Resolving Common Java EE 6 Galleria Errors: A Guide
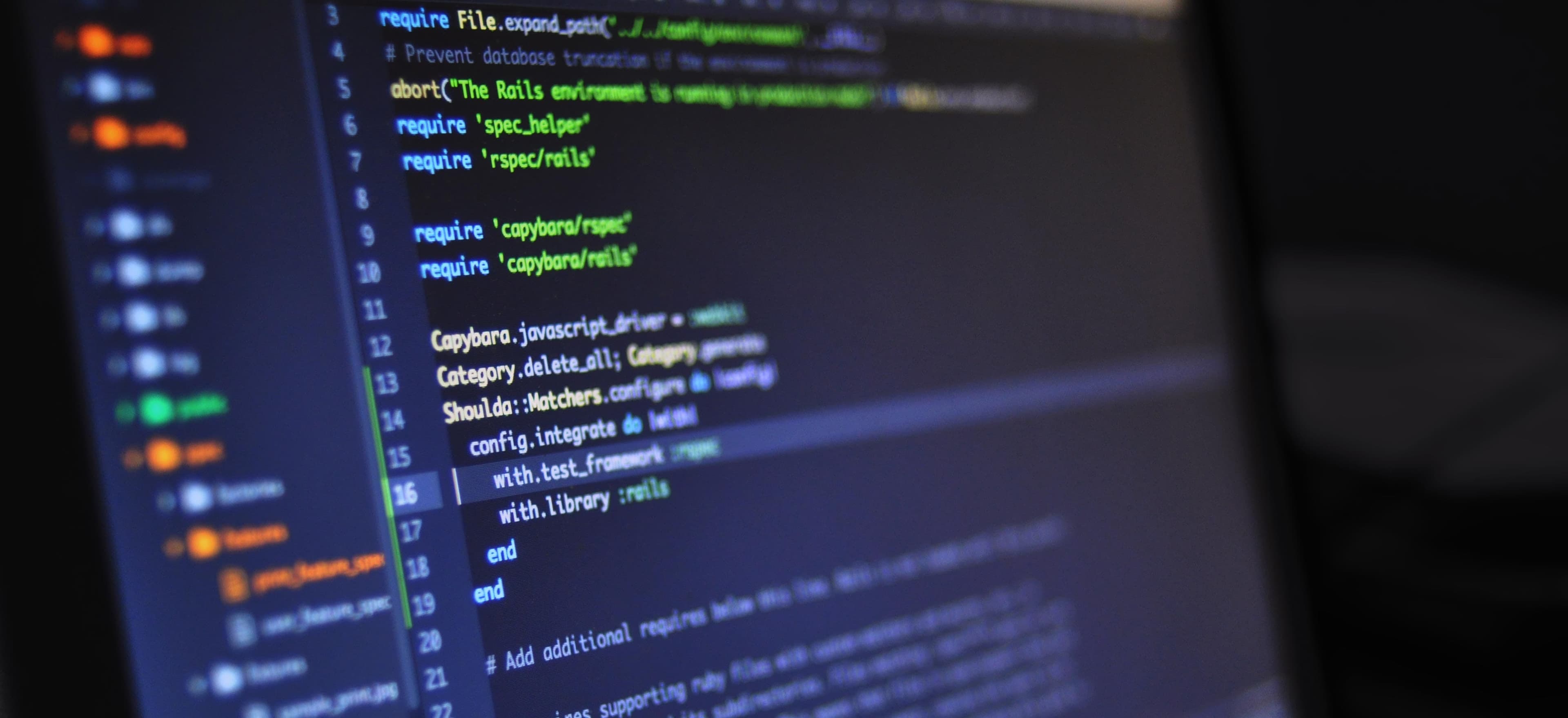
- Published on
Resolving Common Java EE 6 Galleria Errors: A Guide
Java EE 6 has long been a cornerstone in the enterprise application landscape, and Galleria is often one of the frameworks leveraged for creating rich user interfaces. However, like any technology, it is not without its challenges. This blog post dives deep into some common issues developers face when working with Java EE 6 Galleria, providing solutions, code snippets, and valuable insights to help you navigate these hurdles effectively.
Understanding Java EE 6 and Galleria
Java EE (Enterprise Edition) 6 is a set of specifications that extends the Java SE (Standard Edition) with specifications for enterprise features such as distributed computing and web services. Galleria is a JavaScript library designed for creating responsive image galleries and presentations. Integrating these technologies allows developers to create visually engaging and scalable applications.
