Troubleshooting Common Issues with Spring Cloud Config Server
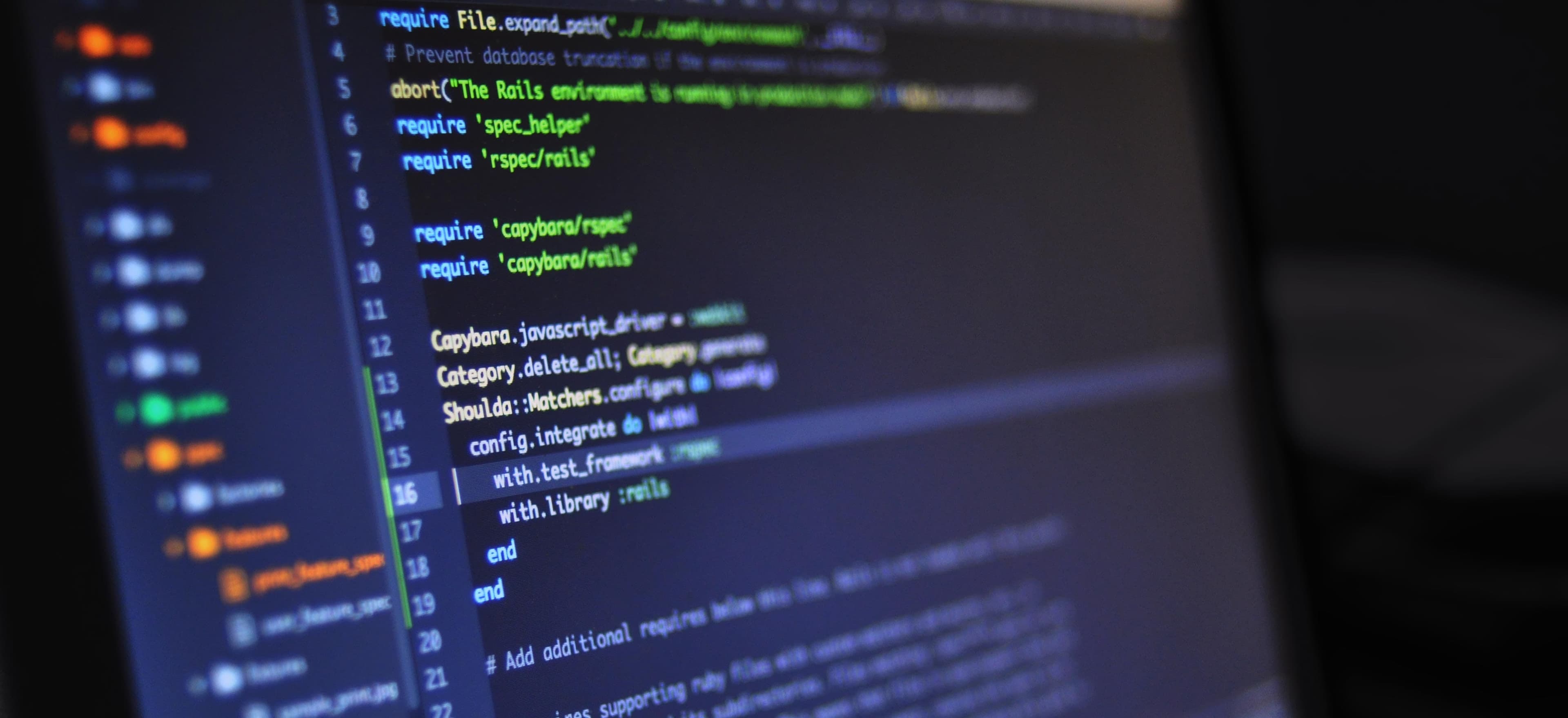
- Published on
Troubleshooting Common Issues with Spring Cloud Config Server
In today's microservices architecture, managing application configurations centrally is crucial. Spring Cloud Config Server provides a powerful solution for managing distributed system configurations. However, as with any advanced technology, issues may arise. In this blog post, we will discuss common problems encountered with Spring Cloud Config Server, along with troubleshooting techniques.
What is Spring Cloud Config Server?
