Why Your Distributed Service Registry Might Fail
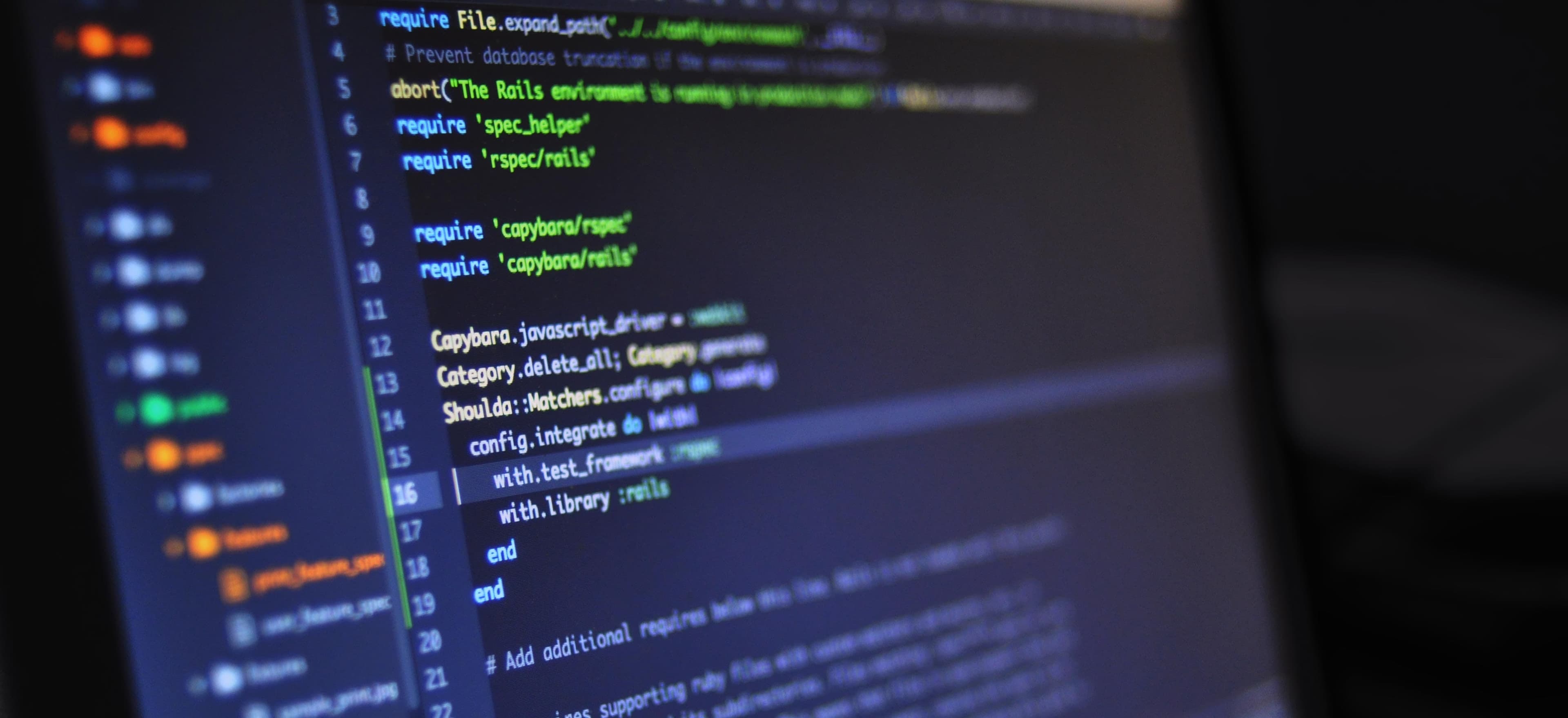
- Published on
Why Your Distributed Service Registry Might Fail
In today's cloud-native ecosystems, microservices architecture has taken center stage, allowing organizations to develop scalable and resilient applications. A key component of this architecture is the service registry. However, many organizations overlook the potential pitfalls of using distributed service registries. In this blog post, we will delve into the common reasons why a distributed service registry might fail, providing insights to help you avoid these traps.
Understanding Service Registries
Before diving into the reasons for failure, let’s clarify what a service registry is. A service registry is a centralized directory where microservices can register themselves and discover other services. It acts as a location service that facilitates the communication between different services efficiently.
