Common Pitfalls When Integrating Java Apps with Neo4j
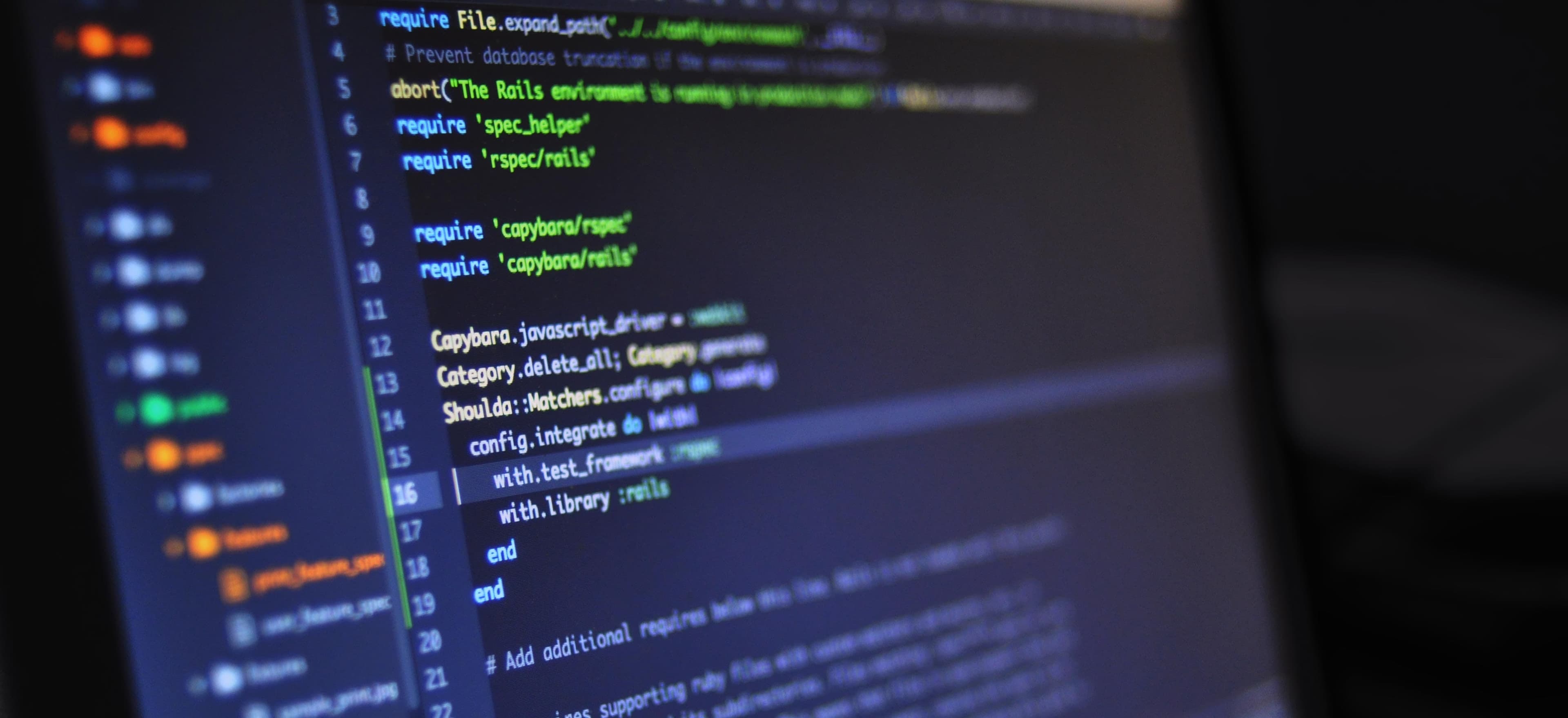
- Published on
Common Pitfalls When Integrating Java Apps with Neo4j
Integrating Java applications with Neo4j, the leading graph database, can massively enhance data relationships and access patterns. However, developers may stumble upon various pitfalls during this process. In this blog post, we'll delve deeply into these challenges and provide useful insights, code snippets, and solutions to ensure a smooth integration process.
Understanding Neo4j and Its Importance
Neo4j is a high-performance graph database, designed to handle large sets of connected data with ease. Using graphs instead of traditional relational tables allows for more complex queries and relationships. Java, being one of the most popular programming languages, pairs well with Neo4j, thanks to the official Neo4j Java Driver.
Before we dive into common pitfalls, let’s set the stage for the kind of integration we're talking about.
Basic Setup: Prerequisites
To follow along with the integration, ensure you have the following:
- Java Development Kit (JDK)
- Maven or Gradle (for dependency management)
- Neo4j Database (local installation or hosted service)
- Neo4j Java Driver (latest version)
You can find the official driver here.
