Common Maven Issues When Setting Up Your Application Server
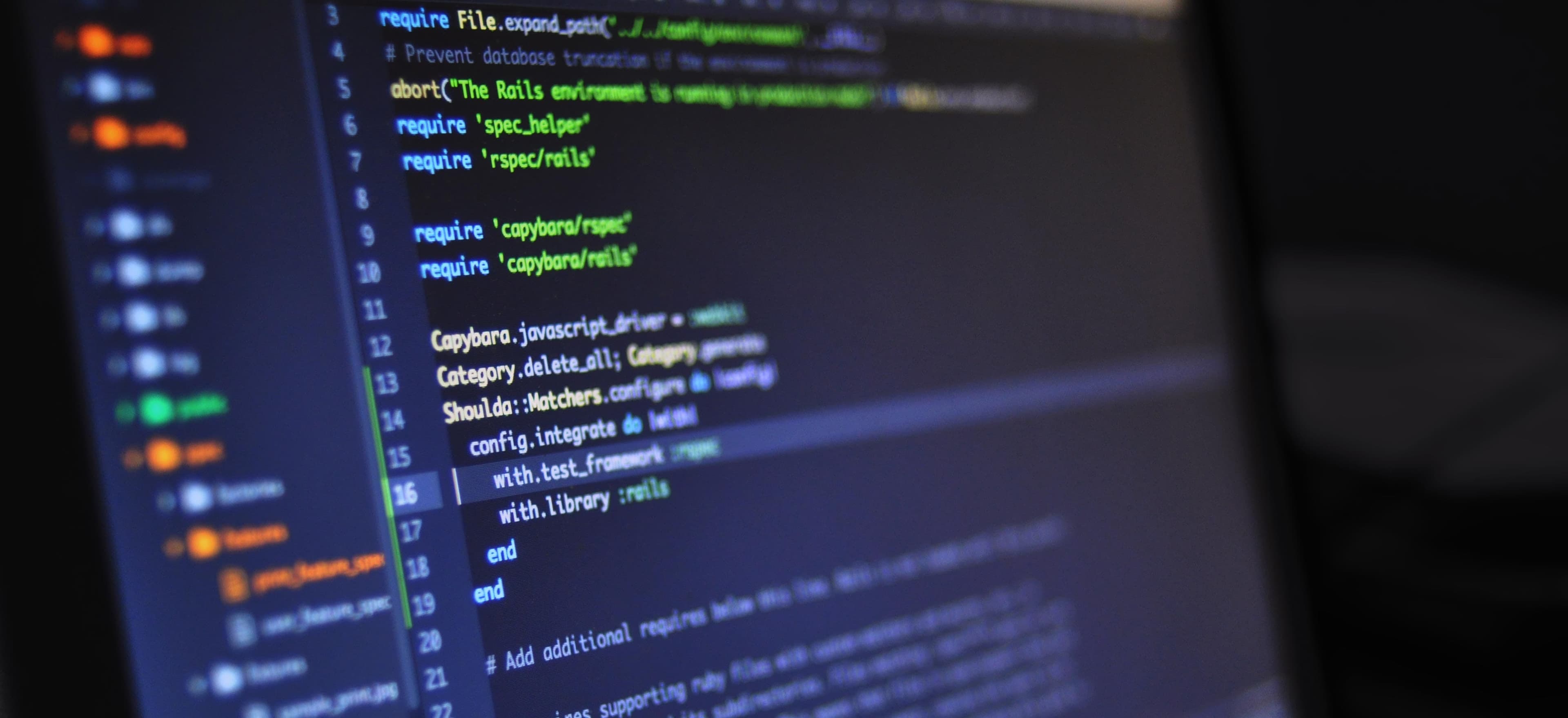
- Published on
Common Maven Issues When Setting Up Your Application Server
Setting up an application server can be a daunting task, especially when integrating with Maven. As developers adopt Maven as a standard build tool, understanding common issues that may arise becomes critical. This blog post explores typical Maven problems, solutions, and best practices to keep your application server up and running smoothly.
What is Maven?
Maven is a powerful build automation tool primarily used for Java projects. It manages project dependencies, builds projects from source code, and deploys applications to servers. Its core advantages include:
- Dependency Management: Automatically handles project libraries.
- Standardization: Follows project structure guidelines.
- Integration: Works seamlessly with continuous integration tools.
