“Overcoming Slow XML Route Loading in Apache Camel 3.1”
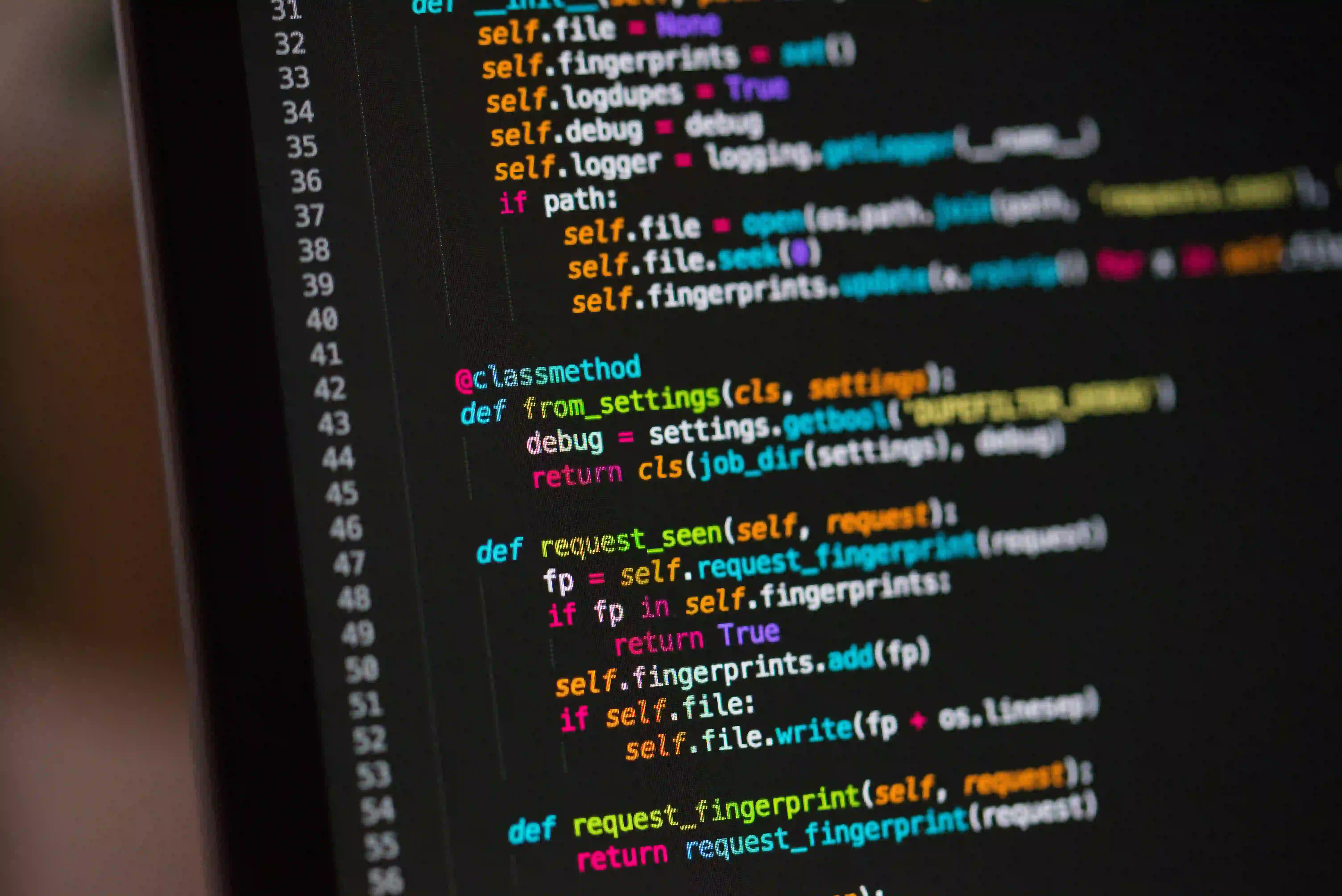
Overcoming Slow XML Route Loading in Apache Camel 3.1
As software systems grow in complexity, developers often seek solutions that can handle intricate integrations efficiently. In such cases, Apache Camel serves as a robust integration framework, particularly well-suited for routing. However, one common challenge that developers encounter is slow XML route loading. In this blog post, we will explore ways to overcome this issue in Apache Camel 3.1.
