Troubleshooting AnimationListener Not Firing in Android
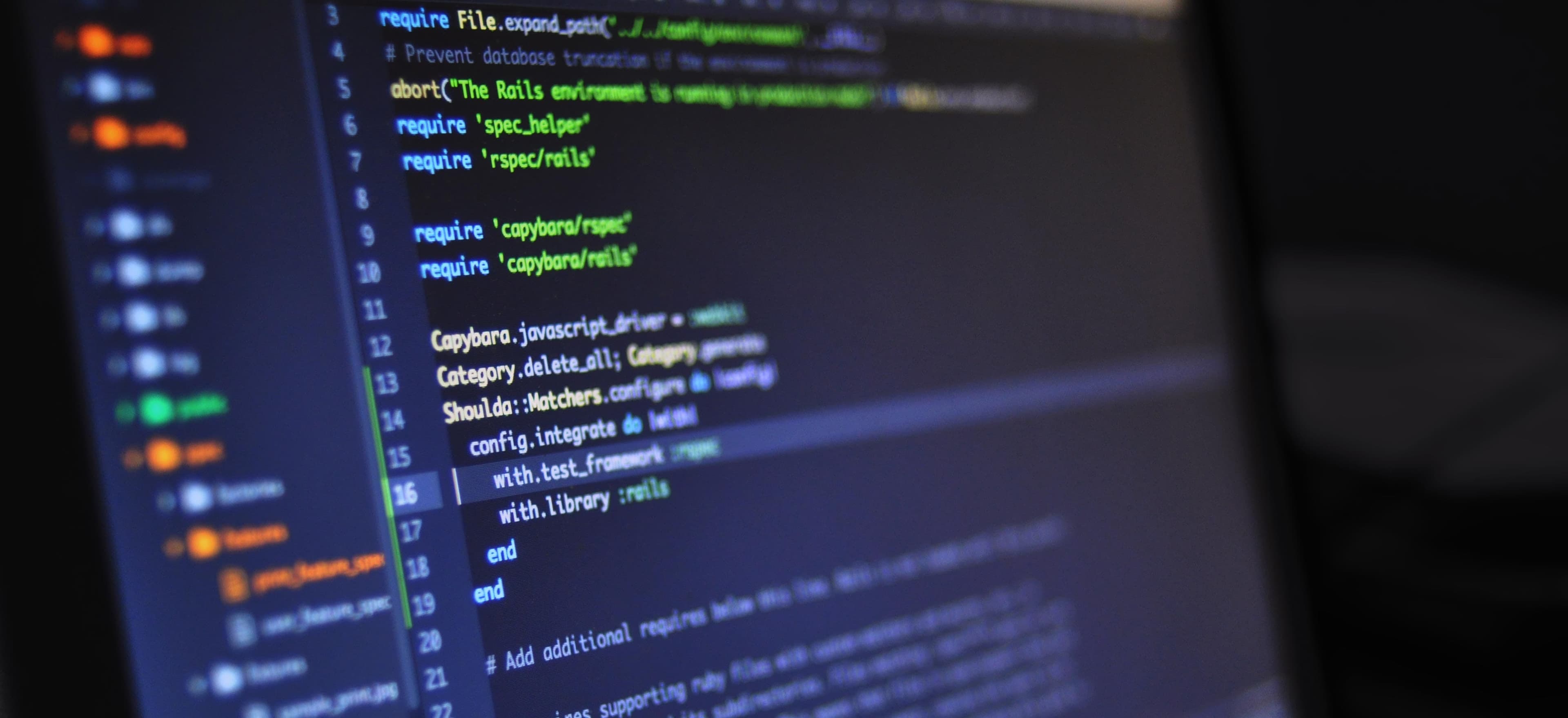
- Published on
Troubleshooting AnimationListener Not Firing in Android
Animation plays a pivotal role in modern Android applications, providing a more engaging user experience. Developers often rely on listener callbacks, such as AnimationListener
, to handle events when animations start, end, or repeat. However, some developers have encountered an issue where the AnimationListener
does not fire as expected. This blog post dives into troubleshooting this problem and ensures that your animations behave as intended.
