Mastering Java's Deferred Execution with Predicates
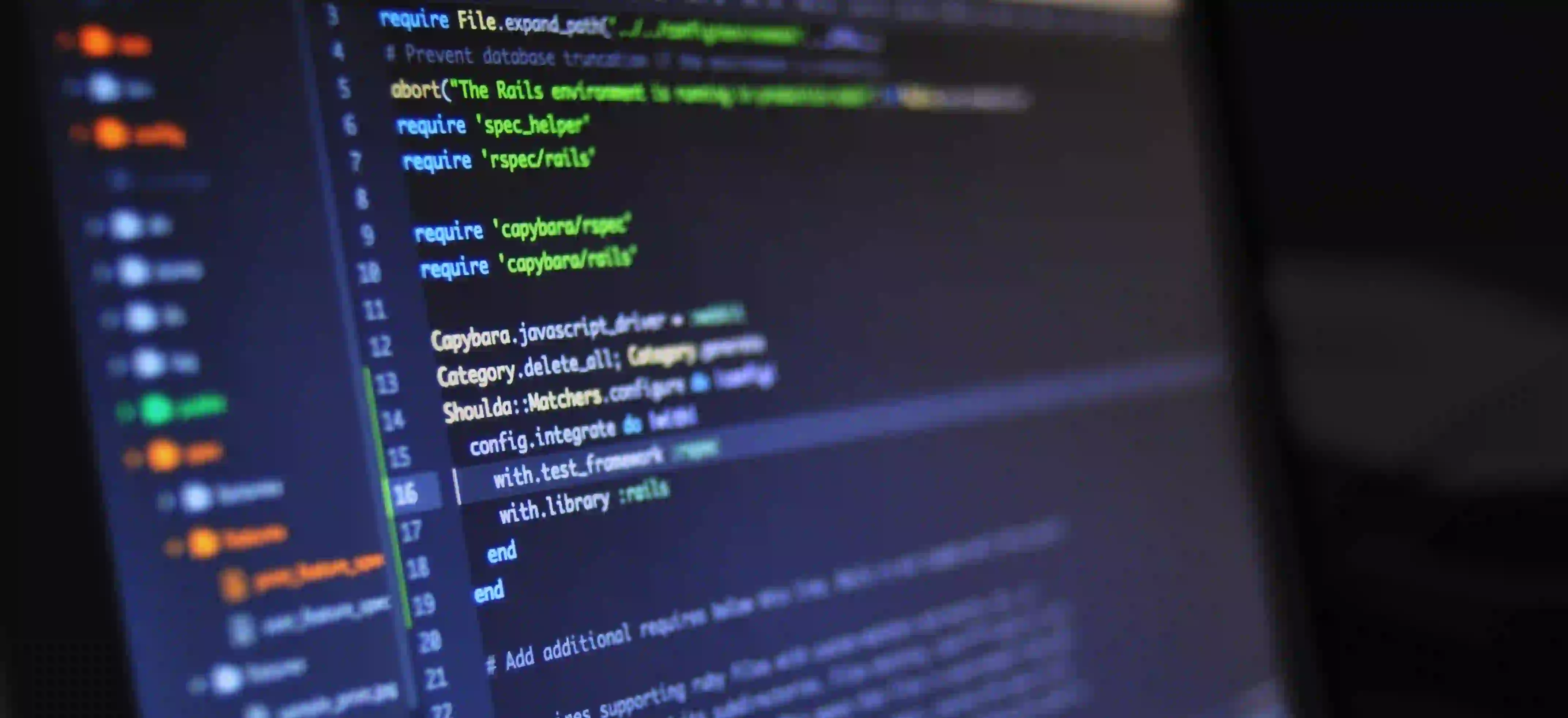
Mastering Java's Deferred Execution with Predicates
Java has evolved significantly over the years, enabling developers to write cleaner and more efficient code. One of the essential concepts that has emerged in this evolution is deferred execution, particularly through the use of predicates. This blog post will explore what deferred execution is, how predicates facilitate it, and practical examples to cement the concepts in a Java context.
What is Deferred Execution?
Deferred execution refers to delaying the evaluation of an expression until its value is actually needed. This concept is widely used in functional programming and allows developers to optimize performance, avoid unnecessary computations, and improve code readability. In Java, the Predicate
interface plays a vital role in achieving this.
