Common Pitfalls When Using Streams in Java 8 with JOOλ
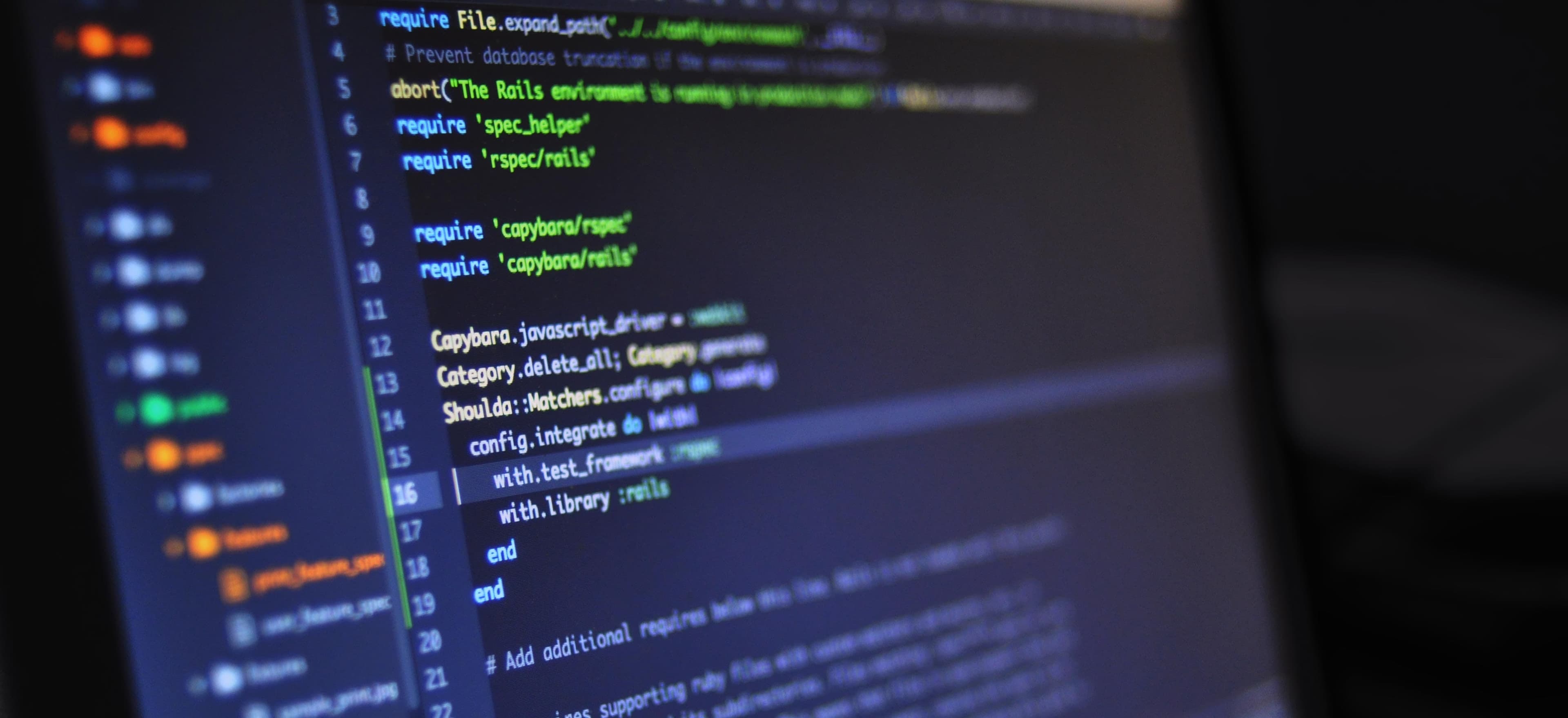
- Published on
Common Pitfalls When Using Streams in Java 8 with JOOλ
Java 8 introduced a powerful stream API that revolutionized the way developers handle collections of data. Alongside this, libraries such as JOOλ (Java Object Oriented Logic) have enhanced functionality, allowing for more expressive data processing. While Java Streams and JOOλ bring significant advantages, developers can easily fall into common pitfalls.
In this blog post, we'll explore some of these pitfalls and provide you with code snippets to illustrate solutions. Let’s dive in!
Understanding Java Streams
Java Streams provide a way to process sequences of elements using a functional approach. Streams can represent both collections and arrays, offering operations such as filtering, mapping, and reducing.
Example: Basic Stream Operations
import java.util.List;
import java.util.Arrays;
public class StreamExample {
public static void main(String[] args) {
List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "David");
// Filtering and converting to uppercase
List<String> filteredNames = names.stream()
.filter(name -> name.length() > 3)
.map(String::toUpperCase)
.toList();
System.out.println(filteredNames); // Outputs: [ALICE, CHARLIE]
}
}
Commentary
In the above code, we filter names with more than three characters and convert them to uppercase. This example shows how concise and expressive stream processing can be.
