Overcoming Latency Issues in Modern Application Performance
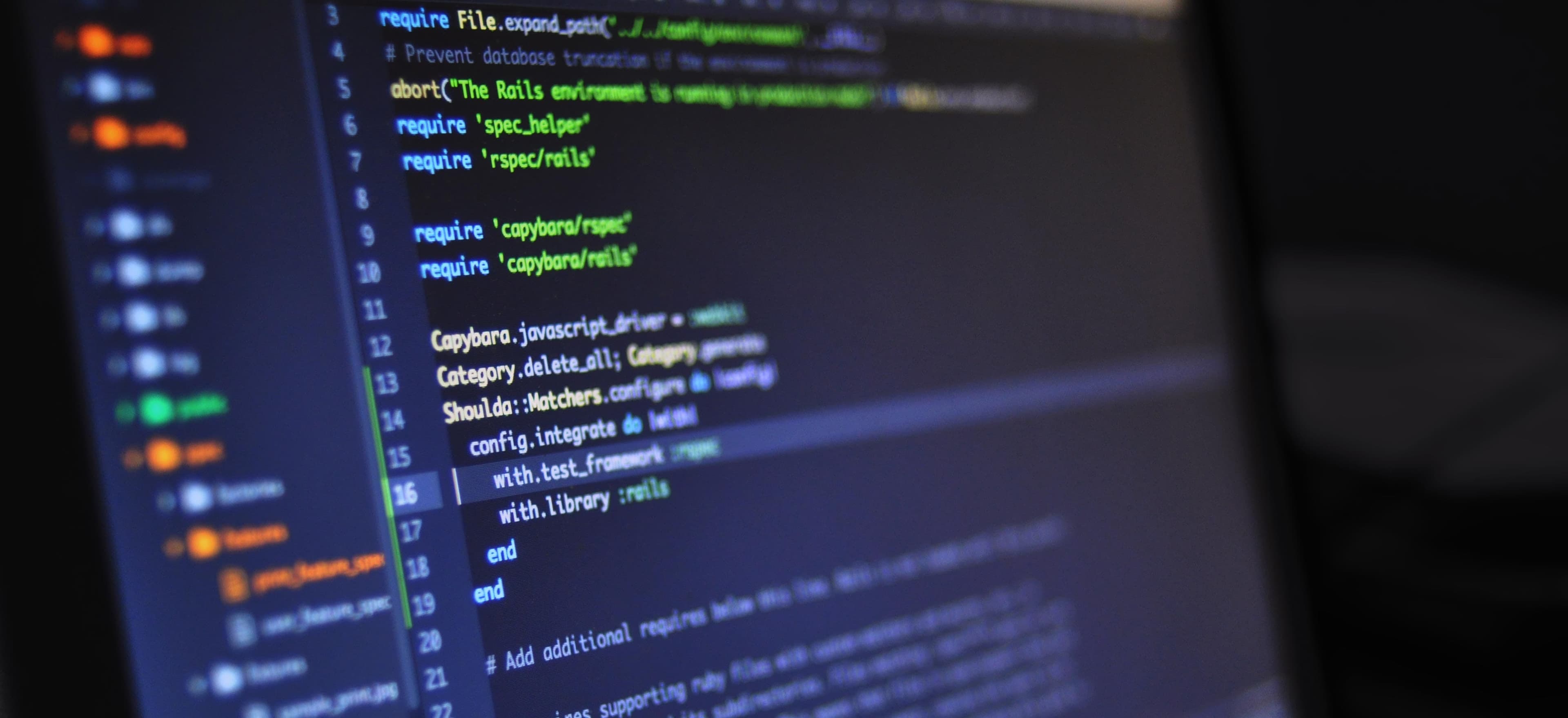
- Published on
Overcoming Latency Issues in Modern Application Performance
In today's fast-paced digital environment, latency is a critical aspect that can hinder application performance. Users expect applications to be responsive and fast, making it essential for developers to address any latency challenges proactively. In this blog post, we will explore common latency issues in modern applications, ways to optimize performance, and specific techniques in Java to mitigate these challenges.
Understanding Latency
Latency refers to the time taken for data to travel from its source to its destination and back again. Various factors contribute to latency, including network speed, server response time, and application logic. The impact of latency can be particularly pronounced in web applications, mobile applications, and microservices architectures.
Major Factors Contributing to Latency
-
Network Latency: The physical distance between the client and server, as well as how data packets traverse the internet, can influence latencies.
