Overcoming Jenkins Build Failures in Android Projects
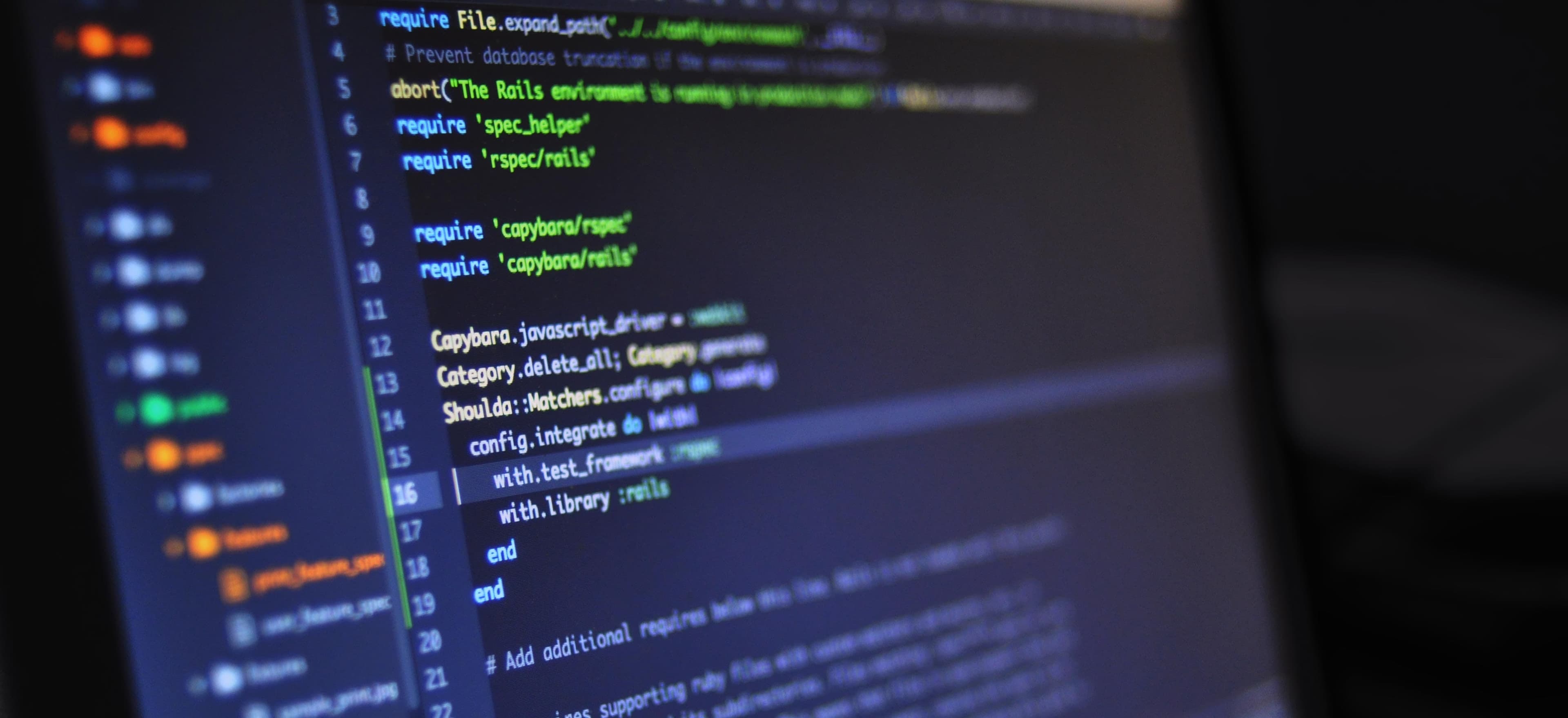
- Published on
Overcoming Jenkins Build Failures in Android Projects
Continuous Integration (CI) is a vital part of modern software development, especially in mobile app development. Jenkins, being a popular open-source CI tool, facilitates the automation of builds and tests for Android projects. However, developers often encounter build failures that can stall progress. In this blog post, we will discuss common causes of Jenkins build failures in Android projects and how to address them effectively.
