Troubleshooting Failed Auto Scaling Group Deployments on AWS
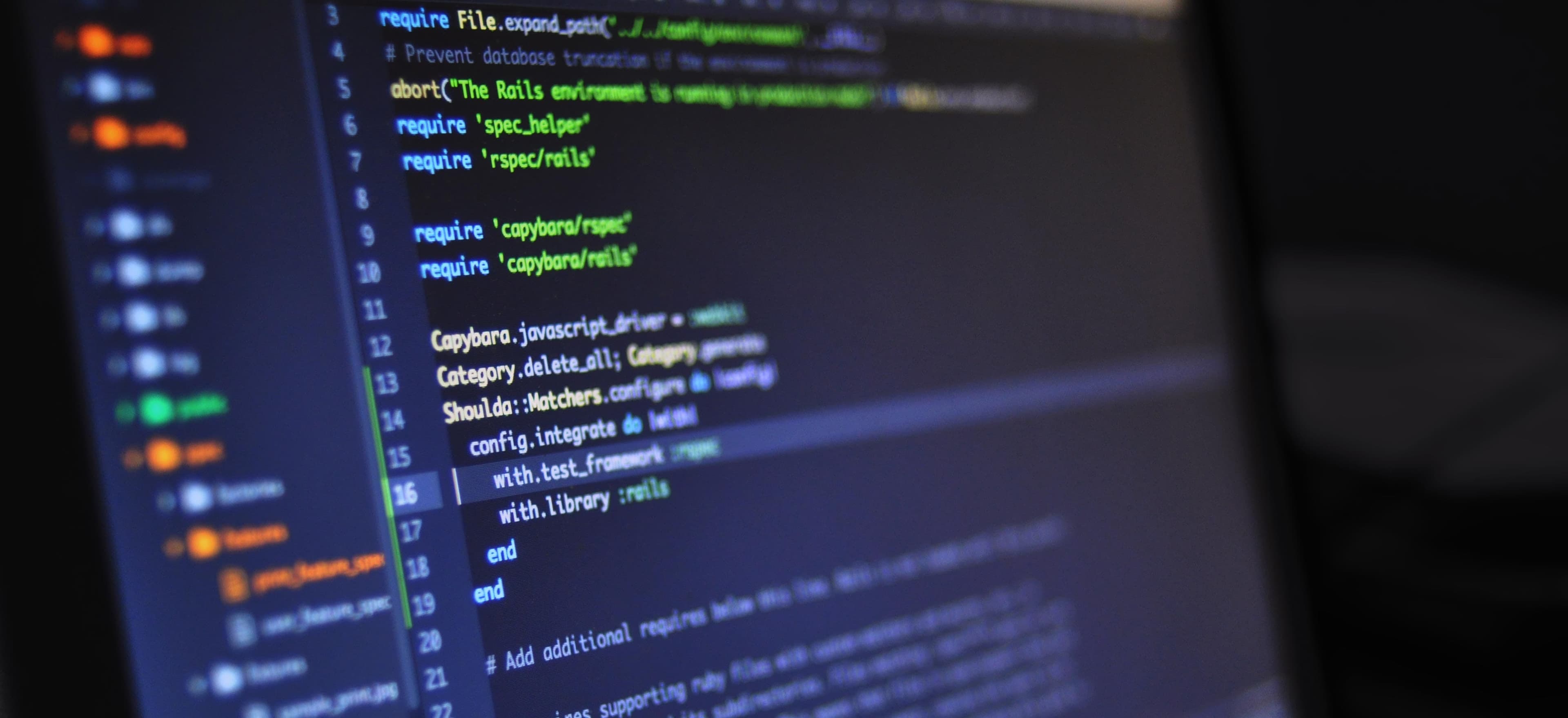
- Published on
Troubleshooting Failed Auto Scaling Group Deployments on AWS
Auto Scaling Groups (ASGs) are a powerful feature of Amazon Web Services (AWS) that help ensure your application can adapt to changes in load. However, deployments in ASGs can sometimes fail, which can be frustrating. Understanding how to troubleshoot these failures is crucial for maintaining application performance and reliability.
In this blog post, we will explore common reasons for failed ASG deployments, how to identify these issues, and the steps you can take to resolve them. By the end, you will be equipped with troubleshooting techniques and practical code snippets to enhance your AWS ASG management.
Understanding Auto Scaling Groups
Before diving into troubleshooting, let's briefly recap what Auto Scaling Groups do. ASGs automatically adjust the number of EC2 instances in response to changes in demand. They are particularly useful for applications with varying workloads, enabling users to scale out during peak times and scale in when not needed.
However, failures can occur for several reasons — from launch configurations to application-level issues. Here are some common causes of failed deployments.
Common Causes of ASG Deployment Failures
-
EC2 Launch Template or Launch Configuration Issues
- Incorrect or outdated configurations can prevent instances from launching correctly.
-
Insufficient IAM Permissions
- If the Auto Scaling Group does not have the required permissions to launch instances, it will fail.
-
Health Check Failures
- The instance might be terminating itself due to unresponsive health checks (e.g., application not starting properly).
-
Availability Zone Limitations
- Hitting the instance limits in a given availability zone can prevent new instances from being launched.
-
Resource Quotas
- AWS imposes limits on the number of resources you can allocate. Exceeding these can cause failures.
-
Networking Issues
- Problems with VPC configurations, security groups, or route tables can hinder communication and instance health.
