Common Mistakes When Using ListView in Android Apps
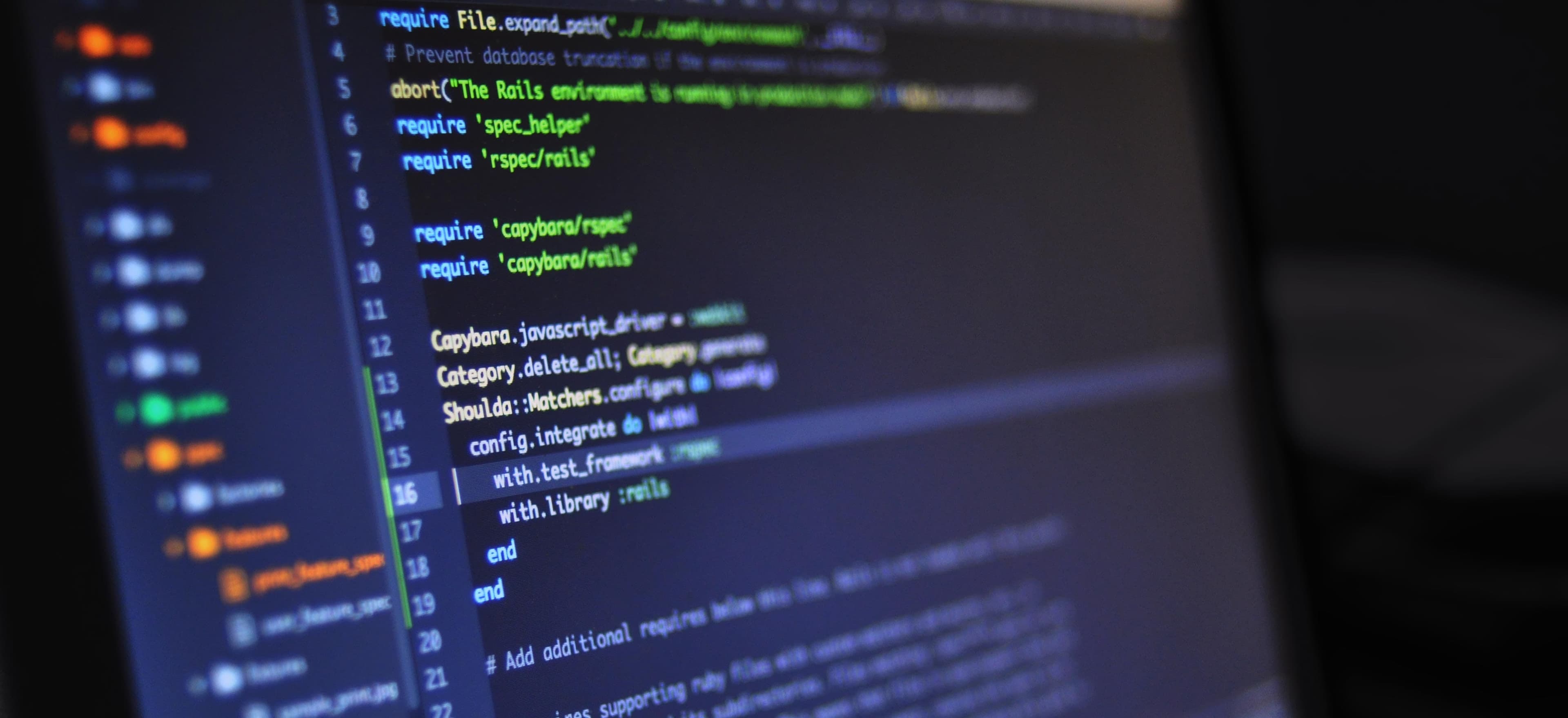
- Published on
Common Mistakes When Using ListView in Android Apps
ListView has been a staple of Android development for many years, allowing developers to create scrollable lists with ease. Despite its popularity, developers often run into pitfalls that can drastically affect performance and user experience. In this post, we'll explore some common mistakes when using ListView in Android applications and how to avoid them. By the end, you'll have a clearer understanding of best practices when working with ListView.
What is ListView?
ListView is a view group in Android that displays items in a vertically scrollable list. Unlike RecyclerView, ListView is a traditional component that has been around since Android's early days. It uses adapters to bind data to UI components, allowing for dynamic list content.
Common Mistakes When Using ListView
1. Not Recycling Views Properly
One of the biggest advantages of using ListView is its ability to recycle views for improved performance. Each time a row in the list scrolls off the screen, the view associated with it can be reused for a new row that comes into view.
Mistake
Failing to implement view recycling correctly will lead to performance issues. For example, if new views are created every time a cell is drawn, it can cause noticeable lag in your app.