Understanding the Predicate Interface
The Predicate
interface is part of the Java Functional Interfaces, introduced in Java 8. It represents a single argument function that returns a boolean value. This characteristic makes it especially useful for filtering collections, making decisions, and creating reusable conditions.
Basic Syntax of a Predicate
import java.util.function.Predicate;
Predicate<Integer> isEven = number -> number % 2 == 0;
In the above snippet, we define a predicate that checks if a number is even. The expression number % 2 == 0
will only be evaluated when this predicate is invoked.
Why Use Predicates?
- Deferred Evaluation: Predicates can delay the execution of code, ensuring that conditions are only tested when necessary.
- Reusability: Once defined, a predicate can be reused across different parts of your application.
- Composability: Predicates can be combined using logical operations, leading to more expressive and concise code.
Now, let’s explore practical applications of predicates and deferred execution in Java.
Filtering Collections Using Predicates
One of the most common uses of predicates in Java is to filter collections. The Stream
API introduced in Java 8 allows for efficient processing of sequences of elements.
Example: Filtering a List
Let’s take a look at how to filter even numbers from a list using a predicate.
import java.util.ArrayList;
import java.util.List;
import java.util.function.Predicate;
import java.util.stream.Collectors;
public class FilterExample {
public static void main(String[] args) {
List<Integer> numbers = List.of(1, 2, 3, 4, 5, 6);
Predicate<Integer> isEven = number -> number % 2 == 0;
List<Integer> evenNumbers = filterNumbers(numbers, isEven);
System.out.println(evenNumbers); // Output: [2, 4, 6]
}
public static List<Integer> filterNumbers(List<Integer> numbers, Predicate<Integer> predicate) {
return numbers.stream()
.filter(predicate)
.collect(Collectors.toList());
}
}
Explanation
- Defining a Predicate: The
isEven
predicate checks if a number is even. - Filtering: The
filterNumbers
method applies the predicate, taking advantage of the deferred execution via thefilter
method of theStream
API. - Efficiency: Only numbers that meet the condition are processed.
Composing Predicates
Predicates can also be composed to create more complex conditions. The and
, or
, and negate
methods allow you to combine multiple predicates seamlessly.
Example: Composing Predicates
import java.util.List;
import java.util.function.Predicate;
import java.util.stream.Collectors;
public class ComposePredicates {
public static void main(String[] args) {
List<Integer> numbers = List.of(1, 2, 3, 4, 5, 6);
Predicate<Integer> isEven = number -> number % 2 == 0;
Predicate<Integer> isGreaterThanTwo = number -> number > 2;
Predicate<Integer> combinedPredicate = isEven.and(isGreaterThanTwo);
List<Integer> result = filterNumbers(numbers, combinedPredicate);
System.out.println(result); // Output: [4, 6]
}
public static List<Integer> filterNumbers(List<Integer> numbers, Predicate<Integer> predicate) {
return numbers.stream()
.filter(predicate)
.collect(Collectors.toList());
}
}
Explanation
- Combining Predicates: The
combinedPredicate
leverages theand
method to check if numbers are both even and greater than two. - Readable: This approach improves readability and maintains separation of concerns. Each predicate serves a single purpose.
Real-World Use Cases
Using predicates for deferred execution is not just a theoretical exercise; there are numerous practical scenarios where it shines.
Example: Database Query Filtering
When dealing with a large dataset or database queries, using predicates allows you to avoid loading unnecessary data. Instead, you can apply filtering based on user-defined conditions.
Example: UI Element Filtering
In user interfaces, predicates can filter items based on user input, enhancing responsiveness without incurring unnecessary load times.
Additional Resources
For those looking to delve deeper into functional programming in Java, consider exploring these resources:
- Java Streams Tutorial - A comprehensive guide to working with the Stream API in Java.
- Understanding Functional Interfaces - An overview of functional interfaces in Java, including predicates.
Final Thoughts
Mastering Java's deferred execution using predicates can significantly enhance the performance of your applications. It allows you to write cleaner, more maintainable code while ensuring that computations are only performed when necessary. By leveraging predicates effectively—filtering collections, composing conditions, and applying them judiciously—you can elevate your Java programming skills to new heights.
Whether you are new to Java or looking to sharpen your skills, understanding deferred execution and predicates will immensely benefit your coding practices. Embrace these concepts, and watch your programming efficiency soar!