Mastering Switch Expressions: Common Pitfalls to Avoid
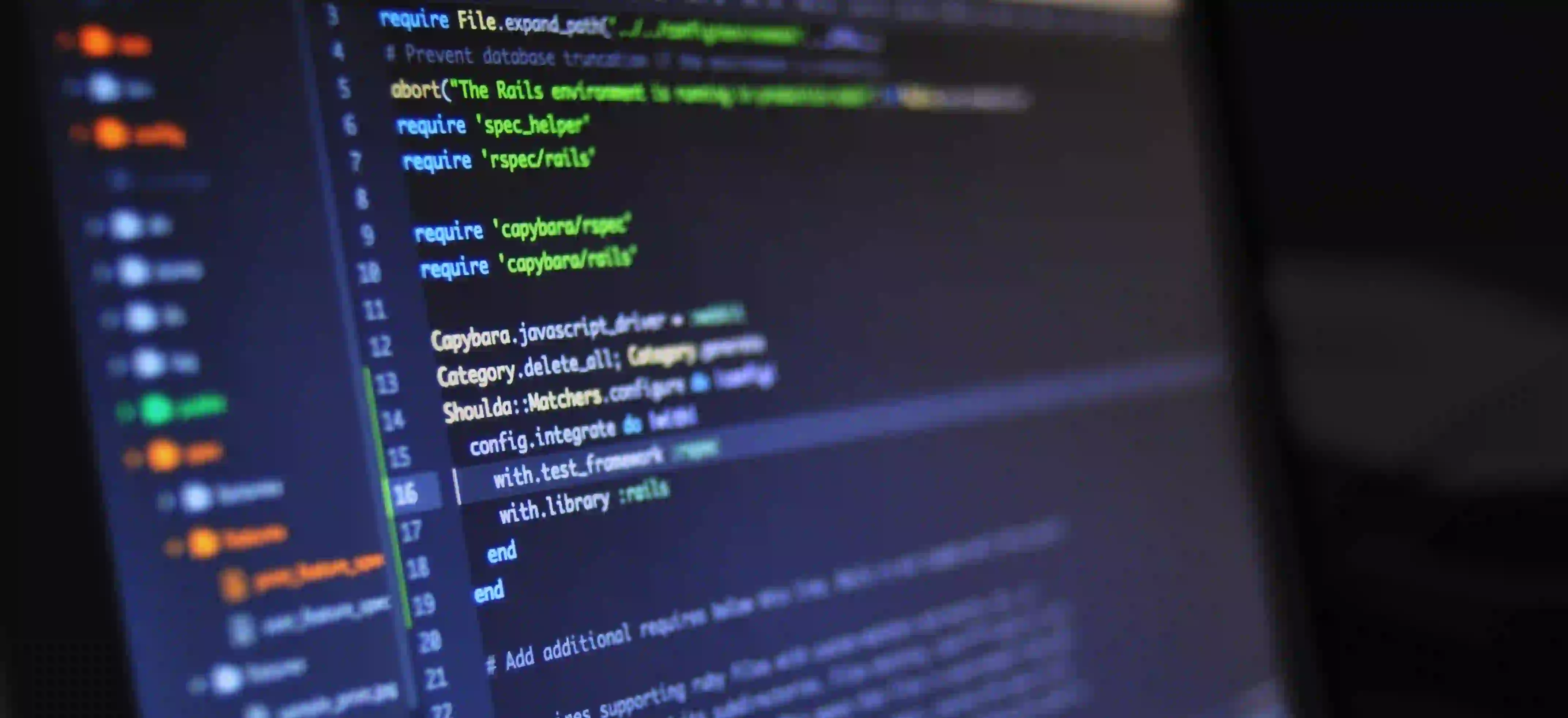
Mastering Switch Expressions: Common Pitfalls to Avoid
Java has evolved tremendously over the years. One of the most notable additions to the language has been the introduction of switch expressions in Java 12 and their subsequent enhancements. These features make code more concise and readable, revolutionizing the way developers handle conditional logic. However, like all powerful features, they come with their own set of challenges. In this blog post, we will delve into the common pitfalls while using switch expressions and how to avoid them, ensuring that your Java code remains robust and efficient.
Understanding Switch Expressions
Before diving into pitfalls, let's clarify what switch expressions are. A switch expression is an enhancement of the traditional switch statement, enabling more concise and expressive conditional statements.
