Mastering Spring AOP: Troubleshooting Aspect Tests
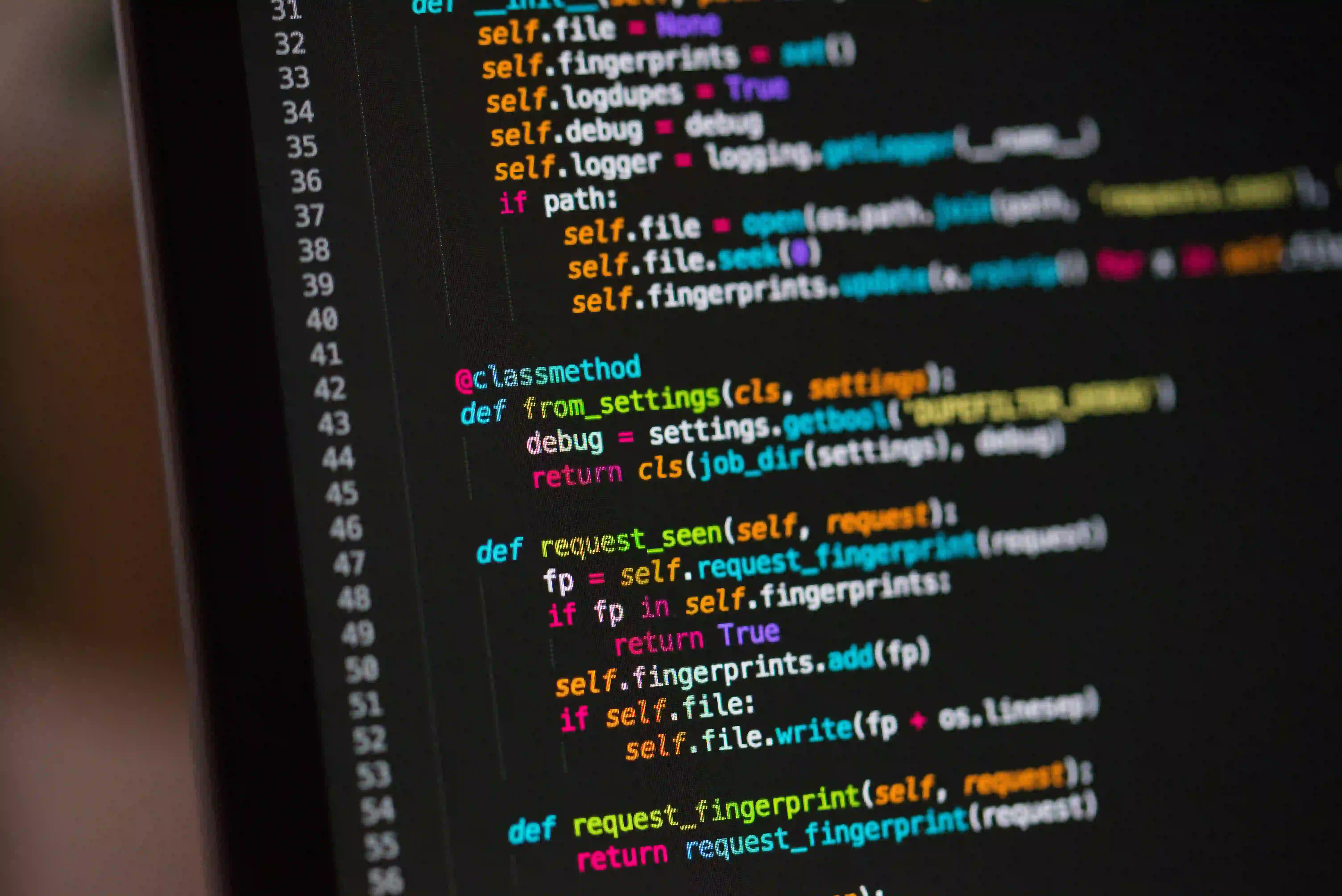
Mastering Spring AOP: Troubleshooting Aspect Tests
A Quick Look to Spring AOP
Spring AOP (Aspect-Oriented Programming) is an essential part of the Spring framework that allows developers to implement cross-cutting concerns like logging, security, or transaction management in a modular way. With AOP, you can keep your business logic separate from secondary actions, which makes your application cleaner and easier to maintain.
In this article, we will explore how to troubleshoot aspect tests in Spring AOP effectively, ensuring that your application runs smoothly and your aspects work as intended.
Understanding Aspects
Before diving into troubleshooting, let’s briefly summarize what aspects are in the context of Spring AOP.
An aspect is a module that contains pointcuts and advice.