Common Maven Issues
Despite its advantages, you may face various challenges during your setup. Below are some common issues and their corresponding solutions.
1. Dependency Resolution Issues
One of the most frequent Maven issues arises from dependency resolution failures. This can stem from several causes:
- Incorrect artifact version specified in the
pom.xml
. - Missing or inaccessible remote repositories.
- Conflicts between different artifact versions.
Example Problem
Suppose you've declared a dependency in your pom.xml
:
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>5.2.0.RELEASE</version>
</dependency>
If this version isn't available in Maven Central or your custom repositories, you'll encounter a "dependency not found" error.
Solution
- Verify Dependency Versions: Always check the Maven Central Repository or your shared repository to confirm that the required version exists.
- Force Update: You can use the
-U
flag to force Maven to update snapshots and releases.
mvn clean install -U
- Check Repository Accessibility: Ensure you can access the repository either via your corporate firewall or proxy settings.
2. Incompatible Plugin Versions
Another potential headache is incompatible or outdated plugins in your Maven build. Over time, plugins may become deprecated or conflict with other dependencies.
Example Problem
Your build might fail with an error related to a Maven plugin:
Plugin execution is skipped: org.apache.maven.plugins:maven-compiler-plugin:3.7.0:compile
Solution
- Specify Plugin Version: Always include explicit versions in your
pom.xml
:
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.8.1</version>
</plugin>
</plugins>
</build>
- Check Compatibility: Refer to the Maven Plugin Documentation to verify version compatibility before upgrading or adding plugins.
3. Incorrect Java Version
With Maven, it's vital to ensure that your Java version matches the version specified in your project.
Example Problem
If your project uses Java 11, but your server runs Java 8, you'll encounter runtime exceptions.
Solution
- Set Java Version in pom.xml: Define the Java version under the
properties
section:
<properties>
<maven.compiler.source>11</maven.compiler.source>
<maven.compiler.target>11</maven.compiler.target>
</properties>
- Check Installed Java Version: Use the following command to confirm your Java version:
java -version
4. Classpath Issues
Classpath problems can lead to ClassNotFoundException
errors if the right dependencies are not available in the runtime.
Example Problem
Running the application might reveal:
Exception in thread "main" java.lang.ClassNotFoundException: com.example.MyApp
Solution
- Verify Dependency Scope: Ensure you are using the correct scope for your dependencies:
<dependency>
<groupId>org.example</groupId>
<artifactId>my-library</artifactId>
<version>1.0</version>
<scope>compile</scope>
</dependency>
- Check GAV Coordinates: Double-check the Group ID, Artifact ID, and Version (GAV) coordinates for typos.
5. Profile Activation
Maven profiles allow you to customize builds, but misconfigured profiles can lead to unexpected behavior.
Example Problem
If you're trying to run a profile, you might receive a warning such as:
No active profile found for the configuration
Solution
- Check profile activation: In your
pom.xml
, ensure you have correctly defined profiles. To activate a profile, use:
mvn clean install -P my-profile
- Explicit Activation: You can also use activation conditions such as operating system or property values in the profile definition.
6. Local Repository Issues
Sometimes, your local .m2
repository could be corrupt, possibly causing missing dependencies.
Example Problem
Attempts to build your project might yield errors like:
Could not transfer artifact
Solution
- Clear Local Repository: If you suspect corruption, clear your
.m2
directory:
rm -rf ~/.m2/repository/org/example
- Rebuild: This forces Maven to re-download the dependencies.
Best Practices
To minimize issues with Maven during application server setup, consider the following best practices:
-
Regular Updates: Keep your Maven version and dependencies updated. You can check for updates using the Maven Versions Plugin.
mvn versions:use-latest-releases
-
Use a Continuous Integration Tool: Implement CI tools like Jenkins or GitHub Actions to automate builds and catch issues early.
-
Write Tests: Incorporate unit and integration tests to ensure code reliability and minimize unexpected runtime issues.
-
Maintain Documentation: Document your project setup, include details on library versions, and share insights with your team.
Bringing It All Together
Setting up an application server with Maven can present various challenges. However, by understanding common issues and implementing best practices, you can streamline the process. Always remain proactive about dependency management, plugin compatibility, and testing to ensure a healthy development environment.
For more in-depth reading, consider checking out the Maven Documentation and Maven Best Practices.
Adopting these practices can turn headaches into a seamless experience. Happy coding!