What is Apache Camel?
Apache Camel is an open-source integration framework that allows developers to define routing and mediation rules in various domain-specific languages (DSLs). It streamlines the task of connecting different applications by providing a rich set of connectors and other functionalities. While Camel 3.1 offers numerous improvements, XML route loading can sometimes be sluggish, especially when dealing with extensive configurations.
Why is XML Route Loading Slow?
Slow XML route loading is primarily due to several factors:
- Large XML Files: Extensive route definitions can lead to significant overhead during parsing.
- Inefficient Resource Usage: The application may consume excessive memory and CPU while loading routes.
- Complex Route Definitions: XML files that employ complex expressions or maintain many dependencies can slow down the loading process.
Understanding these challenges can help us in crafting effective solutions to optimize route loading time.
Strategies to Optimize XML Route Loading
1. Reduce File Size and Complexity
Before diving into advanced techniques, the simplest approach is often the best.
Modularize Route Definitions
If your application uses a single extensive XML file for route definitions, consider splitting it into multiple smaller files. This approach fosters better organization and easier debugging.
<camelContext xmlns="http://camel.apache.org/schema/spring">
<include uri="classpath:routes/first-route.xml"/>
<include uri="classpath:routes/second-route.xml"/>
</camelContext>
By segmenting your routes, you decrease parse time while gaining clear boundaries for your integrations.
2. Use Java DSL Instead of XML
While XML offers declarative configurations, it can come at a cost regarding performance. Java DSL allows for more straightforward and efficient route definitions.
from("direct:start")
.to("log:info")
.to("mock:result");
Java DSL not only enhances readability and maintainability but can also improve loading time due to its compile-time optimizations. Given that routes are defined programmatically, they are easier to modify, debug, and test.
3. Lazy Initialization
Lazy initialization allows Camel to defer the loading of routes until they are needed. This configuration can significantly improve startup times.
To enable lazy initialization, use the following configuration in your camelContext
:
<camelContext xmlns="http://camel.apache.org/schema/spring" lazyLoad=true>
<route id="exampleRoute">
<from uri="direct:start"/>
<to uri="mock:result"/>
</route>
</camelContext>
With this setup, only the essential routes are loaded initially, enabling quicker startup times without losing the overall functionality.
4. Optimize XML Parsing
Utilizing less verbose XML can help in reducing the overhead of XML parsing. Consider alternatives like JSON or YAML for defining Camel routes. If you must stick with XML, ensure that your XML is well-formed and adheres to best practices.
5. Utilize Camel's Property Placeholders
Camel’s property placeholders let you externalize configurations. By reducing hard-coded values in XML, you can eliminate unnecessary bloat.
<propertyPlaceholder location="classpath:application.properties"/>
<route id="dynamicRoute">
<from uri="{{start.endpoint}}"/>
<to uri="{{end.endpoint}}"/>
</route>
Using property placeholders keeps your XML clean, which can indirectly improve performance since less data is loaded into memory during startup.
6. Monitoring and Profiling
Understanding performance bottlenecks is crucial for maintaining efficient routes. Utilize tools like Java Mission Control or VisualVM to monitor the application. Analyzing CPU usage and memory consumption can help identify areas for improvement and possible optimizations.
You might find that certain routes take longer to process than expected. Address these cases by optimizing the logic in your routes rather than the XML structure.
Closing the Chapter
In conclusion, slow XML route loading in Apache Camel 3.1 can be addressed through various strategies. Whether through modularization, switching to Java DSL, enabling lazy initialization, or optimizing XML parsing, there are numerous paths available to enhance performance.
Implementing these suggestions can significantly improve the responsiveness of your integration framework, paving the way for more efficient applications. The tools and techniques discussed empower not only a smoother loading experience but also clearer and maintainable code, essential in today’s fast-paced development environments.
For further reading, check out Apache Camel's official documentation for a deeper understanding of feature usage, and refer to CameL in Action for practical insights on effective integration strategies.
By optimizing XML route loading, you ensure that you're not only improving performance but also enhancing your application’s overall reliability. What methods have you used to optimize your Camel routes? Share your experiences in the comments below!