Sample Maven Dependency
To start, here is how you can include the Neo4j Java Driver in your Maven project:
<dependency>
<groupId>org.neo4j.driver</groupId>
<artifactId>neo4j-java-driver</artifactId>
<version>4.4.0</version>
</dependency>
Common Pitfalls When Integrating Java Apps with Neo4j
1. Not Understanding the Graph Model
One of the most significant pitfalls developers face is not fully appreciating the graph data model. Unlike traditional relational databases, where data is stored in tables, Neo4j uses nodes, relationships, and properties.
Key Insight:
Before writing queries or designing your application structure, invest time understanding how your data can be represented as a graph. Use tools like the Neo4j Graph Data Science Library to visualize and plan the relationships.
2. Inefficient Queries
Another common mistake is writing inefficient Cypher queries. In graph databases, how you formulate your queries can significantly impact performance.
Example of a Poorly Written Query:
MATCH (n:Person) WHERE n.age > 30 RETURN n
While this query may return the desired results, it lacks indexing and may become slow with larger datasets.
Optimized Query:
CREATE INDEX ON :Person(age)
It’s critical to establish indexes on frequently queried properties. The faster your queries run, the better your application will perform overall.
3. Improper Transaction Management
Transaction management in Neo4j is essential. Failing to manage transactions effectively can lead to unexpected behaviors like partial data writes, which can trap your application in an inconsistent state.
Example Code Snippet:
try (Session session = driver.session()) {
session.writeTransaction(tx -> {
tx.run("CREATE (:Person {name: $name})", parameters("name", "John"));
return null;
});
}
In this example, we properly manage a write transaction using a Java try-with-resources
statement that will automatically close the session, ensuring that resources are cleaned up.
4. Ignoring Error Handling
Neglecting robust error handling is another pitfall. Neo4j may throw exceptions like ServiceUnavailableException
, SessionExpiredException
, or others based on various factors, such as network delays or database locks.
Best Practice:
Wrap your transactional code in try-catch blocks and implement proper logging to record errors.
try {
// Transaction code...
} catch (ServiceUnavailableException e) {
log.error("Neo4j service unavailable: {}", e.getMessage());
} catch (Exception e) {
log.error("Unexpected error: {}", e.getMessage());
}
5. Not Utilizing the Neo4j Bolt Protocol
Neo4j communicates using the Bolt Protocol for accessing data. While REST is available, using the Bolt Protocol is essential for performance-efficient, low-latency communication.
Using the Bolt Driver:
driver = GraphDatabase.driver("bolt://localhost:7687", AuthTokens.basic("username", "password"));
By utilizing the Bolt driver, you minimize network overhead, enhancing the overall application speed.
6. Overlooking Connection Pooling
Connection management can often be overlooked. The Neo4j Java Driver supports connection pooling automatically, but failing to configure it can lead to performance bottlenecks in high-load scenarios.
Ensure you initialize the driver only once and reuse the instance throughout your application.
private static final Driver driver = GraphDatabase.driver("bolt://localhost:7687", AuthTokens.basic("username", "password"));
7. Not Using Parameterized Queries
Hardcoding values into Cypher queries can lead to security vulnerabilities, such as SQL Injection in the case of relational databases.
Example of a Secure Approach:
String query = "CREATE (p:Person {name: $name})";
try (Session session = driver.session()) {
session.run(query, parameters("name", "Jane"));
}
Using parameterized queries ensures that input values are securely handled, improving both security and performance.
8. Failing to Leverage Asynchronous Calls
Neo4j's Java Driver supports asynchronous programming models. If your application can handle multiple queries simultaneously, leverage this feature to improve responsiveness.
Example Code Snippet:
CompletableFuture<Void> future = CompletableFuture.runAsync(() -> {
try (Session session = driver.session()) {
session.run("CREATE (a:Person {name: 'Alice'})");
}
});
While handling multiple requests, your application remains responsive to the user, enhancing the overall user experience.
A Final Look
Integrating Java applications with Neo4j can be a powerful way to manage and analyze connected data. Awareness of the common pitfalls outlined in this post— from query optimization to error handling—will help ensure your integration is smooth and efficient. The provided code snippets and tips should serve as a practical guide as you navigate your journey with Neo4j.
For deeper dives into the Neo4j ecosystem, check out the Neo4j Developer Zone where you can find tutorials, guides, and community forums.
Further Reading
- Neo4j Java Driver Documentation
- Crafting Efficient Queries in Neo4j
- Understanding Neo4j’s Data Model
Happy coding, and may your integrations be both seamless and effective!