Why Use a Service Registry?
- Dynamic Discovery: Services can discover each other dynamically at runtime.
- Load Balancing: It can help distribute client requests across multiple service instances.
- Health Monitoring: It often includes health checks to ensure only healthy services receive traffic.
Popular implementations of service registries include Consul, Eureka, and Zookeeper. While they provide these advantages, they can also lead to systemic issues if not implemented or managed correctly.
Common Reasons for Failure
1. Single Point of Failure
A common issue with service registries is that they can become a single point of failure. If the registry goes down, communication between services may be interrupted, leading to a cascade of failures across the system.
Solution:
Implement high availability (HA) setups by running multiple instances of the service registry. For instance, using Consul, you can configure Raft Consensus Algorithm for leader election.
# Example Consul HA Configuration
datacenter = "dc1"
bind_addr = "0.0.0.0"
# Enable high availability
server = true
bootstrap_expect = 3
2. Network Partitioning
Distributed systems are prone to network partitions. If part of the network becomes isolated, the service registry may end up with an incomplete view of available services.
Solution:
Use partition tolerance mechanisms, such as CAP theorem strategies. Consider implementing Sharding, which allows services to be grouped and registered in different nodes.
3. Service Registration Lag
Sometimes, services may not register with the registry immediately, which can cause temporary downtimes where services cannot find each other.
Solution:
Implement a registration grace period for services to give them time to register after starting. Consider using a retry strategy.
import java.util.concurrent.TimeUnit;
public void registerService(Service service) {
try {
// Attempt service registration with a timeout
serviceRegistry.register(service);
} catch (Exception e) {
// Handle registration failure with a backoff strategy
TimeUnit.SECONDS.sleep(2);
registerService(service);
}
}
4. Data Consistency Issues
Having a distributed service registry can lead to data inconsistency between different nodes of the registry. This often results in some services being listed in some registries but not others.
Solution:
Adopt a well-defined consistency model such as Eventual Consistency or Strong Consistency based on your application needs.
5. Performance Bottlenecks
As the number of services increases, the load on the service registry can become a bottleneck. A sluggish registry can lead to delays in service discovery.
Solution:
Optimize performance by:
- Using caching strategies for frequent requests.
- Scaling horizontally to handle higher loads.
// Caching example for service lookups
public class ServiceDiscoveryCache {
private static final ConcurrentHashMap<String, Service> cache = new ConcurrentHashMap<>();
public Service getService(String serviceName) {
return cache.computeIfAbsent(serviceName, this::fetchService);
}
private Service fetchService(String serviceName) {
// Logic to fetch service from registry
}
}
6. Poorly Defined Health Checks
Inadequate health checks can result in unresponsive services continuing to appear in the registry. This can cause the system to send requests to a non-functional service, leading to failures.
Solution:
Define robust health checks that include:
- TCP checks: Verify the service is listening on the expected port.
- HTTP checks: Call an endpoint to verify application-level health.
Example in a configuration file might look like:
# Consul Health Check Example
check {
http = "http://localhost:8080/health"
interval = "10s"
timeout = "5s"
}
7. Configuration Complexity
Managing the configuration for distributed registries can quickly become complex and prone to errors. Misconfigured settings can cripple the performance or reliability of your service discovery.
Solution:
Use configuration management tools like Consul Template or Vault to maintain service registry configurations. This ensures that changes are propagated consistently across the registry.
8. Inadequate Monitoring and Alerting
Without proper monitoring, issues in the service registry can go undetected until they cause serious failures in the system.
Solution:
Integrate comprehensive monitoring tools (like Grafana or Prometheus) that can alert you to abnormalities in registry performance.
A Final Look
While distributed service registries are invaluable in microservices architecture, they are not without challenges. Understanding the risks and deploying appropriate mitigations can help ensure your service registry remains a robust component of your architecture.
For more in-depth reading, consider exploring these resources:
By taking proactive measures, you'll not only reduce the risk of registry failures but also enhance the overall reliability and scalability of your microservice ecosystem. It’s time to get ahead of potential pitfalls and ensure your distributed service registry serves its purpose effectively.
Checkout our other articles