-
Server Response Time: The time taken by a server to process a request and return a response. This can be influenced by server load, application logic, or database queries.
-
Client Processing Time: The time taken on the user's device to render or process content can also contribute to delays.
-
Data Serialization: Transforming complex data structures into a format suitable for transmission can introduce latencies, especially with large payloads.
-
Third-Party Services: Calls to external APIs or services can add latency beyond your control.
Strategies to Overcome Latency Issues
1. Minimize Network Latency
Reducing network latency begins with understanding user geography and deploying strategies like content delivery networks (CDNs). CDNs decrease the distance between the user and the server, thus speeding up data delivery. Additionally, leveraging HTTP/2 can improve performance by enabling multiplexing.
import java.net.HttpURLConnection;
import java.net.URL;
public class NetworkUtils {
public static void sendHttpRequest(String url) throws Exception {
URL obj = new URL(url);
HttpURLConnection connection = (HttpURLConnection) obj.openConnection();
connection.setRequestMethod("GET");
System.out.println("Response Code : " + connection.getResponseCode());
}
}
In this example, the sendHttpRequest
method establishes a connection to a URL, improving network performance management.
2. Optimize Server Response Time
Server-side optimizations are critical in reducing response time. You can achieve optimization through:
-
Efficient Algorithms: Use efficient data structures and algorithms to handle requests. For instance, you can utilize a HashMap for quick lookups versus an ArrayList for slow traversals.
-
Database Optimization: Regularly monitor and optimize your database queries. Use indexing effectively to speed up retrieval times.
-
Caching: Employ caching mechanisms to store frequently accessed data. In Java applications, consider using caching libraries like Ehcache or Caffeine:
import com.github.benmanes.caffeine.cache.Cache;
import com.github.benmanes.caffeine.cache.Caffeine;
public class CacheDemo {
private static Cache<String, String> cache = Caffeine.newBuilder()
.maximumSize(10000)
.expireAfterAccess(10, TimeUnit.MINUTES)
.build();
public static void cacheData(String key, String value) {
cache.put(key, value);
}
public static String getData(String key) {
return cache.getIfPresent(key);
}
}
This code demonstrates how you can easily implement caching using Caffeine, which can drastically reduce the time taken to retrieve data.
3. Enhance Client-Side Performance
Client-side performance can usually be improved by avoiding heavy processing tasks in the main thread. Techniques include:
-
Lazy Loading: Only load images or components as they come into the viewport, saving resources during initial load times.
-
Asynchronous Processing: Using JavaScript's Promises or async/await to handle long-running processes helps keep the UI responsive.
4. Efficient Data Serialization
Overcoming serialization latency is also paramount. Consider using formats like JSON or Protocol Buffers which ensure quicker serialization and deserialization than XML. Java provides libraries like Jackson for handling JSON seamlessly:
import com.fasterxml.jackson.databind.ObjectMapper;
public class JsonUtil {
private static final ObjectMapper objectMapper = new ObjectMapper();
public static String serialize(Object object) throws Exception {
return objectMapper.writeValueAsString(object);
}
public static <T> T deserialize(String json, Class<T> clazz) throws Exception {
return objectMapper.readValue(json, clazz);
}
}
This utility class allows you to serialize and deserialize objects efficiently using Jackson, thus reducing latency during data transmission.
5. Monitor Third-Party Services
When using third-party APIs, it’s essential to monitor their response times and ensure they don’t degrade your application’s performance. Implement error handling and timeout strategies so that your application can gracefully handle external failures.
Measuring Latency and Performance
Monitoring performance and latency is essential for continuous improvement. You could use tools like JMeter for load testing or APM (Application Performance Management) tools like New Relic or AppDynamics to get insights into your application’s performance.
Monitoring meaningful metrics such as:
- Average Response Time
- Error Rate
- Throughput
These can guide you in identifying bottlenecks and areas for enhancement.
The Closing Argument
Latency issues can severely impact user experience and the overall performance of applications. By understanding the sources of latency and employing strategic techniques, you can significantly enhance the responsiveness of your Java applications.
Optimization is not a one-time process; you must continually monitor, analyze, and refine your strategies as user expectations and technologies evolve.
Whether it’s through improved network strategies, backend optimizations, or smarter client-side manipulations, the key is to remain proactive in managing performance. To delve deeper into Java optimization techniques, consider exploring resources like Oracle's Java Performance Tuning and Effective Java for in-depth insights.
Embrace these practices, and watch as your applications become faster and more reliable, ultimately leading to happier users and successful outcomes.