Overcoming CI/CD Challenges in Java Microservices
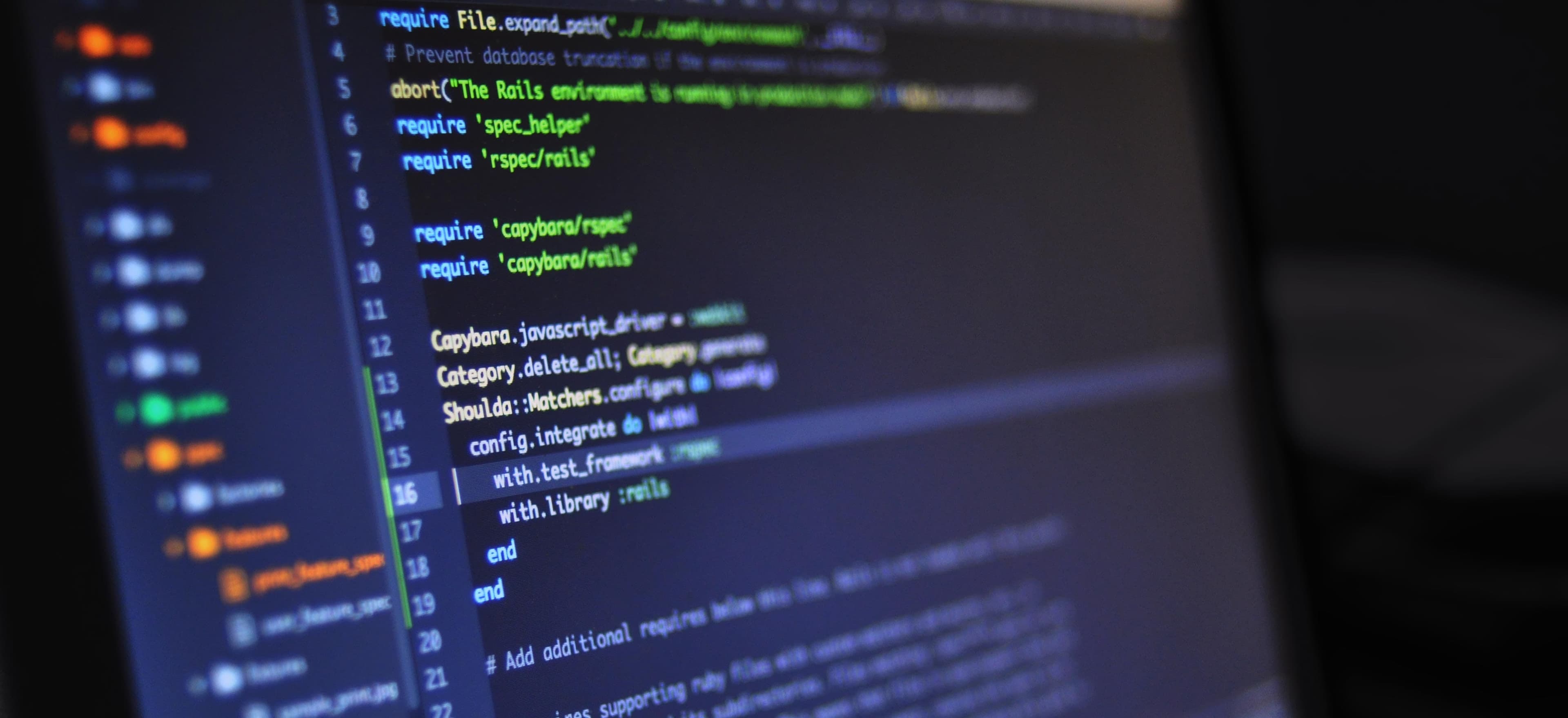
- Published on
Overcoming CI/CD Challenges in Java Microservices
Continuous Integration and Continuous Deployment (CI/CD) are essential practices in modern development workflows, particularly for Java microservices. While the benefits of CI/CD are clear — frequent releases, high-quality code, and quick feedback loops — implementing robust CI/CD pipelines can present unique challenges. This post delves into the common obstacles faced when implementing CI/CD in Java microservices and offers actionable strategies to overcome them.
Understanding CI/CD
CI/CD is a method that combines Continuous Integration, Continuous Deployment, and Continuous Delivery. Here’s a brief overview:
- Continuous Integration (CI): CI involves regularly merging code changes into a central repository, which triggers automated builds and tests. This ensures that new changes do not introduce bugs.
- Continuous Deployment (CD): CD focuses on automatically deploying every change that passes the CI pipeline to production. This allows teams to release features rapidly.
- Continuous Delivery (CD): Sometimes confused with Continuous Deployment, Continuous Delivery ensures that code is always in a deployable state but requires manual intervention to deploy.
