Common Errors When Converting Optional to ArrayList
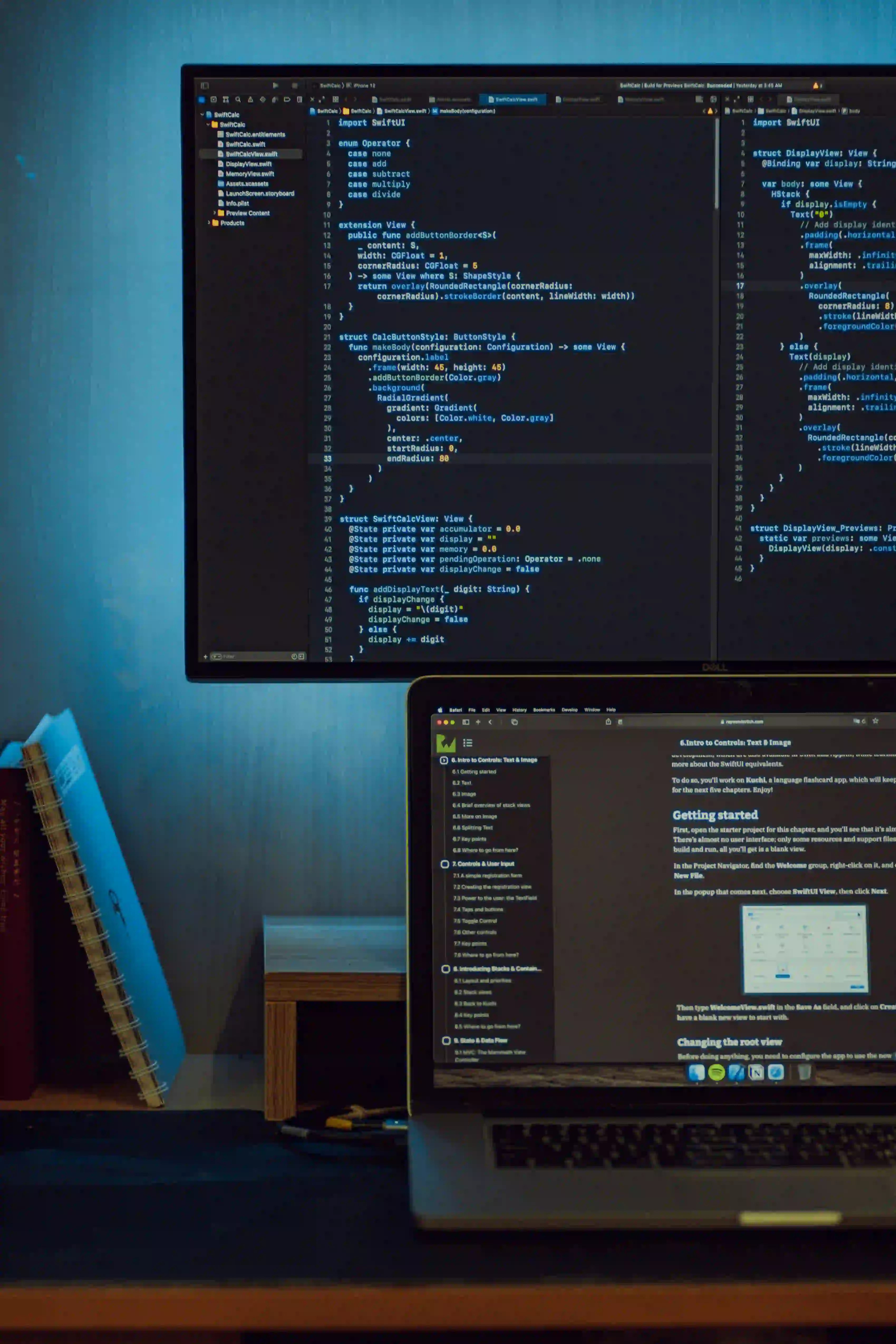
Common Errors When Converting Optional to ArrayList
In modern Java applications, using Optional
has become a popular practice for avoiding NullPointerException
and for better expressing the intent of the code. However, when developers begin to convert Optional
objects into collections like ArrayList
, they often run into common errors. In this post, we will explore the best practices for converting an Optional<T>
to an ArrayList<T>
, discuss typical pitfalls, and provide sample code to demonstrate proper implementation.
