Managing Multiple Property Files in Spring MVC Simplified
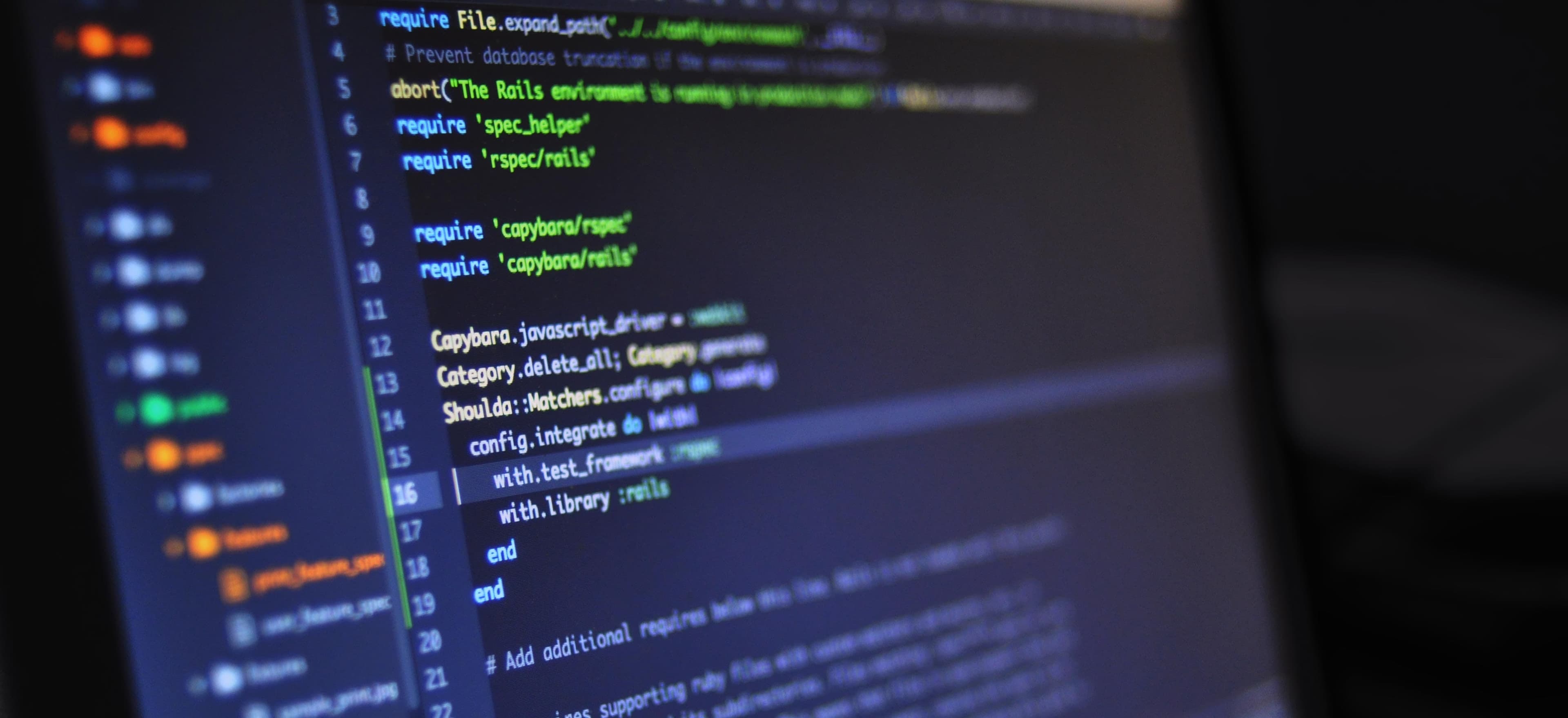
- Published on
Managing Multiple Property Files in Spring MVC Simplified
Managing configuration properties is a crucial aspect of developing applications using Spring MVC. Often times, applications grow and the need arises to manage multiple property files that serve different purposes (dev, test, prod). This blog post will break down how to effectively manage multiple property files in Spring MVC, ensuring that your application remains clean, organized, and maintainable.
Why Use Multiple Property Files?
- Environment-Specific Configurations: Different environments might have different settings (e.g., database connections, logging levels).
