Navigating Jep-277: Tackling Enhanced Deprecation Challenges
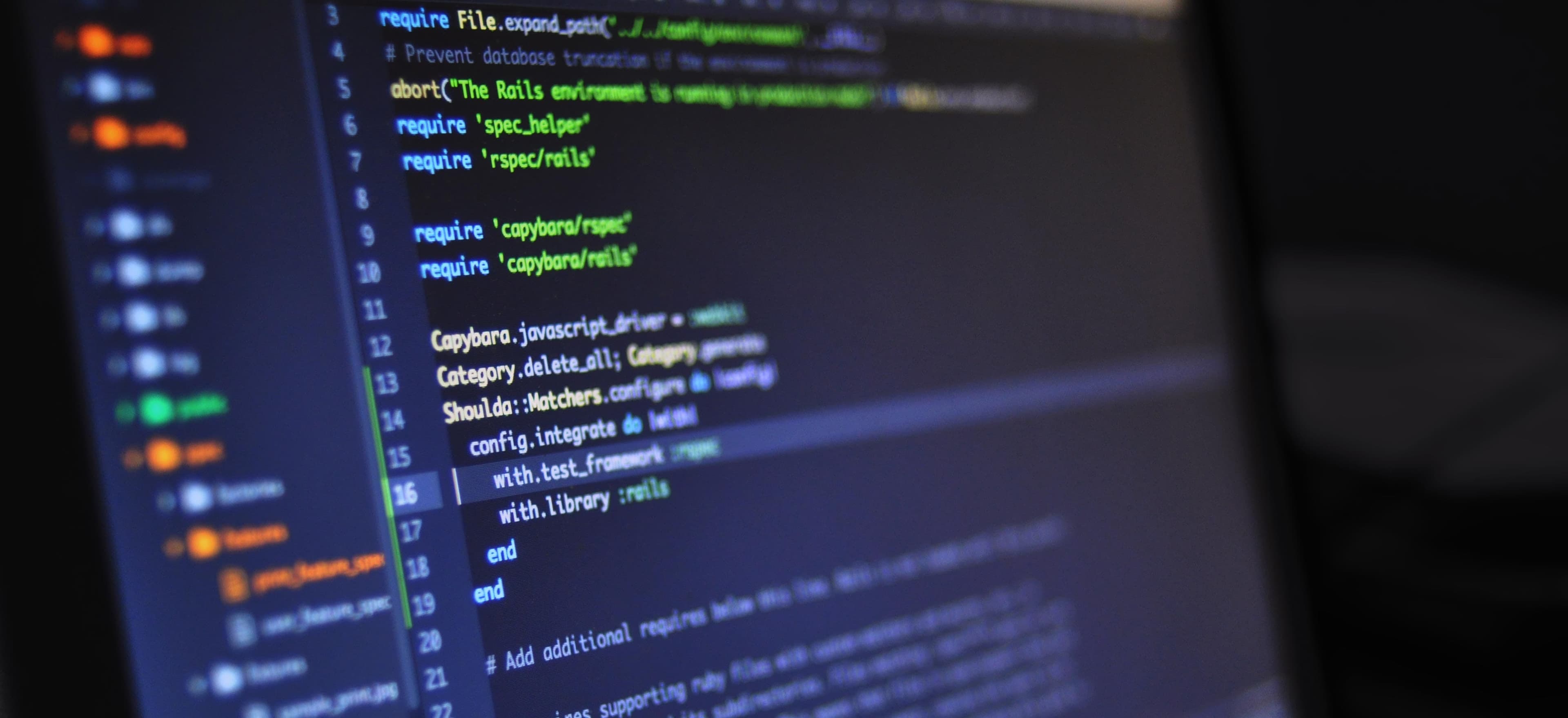
- Published on
Navigating JEP-277: Tackling Enhanced Deprecation Challenges
Java developers have long valued backward compatibility, but as the language evolves, so does the need to address and manage deprecated features. Enter JEP-277, which aims to make the deprecation process clearer and more manageable. In this blog post, we will explore the implications of JEP-277, tackle the challenges of enhanced deprecation, and provide practical insights to help developers adapt effectively.
Understanding JEP-277
Java Enhancement Proposals (JEP) play a crucial role in the evolution of Java. JEP-277, proposed in the Java Development Kit (JDK) 9 cycle, sets out to improve the deprecation process by introducing a clear strategy for dealing with deprecated API elements. It strives to differentiate between the levels of deprecation and the actions developers should take.
The Objectives of JEP-277
-
Clear Guidelines: JEP-277 aims to provide guidelines not just on what to deprecate, but also on how to use deprecation effectively.