Understanding Optional
Before we dive into conversions, it is important to clarify what an Optional
is. Introduced in Java 8, Optional
is a container object which may or may not contain a non-null value. It provides methods to handle the presence and absence of values in a more readable manner.
Basic Structure of Optional
Optional<String> optionalValue = Optional.ofNullable(getStringValue());
The above code wraps a potentially null value in an Optional
. If the value is present, the Optional
contains it; if not, it remains empty.
Why Convert Optional to ArrayList
Often, scenarios arise where we need to collect values held in an Optional
into a list for further processing. For instance, if we are accumulating values from various Optional
instances, transforming them into an ArrayList
can help with batch processing.
Sample Implementation when Converting
Here’s a simple way to convert an Optional<T>
to an ArrayList<T>
:
import java.util.ArrayList;
import java.util.List;
import java.util.Optional;
public class Main {
public static void main(String[] args) {
Optional<String> optionalValue = Optional.of("Hello, World!");
// Convert Optional to ArrayList
List<String> list = new ArrayList<>();
optionalValue.ifPresent(list::add); // Adding value if present
System.out.println(list); // Outputs: [Hello, World!]
}
}
Explanation
- Creating an Optional: We create an
Optional<String>
with a string value. - Using
ifPresent
: The methodifPresent
executes the provided action (in this case, adding the value to the list) only if the value is present in theOptional
. - Output: The list now contains the string value if it was present.
Common Errors When Converting Optional to ArrayList
1. Not Handling the Absence of Values
One of the most common mistakes developers make is trying to directly extract the value without considering the absence scenario.
Optional<String> optionalValue = Optional.empty();
List<String> list = new ArrayList<>();
list.add(optionalValue.get()); // Throws NoSuchElementException
Better Approach
Instead, always check if the value is present:
if (optionalValue.isPresent()) {
list.add(optionalValue.get());
}
2. Using a Null Value for the List
Trying to add a value retrieved from an Optional
directly can lead to issues, especially if the list itself is not initialized.
Optional<String> optionalValue = Optional.of("Hello");
List<String> list = null; // Unintentionally designed to be null
list.add(optionalValue.get()); // Throws NullPointerException
Proper Initialization
Always ensure your list is initialized:
List<String> list = new ArrayList<>();
optionalValue.ifPresent(list::add);
3. Ignoring Optional's Functional Programming Methods
Using imperative styles to convert an Optional
to a list misses out on the benefits of functional programming.
List<String> list = new ArrayList<>();
optionalValue.ifPresent(value -> list.add(value)); // Works but is verbose
A Cleaner and More Functional Approach
You can streamline this operation using stream:
List<String> list = optionalValue.stream()
.collect(Collectors.toList());
This single line succinctly converts the Optional
to a list, handling the absence of values gracefully.
Converting a List of Optionals to an ArrayList
Working with collections of Optional
values can further complicate things. Here’s how to handle it efficiently:
Sample Code
import java.util.Arrays;
import java.util.List;
import java.util.Optional;
import java.util.stream.Collectors;
public class Main {
public static void main(String[] args) {
List<Optional<String>> listOfOptionals = Arrays.asList(
Optional.of("Hello"),
Optional.empty(),
Optional.of("World")
);
List<String> resultList = listOfOptionals.stream()
.flatMap(Optional::stream) // Convert Optional to Stream
.collect(Collectors.toList());
System.out.println(resultList); // Outputs: [Hello, World]
}
}
Explanation
- flatMap: The method
flatMap(Optional::stream)
is used here to flatten the stream ofOptional
to their contained values. If theOptional
is empty, it won’t contribute anything to the stream. - Collecting to List: Finally, we collect the values into an
ArrayList
usingcollect
method.
Lessons Learned
Converting Optional
to ArrayList
may seem straightforward, but common pitfalls abound. Understanding the nuances of Optional
data handling is key to writing robust Java applications.
Always ensure to:
- Handle the absence of values correctly.
- Initialize your lists before adding values.
- Utilize functional programming styles for cleaner, more readable code.
For more information on Optional
in Java, check the official Java Documentation and explore advanced features on Java streams here.
By embracing these practices, you can effectively manage Optional
conversions and enhance the quality of your Java applications. Happy coding!