Mastering Java EE 7 Batch Jobs: Common Scheduling Issues
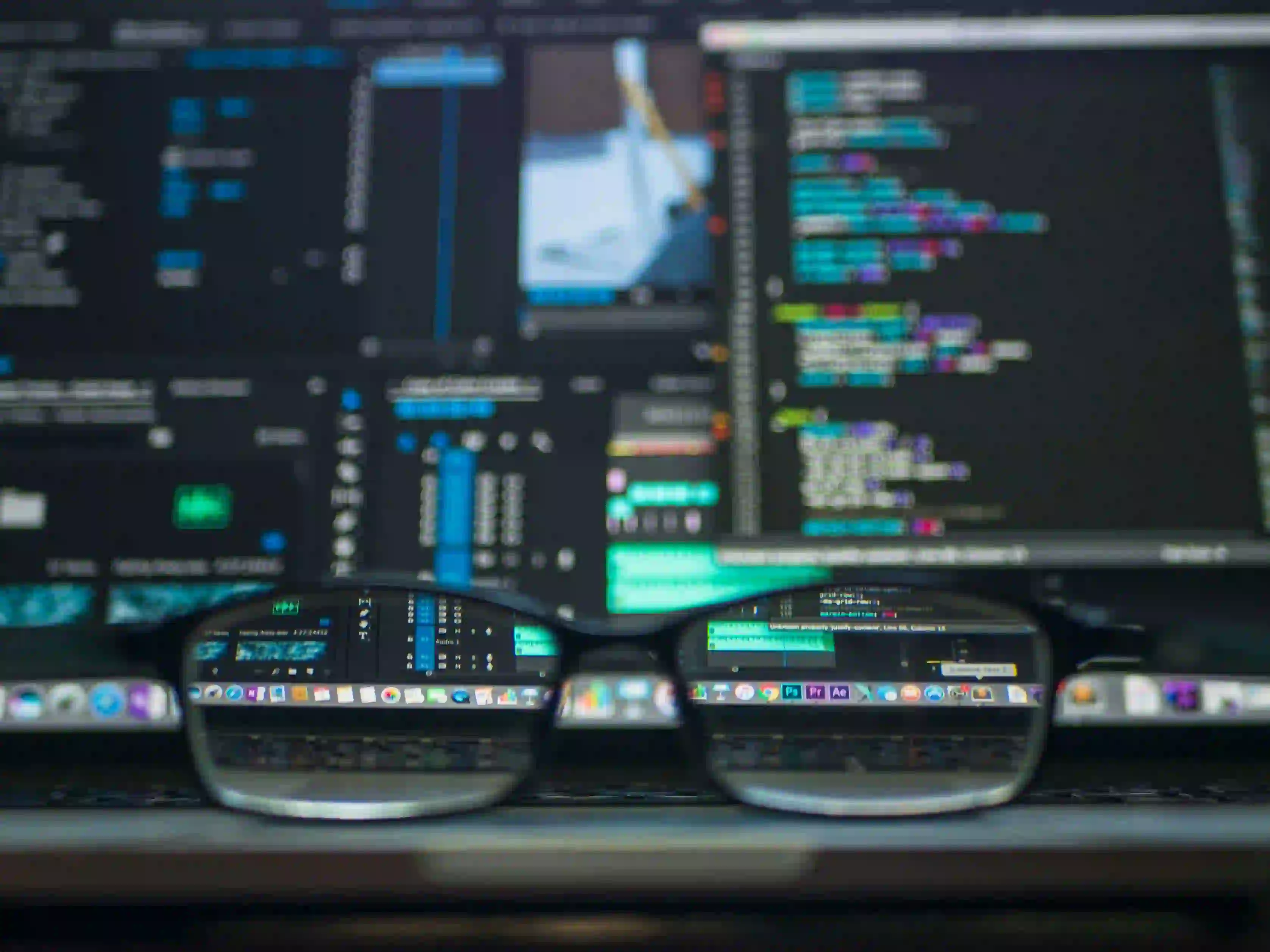
Mastering Java EE 7 Batch Jobs: Common Scheduling Issues
Java EE 7 introduced a robust API for batch processing, addressing the growing need for developers to execute long-running tasks efficiently. However, even with the best tools at our disposal, scheduling batch jobs brings its own set of challenges. This blog post explores common scheduling issues encountered when working with Java EE 7 batch jobs and offers practical solutions to overcome them.
What is Java EE Batch Processing?
Java EE Batch Processing is part of the Java EE platform designed to facilitate the execution of batch jobs. Batch jobs often involve processing large volumes of data, such as reading from a database, applying transformations, and writing the results back to a storage. With the javax.batch
package, developers can leverage:
- Chunk Processing: Break tasks into smaller chunks.
- Restartability: Resume jobs from the last checkpoint after a failure.
