Testing Challenges with Annotated Controllers in Spring WebFlux
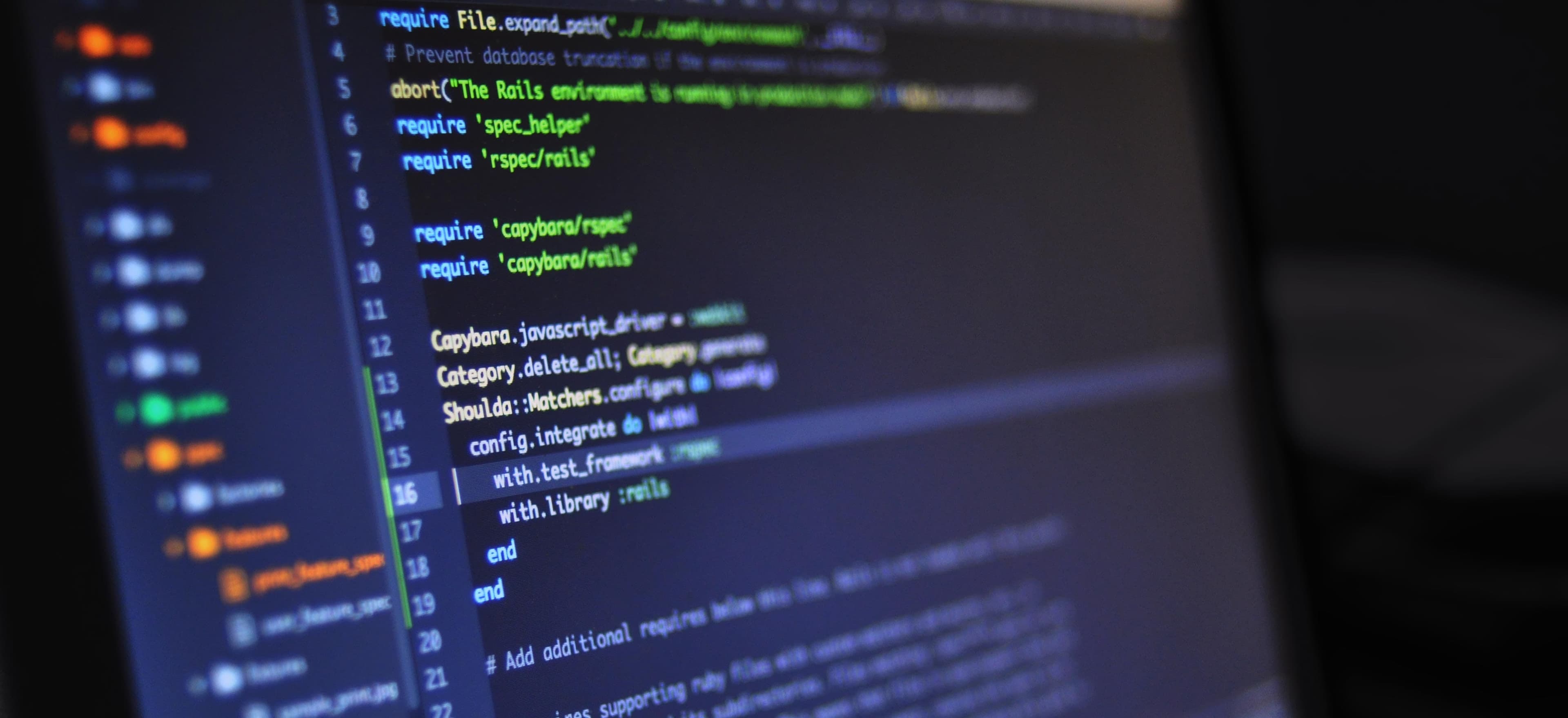
- Published on
Testing Challenges with Annotated Controllers in Spring WebFlux
In modern web application development, asynchronous processing has become a necessity. Spring WebFlux is a powerful framework that allows developers to build non-blocking applications using reactive programming principles. One of the most common challenges developers face when using Spring WebFlux is testing their annotated controllers. This blog post dives deep into the testing challenges associated with annotated controllers in Spring WebFlux while providing practical code snippets and clarifying the rationale behind them.
Understanding Spring WebFlux and Annotated Controllers
Spring WebFlux is part of the Spring Framework that is designed for building reactive web applications. Unlike the traditional Spring MVC, which uses a synchronous model, WebFlux operates asynchronously, making it more efficient, especially under load. It provides a reactive programming model that relies on Project Reactor.
Annotated controllers grant developers an elegant way to build RESTful APIs. They utilize annotations like @RestController
and @RequestMapping
. This simplicity, however, introduces some complexity when it comes to testing.
Why Testing Matters
Testing ensures that your application behaves as expected in a variety of scenarios. In reactive programming, where the flow of data is non-linear, testing can be a tricky endeavor. We want to validate not only the correctness of our business logic but also ensure the application handles backpressure, reacts to slow consumers, and processes.
