ThreadLocal Pitfalls: Avoiding Common Mistakes
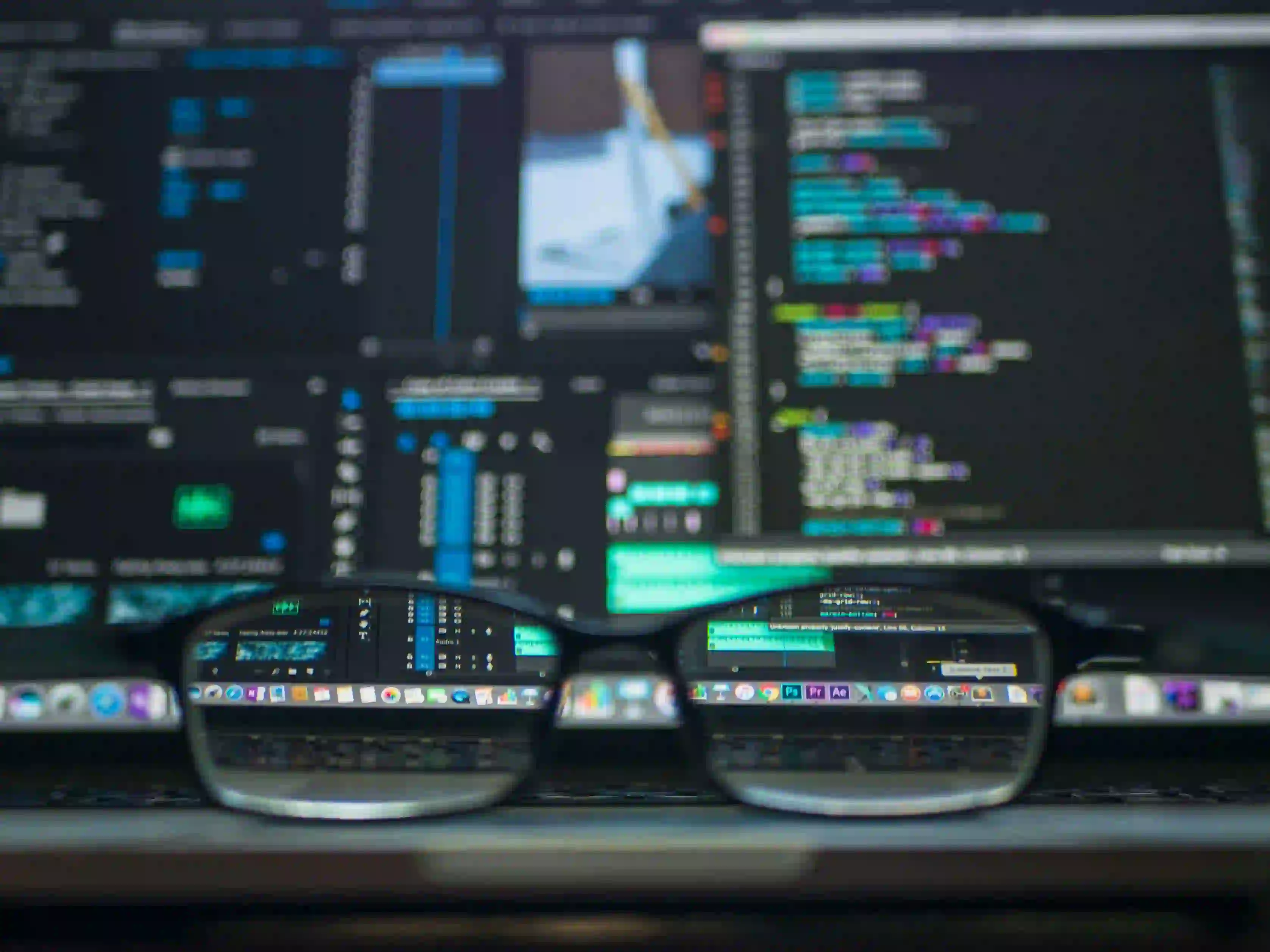
ThreadLocal Pitfalls: Avoiding Common Mistakes
When working in a multithreaded environment, managing state and data consistency is crucial. One of the tools offered by Java to manage thread-specific data is the ThreadLocal
class. While it can provide solutions to common problems like data isolation, it also opens the door to a distinct set of pitfalls. This blog post will explore these potential drawbacks and how to avoid them, ensuring that you utilize ThreadLocal
effectively in your Java applications.
What is ThreadLocal?
