Boosting Code Coverage in Eclipse: Common Pitfalls to Avoid
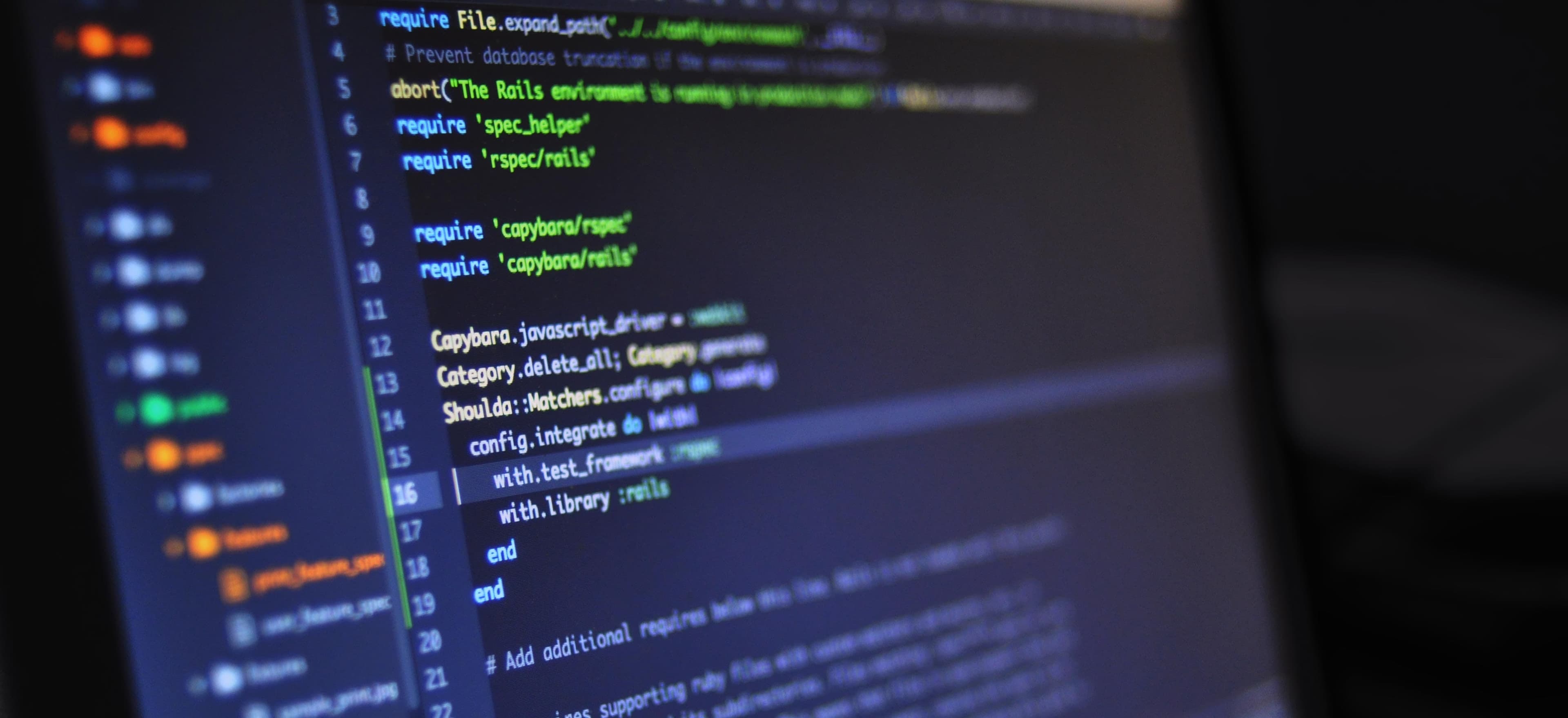
- Published on
Boosting Code Coverage in Eclipse: Common Pitfalls to Avoid
In the realm of software development, ensuring high code coverage is essential. Code coverage is a metric that reflects the percentage of your source code that is executed during testing. Higher coverage often suggests better tested code and thus minimizes unexpected bugs in production. While Eclipse is a powerful Integrated Development Environment (IDE) that offers extensive support for Java development, developers often encounter pitfalls when attempting to enhance code coverage. In this post, we will explore common pitfalls and how to effectively boost your code coverage in Eclipse.
Understanding Code Coverage in Eclipse
Before diving into the common pitfalls, it is essential to understand what code coverage means in the context of Eclipse. Code coverage tools in Eclipse, such as EclEmma, allow developers to visualize which parts of their code are tested and which are not.
