Mastering JUnit Testing for Spring MVC Controllers: Common Pitfalls
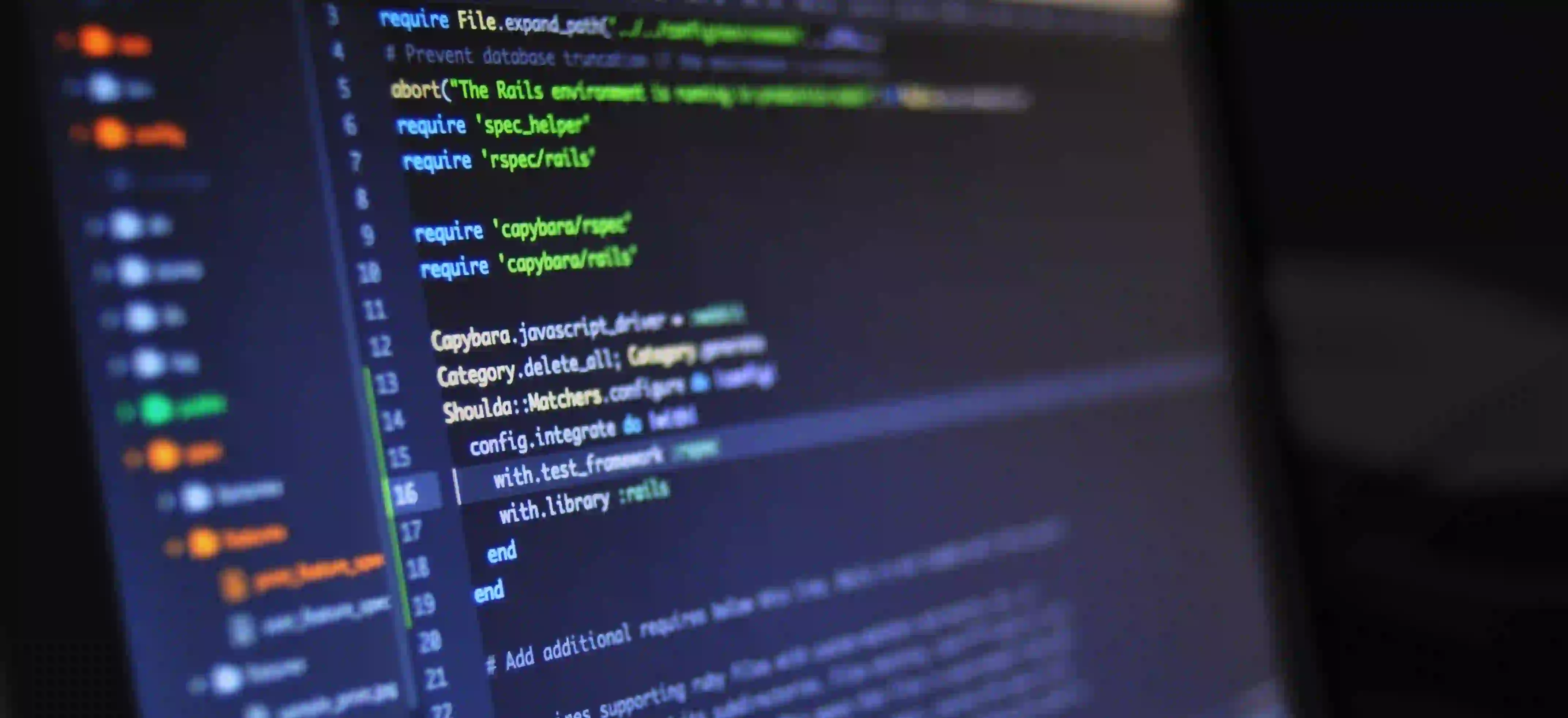
Mastering JUnit Testing for Spring MVC Controllers: Common Pitfalls
JUnit testing is an essential skill for any Java developer, particularly when developing applications using Spring MVC. Mastering JUnit testing for Spring MVC controllers can elevate your code quality and ensure your application behaves as expected under various scenarios. However, pitfalls abound, and new developers can quickly lose their way amidst the complexities of testing. In this post, we will discuss common pitfalls while providing insights into effective JUnit testing strategies. Let's dive in!
Understanding Spring MVC Controllers
Before we dive into the details of JUnit testing, it's important to understand what Spring MVC controllers are and their role within an application. Spring MVC (Model-View-Controller) is an architecture used to develop web applications in a clean and maintainable way. Controllers in Spring MVC handle incoming requests, delegate tasks to services, and determine the appropriate view to render.
