Maximizing Performance: Common Pitfalls of Fork/Join in Java 7
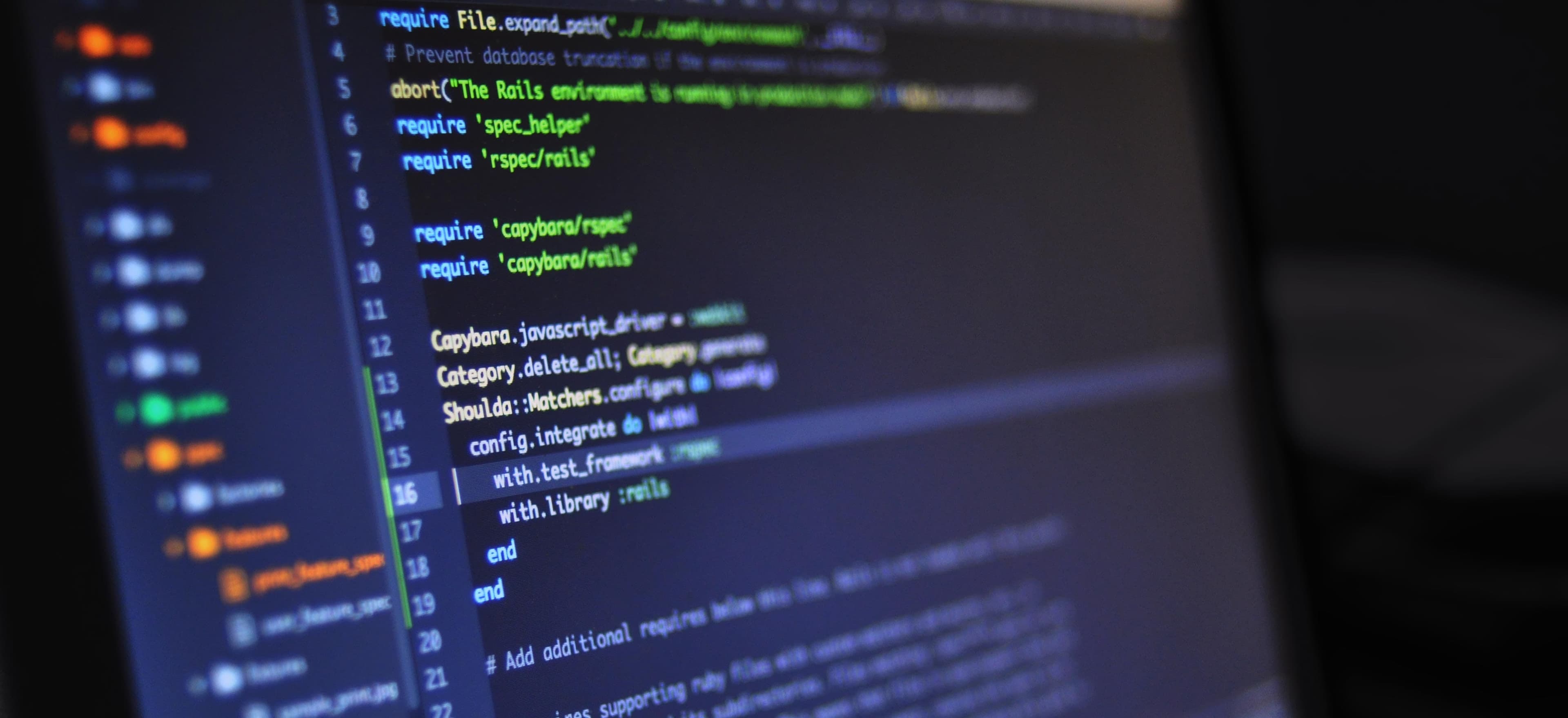
- Published on
Maximizing Performance: Common Pitfalls of Fork/Join in Java 7
Java 7 introduced the Fork/Join framework, an innovative way to harness the power of multicore processors for parallel processing. This framework simplifies the implementation of parallel algorithms and provides a structured framework for effectively dividing tasks. However, as powerful as it can be, there are some common pitfalls developers may encounter when using Fork/Join, which could hinder performance. In this post, we'll explore those pitfalls and discuss strategies for overcoming them to maximize performance.
Understanding the Fork/Join Framework
Before diving into the pitfalls, let’s quickly review how the Fork/Join framework functions. The framework is built around two key components: Forking tasks (breaking down a larger task into smaller, manageable subtasks) and Joining them (aggregating results from those subtasks back into the final result).
The best way to visualize this is by considering a recursive algorithm. This approach efficiently uses available resources by distributing workload across multiple threads.