For an in-depth understanding of CI/CD, refer to Atlassian's CI/CD overview.
Common CI/CD Challenges in Java Microservices
Despite the advantages of CI/CD, many organizations face significant challenges, including:
- Complexity of Microservices Architecture
- Dependency Management
- Environment Parity
- Monitoring and Logging
- Build Time and Performance Issues
Let’s explore each challenge in detail and discuss strategies for overcoming them.
1. Complexity of Microservices Architecture
Challenge: Java microservices involve various services that communicate over the network. This complexity can lead to difficulties in integration testing.
Solution: Implement contract testing. Contract testing ensures that the API interactions between microservices function as expected. You can use tools like Pact for this purpose.
Code Snippet: Here’s how you can use Pact in a Spring Boot microservice.
import au.com.dius.pact.consumer.junit.PactConsumerTestExt;
import au.com.dius.pact.consumer.junit.loader.PactFolder;
import org.junit.jupiter.api.Test;
import org.junit.jupiter.api.extension.ExtendWith;
@PactFolder("pacts")
@ExtendWith(PactConsumerTestExt.class)
public class UserServicePactTest {
@Pact(consumer = "user-service", provider = "payment-service")
public RequestResponsePact createPact(PactDslWithProvider builder) {
return builder
.uponReceiving("Request to get user")
.path("/user/1")
.method("GET")
.willRespondWith()
.status(200)
.body(new PactDslJsonBody()
.numberType("id", 1)
.stringType("name", "John Doe"))
.toPact();
}
// Test method
@Test
public void testGetUser() {
// Your test implementation here
}
}
Why This Works: The above code sets up a contract test that ensures the user-service
correctly interacts with the payment-service
. By verifying that expectations are met before deployment, you minimize integration issues later.
2. Dependency Management
Challenge: Microservices often have numerous dependencies, which can complicate builds and deployments.
Solution: Employ tools like Maven or Gradle for dependency management. Make sure each microservice has its own pom.xml
or build.gradle
file for isolated dependencies.
Example of Maven Dependency Declaration:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
<version>2.5.0</version>
</dependency>
Why This Matters: Using a dependency management tool simplifies the process of managing libraries, making it easier to specify versions, and reducing conflicts across microservices.
3. Environment Parity
Challenge: Ensuring that every environment (development, testing, production) resembles one another can be daunting, as differences can lead to unexpected issues.
Solution: Use containerization, primarily through Docker. Docker allows developers to package an application and its dependencies into a container that can run consistently across multiple environments.
Dockerfile Example:
FROM openjdk:11-jre-slim
RUN mkdir /app
COPY target/my-java-microservice.jar /app/my-java-microservice.jar
CMD ["java", "-jar", "/app/my-java-microservice.jar"]
Why Docker: This Dockerfile describes a simple way to package a Java microservice, ensuring that it runs the same in development and production.
4. Monitoring and Logging
Challenge: Lack of proper monitoring can lead to unnoticed issues in a microservices architecture. Due to distributed systems, tracing problems can be time-consuming.
Solution: Implement centralized logging and monitoring using tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Prometheus combined with Grafana.
Example Configuration for Micrometer:
management:
metrics:
export:
prometheus:
enabled: true
Why This is Important: By aggregating logs and metrics, you can gain insight into system performance and quickly identify fault patterns across multiple services.
5. Build Time and Performance Issues
Challenge: Large projects can lead to long build times, which hampers developer productivity and slows down the CI/CD pipeline.
Solution: Optimize the build process by using incremental builds, parallel execution, or caching dependencies.
Example of Enabling Caching in Gradle:
buildCache {
local {
enabled = true
}
}
Why Optimization Matters: Caching speeds up repetitive tasks, allowing developers to focus more on writing code rather than waiting for builds to complete.
Best Practices for CI/CD in Java Microservices
- Automate Everything: Automate build, test, and deployment processes to reduce human error.
- Branch Strategy: Use feature branches and trunk-based development to manage code changes effectively.
- Frequent Releases: Release small increments regularly rather than massive updates. This minimizes risk and simplifies troubleshooting.
- Documentation: Maintain clear documentation for setup, dependencies, and API contracts to ease onboarding for new developers.
Wrapping Up
Implementing CI/CD for Java microservices comes with its challenges, but with the right strategies and tools, organizations can overcome these hurdles. By focusing on contract testing, dependency management, consistent environments, proactive monitoring, and build optimization, you can create a resilient and efficient CI/CD pipeline.
For further reading and deep dives into the practices mentioned, consider checking out Martin Fowler's resources on CI/CD and Effective Microservices.
By tackling these CI/CD challenges head-on, your Java microservices can flourish, leading to enhanced productivity and an improved development experience.