Common Docker Pitfalls for Java Developers to Avoid
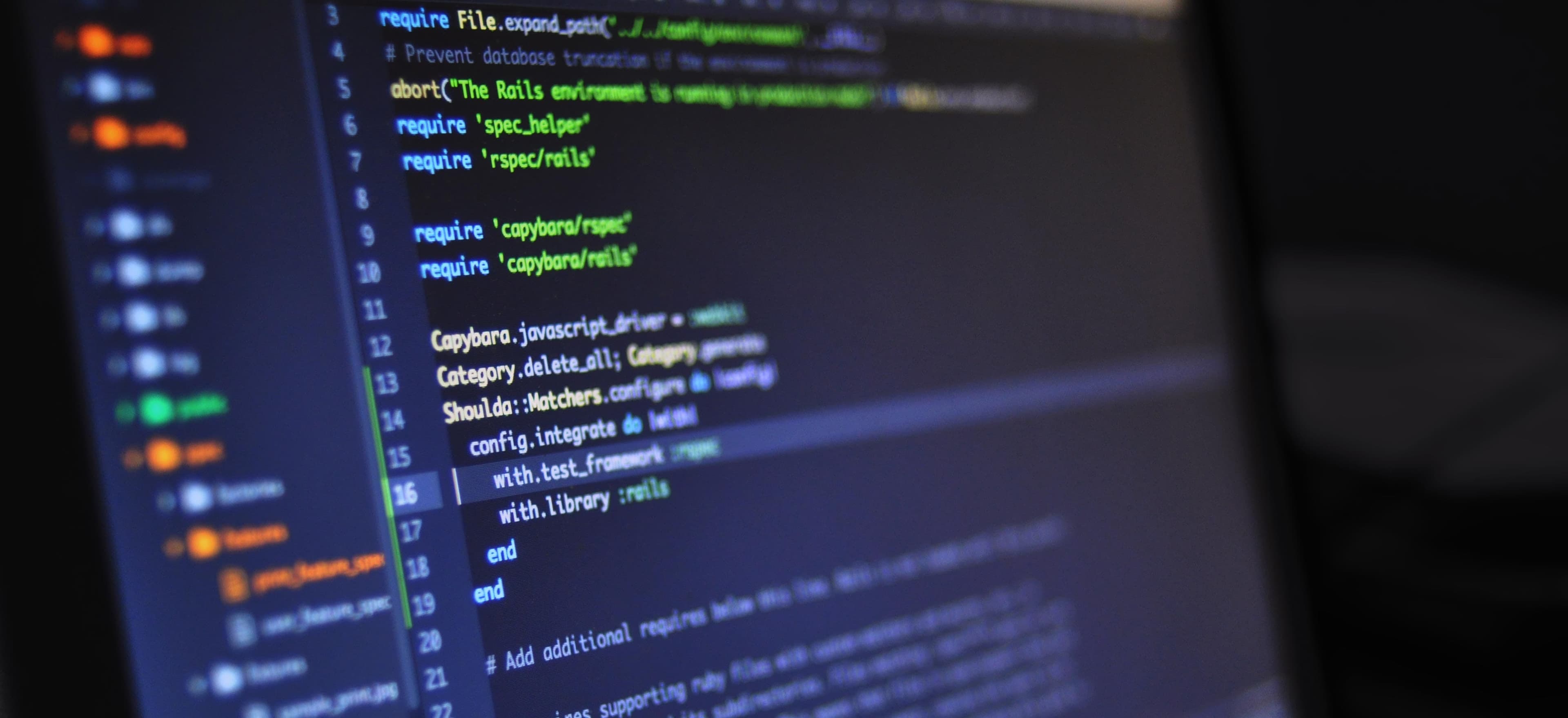
- Published on
Common Docker Pitfalls for Java Developers to Avoid
Docker has transformed the way developers build, share, and run applications. Particularly in the realm of Java development, it's crucial to recognize best practices. However, it’s also essential to be aware of the common pitfalls that can lead to performance issues, security vulnerabilities, or even a failed deployment. In this blog post, we'll explore these pitfalls while providing practical solutions and code snippets to help Java developers make the most of Docker.
Understanding Docker Basics
Before diving into pitfalls, let's clarify what Docker is. Docker is a containerization platform, providing developers with a method to package applications and their dependencies into a standardized unit called a container. These containers are lightweight, easy to manage, and can run anywhere that Docker is installed.
Why Use Docker for Java Development?
- Isolation: Docker containers encapsulate everything that is needed to run the application.
- Consistency: The application will behave the same with the same configurations across different environments.
- Scalability: You can easily scale applications by launching multiple containers.
Pitfall 1: Not Using Multi-Stage Builds
One of the most common oversights is failing to leverage Docker's multi-stage builds. This feature allows you to separate the build environment from the final production image, significantly reducing the image size.
Example of Multi-Stage Build
# Use a specific version of the JDK for building the application
FROM openjdk:17-jdk-alpine AS build
# Set the working directory
WORKDIR /app
# Copy the build files
COPY . .