Basic Syntax
Here’s a simple example of a switch expression in action:
String dayType = switch (day) {
case MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY -> "Weekday";
case SATURDAY, SUNDAY -> "Weekend";
};
In this snippet, the switch
statement evaluates day
, returning "Weekday" or "Weekend" based on the value. The ->
operator is a critical component, marking the return value for each case. This syntax simplifies the structure and improves readability compared to the traditional switch
statement.
Why Use Switch Expressions?
Switch expressions offer several advantages:
- Conciseness: Reduces boilerplate code.
- Return Values: Directly return values without additional variable declarations.
- Control Fall-Through: Eliminates errors from unintentional fall-through.
Common Pitfalls and How to Avoid Them
While switch expressions bring many benefits, they also introduce some pitfalls. Let’s explore some of these common issues and how to sidestep them.
1. Missing Default Case
Pitfall: Not using a default case can lead to unexpected behavior when none of the cases match.
Example:
String status = switch (orderStatus) {
case "SHIPPED" -> "Your order has been shipped";
case "DELIVERED" -> "Your order has been delivered";
};
Why It's a Problem: If orderStatus
is neither "SHIPPED" nor "DELIVERED", the value of status
will be uninitialized, which may lead to NullPointerException
.
Solution: Always include a default case.
String status = switch (orderStatus) {
case "SHIPPED" -> "Your order has been shipped";
case "DELIVERED" -> "Your order has been delivered";
default -> "Unknown order status";
};
2. Improper Use of Blocks
Pitfall: Using blocks where they aren’t necessary can add unnecessary complexity.
Example:
String grade = switch (score) {
case 90, 100 -> {
System.out.println("Excellent");
yield "A";
}
case 80 -> {
System.out.println("Good");
yield "B";
}
default -> "F";
};
Why It's a Problem: The use of blocks and yield
adds complexity and verbosity when it can be avoided.
Solution: Use single expressions wherever possible.
String grade = switch (score) {
case 90, 100 -> "A";
case 80 -> "B";
default -> "F";
};
3. Inefficient Case Grouping
Pitfall: Creating excessively lengthy case statements without grouping can lead to redundancy.
Example:
String dayType = switch (day) {
case "MONDAY" -> "Weekday";
case "TUESDAY" -> "Weekday";
case "WEDNESDAY" -> "Weekday";
case "THURSDAY" -> "Weekday";
case "FRIDAY" -> "Weekday";
case "SATURDAY" -> "Weekend";
case "SUNDAY" -> "Weekend";
};
Why It's a Problem: This approach is repetitive and less maintainable.
Solution: Use case grouping.
String dayType = switch (day) {
case "MONDAY", "TUESDAY", "WEDNESDAY", "THURSDAY", "FRIDAY" -> "Weekday";
case "SATURDAY", "SUNDAY" -> "Weekend";
};
4. Not Leveraging Enum Types
Pitfall: Not using enum types can lead to code that is less clean and harder to manage.
Example:
String fruitType = switch (fruit) {
case "APPLE" -> "Red or Green";
case "BANANA" -> "Yellow";
case "GRAPE" -> "Green or Purple";
};
Why It's a Problem: If you make a typo in the string, the switch logic might not work as expected.
Solution: Utilize enums for better type safety.
public enum Fruit {
APPLE, BANANA, GRAPE;
}
String fruitType = switch (fruit) {
case APPLE -> "Red or Green";
case BANANA -> "Yellow";
case GRAPE -> "Green or Purple";
};
5. Failing to Handle Null Values
Pitfall: Ignoring the possibility of null values can lead to runtime exceptions.
Example:
String response = switch (userInput) {
case "YES" -> "User confirmed.";
case "NO" -> "User declined.";
};
Why It's a Problem: If userInput
is null, the switch expression will throw a NullPointerException
.
Solution: Handle possible null values explicitly.
String response = switch (userInput != null ? userInput : "UNKNOWN") {
case "YES" -> "User confirmed.";
case "NO" -> "User declined.";
default -> "Input was null or unrecognized.";
};
Wrapping Up
Switch expressions have significantly simplified how we write conditional logic in Java. By being aware of the common pitfalls and understanding how to avoid them, you can utilize this feature effectively, leading to cleaner and more maintainable code.
As Java continues to evolve, staying updated with best practices will ensure that you keep pace with new features while preventing common issues. For more on Java enhancements, check out the Java Tutorials and Java Platform, Standard Edition documentation.
Happy coding, and may your switch expressions be concise and effective!