Common Pitfalls
1. Not Understanding Stream Pipeline Termination
One of the fundamental principles of using streams is that they are designed to be read and processed in a pipeline fashion, where operations such as filter
, map
, and reduce
are chained together. However, the pipeline must be terminated with a terminal operation (e.g., collect
, forEach
, reduce
).
Failing to do so results in your stream processing logic never executing.
import java.util.List;
import java.util.Arrays;
public class StreamTerminationExample {
public static void main(String[] args) {
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
// This stream will not execute as there is no terminal operation
names.stream()
.filter(name -> name.startsWith("A")); // Nothing happens here
}
}
Commentary
In this case, the filter operation is applied but not executed. Remember to include a terminal operation to trigger the processing.
2. Confusion Between Stateful and Stateless Operations
Understanding the difference between stateful and stateless operations is crucial. Stateless operations (like filter
or map
) do not depend on the state of the entire stream. Stateful operations (like distinct
, sorted
) require knowledge of all elements before producing results. This can lead to performance issues if misused, particularly in parallel processing.
Example: Stateful vs Stateless
import java.util.Arrays;
import java.util.List;
public class StatefulExample {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(3, 1, 4, 4, 2);
// Stateless operation
long count = numbers.stream()
.filter(n -> n > 2)
.count();
// Stateful operation
long distinctCount = numbers.stream()
.distinct()
.count();
System.out.println("Count: " + count); // Outputs: Count: 3
System.out.println("Distinct Count: " + distinctCount); // Outputs: Distinct Count: 3
}
}
Commentary
In this example, the filter
method is stateless and directly counts numbers greater than two. Conversely, distinct
requires the entire stream to evaluate distinct elements, which can slow performance.
3. Modifying Collections While Streaming
Another common pitfall is modifying a collection while processing it with streams. This can lead to unexpected behavior and ConcurrentModificationException
.
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
public class ModificationExample {
public static void main(String[] args) {
List<String> names = new ArrayList<>(Arrays.asList("Alice", "Bob", "Charlie"));
// Avoid modifying the list directly while streaming
names.stream()
.filter(name -> {
if (name.startsWith("A")) {
names.remove(name); // This will throw an exception
}
return true;
}).count(); // This will result in ConcurrentModificationException
}
}
Commentary
Attempting to modify the names
list during the streaming process will throw an exception. Instead, it’s advisable to create a new list from your stream.
4. Overusing Parallel Streams
While parallel streams can significantly increase performance, using them indiscriminately can reduce performance and lead to unexpected results. If you're working with small collections or stateless operations, the overhead may outweigh the benefits.
Example: Parallel Streams
import java.util.Arrays;
import java.util.List;
public class ParallelStreamExample {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
// Using parallel stream - may not be beneficial here
int sum = numbers.parallelStream()
.map(n -> n * n) // Squaring numbers
.reduce(0, Integer::sum);
System.out.println("Sum of squares: " + sum); // Outputs: Sum of squares: 55
}
}
Commentary
In this example, using a parallel stream on a small list may not lead to performance gains. Always evaluate the context before deciding to go parallel.
Utilizing JOOλ with Streams
JOOλ is an extension of the Java Stream API that provides additional functionality for working with data in a more expressive and functional way. It is particularly well-suited for complex data processing tasks. Below are some common JOOλ functionalities you may incorporate into your Java Stream operations.
Example: JOOλ with Stream
import org.jooq.lambda.Seq;
import java.util.List;
import java.util.Arrays;
public class JOOλExample {
public static void main(String[] args) {
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
// Using JOOλ to perform functional operations
List<String> result = Seq.seq(names)
.filter(name -> name.length() > 3)
.map(String::toUpperCase)
.toList();
System.out.println(result); // Outputs: [ALICE, CHARLIE]
}
}
Commentary
The above code demonstrates using JOOλ's Seq
to process a list of names effectively. It provides additional utilities that enhance productivity and reduce boilerplate code.
Final Considerations
Streams in Java 8, together with libraries like JOOλ, can simplify coding patterns and enhance performance. However, it is crucial to avoid the common pitfalls discussed above.
- Ensure you include terminal operations to execute the stream pipeline.
- Understand the implications of stateful vs. stateless operations.
- Avoid modifying collections during a stream process.
- Be judicious in using parallel streams.
By adhering to these guidelines, you can leverage the full power of Java Streams and JOOλ while minimizing headaches down the line.
For an in-depth exploration of streams, refer to the official Java Documentation or check out JOOλ’s documentation.
Happy coding!