Solution
Utilize the ViewHolder
pattern. This minimizes the number of calls to findViewById
, which is expensive in terms of performance. Here's how you can implement that:
public class MyAdapter extends BaseAdapter {
private final Context context;
private final List<MyData> dataList;
public MyAdapter(Context context, List<MyData> dataList) {
this.context = context;
this.dataList = dataList;
}
@Override
public int getCount() {
return dataList.size();
}
@Override
public Object getItem(int position) {
return dataList.get(position);
}
@Override
public long getItemId(int position) {
return position;
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
ViewHolder holder;
if (convertView == null) {
convertView = LayoutInflater.from(context).inflate(R.layout.list_item, parent, false);
holder = new ViewHolder();
holder.textView = convertView.findViewById(R.id.textView);
convertView.setTag(holder);
} else {
holder = (ViewHolder) convertView.getTag();
}
MyData data = dataList.get(position);
holder.textView.setText(data.getText());
return convertView;
}
static class ViewHolder {
TextView textView;
}
}
In this example, we check if convertView
is null
. If it is, we inflate a new layout and set up the ViewHolder
. This enables the ListView to efficiently use the views as they scroll off-screen.
2. Using ListView Without an Adapter
Mistake
One of the most glaring mistakes is trying to implement ListView without using an adapter. Some developers underestimate the role of the adapter in binding data to the ListView.
Solution
Always use an adapter! Whether it’s ArrayAdapter
, SimpleCursorAdapter
, or a custom adapter like the one demonstrated above, ensure to bind your data sources appropriately.
ListView listView = findViewById(R.id.listView);
MyAdapter adapter = new MyAdapter(this, myDataList);
listView.setAdapter(adapter);
3. Not Handling Item Click Events
Another common oversight is neglecting to handle item click events, leading to a poor user experience.
Mistake
Not implementing item click listeners leaves users without interactivity, defeating the purpose of using a list.
Solution
Use setOnItemClickListener
to define what happens when a user clicks on a list item:
listView.setOnItemClickListener(new AdapterView.OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> parent, View view, int position, long id) {
MyData clickedData = dataList.get(position);
Toast.makeText(context, "Clicked: " + clickedData.getText(), Toast.LENGTH_SHORT).show();
}
});
Implementing click listeners not only enhances user engagement but also makes your app feel more interactive.
4. Forgetting to Optimize for Large Data Sets
When dealing with a large number of items, failing to optimize your ListView can lead to sluggish performance.
Mistake
With large datasets, apps can slow down significantly if you do not apply pagination or lazy loading techniques.
Solution
Consider implementing pagination to load items in chunks or using a background thread for setting data into the adapter.
For instance, you could load the first 20 items and then pull more as the list is scrolled:
listView.setOnScrollListener(new AbsListView.OnScrollListener() {
boolean isLoading = false;
@Override
public void onScrollStateChanged(AbsListView view, int scrollState) { }
@Override
public void onScroll(AbsListView view, int firstVisibleItem, int visibleItemCount, int totalItemCount) {
if (totalItemCount > 0 && (firstVisibleItem + visibleItemCount >= totalItemCount - 5) && !isLoading) {
isLoading = true;
loadMoreData();
}
}
private void loadMoreData() {
// Load next batch of items
isLoading = false;
}
});
This would enhance the user experience by ensuring smooth scrolling, even with extensive datasets.
5. Neglecting to Handle Configuration Changes
Android apps can experience configuration changes, such as screen rotations, affecting the ListView state.
Mistake
Assuming that your ListView will maintain its state automatically is a misconception that can lead to frustration for users. Data can get lost if not handled.
Solution
Use onSavedInstanceState
and onRestoreInstanceState
methods to retain the state of ListView across configuration changes.
@Override
protected void onSaveInstanceState(Bundle outState) {
super.onSaveInstanceState(outState);
outState.putParcelableArrayList("myDataList", (ArrayList<? extends Parcelable>) dataList);
}
@Override
protected void onRestoreInstanceState(Bundle savedInstanceState) {
super.onRestoreInstanceState(savedInstanceState);
dataList = savedInstanceState.getParcelableArrayList("myDataList");
adapter.notifyDataSetChanged();
}
6. Incorrect Use of Layout Parameters
Improper layout parameters can disrupt how ListView displays its items, affecting both aesthetics and usability.
Mistake
Using inconsistent or incompatible layout parameters could lead to misaligned or improperly sized views.
Solution
Define appropriate layout parameters for the ListView and its item views, using match_parent or wrap_content carefully based on the requirements.
<ListView
android:id="@+id/listView"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
This sets the ListView to take up the full width while adapting its height to match its contents.
To Wrap Things Up
ListView remains a highly effective way to display lists of data in Android applications. However, avoiding the common mistakes outlined above is essential for optimal performance and user experience. From properly recycling views and implementing adapters to ensuring robust error handling during configuration changes, employing best practices will undoubtedly lead to smoother, more responsive applications.
For more information and advanced topics concerning Android development, consider checking out the official Android documentation and explore how they are evolving towards modern alternatives like RecyclerView.
If you’re new to Android development or looking to deepen your knowledge, enhancing your grasp on ListView and its correct usage can significantly contribute to your skillset. So, take these insights and apply them to your projects, ensuring not only functionality but also a seamless user experience.