Common Spring Data JPA Configuration Pitfalls to Avoid
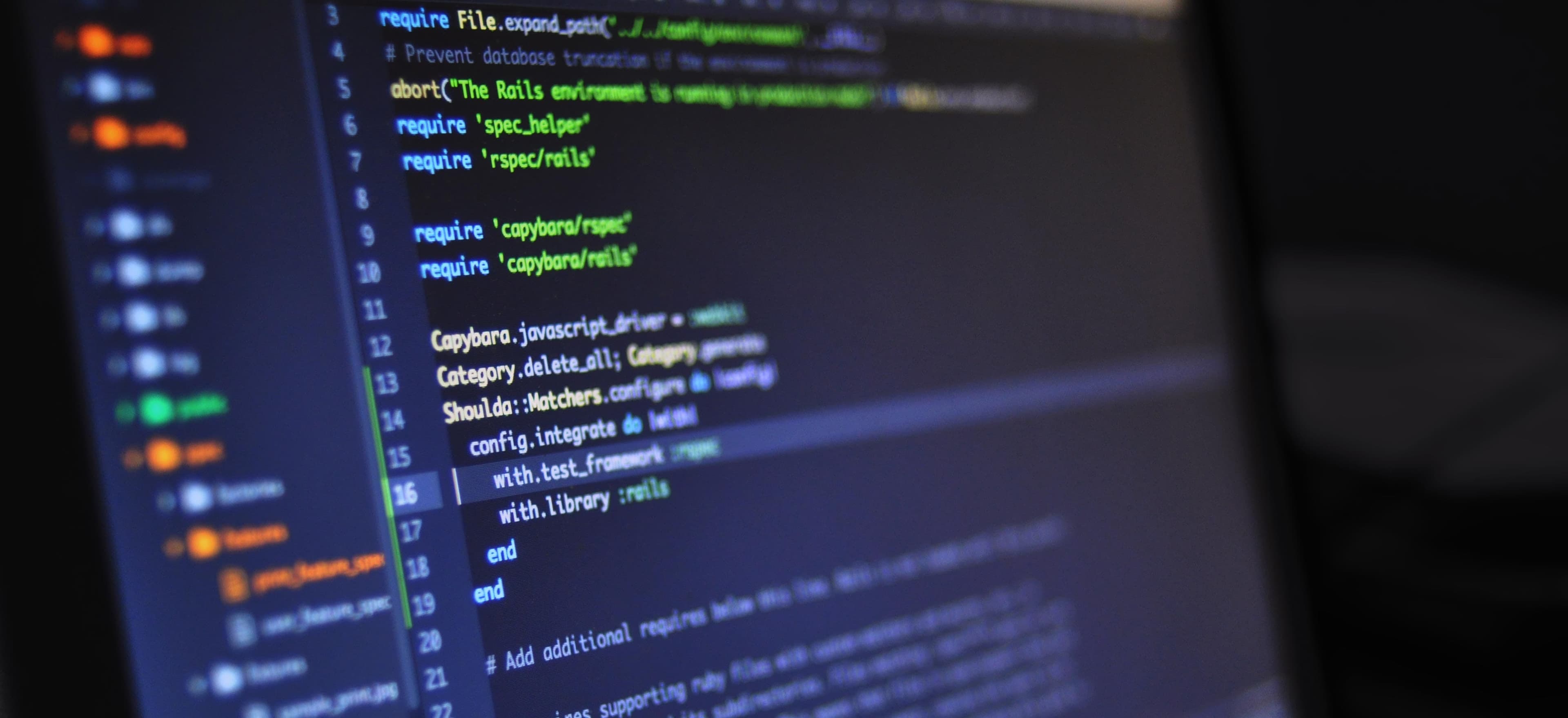
- Published on
Common Spring Data JPA Configuration Pitfalls to Avoid
Spring Data JPA is an incredibly powerful tool that simplifies database interactions in a Spring application. However, like any robust framework, it can lead to misconfigurations that may cause unexpected behavior if not properly understood. In this blog post, we will delve into some common pitfalls encountered while configuring Spring Data JPA and how to avoid them.
Understanding Spring Data JPA
Before we jump into the pitfalls, it’s essential to grasp what Spring Data JPA offers. At its core, Spring Data JPA is a part of the Spring Data project, designed to facilitate the implementation of JPA (Java Persistence API) based repositories. With its repository support, querying capabilities, and customization options, it enhances data Persistence while reducing boilerplate code.
Crafting Your Entity Class
Using JPA effectively often starts with your entity class. A common pitfall here is not properly annotating the class and fields.
