Common Issues When Using JAXB with OpenJDK 11
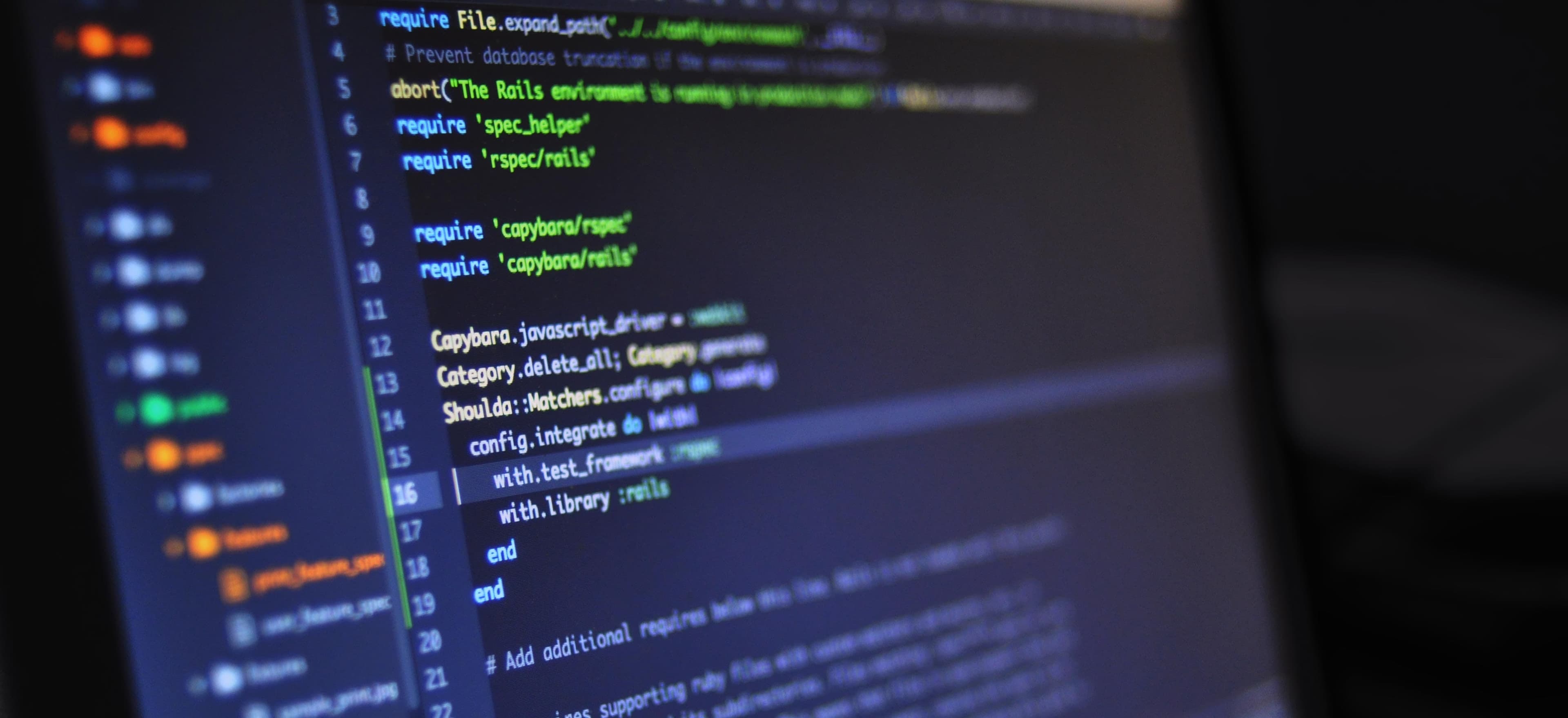
- Published on
Common Issues When Using JAXB with OpenJDK 11
Java Architecture for XML Binding (JAXB) has been a crucial tool for developers needing to convert Java objects to XML and vice versa. However, with the introduction of OpenJDK 11, many developers have encountered challenges using JAXB, primarily because it was removed from the Java SE platform starting from Java 11. This blog post explores these common issues, their implications, and how to effectively resolve them while keeping your application up and running.
Understanding JAXB and Its Importance
JAXB simplifies XML handling in Java. It allows for:
- Marshalling: The process of converting a Java object into an XML representation.
- Unmarshalling: The process of converting an XML representation back into a Java object.
These capabilities are essential when dealing with web services, data interchange between applications, or persisting data.