Here’s a simple Spring MVC controller to illustrate the concepts:
@Controller
public class UserController {
@Autowired
private UserService userService;
@GetMapping("/users/{id}")
public ResponseEntity<User> getUserById(@PathVariable Long id) {
User user = userService.findById(id);
return ResponseEntity.ok(user);
}
}
In the code above, UserController
handles GET requests for user details, utilizing a service class to fetch user data.
Setting Up JUnit with Spring MVC
To effectively test Spring MVC controllers, you'll need to include JUnit and some Spring testing libraries in your project. If you're using Maven, include the following dependencies in your pom.xml
:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
This starter brings JUnit, AssertJ, and Mockito among others, creating an efficient testing environment.
Common Pitfalls in JUnit Testing for Spring MVC Controllers
1. Not Isolating the Controller
Many developers make the mistake of not isolating the controller from its dependencies, which leads to tests that are hard to maintain and debug.
Why?
When you test controllers with their dependencies, you're not truly testing the controller functionality. You're testing the entire integration.
Solution:
Use mocking frameworks like Mockito to create mocks of your service layer.
Here’s how you can isolate the controller:
@RunWith(SpringRunner.class)
@WebMvcTest(UserController.class)
public class UserControllerTest {
@Autowired
private MockMvc mockMvc;
@MockBean
private UserService userService;
@Test
public void testGetUserById() throws Exception {
User user = new User(1L, "John Doe");
when(userService.findById(1L)).thenReturn(user);
mockMvc.perform(get("/users/{id}", 1L))
.andExpect(status().isOk())
.andExpect(jsonPath("$.name").value("John Doe"));
}
}
In this code:
- We use
@MockBean
to create a mock ofUserService
, allowing theUserController
to be tested independently.
2. Ignoring HTTP Status Codes
Another common pitfall is not checking the correct HTTP status codes returned by the controllers. Each controller method should respond with suitable HTTP status codes.
Why?
Failing to verify HTTP statuses can result in undetected errors in handling various situations.
Solution:
Always assert the expected HTTP status in each test.
Continuing from our previous example, we can modify it to check for the correct status code:
@Test
public void testGetUserById_ShouldReturnNotFound() throws Exception {
when(userService.findById(2L)).thenThrow(new UserNotFoundException());
mockMvc.perform(get("/users/{id}", 2L))
.andExpect(status().isNotFound());
}
This test checks that when a user is not found, we return a 404 HTTP response, ensuring that our application provides meaningful error handling.
3. Simplifying Complex Scenarios
Many developers tend to overlook more complex scenarios, focusing instead on the happy paths (the scenario where everything works perfectly).
Why?
While covering happy paths is important, neglecting edge cases can lead to dangerous assumptions in your application.
Solution:
Use parameterized tests for complex scenarios, or create multiple test methods to cover edge cases.
Here’s an example:
@ParameterizedTest
@ValueSource(longs = {0, -1, -100})
public void testGetUserById_InvalidId_ShouldReturnBadRequest(long invalidId) throws Exception {
mockMvc.perform(get("/users/{id}", invalidId))
.andExpect(status().isBadRequest());
}
This parameterized test checks how the controller reacts to invalid IDs, providing better coverage.
4. Forgetting to Clean Up Tests
JUnit tests can pollute shared state if not properly cleaned up. This situation typically arises when shared mutable objects are not reset between tests.
Why?
This oversight can lead to flaky tests, where the outcome of one test can influence another.
Solution:
Use the @Before
or @After
annotations to initialize or reset shared resources.
Here's an example of cleanup in tests:
@Before
public void setUp() {
// Perform necessary setup
}
@After
public void tearDown() {
Mockito.reset(userService);
}
Using Mockito.reset(userService)
ensures that our mocks do not carry over any state from previous tests.
5. Not Verifying Interactions with Mocks
Finally, a common mistake is neglecting to verify the interactions with mocks, mainly what methods were called and how many times.
Why?
Failing to verify interactions can obscure issues with service layering.
Solution:
Use Mockito.verify()
to check that expected methods are called.
Here’s how:
@Test
public void testGetUserById_VerifyServiceCall() throws Exception {
User user = new User(1L, "John Doe");
when(userService.findById(1L)).thenReturn(user);
mockMvc.perform(get("/users/{id}", 1L))
.andExpect(status().isOk());
Mockito.verify(userService).findById(1L);
}
This not only confirms that the controller produces the expected outcome, but also that the underlying service method is called with the right parameters.
The Closing Argument
Mastering JUnit testing for Spring MVC controllers can significantly enhance your application's robustness. By avoiding pitfalls such as failing to isolate controllers, ignoring HTTP statuses, oversimplifying scenarios, neglecting cleanups, and not verifying interactions, you can create tests that are not only reliable but also maintainable.
For further reading on effective testing techniques, you may find these helpful:
Now that you have this guide at your disposal, take your time to implement these strategies and watch your testing practices elevate your Spring MVC development! Happy testing!