-
Support for Migration: It aims to help users transition away from deprecated features in a clearer and more structured manner.
-
Consistency: Developers can expect a more consistent deprecation experience across the JDK and other libraries.
The Challenges of Managing Deprecation
While JEP-277 brings clarity, it also introduces challenges in managing deprecations. Here are some common challenges you might face:
1. Legacy Code
Legacy code bases often rely heavily on deprecated features. Developers need to decide whether to invest time in refactoring or simply maintain these technologies.
2. Awareness and Communication
Developers might not always be aware of deprecated features or the implications of usage warnings. Effective communication is vital to ensure that teams stay updated regarding the deprecation roadmap.
3. Gradual Migration
Deprecation is a gradual process, and having to migrate code in small increments can be cumbersome. Developers may face complex scenarios when transitioning between versions and need to maintain functionality throughout.
Enhanced Deprecation: How to Tackle the Challenges
Now that we understand the challenges, let’s explore the strategies for tackling them effectively.
Communicate the Deprecation Roadmap
Being informed opens the door to better decision-making. Regular updates on the deprecation roadmap within your development teams can help avoid surprises.
Here’s how you can implement a communication plan:
- Documentation: Keep your project documentation updated with the deprecation notices and timelines.
- Regular Check-ins: Have regular meetings to discuss deprecated features and plans for migration.
- Use Linting Tools: Tools such as Checkstyle or PMD can help monitor deprecated usages in your code.
Refactor Gradually
Refactoring slowly rather than all at once can ease the migration process. Refactoring is often seen as a mega-task, which can discourage action. Still, small incremental changes can carry the same weight.
For instance, suppose you have a method that uses a deprecated API. Instead of overhauling your codebase, consider isolating its usage:
@Deprecated
public void oldMethod() {
// deprecated API usage
System.out.println("This method is deprecated.");
}
You can wrap it in a conditional check as a temporary solution:
public void newMethod() {
if (shouldUseOldMethod()) {
oldMethod();
} else {
// Call the new method
System.out.println("Using the new method.");
}
}
private boolean shouldUseOldMethod() {
// Implement your logic for when to use the old method
return true; // or false based on some conditions
}
By gradually migrating to newer APIs, you reduce the risk of breaking changes.
Addressing Legacy Code
When dealing with legacy systems, consider the following strategies:
- Identify Areas of Concern: Start by scanning your existing codebase for deprecated features using automated tools.
- Set Priorities: Focus on the most critical areas that are frequently used.
- Prepare a Transition Plan: Develop a roadmap for replacing deprecated features that outlines specific deadlines and goals.
Documentation for Transition
Effective documentation is crucial. It should detail the reasons for deprecation and the consequences of not upgrading. It’s also beneficial to include examples of how to replace deprecated practices with their newer counterparts.
For example, let’s transition from an outdated method:
@Deprecated
public void legacyPrint() {
System.out.println("This is a legacy print method.");
}
To a more modern approach:
public void modernPrint(String message) {
System.out.println(message);
}
Promote Team Collaboration
Involve your teams in the deprecation challenges. Collaboration can lead to innovative ideas for migration. Here are some ways to strengthen teamwork:
- Pair Programming: This hands-on practice can be instrumental in tackling complex refactoring.
- Code Reviews: Regular code reviews can help identify and address deprecations before they become problematic.
Utilize Modern Tools
Today, many tools can aid in managing deprecation issues effectively:
-
Integrated Development Environments (IDEs): IDEs like IntelliJ and Eclipse often highlight deprecated methods as you write.
-
Build Tools: Use Maven or Gradle plugins to enforce deprecation warnings as build failures.
-
Static Analysis Tools: These can scan through code and identify deprecated classes, methods, and APIs.
Here’s an example using a Maven dependency to check for deprecated APIs:
<dependency>
<groupId>org.codehaus.mojo</groupId>
<artifactId>versions-maven-plugin</artifactId>
<version>2.8.1</version>
</dependency>
Once configured, you can run:
mvn versions:display-dependency-updates
Doing so will allow you to see the status of your dependencies, including if any are marked for deprecation.
Summary
Navigating the challenges presented by JEP-277 requires a proactive approach. Clear communication, gradual refactoring, effective documentation, team collaboration, and modern tools are your allies in tackling enhanced deprecation challenges.
By embracing these strategies, you can ensure your team is prepared to embrace the evolution of Java while maintaining efficiency and reliability in your codebase. As Java continues to evolve, staying ahead of deprecations will enhance not only code quality but also developer experience.
For further reading, consider checking out the official Java Documentation for best practices around deprecation and API design.