- Partitioning: Divide workloads across multiple JVMs.
The API enables efficient job management and error handling, making it an essential tool for enterprise applications.
Understanding Common Scheduling Issues
Despite the powerful features of Java EE Batch Processing, developers still encounter several common scheduling problems. Let's dive into these issues and explore how to resolve them.
1. Job Overlapping
One of the most frequent problems is job overlapping, where multiple instances of the same job run concurrently. This can lead to resource contention and unpredictable results.
Solution: Implement a job locking strategy.
Here is an example of how you can use a database table to manage job locks:
public void acquireLock(String jobName) throws SQLException {
String sql = "SELECT job_name FROM job_locks WHERE job_name = ? FOR UPDATE";
try (Connection connection = dataSource.getConnection();
PreparedStatement statement = connection.prepareStatement(sql)) {
statement.setString(1, jobName);
ResultSet resultSet = statement.executeQuery();
if (resultSet.next()) {
throw new IllegalStateException("Job is already running: " + jobName);
} else {
// Lock this job
String insertSql = "INSERT INTO job_locks (job_name) VALUES (?)";
try (PreparedStatement insertStatement = connection.prepareStatement(insertSql)) {
insertStatement.setString(1, jobName);
insertStatement.executeUpdate();
}
}
}
}
Why this works: The database transaction ensures that only one job instance can acquire the lock. If another instance tries to access the locked job, it will throw an exception.
2. Resource Exhaustion
Batch jobs often consume significant CPU and memory resources. When multiple jobs are scheduled to run simultaneously, resource exhaustion may occur, leading to failures or crashes.
Solution: Use a well-planned job scheduling strategy.
Consider using a scheduling library, like Quartz, which allows more fine-grained control over job execution:
public class MyJob implements Job {
public void execute(JobExecutionContext context) throws JobExecutionException {
// Job logic here
}
}
SchedulerFactory schedulerFactory = new StdSchedulerFactory();
Scheduler scheduler = schedulerFactory.getScheduler();
JobDetail jobDetail = JobBuilder.newJob(MyJob.class)
.withIdentity("myJob", "group1")
.build();
Trigger trigger = TriggerBuilder.newTrigger()
.withIdentity("myTrigger", "group1")
.withSchedule(SimpleScheduleBuilder.repeatSecondlyForTotalCount(10, 60))
.build();
scheduler.scheduleJob(jobDetail, trigger);
scheduler.start();
Why this works: Using Quartz helps distribute job execution efficiently and allows jobs to be scheduled well into the future without overlapping, thus minimizing the impact on system resources.
3. Error Handling and Retries
Batch jobs may encounter exceptions due to various reasons, such as data corruption or temporary unavailability of resources. A well-defined error handling strategy is crucial.
Solution: Implement a retry mechanism with exponential backoff.
This example demonstrates how to catch exceptions and retry the job execution:
public void processBatchJob() {
int retryCount = 0;
while (retryCount < MAX_RETRIES) {
try {
// Your batch job logic here
break; // Exit loop if successful
} catch (Exception e) {
retryCount++;
long backoffTime = Math.pow(2, retryCount) * 1000; // Exponential backoff
Thread.sleep(backoffTime);
}
}
if (retryCount == MAX_RETRIES) {
// Handle failure after max retries
logFailure();
}
}
Why this works: This implementation leverages exponential backoff to reduce the load on the system during retry attempts. It increases the wait time successively, giving resource situation a chance to stabilize.
4. Configuration Management
Managing batch job configurations can become cumbersome, especially in large applications with multiple jobs. Misconfigured parameters can lead to unexpected behavior.
Solution: Centralize configuration management.
Consider using external configuration files or an environment variable approach to maintain job parameters. Here's how you might read from a properties file:
Properties properties = new Properties();
try (InputStream input = new FileInputStream("config.properties")) {
properties.load(input);
String myJobParam = properties.getProperty("myJob.param");
} catch (IOException ex) {
ex.printStackTrace();
}
Why this works: Centralizing your configuration enables easy management and adjustments without altering the code. Furthermore, it promotes better maintainability and scalability for your batch jobs.
Closing the Chapter
Batch processing in Java EE 7 is an essential feature that, when effectively utilized, can significantly enhance your application's performance. However, understanding and addressing common scheduling issues is equally important. By implementing strategies to manage job overlap, optimize resource consumption, handle errors gracefully, and streamline configuration processes, developers can ensure that their batch jobs run smoothly.
When implementing batch jobs, remember that continuous monitoring and refinement are key. As needs change and systems evolve, refining your approach will yield even better results.
For additional resources on these topics, you may want to explore the Java EE Batch Specification or read about Quartz Scheduler, which provides extensive capabilities for scheduling jobs.
Feel free to apply these insights to your batch processing logic, and embrace the power of Java EE for managing complex tasks with ease!