# Build the application using Maven
RUN ./mvnw clean package
# Use a smaller image for production
FROM openjdk:17-jre-alpine
# Copy the JAR from the build stage
COPY --from=build /app/target/myapp.jar /app/myapp.jar
# Command to run the application
ENTRYPOINT ["java", "-jar", "/app/myapp.jar"]
Why This Matters: By using multi-stage builds, you eliminate unnecessary dependencies and files from the final image, making it lighter and faster to pull. Read more on Docker multi-stage builds for additional context.
Pitfall 2: Ignoring Dockerfile Best Practices
Your Dockerfile
can make or break your application. Here are some critical best practices:
- Use official base images whenever possible.
- Minimize the number of layers by combining commands.
- Specify the exact version of dependencies to avoid surprises.
Example of an Optimized Dockerfile
FROM openjdk:17-jre-alpine
WORKDIR /app
# Combine RUN statements to reduce layers
COPY pom.xml ./
RUN ./mvnw dependency:go-offline
COPY src ./src
# Build the JAR file
RUN ./mvnw package
COPY target/myapp.jar /app/myapp.jar
ENTRYPOINT ["java", "-jar", "/app/myapp.jar"]
Why This Matters: Following best practices ensures that your images are smaller, safer, and easier to maintain. For further reading, check out the official Dockerfile best practices.
Pitfall 3: Failing to Separate Application Logic from Configuration
When running a Java application in a Docker container, you might make the mistake of hardcoding configuration values. Instead, consider using environment variables or external configuration files.
Example of Using Environment Variables
# Dockerfile
FROM openjdk:17-jre-alpine
WORKDIR /app
COPY target/myapp.jar /app/myapp.jar
# Set environment variables
ENV DB_HOST=localhost
ENV DB_PORT=3306
ENV DB_USER=user
ENV DB_PASS=pass
ENTRYPOINT ["java", "-jar", "/app/myapp.jar"]
In your Java application, you would retrieve these variables seamlessly:
String dbHost = System.getenv("DB_HOST");
String dbPort = System.getenv("DB_PORT");
Why This Matters: Separating configuration from application code enhances flexibility. You can change configurations without modifying the codebase and redeploying. For additional insights, you can explore this guide on 12-factor app principles.
Pitfall 4: Using a Single Container for Multiple Services
Many developers mistakenly try to run multiple services within a single Docker container. This violates the microservices principle. Each container should ideally run a single responsibility.
Recommended Approach
Instead of combining multiple services in a single container, consider using Docker Compose:
version: '3'
services:
app:
build: .
ports:
- "8080:8080"
db:
image: mysql:5.7
environment:
MYSQL_ROOT_PASSWORD: example
Why This Matters: By using Docker Compose, you create a scalable, maintainable application architecture where each service can be scaled independently. Learn more about Docker Compose for effective service management.
Pitfall 5: Neglecting Container Security
Security should never be an afterthought. Here are key practices to keep your container secure:
- Use the least privileged user to run your application.
- Regularly update your base images and avoid outdated libraries.
- Utilize tools such as Docker Bench security for best practices.
Running a Container with a Non-Root User
FROM openjdk:17-jre-alpine
RUN addgroup -S app && adduser -S app -G app
USER app
WORKDIR /app
COPY target/myapp.jar /app/myapp.jar
ENTRYPOINT ["java", "-jar", "/app/myapp.jar"]
Why This Matters: Running containers as non-root users minimizes potential damage in case of a breach. For more information on securing your Docker containers, refer to the Docker security documentation.
Pitfall 6: Neglecting the Importance of Logs
Failing to capture logs in a structured and accessible manner can severely hamper debugging efforts. Lightweight logging frameworks can help to facilitate cloud logging.
Example Code for Logging
If you are using SLF4J with Logback, for example, your Docker configuration could involve defining a logging configuration that routes logs appropriately.
# logback-spring.xml
<configuration>
<appender name="CONSOLE" class="ch.qos.logback.core.ConsoleAppender">
<encoder>
<pattern>%d{yyyy-MM-dd HH:mm:ss} - %msg%n</pattern>
</encoder>
</appender>
<root level="INFO">
<appender-ref ref="CONSOLE"/>
</root>
</configuration>
Why This Matters: Centralized logging allows for better monitoring and quicker responses to issues. Docker’s logs can be accessed easily via the command line, using docker logs <container_id>
. For more information on logging in Docker, check out Docker logging docs.
The Bottom Line
Dockerization can significantly enhance the way Java developers deploy their applications, but caution must be exercised to avoid common pitfalls. Remember to:
- Utilize multi-stage builds.
- Follow Dockerfile best practices.
- Separate configuration from application logic.
- Avoid embedding multiple services in a single container.
- Prioritize container security.
- Implement robust logging mechanisms.
By navigating through these pitfalls, Java developers can build efficient, secure, and maintainable applications using Docker.
For additional resources, consider exploring the official Docker documentation and Maven documentation to deepen your understanding of integrating Java with Docker effectively.
With this knowledge, you're now better prepared to take full advantage of Docker in your Java development practices. Happy coding!
Checkout our other articles