Common Testing Challenges
1. Reactive Types Overhead
In WebFlux, the primary data types you’ll be working with are Mono
and Flux
. These types represent single or multiple asynchronous values, respectively. While these types are powerful, they introduce testing overhead since traditional testing strategies, which often rely on blocking calls, are not compatible.
Example:
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
import reactor.core.publisher.Mono;
@RestController
public class ExampleController {
@GetMapping("/example")
public Mono<String> getExample() {
return Mono.just("Hello, Spring WebFlux!");
}
}
When testing the getExample
method, you are working with a Mono<String>
. This is not a standard type that you can handle with straightforward assertions in your tests.
2. Asynchronous Execution
When a controller method returns a reactive type, the execution is asynchronous. This means if you are testing a method like our getExample
, you will need to take the completion of the reactive pipeline into account.
Testing Annotated Controllers Using WebFlux Test
Fortunately, Spring provides tools that help you test reactive controllers easily. The @WebFluxTest
annotation sets up a Spring testing context for a WebFlux application, allowing us to test controllers in isolation.
Setting Up the Test
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.autoconfigure.web.reactive.WebFluxTest;
import org.springframework.test.web.reactive.MockMvc;
import org.springframework.web.reactive.result.method.annotation.ResponseEntityExceptionHandler;
import static org.springframework.test.web.reactive.request.MockMvcRequestBuilders.get;
import static org.springframework.test.web.reactive.result.MockMvcResultMatchers.status;
import static org.springframework.test.web.reactive.result.MockMvcResultMatchers.content;
@WebFluxTest(ExampleController.class)
public class ExampleControllerTest {
@Autowired
private MockMvc mockMvc;
@Test
public void testGetExample() throws Exception {
mockMvc.perform(get("/example"))
.andExpect(status().isOk())
.andExpect(content().string("Hello, Spring WebFlux!"));
}
}
Explanation:
-
@WebFluxTest: This annotation is specifically designed for testing WebFlux applications. It auto-configures Spring components but only loads the necessary ones, excluding services or repositories by default.
-
MockMvc: This utility allows you to send mock HTTP requests and assert the responses. It handles the asynchronous nature of WebFlux behind the scenes, making it easier for developers to write tests.
-
Asynchronous Assertions: In the
testGetExample
method, notice how we seamlessly perform assertions against theMono<String>
return type without needing to block the thread.
Challenges in Error Handling
A crucial part of testing involves simulating errors. When using reactive types, the error handling within your pipeline must be tested robustly.
Example Controller Method with Error Handling:
@GetMapping("/error")
public Mono<String> getError() {
return Mono.error(new RuntimeException("This is an intentional error!"));
}
Testing the Error Case:
@Test
public void testGetError() throws Exception {
mockMvc.perform(get("/error"))
.andExpect(status().is5xxServerError())
.andExpect(result -> assertTrue(result.getResolvedException() instanceof RuntimeException));
}
Why This Matters:
Testing your controller's response to errors ensures that your application can handle faults gracefully. In a reactive application, simply catching an exception at one point might not suffice; understanding the flow is vital.
Concurrency Issues
Another pressing challenge with annotated controllers in WebFlux is dealing with concurrency, especially when shared resources are involved. In typical Spring applications, using synchronized
blocks can mitigate some issues. However, in a non-blocking setup with RabbitMQ, for example, you'll need to use reactive patterns to prevent thread contention.
Managing Concurrency
You can manage shared resources via Sinks
in Project Reactor or by using specific synchronization techniques catered to non-blocking IO.
Example:
When using Sinks
, concurrency issues can be managed like so:
import reactor.core.publisher.Sinks;
@RestController
public class ReactiveResourceController {
private final Sinks.Many<String> sink = Sinks.many().multicast().directAllOrNothing();
@PostMapping("/publish")
public Mono<Void> publish(@RequestBody String message) {
return Mono.fromRunnable(() -> sink.tryEmitNext(message).orThrow());
}
@GetMapping("/subscribe")
public Flux<String> subscribe() {
return sink.asFlux();
}
}
This code snippet makes it easier to manage multiple users subscribing and publishing messages concurrently.
Key Takeaways
Testing in Spring WebFlux can be challenging due to the asynchronous nature of reactive programming. However, with the right approach and tools like MockMvc
, you can comfortably write tests for your annotated controllers.
We explored various elements, including managing Mono and Flux, error handling, and concurrency management. To deepen your understanding of Spring WebFlux and its testing strategies, refer to the official Spring WebFlux Documentation and Testing Spring WebFlux Applications.
By harnessing Spring’s testing capabilities, you can ensure that your application not only meets functional requirements but also excels in addressing edge cases and concurrency challenges. Happy coding!