ThreadLocal
is a class in Java that allows you to create thread-local variables. Each thread accessing a ThreadLocal
variable has its own, independently initialized copy of the variable. This means that changes made by one thread do not affect the value seen by other threads.
ThreadLocal<Integer> threadLocalValue = ThreadLocal.withInitial(() -> 1);
In this code snippet, we create a ThreadLocal
variable, initializing it to 1 for each thread accessing it. This encapsulation of state can help avoid concurrency issues—in theory. However, improper use of ThreadLocal
can lead to memory leaks, unintended data retention, and difficulty in debugging.
Common Pitfalls
1. Not Removing Values
One of the most common mistakes is failing to remove values from the ThreadLocal
. Since the variables are tied to the lifespan of the thread, if a thread is reused (as is common in thread pools), stale values might linger.
try {
threadLocalValue.set(10);
// Perform operations
} finally {
threadLocalValue.remove(); // Always remove when done
}
Why? When values are not removed, they remain in memory, potentially leading to memory leaks, especially in long-running applications. This can be avoided by always enclosing your ThreadLocal
usage in a try-finally
block to ensure the removal.
2. Misunderstanding Scope
ThreadLocal
variables are scoped to the individual thread. If you mistakenly assume that modifying a ThreadLocal
variable in one thread would impact the value seen by another thread, you may introduce bugs.
ThreadLocal<String> userContext = ThreadLocal.withInitial(() -> "Guest");
new Thread(() -> {
userContext.set("User1");
System.out.println(userContext.get()); // Outputs User1
}).start();
System.out.println(userContext.get()); // Outputs Guest
Why? This misunderstanding leads to assumptions about shared mutable state. Always be clear on the scope of your ThreadLocal
variables and their implications on your application’s threading model.
3. Overusing ThreadLocal
While ThreadLocal
can be useful, overusing it can complicate your application’s architecture. Using it for every single thread-specific variable can lead to unnecessary complexity.
ThreadLocal<Double> price = ThreadLocal.withInitial(() -> 0.0);
ThreadLocal<String> orderStatus = ThreadLocal.withInitial(() -> "Pending");
Why? Introducing many ThreadLocal
instances can make debugging difficult and impact performance due to excessive context-switching overhead. Reserve ThreadLocal
for scenarios where you genuinely need thread-specific storage, such as session or request context data.
4. Failure to Document
Given that ThreadLocal
can lead to complex interactions within your codebase, failing to document its usage can lead to confusion for other developers.
/**
* ThreadLocal variable to store the user context for the current session.
* This should be removed after use to prevent memory leaks.
*/
private static final ThreadLocal<UserContext> userContextThreadLocal = ThreadLocal.withInitial(UserContext::new);
Why? Clear documentation minimizes misinterpretation. Providing context about why you're using ThreadLocal
, what it stores, and how to manage its lifecycle is beneficial for anyone maintaining the code.
5. Inadvertent Recycling of Threads
When using thread pools from ExecutorService
, threads can be recycled, leading to unintentional access to ThreadLocal
variables initialized in older tasks.
ExecutorService executor = Executors.newFixedThreadPool(2);
executor.submit(() -> {
threadLocalValue.set("Value1");
});
executor.submit(() -> {
System.out.println(threadLocalValue.get()); // Could yield unexpected results
});
Why? This might not throw an error, but it can lead to unintended behavior where one task reads state from another. Ensure that you clean up your ThreadLocal
variables after each task execution.
Best Practices for Using ThreadLocal
Use with Care
Employ ThreadLocal
only when necessary. Assess alternatives like using method parameters or design patterns such as Dependency Injection or Builder patterns.
Always Remove
Conclude your ThreadLocal
usage with remove()
to eliminate stale data challenges and prevent memory leaks.
Limit Scope
Restrict the use of ThreadLocal
in classes that are going to be executed in a full-stack capacity - keep it local.
Document Thoroughly
Make it a standard to document any ThreadLocal
instances. Explain their purpose, lifespan, and data management behavior.
To Wrap Things Up
ThreadLocal
offers an excellent way to manage thread-specific variables in Java, but it should be used wisely. Awareness of its drawbacks—from memory management to unexpected data retention—can empower you to leverage its capabilities effectively. By exercising caution and adopting best practices, you can avoid common pitfalls associated with ThreadLocal
and enhance the robustness of your multithreaded applications.
For a deeper dive into multithreading in Java, consider reading Java Concurrency in Practice for comprehensive insights on concurrency concepts.
For additional resources on best practices involving multithreading and pattern implementations, check out this Java Concurrency tutorial.
Happy coding!