Choosing the Right Big Data Framework for Java Projects
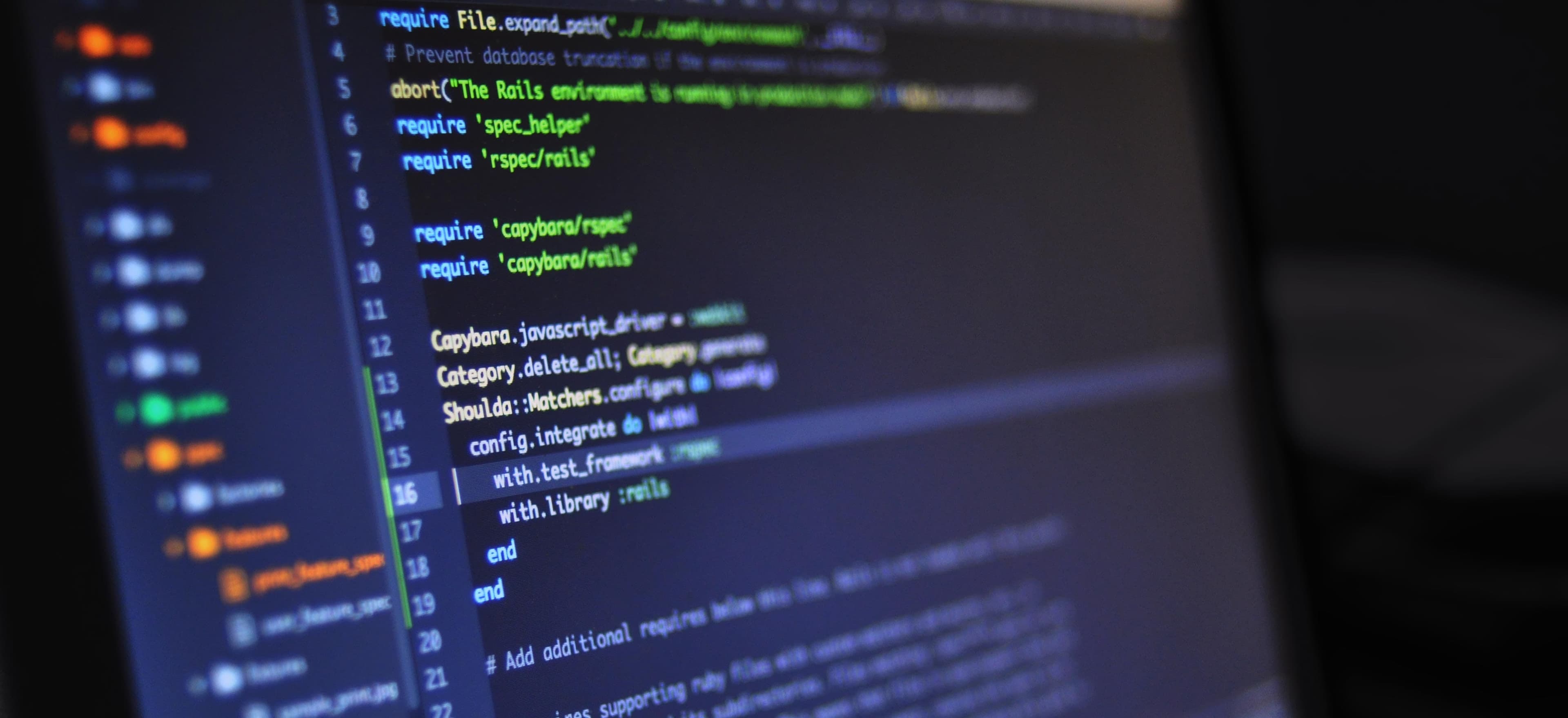
- Published on
Choosing the Right Big Data Framework for Java Projects
In today's data-driven world, the ability to process and analyze vast amounts of data is an asset that cannot be underestimated. For Java developers, selecting the right Big Data framework can significantly impact the success of your projects. This blog post will explore the top Big Data frameworks available for Java, providing insights into their strengths and weaknesses while guiding you on how to make an informed choice.
Why Big Data Matters
Big Data refers to the massive volume of structured, semi-structured, and unstructured data that inundates businesses daily. Leveraging Big Data effectively can lead to data insights that drive decision-making, optimize processes, and enhance customer experiences. According to a recent report, over 90% of the world's data was created in the past two years alone. Thus, having the right framework is essential for effectively managing and analyzing this data.