Understanding Jenkins and Its Role in Android Development
Jenkins provides a robust platform for automating parts of your development process. For Android developers, Jenkins offers several advantages:
- Automated Builds: Reduces manual intervention and ensures consistent builds.
- Continuous Testing: Enables immediate feedback through automated unit and integration tests.
- Scalability: Supports multiple builds in parallel, enhancing productivity.
Despite its benefits, build failures can still occur due to various reasons. Below are common causes and strategies for troubleshooting these failures.
Common Causes of Jenkins Build Failures
1. Environmental Issues
Description: Jenkins runs on different environments—whether it is on a local machine, a virtual cloud server, or dedicated hardware. Environment configurations can significantly affect the build process.
Solution: Ensure that the environment is correctly configured, including the Java version and Android SDK.
# Check your Java installation
java -version
# Check your Android SDK installation
echo $ANDROID_HOME
Make sure that these versions match the requirements specified in your Android project (e.g., Java 11).
2. Dependency Conflicts
Description: Android projects rely heavily on various libraries and dependencies (e.g., Gradle dependencies). Version conflicts can lead to build failures.
Solution: Always define specific versions for your dependencies. Using the implementation
keyword in your build.gradle
file can help manage dependencies properly.
dependencies {
implementation 'com.google.code.gson:gson:2.8.6'
implementation 'androidx.appcompat:appcompat:1.0.2'
}
Avoid using dynamic versions (like +
), as they can change over time and introduce instability.
3. CI Configuration Issues
Description: Sometimes, Jenkins itself isn't properly configured for Android. The CI server may not have the necessary tools installed.
Solution: Ensure that your Jenkins job is set up correctly. Here’s an example of a Jenkins pipeline script specifically for Android builds:
pipeline {
agent any
stages {
stage('Checkout') {
steps {
git 'https://github.com/your-android-project.git'
}
}
stage('Build') {
steps {
script {
sh './gradlew assembleDebug'
}
}
}
}
}
This script checks out your Android project and runs the Gradle build command. Adjust paths and flags as necessary.
4. Resource Limitations
Description: Jenkins builds can fail if they run out of system resources, such as memory or disk space. This is often the case when working with large Android projects or when concurrent builds are not managed.
Solution: Monitor your Jenkins node resources and increase them as necessary. You might also want to configure Jenkins to limit concurrency using the following:
pipeline {
agent any
options {
disableConcurrentBuilds()
}
}
This option ensures that only one build runs at a time for your job, helping to conserve resources.
Best Practices for Debugging Jenkins Build Failures
View Logs
Your first step in troubleshooting should always be to check the Jenkins build logs. These logs often contain error messages that provide context for the failure.
Build Locally
If you encounter a persistent build failure, replicate the build process locally. Use the same build commands as in Jenkins. This helps determine if the issue is related to Jenkins or if it exists in the codebase itself.
Clean Caches
Sometimes old build caches might introduce problems. In Jenkins, you can clean the cache using the following command in your build.gradle
:
clean {
delete rootProject.buildDir
}
Keep Dependencies Updated
Regularly update your dependencies by checking for new versions and compatibility fixes.
# Update dependencies using Gradle
./gradlew dependencyUpdates
Utilize Community Resources
When facing issues, don’t hesitate to refer to community forums or documentation. Resources such as Stack Overflow and the Jenkins User Documentation can provide answers and troubleshooting tips.
To Wrap Things Up
While Jenkins is a powerful tool for continuous integration in Android development, it comes with its set of challenges. Environment configurations, dependency conflicts, CI settings, and resource issues are just a few common pitfalls that can cause build failures. By implementing the solutions and best practices discussed in this article, you can minimize distractions and improve your CI/CD workflow.
Maintaining a reliable CI/CD pipeline is critical in delivering high-quality Android applications consistently. Stay proactive in addressing potential issues, use the tools available to you, and don’t hesitate to seek help when needed. Happy coding!
For more information about Jenkins and Android development, consider exploring Jenkins CI for Beginners and the Android Developer Guide. These resources can provide deeper insights into both Jenkins and Android ecosystems.