The Shift in OpenJDK 11
Starting from Java 11, JAXB is no longer part of the JDK. Instead, it has been moved to separate modules, which means if you were accustomed to relying on JAXB as an integral part of your Java development, you will need to make adjustments. Here are some common issues encountered when using JAXB with OpenJDK 11.
Issue 1: Missing JAXB API
One of the primary issues developers face is trying to compile or run code that imports JAXB classes without the necessary dependencies. This situation leads to ClassNotFoundException
or NoClassDefFoundError
.
Resolution: Add dependencies manually. You can include JAXB in your project using Maven or Gradle.
For Maven
Add the following dependencies into your pom.xml
:
<dependencies>
<dependency>
<groupId>org.glassfish.jaxb</groupId>
<artifactId>jaxb-runtime</artifactId>
<version>2.3.3</version>
</dependency>
<dependency>
<groupId>javax.xml.bind</groupId>
<artifactId>jaxb-api</artifactId>
<version>2.3.1</version>
</dependency>
</dependencies>
Why: These dependencies inform Maven to include the JAXB implementation in your classpath.
For Gradle
Use the following in your build.gradle
:
dependencies {
implementation 'org.glassfish.jaxb:jaxb-runtime:2.3.3'
implementation 'javax.xml.bind:jaxb-api:2.3.1'
}
Why: Similar to Maven, this ensures that your Gradle setup includes the necessary libraries for JAXB functionalities.
Issue 2: JAXBContext Initialization Errors
After managing dependencies, you might still experience issues initializing JAXB with JAXBContext
. Errors like javax.xml.bind.JAXBException
can present themselves. This is often due to incorrect package names when creating the context.
Example Code:
import javax.xml.bind.JAXBContext;
import javax.xml.bind.JAXBException;
public class XmlProcessing {
public static void main(String[] args) {
try {
JAXBContext context = JAXBContext.newInstance(MyClass.class);
} catch (JAXBException e) {
e.printStackTrace();
// Take corrective actions here
}
}
}
Why: Ensure that MyClass
is annotated with JAXB annotations like @XmlRootElement. If it is not recognized as a valid JAXB class, it won't initialize properly.
Issue 3: JAXB Annotation Support
You may find that certain JAXB annotations like @XmlAccessType
or @XmlType
are not recognized or cause compilation issues. This can occur if the appropriate JAXB library is not included in your project correctly.
Example Code:
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
@XmlRootElement
public class Person {
private String name;
private int age;
@XmlElement
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
@XmlElement
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
}
Why: If @XmlElement
and others are not recognized, it indicates that the JAXB API is missing from your classpath.
Issue 4: XML Binding Configuration
When you marshal or unmarshal complex types, you may encounter issues with namespace management or XML schema binding. JAXB expects a specific structure defined by its annotations.
Example Code:
import javax.xml.bind.JAXBContext;
import javax.xml.bind.JAXBException;
import javax.xml.bind.Marshaller;
public class MarshalExample {
public static void main(String[] args) {
try {
JAXBContext jaxbContext = JAXBContext.newInstance(Person.class);
Marshaller marshaller = jaxbContext.createMarshaller();
marshaller.setProperty(Marshaller.JAXB_FORMATTED_OUTPUT, true);
Person person = new Person();
person.setName("John Doe");
person.setAge(30);
marshaller.marshal(person, System.out);
} catch (JAXBException e) {
e.printStackTrace();
}
}
}
Why: Properly configuring the Marshaller
ensures that XML output adheres to the desired format and structure. If the fields aren’t displaying correctly, check your annotation definitions.
Issue 5: Classpath-related Issues in IDEs
Integrated Development Environments, such as Eclipse or IntelliJ, sometimes fail to recognize the newly added JAXB dependencies, leading to unresolved references.
Resolution: Refresh project dependencies.
- In Maven: Right-click the project > Maven > Update Project.
- In Gradle: Use the Refresh button on the Gradle panel.
Why: This refresh ensures the IDE’s project structure recognizes the added libraries and their dependencies.
Bringing It All Together
Transitioning to OpenJDK 11 brings significant improvements and changes, especially with JAXB being removed from the JDK. By being aware of these common issues and their solutions, you will ensure a smoother development experience. Remember that adopting external libraries via dependency management systems like Maven or Gradle is the key to managing potential hurdles.
For more detailed information on JAXB, check out the official documentation. By keeping your codebase updated and using JAXB properly, you can continue to leverage XML processing in your Java applications effectively.
Stay tuned for more discussions on Java-related topics, and happy coding!