Common Galleria Errors
When working with Galleria in a Java EE 6 context, several common errors may arise. Understanding these issues is critical for building robust applications. Let's discuss some of these problems and their solutions.
1. Galleria Initialization Error
Error Message: "Galleria is not defined"
This error typically indicates that the Galleria library has not been loaded correctly before you attempt to use it.
Solution
Ensure that you include the Galleria library correctly in your HTML. Here is an example of how to do this:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Galleria Example</title>
<link rel="stylesheet" href="path/to/galleria.css">
<script src="path/to/jquery.min.js"></script>
<script src="path/to/galleria.min.js"></script>
<script>
$(document).ready(function() {
Galleria.run('.galleria');
});
</script>
</head>
<body>
<div class="galleria">
<img src="image1.jpg">
<img src="image2.jpg">
<img src="image3.jpg">
</div>
</body>
</html>
Why This Works
- Order of Inclusion: The order matters; make sure jQuery is loaded before Galleria, as Galleria is dependent on jQuery.
- Document Ready: By wrapping your Galleria initialization code in
$(document).ready()
, you ensure that the DOM is fully loaded before trying to run Galleria.
2. Image Not Displaying
Error Message: "Image not found"
This is a straightforward problem but can frequently occur if the image paths are incorrect.
Solution
Double-check your image file paths in the HTML. For example:
<img src="invalid/path/image.jpg"> <!-- This will cause an error -->
To fix:
<img src="assets/images/image1.jpg"> <!-- Correct path -->
Why This Works
mUpdate your HTML with the correct image paths. Ensuring paths are accurate guarantees that Galleria can access the images you want to display.
3. CSS Conflict Issues
Error Message: "Layout issues or misalignments"
CSS conflicts could arise when Galleria styles clash with your existing CSS.
Solution
Inspect the CSS by using the browser’s developer tools. Here are tips to isolate the issue:
- Namespace Your CSS: Use more specific selectors for your styles to avoid conflicts.
- Override Styles: You may want to override certain styles applied by Galleria. Here's an example:
.galleria {
width: 80%;
margin: auto;
border: 2px solid #333; /* Add your custom styling */
}
/* Override Galleria styles if needed */
.galleria img {
max-width: 100%; /* Ensures responsive images */
}
Why This Works
By using specific CSS selectors, you reduce the chances of conflicts while ensuring that your gallery remains visually integrated with the overall design of your project.
4. Browser Compatibility Issues
Error Message: "Gallery not functioning in certain browsers"
Galleria relies on JavaScript, and different browsers may render features inconsistently.
Solution
Always check if your Galleria implementation is supported across major browsers by referring to the Galleria documentation and ensuring you're using their recommended version.
Additionally, validate your code through tools like Can I use to check for compatibility across different browsers.
5. Performance Issues with Large Images
Large images can slow down your application's performance or fail to load altogether.
Solution
Optimize the images you use in your Galleria instance. Use formats like WebP or compress your images without sacrificing quality. Here’s an example of a basic optimization function in Java:
public void optimizeImage(File inputImage) throws IOException {
BufferedImage image = ImageIO.read(inputImage);
BufferedImage resizedImage = resizeImage(image, 800, 600); // Set your desired dimensions
ImageIO.write(resizedImage, "jpg", new File("optimized_image.jpg"));
}
// Helper method to resize image
private BufferedImage resizeImage(BufferedImage originalImage, int newWidth, int newHeight) {
BufferedImage newImage = new BufferedImage(newWidth, newHeight, BufferedImage.TYPE_INT_RGB);
Graphics2D g = newImage.createGraphics();
g.drawImage(originalImage, 0, 0, newWidth, newHeight, null);
g.dispose();
return newImage;
}
Why This Works
By optimizing images, you reduce the loading time and enhance the performance of your gallery, which is crucial for user experience.
Final Considerations
Resolving common Java EE 6 Galleria errors requires a blend of troubleshooting skills, knowledge of the technologies, and code optimization strategies. By addressing issues like initialization problems, image paths, CSS conflicts, performance lags, and browser support, you can create an effective and visually appealing gallery.
Make sure to consider these solutions as you develop your application, thereby enhancing both its functionality and user experience.
This guide is just the beginning of your journey with Java EE 6 and Galleria. Keep experimenting, and don't hesitate to explore the rich documentation available online for both technologies. Happy coding!
Checkout our other articles