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String username;
private String password;
// Constructors, getters, setters here
}
Why This Matters:
- @Entity annotation indicates that this class is a JPA entity and should be mapped to a database table.
- @Id marks the primary key field, and @GeneratedValue specifies how the primary key value should be generated.
Pitfall #1: Forgetting to Specify the Entity Name
By default, JPA will use the class name as the table name, but this can lead to issues if the table name in the database is different. To avoid confusion, always specify the table name in the entity.
@Entity
@Table(name = "users")
public class User { ... }
Pitfall #2: Neglecting Transactions
Spring Data JPA heavily relies on transaction management. Forgetting to manage transactions can cause data inconsistency issues. Ensure repository methods are annotated with @Transactional.
import org.springframework.transaction.annotation.Transactional;
import org.springframework.stereotype.Service;
@Service
public class UserService {
@Transactional
public User createUser(User user) {
return userRepository.save(user);
}
}
Why This Matters:
Without transactions, if an exception occurs, your database may end up in an inconsistent state, leading to potential data loss.
Pitfall #3: Not Correctly Configuring application.properties
Improper configuration in application.properties is another common issue faced by developers. A sample configuration should look something like this:
spring.datasource.url=jdbc:mysql://localhost:3306/testdb
spring.datasource.username=root
spring.datasource.password=yourpassword
spring.jpa.hibernate.ddl-auto=update
spring.jpa.show-sql=true
Why This Matters:
- spring.jpa.hibernate.ddl-auto determines how the database schema is created or updated.
- spring.jpa.show-sql allows you to view generated SQL, which is invaluable for debugging.
Pitfall #4: Poorly Defined Relationship Mappings
Another common oversight is incorrect relationship mappings between entities. In JPA, it is crucial to specify the correct relationships (OneToMany, ManyToOne, etc.) properly.
@Entity
public class Post {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@ManyToOne(fetch = FetchType.LAZY)
@JoinColumn(name = "user_id")
private User user;
// Other fields and methods...
}
Why This Matters:
Improper mapping can lead to LazyInitializationExceptions or incorrect data retrieval, which can complicate the debugging process.
Pitfall #5: Inadequate Exception Handling
Failing to implement proper exception handling can lead to critical issues during runtime. When working with JPA, it's essential to anticipate potential data access exceptions.
@Repository
public interface UserRepository extends JpaRepository<User, Long> {
Optional<User> findByUsername(String username);
}
@Service
public class UserService {
public User getUser(String username) {
return userRepository.findByUsername(username)
.orElseThrow(() -> new UsernameNotFoundException("User not found"));
}
}
Why This Matters:
Not handling exceptions properly can result in the application failing or producing unhelpful error messages. Always ensure you provide meaningful feedback.
Pitfall #6: Entity State Management
The persistence context in JPA manages the state of your entities, but it's easy to mismanage entity states. One issue is failing to detach entities when necessary.
If you find yourself not using an entity after retrieval, you can detach it with:
entityManager.detach(user);
Why This Matters:
Not managing your entities' states can lead to memory leaks or inconsistent data states in complex applications.
Pitfall #7: Inefficient Fetching Strategies
It's crucial to select appropriate fetching strategies to avoid performance issues. Using FetchType.LAZY can help reduce the amount of data retrieved, which is efficient when handling large datasets or complex entity relationships.
@ManyToMany(fetch = FetchType.LAZY)
private Set<Role> roles;
Why This Matters:
Choosing the wrong fetch strategy can lead to N+1 select problems, severely affecting performance.
Best Practices for Spring Data JPA Configuration
While avoiding pitfalls is essential, implementing best practices can enhance your experience with Spring Data JPA. Here are a few to consider:
-
Utilize Projections: Use projections to fetch only the data you need.
-
Leverage Spring Data JPA Specifications: Use specification support to create reusable query conditions.
-
Keep Your Repositories Independent: Minimize dependencies in your repository layer to decrease complexity.
-
Contextualize Queries: Use derived queries wisely; they can make the code cleaner and easier to understand.
-
Regularly Review Your Database Schema: Database changes can have ripple effects through your application, so it’s good practice to review.
For a deeper understanding of these concepts, you can refer to the official Spring Data JPA documentation and the Java Persistence API (JPA).
In Conclusion, Here is What Matters
Spring Data JPA provides powerful capabilities for managing relational data, but misconfigurations can lead to problematic behaviors in your application. By recognizing the common pitfalls outlined in this post and adhering to best practices, you can ensure a more stable, maintainable, and efficient data access layer.
Understanding how to effectively leverage Spring Data JPA will undoubtedly enhance your application’s capability to interact with data efficiently and effectively. Happy coding!