Spring Cloud Config Server is part of the Spring Cloud suite and offers server-side and client-side support for externalized configuration in a distributed system. It allows applications to fetch configuration properties from a centralized server rather than hard-coding them.
Package and Dependency Setup
Before diving into troubleshooting, ensure you have the necessary dependencies set up correctly in your pom.xml
file if you're using Maven:
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-config-server</artifactId>
</dependency>
Additionally, include the Spring Cloud Dependencies:
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-dependencies</artifactId>
<version>2021.0.0</version>
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
</dependencyManagement>
Ensure your application is up to date with the latest Spring Cloud version. To find the current versions, check the official Spring Cloud Release Notes.
Common Issues and Troubleshooting Steps
1. Configuration Retrieval Failure
Issue: The application fails to retrieve configuration properties from the Config Server.
Solution: Check your application properties. Ensure you've specified the correct URL for the Config Server. Here's how you can define it:
spring.cloud.config.uri=http://localhost:8888
Why: If the URL is incorrect, your application cannot locate the Config Server, leading to retrieval failure.
Additionally, ensure that your config repository (like Git) is accessible and that the connection parameters are correct.
2. Git Repository Access Issues
Issue: Errors occur when the Config Server tries to access the repository.
Solution: If you're using a Git backend, validate your access permissions and authentication.
Here’s a sample configuration for Git in your application properties:
spring.cloud.config.server.git.uri=https://github.com/username/config-repo
spring.cloud.config.server.git.clone-on-start=true
You may also need to provide credentials:
spring.cloud.config.server.git.username=<your-username>
spring.cloud.config.server.git.password=<your-token>
Why: Authentication issues lead to failed attempts to access the configuration repository.
3. Inconsistent Profiles
Issue: The application is loading unexpected configurations, often due to incorrect profiles.
Solution: Review the active profiles set in your application. It can be specified in multiple ways—such as environment variables or application properties.
To set profiles explicitly, use this in your application.properties
:
spring.profiles.active=dev
Ensure that your configuration files are structured accordingly in your repository. For example, you should have files like application-dev.yml
, application-prod.yml
, etc.
Why: If the active profile doesn't match the configuration file names, the Config Server won't load the correct values.
4. Network Issues
Issue: Network issues can prevent your application from reaching the Config Server.
Solution: Check your networking configuration, including firewalls and proxies that may be blocking access. You can test connectivity using cURL by running a command like:
curl http://localhost:8888/application/default
Why: Even if your configurations are correct, network connectivity plays a critical role in ensuring your application can communicate with the Config Server.
5. Caching Issues
Issue: The application receives outdated properties due to caching.
Solution: Spring Cloud Config Server caches configuration properties by default. You can disable caching for development purposes as follows:
spring.cloud.config.server.git.refresh-interval=0
When running in production, consider setting a refresh interval that suits your system's needs to ensure new configurations are pulled more frequently.
Why: Caching can lead to inconsistencies if configurations are updated but the application isn't aware of these changes.
6. Cross-Origin Resource Sharing (CORS) Issues
Issue: CORS issues arise when the client application fails to retrieve configuration due to browser restrictions.
Solution: Enable CORS in your Config Server by adding the following configuration to your application.properties
:
spring.web.cors.allowed-origins=*
Be cautious with this setting in production. Limiting origins can prevent unauthorized access.
Why: CORS policies exist to maintain security, but they need to be configured properly to allow expected interactions.
Logging and Monitoring
Effective logging and monitoring of your Spring Cloud Config Server can help quickly identify issues. Consider enabling debug logging in your application.properties
:
logging.level.org.springframework.cloud=DEBUG
This will provide detailed output and can help trace the specific failures occurring during the property retrieval process.
Additional Resources
For deeper dives into each of these troubleshooting techniques, consider reviewing the official Spring Cloud Config Documentation and community discussions on Stack Overflow.
Bringing It All Together
Spring Cloud Config Server is a crucial tool for managing configurations in microservices. Understanding common issues and their resolutions enhances your ability to maintain a smoother development process. By focusing on proper setup, network reliability, and logging, you can minimize downtime and improve the performance of your distributed systems.
With these solutions, you should be well-equipped to troubleshoot and resolve common issues effectively. Remember, efficient configuration management leads to a more robust microservices architecture. Happy coding!
Checkout our other articles