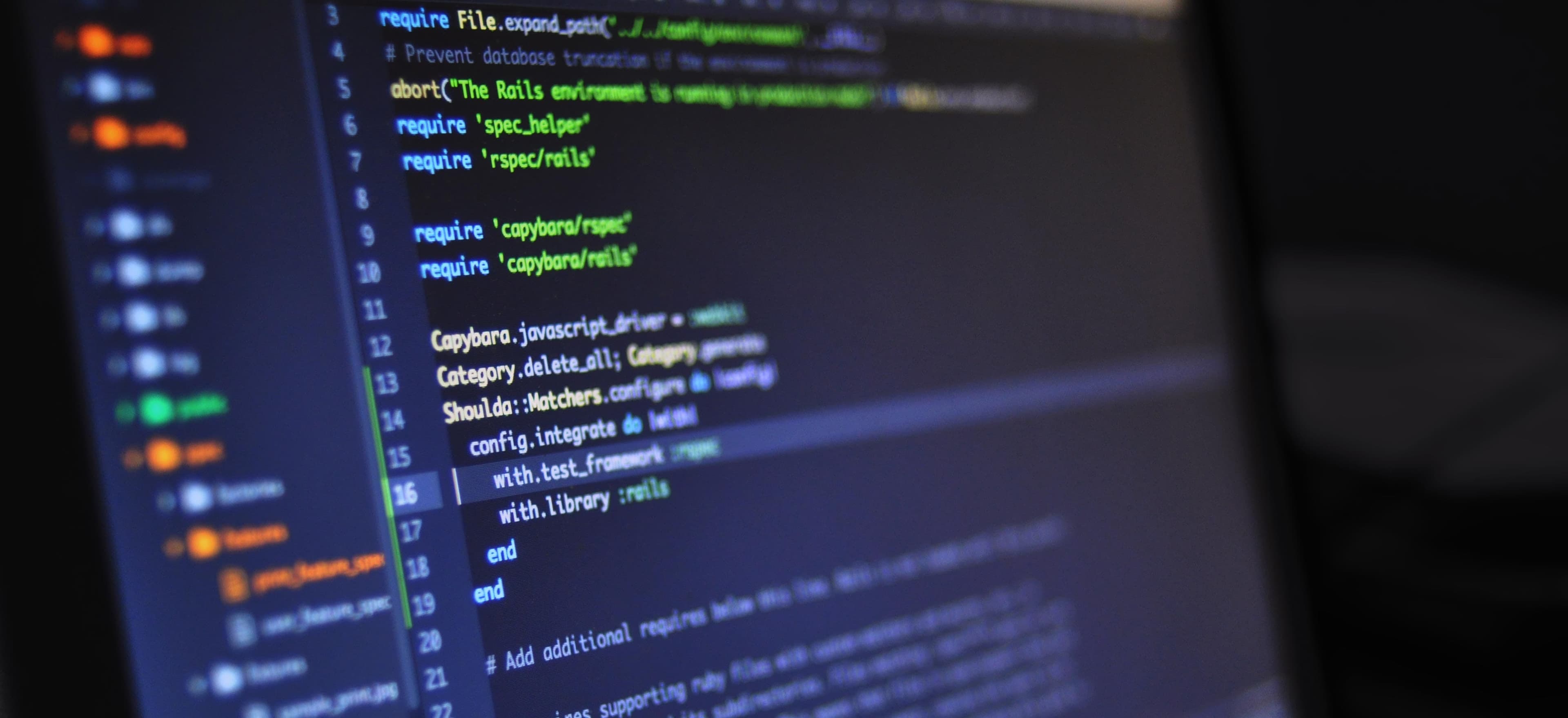
- Published on
Common Pitfalls in Google App Engine JAX-RS REST Services
Developing RESTful services on Google App Engine (GAE) using JAX-RS can be a powerful yet challenging endeavor. While JAX-RS simplifies creating web services in Java, GAE introduces its own set of rules and constraints. This blog post will delve deep into common pitfalls developers face when leveraging JAX-RS on GAE, offering clarity, guidance, and exemplary code snippets to help you navigate these challenges effectively.
Understanding JAX-RS and Google App Engine
JAX-RS (Java API for RESTful Web Services) is a set of APIs to create REST web services in Java. It provides annotations to simplify the development process while adhering closely to the REST architectural style. Meanwhile, Google App Engine is a scalable cloud computing platform that allows developers to build and deploy applications in various programming languages, including Java.
While both technologies complement each other, integrating them can lead to pitfalls that might not be immediately obvious. Let's explore some of the most common challenges developers encounter.
1. Dependency Management Issues
One of the first challenges that developers face is ensuring proper dependency management. GAE has a set of libraries that are often outdated or incompatible with newer libraries that you might want to use for your JAX-RS application.