-
Scaling Policies Configuration
- Misconfigured scaling policies can create a conflicting scenario where instances cannot maintain the desired state.
Step-by-Step Troubleshooting Guide
Step 1: Check Auto Scaling Events
AWS provides a detailed event log for Auto Scaling Groups. You can access this in the AWS Management Console:
- Navigate to EC2 Dashboard.
- Click on Auto Scaling Groups.
- Select your ASG and check the Activity History.
Look for any error messages or warnings that might point to the cause of the failed deployment.
Step 2: Review Launch Configuration or Launch Template
Verify whether your launch configuration or template is set up correctly:
- AMI Id: Ensure the AMI ID is correct and available in your AWS account.
- Instance Type: Select a valid instance type that meets your needs.
- Security Groups: Make sure to include necessary security groups.
Here is a sample snippet to create a simple launch configuration:
import software.amazon.awssdk.services.autoscaling.AutoScalingClient;
import software.amazon.awssdk.services.autoscaling.model.CreateLaunchConfigurationRequest;
import software.amazon.awssdk.services.autoscaling.model.CreateLaunchConfigurationResponse;
public class CreateLaunchConfig {
public static void main(String[] args) {
AutoScalingClient asgClient = AutoScalingClient.create();
CreateLaunchConfigurationRequest launchConfigRequest = CreateLaunchConfigurationRequest.builder()
.launchConfigurationName("MyLaunchConfig")
.imageId("ami-12345abc") // Confirm this AMI ID
.instanceType("t2.micro")
.securityGroups("my-security-group")
.build();
CreateLaunchConfigurationResponse response = asgClient.createLaunchConfiguration(launchConfigRequest);
System.out.println("Launch Configuration Created: " + response.launchConfigurationName());
}
}
Why this code? This Java snippet creates a launch configuration in an Auto Scaling group. It’s essential to confirm the configuration to avoid deployment failures.
Step 3: Validate IAM Permissions
Ensure that your Auto Scaling Group has the right permissions. Although it’s common to let AWS manage permissions through roles, review the IAM role associated with the ASG.
- Navigate to the IAM console.
- Check the policies attached to the role and ensure there are permissions for:
- ec2:RunInstances
- ec2:DescribeInstances
- ec2:TerminateInstances
A problematic policy can easily lead to failed deployments.
Step 4: Review Instance Health Checks
When an instance fails to respond to defined health checks, it is marked as unhealthy and terminated.
- Review your health check settings in the ASG configuration.
- Check application logs to understand whether the service is failing to start.
You can utilize metrics from Amazon CloudWatch to get insights into your EC2 instance health:
import software.amazon.awssdk.services.cloudwatch.CloudWatchClient;
import software.amazon.awssdk.services.cloudwatch.model.GetMetricStatisticsRequest;
public class CloudWatchMetrics {
public static void main(String[] args) {
CloudWatchClient cwClient = CloudWatchClient.create();
GetMetricStatisticsRequest request = GetMetricStatisticsRequest.builder()
.namespace("AWS/EC2")
.metricName("StatusCheckFailed")
.period(60)
.statisticsWithStrings("Average")
.dimensions(... ) // Include instance id as a dimension
.build();
// Execute the request and handle response
}
}
Why this snippet? It fetches EC2 instance metrics from CloudWatch to determine if the instance health checks are being violated.
Step 5: Look into Availability Zone Limits and Resource Quotas
Access the Limits section of the EC2 console to ensure that you haven’t hit your instance limits for your desired availability zone. If necessary, request a limit increase.
Step 6: Analyze Logs for Deeper Insights
Logs are often the best pathway to discovery. AWS CloudTrail can help you track what happened during the scaling event. Search through logs for any errors related to the scaling activity.
Consider enabling detailed logging for your EC2 instances, which will help improve visibility into issues occurring at the application level.
Step 7: Modify Scaling Policies
If your scaling policies are configured incorrectly, redesign them. It may be beneficial to implement CloudWatch alarms to base your scaling decisions on application load metrics.
Try this example to create an Auto Scaling policy:
import software.amazon.awssdk.services.autoscaling.AutoScalingClient;
import software.amazon.awssdk.services.autoscaling.model.PutScalingPolicyRequest;
public class CreateScalingPolicy {
public static void main(String[] args) {
AutoScalingClient asgClient = AutoScalingClient.create();
PutScalingPolicyRequest scalingPolicyRequest = PutScalingPolicyRequest.builder()
.autoScalingGroupName("MyAutoScalingGroup")
.policyName("ScaleOutPolicy")
.scalingAdjustment(1) // Increases group size by 1
.adjustmentType("ChangeInCapacity")
.build();
asgClient.putScalingPolicy(scalingPolicyRequest);
System.out.println("Scaling Policy Created");
}
}
Why this code? This Java snippet creates a scaling policy to adjust instance capacity, which is vital to react to applications correctly.
Closing Remarks
Troubleshooting failed Auto Scaling Group deployments in AWS takes diligence and an understanding of multiple interlinked components. By following the outlined steps, you can pinpoint issues more effectively. Remember to leverage the available AWS tools and logs for deeper insights.
For more in-depth details on Auto Scaling Groups, refer to the AWS Auto Scaling User Guide and the AWS EC2 documentation.
With the right knowledge and approach, you'll be prepared to tackle any ASG issues confidently!
Checkout our other articles