Code Snippet: A Simple Fork/Join Example
Here is a simple example demonstrating a Fork/Join task that calculates the sum of an array:
import java.util.concurrent.RecursiveTask;
import java.util.concurrent.ForkJoinPool;
public class SumTask extends RecursiveTask<Long> {
private static final int THRESHOLD = 10; // Threshold for forking
private final long[] numbers;
private final int start;
private final int end;
public SumTask(long[] numbers, int start, int end) {
this.numbers = numbers;
this.start = start;
this.end = end;
}
@Override
protected Long compute() {
if (end - start <= THRESHOLD) {
long sum = 0;
for (int i = start; i < end; i++) {
sum += numbers[i];
}
return sum;
} else {
int mid = (start + end) / 2;
SumTask leftTask = new SumTask(numbers, start, mid);
SumTask rightTask = new SumTask(numbers, mid, end);
leftTask.fork(); // Start the left task
long rightResult = rightTask.compute(); // Compute the right task
long leftResult = leftTask.join(); // Join the left task
return leftResult + rightResult; // Return the combined result
}
}
public static void main(String[] args) {
long[] numbers = new long[100];
for (int i = 0; i < numbers.length; i++) {
numbers[i] = i + 1; // Filling the array with numbers 1 to 100
}
ForkJoinPool pool = new ForkJoinPool();
SumTask task = new SumTask(numbers, 0, numbers.length);
long result = pool.invoke(task);
System.out.println("Total Sum: " + result);
}
}
This basic implementation calculates the sum of an array of numbers using the Fork/Join framework. Notice how it forks and joins tasks based on the defined threshold.
Common Pitfalls in Fork/Join
Even with its benefits, developers often make mistakes when using the Fork/Join framework. Here are some pitfalls to avoid:
1. Improper Task Granularity
Issue: Setting the wrong threshold can either lead to excessive task creation or insufficient parallelism. If you set a threshold that is too high, you will lose the benefits of parallelism. Conversely, if it’s too low, the overhead of managing many small tasks can outweigh the benefits of parallel execution.
Solution: Experiment to find the optimal threshold for your specific application. It is often a good idea to test the performance with various thresholds and choose the one that yields the best results.
2. Excessive Spawning of Tasks
Issue: Creating too many tasks can overwhelm the system’s resources. Each task requires memory overhead, which may lead to increased garbage collection (GC) and degraded performance.
Solution: Be cautious about the number of tasks spawned. The recursive splitting logic should balance the amount of work and the depth of the task hierarchy.
3. Blocking Operations Inside Tasks
Issue: Blocking operations (like I/O operations or synchronized blocks) within Fork/Join tasks can severely degrade performance. Since Fork/Join is designed for parallelism, blocking calls can halt task execution and reduce efficiency.
Solution: Avoid blocking operations in Fork/Join tasks. If blocking is unavoidable, consider using other concurrency mechanisms like Executors and CompletableFutures in tandem with Fork/Join for optimal performance.
4. Improper Use of Join Method
Issue: The join()
method can be misused if you try to join tasks that are long-running or that have been forked without proper consideration of the task lifecycle.
Solution: Use join()
judiciously. Ensure that you only join tasks that have completed or that you know are safe to join. Avoid joining too many tasks in a single thread to prevent blocking.
5. Non-Thread-Safe Operations
Issue: Using shared mutable state across tasks may introduce thread-safety issues and race conditions.
Solution: Design your tasks to be stateless or use thread-safe data structures (like ConcurrentHashMap
or AtomicInteger
) when sharing state across tasks. This reduces the risks of concurrent modification and potential exceptions.
6. Not Monitoring Performance
Issue: Failing to monitor the performance of your Fork/Join tasks may lead to unoptimized and inefficient code.
Solution: Use profiling tools (like Java Mission Control or Java VisualVM) to monitor your application in real-time, allowing you to visualize the performance bottlenecks and refine your tasks accordingly.
Testing and Iterating
To maximize performance, continuously test various strategies. Benchmark against non-Fork/Join implementations to understand how much performance gain you achieve.
Leverage tools like JMH (Java Microbenchmark Harness) to create accurate benchmarks:
public class ForkJoinBenchmark {
@Benchmark
public void testForkJoin() {
long[] numbers = ... // Your setup code
ForkJoinPool pool = new ForkJoinPool();
SumTask task = new SumTask(numbers, 0, numbers.length);
pool.invoke(task);
}
// Additional benchmarks can be added here
}
Iterate through your design, assess performance, and adjust the thresholds, task sizes, and method logistics based on your findings.
The Closing Argument
The Fork/Join framework in Java 7 is a powerful tool to unlock the parallel processing capabilities of modern multi-core architectures. However, to truly maximize its potential, developers must be aware of common pitfalls that can destroy performance. By observing proper task granularity, avoiding excessive tasks, maintaining thread safety, and monitoring performance, you can harness the full power of Fork/Join.
For further reading, consider exploring the Fork/Join Framework documentation and additional insights on performance testing in Java.
By understanding and addressing these common issues, you can successfully implement Fork/Join in your applications, resulting in faster, more efficient code capable of handling complex parallel tasks with ease.