Common Pitfalls in Type-Safe Kubernetes Manifest DSL Usage
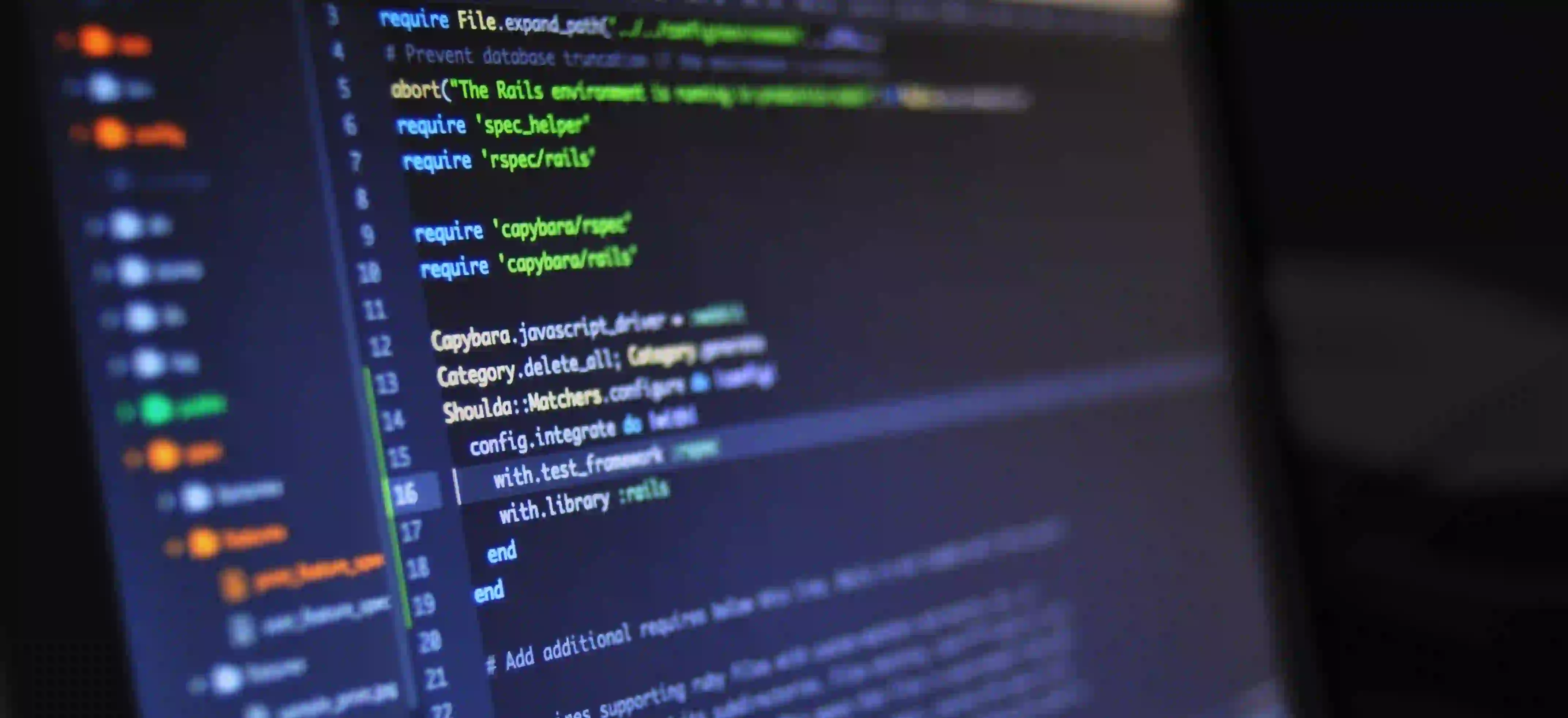
Common Pitfalls in Type-Safe Kubernetes Manifest DSL Usage
Kubernetes, the open-source container orchestration platform, is an essential tool for managing containerized applications in a clustered environment. While it simplifies deploying and scaling applications, creating and working with Kubernetes manifests can often be a challenging endeavor. To mitigate the complexities involved, type-safe Domain-Specific Languages (DSLs) have emerged as a proactive approach to defining these manifests. However, even with type safety at hand, developers can stumble upon several pitfalls. This blog post aims to highlight these common pitfalls, providing you a roadmap to navigate the complexities of using type-safe Kubernetes manifest DSLs effectively.