How To Avoid:
-
Use Maven or Gradle: Ensure you are managing your dependencies properly using a build tool. Add necessary JARs in your
pom.xml
(for Maven) orbuild.gradle
(for Gradle). -
Check Compatibility: Refer to the GAE documentation regarding supported libraries. Ensure that the versions you are using for JAX-RS are compatible with the runtime of GAE.
<dependency>
<groupId>javax.ws.rs</groupId>
<artifactId>javax.ws.rs-api</artifactId>
<version>2.1</version>
</dependency>
In the above example, ensure that the JAX-RS version specified is supported in GAE.
2. Improper URL Mapping
URL mapping can get tricky in JAX-RS if not handled properly. An incorrect URL configuration can lead to runtime errors that are difficult to diagnose.
How To Avoid:
When defining your resource classes and methods, make sure to check the following:
- Ensure that your class-level and method-level
@Path
annotations are correctly set. - Be familiar with how GAE handles URL routing and ensure your paths do not collide.
Example:
@Path("/users")
public class UserResource {
@GET
@Path("/{id}")
public Response getUser(@PathParam("id") String id) {
// Implementation
}
}
In this code snippet, ensure that the method for fetching a user is correctly mapped to /users/{id}
. This straightforward structure helps you debug routing issues.
3. Misunderstanding GAE Quotas and Limits
Google App Engine enforces specific quotas and limits on various resources, such as memory, CPU usage, and outgoing requests. Overstepping these limits leads to errors and degraded performance.
How To Avoid:
-
Monitor Usage: Make use of Google Cloud Console to monitor your application's performance and resource usage. Set up alerts to notify you when you approach these quotas.
-
Optimize Code: Review your codebase for performance optimization, avoiding unnecessary computations.
An example of optimized code:
@POST
@Path("/createUser")
public Response createUser(User user) {
if (isValidUser(user)) {
// Logic to persist user
}
return Response.status(Response.Status.BAD_REQUEST).build();
}
// Validation method
private boolean isValidUser(User user) {
return user.getName() != null && !user.getName().isEmpty();
}
This code checks for user validity in a controlled manner, optimizing server resources by not executing further logic if the user is not valid.
4. Handling Serialization and Deserialization
When sending or receiving JSON data, it’s crucial to correctly serialize and deserialize Java objects. A misconfiguration can lead to data loss or errors in processing JSON objects.
How To Avoid:
- Utilize libraries like Jackson or Gson for handling JSON. Make sure your media types are set up correctly.
Example configuration for Jackson in JAX-RS:
@Provider
@Produces(MediaType.APPLICATION_JSON)
@Consumes(MediaType.APPLICATION_JSON)
public class JacksonJsonProvider extends ObjectMapper implements ContextResolver<ObjectMapper> {
@Override
public ObjectMapper getContext(Class<?> type) {
return this; // Returning the default ObjectMapper
}
}
Here, a custom ObjectMapper
integrates with JAX-RS allowing seamless JSON handling.
5. Exception Handling in the Application
While developing REST APIs, proper exception handling is crucial for providing meaningful error messages to API consumers. Ignoring this aspect leads to generic errors which can confuse users and developers alike.
How To Avoid:
- Implement a global exception handler using
ExceptionMapper
.
Example:
@Provider
public class CustomExceptionMapper implements ExceptionMapper<Throwable> {
@Override
public Response toResponse(Throwable exception) {
return Response.status(Response.Status.INTERNAL_SERVER_ERROR)
.entity("Error occurred: " + exception.getMessage())
.type(MediaType.TEXT_PLAIN)
.build();
}
}
This handler catches exceptions and returns a uniform error response.
6. Security Concerns
Security is paramount in APIs. Failing to implement secure communication can expose your application to vulnerabilities.
How To Avoid:
-
Implement OAuth2: Secure your API using OAuth2 or other authentication methods. Prevent unauthorized access to sensitive endpoints.
-
Validating Inputs: Always validate and sanitize inputs to prevent various types of attacks, such as SQL injection or XSS.
@POST
@Path("/addComment")
public Response addComment(Comment comment) {
if(comment.getText() != null && comment.getText().length() > 255) {
throw new WebApplicationException("Comment is too long", Response.Status.BAD_REQUEST);
}
// Logic to add comment
}
In this example, checking the length of the comment helps safeguard your application from potential threats effectively.
Lessons Learned
Deploying JAX-RS REST services on Google App Engine can be incredibly fruitful but fraught with pitfalls. By being aware of common issues, such as dependency management, URL mapping mistakes, GAE limitations, serialization challenges, exception handling, and security concerns, you can build reliable and efficient RESTful services.
For more on building robust applications, the Google Cloud documentation is a valuable resource, and JAX-RS specifications provide comprehensive insights into proper usage. By understanding these common pitfalls and implementing the tips outlined above, you can enhance your development process and produce high-quality applications.
Embrace the JAX-RS and GAE journey with confidence, and remember, the road may be bumpy, but knowledge and preparation can smooth the path ahead!