Why Code Coverage Matters
- Quality Assurance: High code coverage often leads to fewer bugs and more reliable software.
- Refactoring Confidence: High coverage ensures that changes or optimizations can be made safely.
- Documentation: Code coverage reports can serve as a form of documentation to indicate tested functionality.
Setting Up EclEmma in Eclipse
Before we tackle the pitfalls, let's ensure you have EclEmma set up properly.
- Install EclEmma: You can install it from the Eclipse Marketplace. Go to
Help
>Eclipse Marketplace
, search for EclEmma, and install it. - Run Your Tests: After installation, you can run your JUnit tests by clicking on the Coverage As > JUnit Test option.
Example: Getting Coverage Data
Once you run your tests with coverage, EclEmma will generate a coverage report. You will see colored metrics on your source code:
- Green: Lines executed (covered).
- Yellow: Partially covered (conditional statements).
- Red: Not executed.
This visual representation helps you identify areas that require further testing.
Common Pitfalls to Avoid
1. Ignoring Code Complexity
It's easy to think that just writing tests for every method will suffice. However, complex code structures often impede code coverage.
Solution:
- Break down complex methods into smaller, more manageable units.
- Utilize design patterns such as Strategy or Decorator to enhance modularity.
Code Snippet:
// Original Complex Method
public void processOrder(Order order) {
if (order.validate()) {
chargeCustomer(order);
sendConfirmation(order);
} else {
throw new InvalidOrderException();
}
}
Why Restructure? By isolating the validation process:
public void processOrder(Order order) {
OrderValidator validator = new OrderValidator();
if (validator.validate(order)) {
processValidatedOrder(order);
} else {
throw new InvalidOrderException();
}
}
private void processValidatedOrder(Order order) {
chargeCustomer(order);
sendConfirmation(order);
}
2. Overreliance on Coverage Metrics
While high coverage metrics are desirable, they don't guarantee quality. Achieving 100% code coverage is unrealistic and could lead to false security.
Solution:
- Use code coverage to guide your testing efforts. Aim for a balance between coverage and meaningful tests.
- Periodically review tests to ensure they validate functionality rather than merely executing code.
3. Failing to Test Edge Cases
When focusing on the mainstream functionality, developers often neglect edge cases, which can lead to significant issues in production.
Solution:
- Always consider edge cases when writing tests. For example, what happens if the input is null or the boundary values are reached?
Code Snippet:
// Method without edge case testing
public int divide(int a, int b) {
return a / b; // What if b is 0?
}
Why Test Edge Cases? Incorporating edge cases will yield:
public int divide(int a, int b) {
if (b == 0) {
throw new ArithmeticException("Divisor cannot be zero");
}
return a / b;
}
4. Not Leveraging Testing Frameworks
If you are not using a robust testing framework like JUnit or TestNG, this could hinder your coverage improvement efforts.
Solution:
- Utilize frameworks that support parameterized tests. This allows you to run multiple scenarios with minimal code duplication.
Code Snippet:
Using JUnit Parameterized tests:
@RunWith(Parameterized.class)
public class DivisionTest {
private int dividend;
private int divisor;
private int expectedResult;
public DivisionTest(int dividend, int divisor, int expectedResult) {
this.dividend = dividend;
this.divisor = divisor;
this.expectedResult = expectedResult;
}
@Parameterized.Parameters
public static Collection<Object[]> data() {
return Arrays.asList(new Object[][] {
{ 6, 3, 2 },
{ 15, 5, 3 },
{ 0, 1, 0 }
});
}
@Test
public void testDivision() {
assertEquals(expectedResult, divide(dividend, divisor));
}
}
5. Not Reviewing and Refactoring Tests
Often, as the codebase grows, tests can become stale or irrelevant. Failing to review and refactor test cases can lead to coverage dip.
Solution:
- Regularly conduct code reviews focused on tests.
- Refactor and update all dependencies in tests to match the current code structure.
Final Considerations
Boosting code coverage in Eclipse, while avoiding common pitfalls, is an ongoing process that requires diligence. Remember, higher code coverage should improve the quality of your software and not merely serve as a metric. Examine your code for complexity, test edge cases, leverage frameworks, and focus on the quality of your tests in relation to the code.
Additional Resources
- For more in-depth knowledge on code coverage metrics, you can visit Codecov Blog where they provide valuable insights.
- If you're looking for good JUnit testing practices, check out JUnit 5 User Guide.
By employing these strategies and avoiding common pitfalls, you can significantly enhance your code coverage in Eclipse and contribute to a more robust software development process. Happy coding!