Top Big Data Frameworks for Java
Java has several robust frameworks suited for Big Data processing. Below, we delve into some of the most popular frameworks, exploring their merits and suitable applications.
1. Apache Hadoop
Apache Hadoop is one of the most widely adopted Big Data frameworks. It's an open-source software framework that enables distributed storage and processing of large datasets.
Key Features:
- Scalability: Easily scales from a single server to thousands of machines.
- Fault Tolerance: Replicates data across multiple nodes to ensure reliability.
- Flexible Data Processing: Supports various data processing models (Batch processing, real-time processing, etc.).
When to Use:
Hadoop is best suited for batch processing tasks, such as large data analytics. Its strength lies in its ability to handle massive datasets that require high-throughput data processing.
Basic Code Example:
Here’s a simple example of how to read a text file using Hadoop's MapReduce framework:
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.Path;
import org.apache.hadoop.io.LongWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Job;
import org.apache.hadoop.mapreduce.Mapper;
import org.apache.hadoop.mapreduce.Reducer;
import org.apache.hadoop.mapreduce.lib.input.FileInputFormat;
import org.apache.hadoop.mapreduce.lib.output.FileOutputFormat;
import java.io.IOException;
public class WordCount {
public static class TokenizerMapper extends Mapper<LongWritable, Text, Text, LongWritable> {
private final static LongWritable one = new LongWritable(1);
private Text word = new Text();
@Override
public void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
String[] words = value.toString().split("\\s+");
for (String w : words) {
word.set(w);
context.write(word, one);
}
}
}
public static class IntSumReducer extends Reducer<Text, LongWritable, Text, LongWritable> {
private LongWritable result = new LongWritable();
@Override
public void reduce(Text key, Iterable<LongWritable> values, Context context) throws IOException, InterruptedException {
long sum = 0;
for (LongWritable val : values) {
sum += val.get();
}
result.set(sum);
context.write(key, result);
}
}
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
Job job = Job.getInstance(conf, "word count");
job.setJarByClass(WordCount.class);
job.setMapperClass(TokenizerMapper.class);
job.setCombinerClass(IntSumReducer.class);
job.setReducerClass(IntSumReducer.class);
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(LongWritable.class);
FileInputFormat.addInputPath(job, new Path(args[0]));
FileOutputFormat.setOutputPath(job, new Path(args[1]));
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
}
Why This Code is Important:
- Mapper Function: Processes each line and emits a key-value pair where the key is the word and the value is 1.
- Reducer Function: Aggregates counts for each word, providing a total count for each unique word in the dataset.
For more information on Apache Hadoop, visit Apache Hadoop.
2. Apache Spark
Apache Spark is a unified analytics engine for big data processing, with built-in modules for streaming, SQL, machine learning, and graph processing.
Key Features:
- In-Memory Processing: Processes data in-memory, leading to faster execution times.
- Versatile: Suited for a variety of tasks, including batch processing, interactive queries, and streaming data.
- Rich API: Provides APIs in Java, Scala, Python, and R.
When to Use:
Spark is ideal for scenarios that require real-time data processing and analytics. It's particularly suitable for machine learning algorithms due to its ability to handle iterative tasks.
Basic Code Example:
Here’s an example of how to perform word count with Apache Spark in Java:
import org.apache.spark.SparkConf;
import org.apache.spark.api.java.JavaPairRDD;
import org.apache.spark.api.java.JavaRDD;
import org.apache.spark.api.java.JavaSparkContext;
import org.apache.spark.api.java.function.Function2;
import scala.Tuple2;
import java.util.Arrays;
import static org.apache.spark.api.java.function.Function.identity;
public class SparkWordCount {
public static void main(String[] args) {
SparkConf conf = new SparkConf().setAppName("WordCount");
JavaSparkContext sc = new JavaSparkContext(conf);
JavaRDD<String> lines = sc.textFile(args[0]);
JavaPairRDD<String, Integer> counts = lines
.flatMap(line -> Arrays.asList(line.split(" ")).iterator())
.mapToPair(word -> new Tuple2<>(word, 1))
.reduceByKey((Function2<Integer, Integer, Integer>) Integer::sum);
counts.saveAsTextFile(args[1]);
}
}
Why This Code is Important:
- FlatMap: Transforms each line into a sequence of words, allowing for flexibility in data parsing.