- Pointcut: Expressions that define where the aspect should apply.
- Advice: Code that gets executed at a specific join point.
Example Aspect
Here's a basic example of a logging aspect:
@Aspect
@Component
public class LoggingAspect {
@Before("execution(* com.example.service.*.*(..))")
public void logBefore(JoinPoint joinPoint) {
System.out.println("Executing method: " + joinPoint.getSignature().getName());
}
}
In this example, the logBefore
method is executed before any method in the service
package, printing the name of the method being executed. The @Aspect
annotation marks this class as an aspect, while the @Component
makes it a Spring-managed bean.
Why Use AOP?
- Separation of Concerns: Improve code structure and maintainability.
- Reusability: Aspects can be reused across different parts of your application.
- Easy Testing: Testing aspects separately can help identify issues quickly.
Troubleshooting Aspect Tests in Spring AOP
Oops! You’ve written your aspect and tests, but they’re not working as expected. Let’s troubleshoot with some common strategies.
1. Ensure AspectJ Configuration
One of the first places to check is whether you have correctly configured AspectJ in your Spring application. Without proper configuration, your aspects may not be applied.
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:aop="http://www.springframework.org/schema/aop"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop.xsd">
<aop:aspectj-autoproxy />
</beans>
Make sure the <aop:aspectj-autoproxy />
element is included in your Spring context configuration. This ensures that Spring processes the @Aspect
annotations properly.
2. Check Pointcut Expressions
Your pointcut expressions govern when and where your aspects kick in. If your aspects aren't being triggered, it's likely that your pointcut is misconfigured.
Example of a Potentially Incorrect Pointcut
@Before("execution(* com.example.service.*.*(..))")
This expression will only trigger for methods in classes inside the com.example.service
package. If you forget to include certain packages or methods, your aspect won't activate.
3. Use Mockito for Unit Testing
When testing aspects, consider using Mockito to mock dependencies. This practice allows you to isolate your tests and ensure it’s indeed the aspect that is being tested and not other components.
Example of Mocking with Mockito
Here's how you might set up a simple test with Mockito:
@RunWith(MockitoJUnitRunner.class)
public class LoggingAspectTest {
@InjectMocks
private LoggingAspect loggingAspect;
@Mock
private SomeService someService;
@Test
public void testLogBefore() {
// Act
loggingAspect.logBefore(Mockito.mock(JoinPoint.class));
// Assert (Check appropriate method call)
}
}
4. Validate Join Points
Sometimes the issue lies in how you've defined your join points. To ensure that your advice is executing correctly, you can log within your advice method to trace whether it was called.
Adding Logs to Join Points
@Before("execution(* com.example.service.*.*(..))")
public void logBefore(JoinPoint joinPoint) {
System.out.println("Before method: " + joinPoint.getSignature().toShortString());
}
By adding a log statement, you can track whether the join point is matching as intended.
5. Testing with Spring Context
To run your aspect tests within the Spring context, you may want to use the @SpringBootTest
annotation. This can ensure that your beans are appropriately loaded.
Example of Context-Based Test
@SpringBootTest
public class AspectIntegrationTest {
@Autowired
private SomeService someService;
@Test
public void testAspect() {
someService.someMethod(); // Your method under test
}
}
Using Spring’s test framework ensures that the full context is loaded with all beans, including your aspects.
6. Profile Activation
If you have multiple profiles and you are only enabling certain aspects under specific conditions, make sure that the correct profile is active during the test execution.
You can specify the profile you want to activate like this:
@ActiveProfiles("test")
@SpringBootTest
public class YourServiceTest {
}
7. Check for Application Context Issues
Sometimes the problem isn't in the test itself but rather how the application context is set up. Ensure there are no missing dependencies or configurations causing your aspect not to load.
Run your application with debug logs to check if the aspect is being instantiated correctly:
-Ddebug
8. Use @EnableAspectJAutoProxy
When using Java configuration instead of XML, don't forget to include @EnableAspectJAutoProxy
in your configuration class.
@Configuration
@EnableAspectJAutoProxy
public class AppConfig {
// Your bean definitions
}
Closing the Chapter
Mastering Spring AOP means more than just writing aspects; it’s about ensuring they work effectively in conjunction with your application’s business logic. Troubleshooting can initially seem daunting, but with the strategies outlined above, you can identify and resolve issues more efficiently. Remember to validate your configurations, pointcuts, and ensure the appropriate Spring context is being used.
For further reading on Spring AOP, consider checking out the Spring Documentation for a deeper understanding.
By mastering Spring AOP troubleshooting techniques, you can build more robust applications, leading to a better overall experience for your end-users. Happy coding!