Understanding Animation in Android
Before we tackle the troubleshooting process, let’s clarify what an AnimationListener
is and how it works. In Android, animations can be managed using the Animation
class. An AnimationListener
can be attached to an animation object, allowing you to track the animation's lifecycle events. Here’s a simple listener setup:
Animation animation = new AlphaAnimation(0f, 1f);
animation.setDuration(1000);
animation.setAnimationListener(new Animation.AnimationListener() {
@Override
public void onAnimationStart(Animation animation) {
Log.d("AnimationListener", "Animation Started");
}
@Override
public void onAnimationEnd(Animation animation) {
Log.d("AnimationListener", "Animation Ended");
}
@Override
public void onAnimationRepeat(Animation animation) {
Log.d("AnimationListener", "Animation Repeated");
}
});
This code initializes a fade-in animation and attaches listeners that log messages to the console.
Common Issues with AnimationListener
When you notice that the AnimationListener
is not firing, consider the following potential issues:
1. Animation Not Being Started
It may sound simple, but ensuring that the animation actually starts is a fundamental step. If you forget to call start()
, the listener won't trigger. Always check your flow:
view.startAnimation(animation);
2. Animation Being Interrupted
Animations can be interrupted by other animations or changes in the UI. If another animation is started on the same view before the first animation finishes, it can cause the listener not to be notified.
3. View Replacement or Removal
If the target view is removed from the view hierarchy while the animation is running, the listener will not fire because the Animation Lifecycle is bound to the view's lifecycle. Ensure that the view remains intact throughout the animation.
4. Animation Not Fully Attached
Confirm that your animation is properly attached to the view. If you create an animation but never set it on a view, the listener won't activate:
view.clearAnimation(); // Clears any existing animations before starting
view.startAnimation(animation);
Debugging Steps
If none of the above suggestions seem to work, follow these debugging steps:
Step 1: Verify Animation Object
Check that you are attaching the listener to the correct animation object. If you inadvertently create a new animation instance without a listener, it will not respond as expected.
Step 2: Check for Conflicting Codes
Review other parts of your code that may interact with animations. Look out for:
- Multiple animations targeting the same view.
- View visibility changes during the animation.
Step 3: Utilize Logs and Breakpoints
Insert logs in your listener methods, as shown earlier, and use breakpoints in your IDE to check if the code enters the listener functions when you expect it to. For instance:
@Override
public void onAnimationEnd(Animation animation) {
Log.d("AnimationListener", "animation ended");
// Additional logic could be placed here
}
Example: Firing AnimationListener Correctly
Here is a complete example that demonstrates the proper use of an AnimationListener
in an Android activity.
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
final ImageView imageView = findViewById(R.id.imageView);
Animation fadeIn = new AlphaAnimation(0.0f, 1.0f);
fadeIn.setDuration(2000);
fadeIn.setAnimationListener(new Animation.AnimationListener() {
@Override
public void onAnimationStart(Animation animation) {
Log.d("AnimationListener", "Fade In Started");
}
@Override
public void onAnimationEnd(Animation animation) {
Log.d("AnimationListener", "Fade In Ended");
}
@Override
public void onAnimationRepeat(Animation animation) {
Log.d("AnimationListener", "Fade In Repeated");
}
});
imageView.setOnClickListener(view -> {
imageView.startAnimation(fadeIn);
});
}
}
Resources
-
If you want to learn more about different types of animations in Android, consider checking out the official Android documentation on Animation.
-
To delve further into UI best practices for animations in Android, refer to Material Design guidelines.
Lessons Learned
Animation is an essential tool for enhancing the user experience in Android applications. When your AnimationListener
doesn't trigger, it often leads to confusion. By understanding how animations work, ensuring that all steps in the animation lifecycle are followed, and employing debugging techniques, you can successfully troubleshoot these issues.
Don’t forget to keep the user experience in mind while designing animations. Good luck with your Android projects, and happy coding!
Checkout our other articles