- ReduceByKey: Efficiently combines counts for each unique word, demonstrating Spark's ability to handle parallel operations.
To learn more about Apache Spark, check out Apache Spark.
3. Apache Flink
Apache Flink is another well-regarded framework known for its stream processing capabilities and high throughput.
Key Features:
- Stream Processing: Capable of processing events in real-time or in batch processing mode.
- Stateful Computation: Maintains the state of streaming data elements, crucial for applications like fraud detection.
- Integration: Works well with other data processing systems like Hadoop.
When to Use:
Flink is particularly suited for applications that require real-time processing and low-latency response, like monitoring systems or data-driven applications in financial services.
Basic Code Example:
Here's a simple Flink job that counts words in a text file:
import org.apache.flink.api.common.functions.FlatMapFunction;
import org.apache.flink.api.java.tuple.Tuple2;
import org.apache.flink.streaming.api.environment.StreamExecutionEnvironment;
import org.apache.flink.util.Collector;
public class FlinkWordCount {
public static void main(String[] args) throws Exception {
final StreamExecutionEnvironment env = StreamExecutionEnvironment.getExecutionEnvironment();
env.readTextFile(args[0])
.flatMap(new Tokenizer())
.keyBy(value -> value.f0)
.sum(1)
.writeAsText(args[1]);
env.execute("Word Count");
}
public static final class Tokenizer implements FlatMapFunction<String, Tuple2<String, Integer>> {
@Override
public void flatMap(String value, Collector<Tuple2<String, Integer>> out) {
String[] words = value.split("\\W+");
for (String word : words) {
if (word.length() > 0) {
out.collect(new Tuple2<>(word, 1));
}
}
}
}
}
Why This Code is Important:
- FlatMapFunction: Allows the transformation of input lines into key-value tuples while ensuring clean word extraction.
- KeyBy: Groups elements so that subsequent calculations, like sums, can be performed per word.
For more information on Apache Flink, visit Apache Flink.
4. Apache Kafka
While not a direct Big Data processing framework itself, Apache Kafka plays a crucial role in the Big Data ecosystem as a distributed streaming platform.
Key Features:
- High Throughput: Handles large volumes of real-time data streams.
- Scalability: Easily scales by adding new brokers to the cluster.
- Durability: Guarantees message delivery, ensuring data consistency.
When to Use:
Kafka is an excellent choice for building real-time data pipelines and streaming applications. Its integration with other frameworks like Spark and Flink increases its utility in Big Data scenarios.
Basic Code Example:
Here’s a simple example of producing messages to a Kafka topic using Java:
import org.apache.kafka.clients.producer.KafkaProducer;
import org.apache.kafka.clients.producer.ProducerRecord;
import org.apache.kafka.clients.producer.RecordMetadata;
import java.util.Properties;
public class SimpleProducer {
public static void main(String[] args) {
Properties props = new Properties();
props.put("bootstrap.servers", "localhost:9092");
props.put("key.serializer", "org.apache.kafka.common.serialization.StringSerializer");
props.put("value.serializer", "org.apache.kafka.common.serialization.StringSerializer");
KafkaProducer<String, String> producer = new KafkaProducer<>(props);
for (int i = 0; i < 10; i++) {
ProducerRecord<String, String> record = new ProducerRecord<>("my-topic", Integer.toString(i), "message-" + i);
producer.send(record, (RecordMetadata metadata, Exception exception) -> {
if (exception != null) {
exception.printStackTrace();
} else {
System.out.printf("Produced message to topic %s partition %d with offset %d%n",
metadata.topic(), metadata.partition(), metadata.offset());
}
});
}
producer.close();
}
}
Why This Code is Important:
- ProducerRecord: Represents one record for sending to a Kafka topic.
- Asynchronous Callback: Handles exceptions and confirms message delivery in an efficient manner.
To learn more about Apache Kafka, visit Apache Kafka.
A Final Look
Choosing the right Big Data framework is essential to the success of your Java projects. Apache Hadoop is ideal for batch processing, while Apache Spark and Flink cater to real-time data needs. At the same time, Kafka serves as an invaluable tool for streaming data applications. Having a solid understanding of these frameworks will enable you to harness the power of Big Data effectively.
Always assess your project requirements—data volume, processing requirements, and desired outcomes—before making a decision. By utilizing the right tools, you're a step closer to transforming raw data into actionable insights.
For additional readings on Big Data frameworks, check out the following resources:
Happy coding!
Checkout our other articles