- Separation of Concerns: Group related properties together, like security settings, database configurations, and application-specific properties.
- Easy Maintenance: Easier to update and manage configurations when properties are segmented into logical groupings.
Getting Started with Spring MVC
To begin, you need a basic Spring MVC setup. If you don’t have one already, you can easily generate a project using Spring Initializr. Include dependencies for Spring Web, Thymeleaf (optional for views), and Spring Boot.
Basic Project Structure
Your project structure should look something like this:
src
└── main
├── java
│ └── com
│ └── example
│ └── demo
│ ├── DemoApplication.java
│ └── controller
│ └── HelloController.java
└── resources
├── application.properties
├── application-dev.properties
├── application-test.properties
└── application-prod.properties
Creating Property Files
Create several property files in the src/main/resources
directory:
- application.properties - Default configuration.
- application-dev.properties - Development-specific configurations.
- application-test.properties - Test-specific configurations.
- application-prod.properties - Production-specific configurations.
Example of Properties
Here’s an example of what these files might contain:
-
application.properties
server.port=8080 logging.level.root=INFO
-
application-dev.properties
spring.datasource.url=jdbc:mysql://localhost:3306/devdb spring.datasource.username=dev_user spring.datasource.password=dev_password
-
application-test.properties
spring.datasource.url=jdbc:mysql://localhost:3306/testdb spring.datasource.username=test_user spring.datasource.password=test_password
-
application-prod.properties
spring.datasource.url=jdbc:mysql://localhost:3306/proddb spring.datasource.username=prod_user spring.datasource.password=prod_password
Choosing Active Profiles
Spring allows you to specify which property file to load by using profiles. You can activate a profile through different methods:
-
application.properties
spring.profiles.active=dev
-
Command Line: You can specify the profile when starting the application:
mvn spring-boot:run -Dspring-boot.run.profiles=dev
-
Environment Variables: Set the environment variable:
export SPRING_PROFILES_ACTIVE=prod
Each method allows you to specify the active profile that dictates which property file Spring will utilize.
Accessing Properties in Code
Now that we have multiple property files set up, it's essential to know how to utilize these properties within your application. Below is a simple example of how to achieve this.
Example Java Code
1. Create a Configuration Class
Let’s create a configuration class that reads properties.
package com.example.demo.config;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.context.annotation.Configuration;
@Configuration
public class AppConfig {
@Value("${spring.datasource.url}")
private String datasourceUrl;
@Value("${spring.datasource.username}")
private String datasourceUsername;
@Value("${spring.datasource.password}")
private String datasourcePassword;
public String getDatasourceUrl() {
return datasourceUrl;
}
public String getDatasourceUsername() {
return datasourceUsername;
}
public String getDatasourcePassword() {
return datasourcePassword;
}
}
Why Use @Value?
Using @Value
allows you to inject property values directly into your classes. This promotes cleaner code and enhanced maintainability since changes in configuration can be made without modifying the Java code.
2. Using the Config Class in a Controller
Now let’s use this configuration in a controller:
package com.example.demo.controller;
import com.example.demo.config.AppConfig;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class HelloController {
@Autowired
private AppConfig appConfig;
@GetMapping("/config")
public String getConfig() {
return "Database URL: " + appConfig.getDatasourceUrl() +
", Username: " + appConfig.getDatasourceUsername();
}
}
Why Autowire Configurations?
By using dependency injection through @Autowired
, you ensure that your configuration is managed by the Spring container. This way, you can easily swap out configurations for testing without changing the implementation.
Utilizing Profiles for Different Environments
The real power comes when you can easily switch between your development, testing, and production configurations. When you set the active profile properly, Spring automatically picks the needed properties based on the environment.
Checklist to Remember
- Ensure your application is set up to include multiple configuration files.
- Change the active profile as necessary for your environment.
- Utilize
@Value
annotations for cleaner code. - Minimize hardcoding within your application.
Closing Remarks
Managing multiple property files in Spring MVC does not need to be a cumbersome task. By leveraging Spring's built-in support for profiles and property files, you can create a streamlined, organized application that is easy to maintain across different environments. This capability leads to efficient configuration management and a more productive development cycle.
For more detailed information on profile management, check the Spring official documentation on profiles.
Happy coding!