What is a Type-Safe Kubernetes Manifest DSL?
At its core, a type-safe Kubernetes manifest DSL provides a framework to define Kubernetes resources using programming language constructs, ensuring compile-time type checking. Examples include tools like Kustomize and the Kubernetes Client Libraries in various programming languages, such as Java and Golang.
Advantages of Type-Safe DSLs
- Early Error Detection: Reduce runtime errors through compile-time checks.
- Improved Readability: Code expressed as a DSL can be easier to understand than YAML files.
- Refactoring Support: When the DSL is integrated into a programming environment, developers can refactor their configurations efficiently.
With these benefits, it’s easy to see why more teams are adopting type-safe DSLs. However, pitfalls lurk in the shadows.
Common Pitfalls
1. Overcomplicating the DSL
Description
It may be tempting to extend the DSL for every nuance required by your application. However, adding excessive complexity can lead to maintainability issues.
Commentary
When creating Kubernetes manifests, keep it simple. Use annotations and attributes rather than creating custom types for every configuration element. This practice keeps the focus on essential configurations.
Code Example
import io.fabric8.kubernetes.api.model.PodBuilder;
PodBuilder pod = new PodBuilder()
.withNewMetadata()
.withName("example-pod")
.withNamespace("default")
.endMetadata()
.withNewSpec()
.addNewContainer()
.withName("nginx")
.withImage("nginx:latest")
.endContainer()
.endSpec();
By avoiding custom abstractions for simple configurations, you maintain clarity and ensure that future developers can easily grasp the purpose of your code.
2. Ignoring Kubernetes API Versions
Description
Kubernetes resources evolve over time, with frequent changes in API versions. It is crucial to specify the correct version to ensure compatibility.
Commentary
Always check the API version in your manifests. With type-safe DSLs, this should be a walk in the park, yet many developers overlook it. Using outdated API versions can lead to deprecated behaviors or even runtime failures.
Example Code Snippet
import io.fabric8.kubernetes.api.model.extensions.DeploymentBuilder;
// Specify the correct API version
DeploymentBuilder deployment = new DeploymentBuilder()
.withNewMetadata()
.withName("example-deployment")
.endMetadata()
.withNewSpec()
.withReplicas(3)
.withNewSelector()
.addToMatchLabels("app", "example")
.endSelector()
.withNewTemplate()
.withNewMetadata()
.addToLabels("app", "example")
.endMetadata()
.withNewSpec()
.addNewContainer()
.withName("example-container")
.withImage("example-image:latest")
.endContainer()
.endSpec()
.endTemplate()
.endSpec();
Ensure you check the official Kubernetes API documentation regularly for updates.
3. Overusing Dynamic Features
Description
Type-safe DSLs often provide dynamic features that allow configurations to be modified at runtime. While this versatility can be beneficial, it may add unnecessary complexity.
Commentary
Leverage dynamic features only when truly necessary. Over-relying on them can lead to fragile configurations that are difficult to debug.
import io.fabric8.kubernetes.api.model.ConfigMapBuilder;
import java.util.HashMap;
// Create a ConfigMap with dynamic features
HashMap<String, String> dataMap = new HashMap<>();
dataMap.put("key1", "value1");
ConfigMapBuilder configMap = new ConfigMapBuilder()
.withNewMetadata()
.withName("example-config")
.endMetadata()
.withData(dataMap);
Dynamic features can certainly simplify certain scenarios but should be handled with care to prevent added complexity in the long term.
4. Not Validating Manifests
Description
Even in type-safe DSLs, the translation to Kubernetes resources may introduce unexpected issues. Failing to validate the generated manifests can result in deployment failures.
Commentary
Make manifest validation a part of your workflow. Use tools like kubectl
to dry-run your deployments and check for any inconsistencies.
kubectl apply -f deployment.yaml --dry-run=client
This dry-run option allows you to check for errors without applying any changes.
5. Lack of Documentation
Description
Developers often overlook documenting their Kubernetes manifests, making future maintenance a chore for both themselves and others.
Commentary
Investing time in documentation pays off significantly. Type-safe DSLs allow for embedded comments, making it easy to explain complex configurations.
Code Example with Comments
import io.fabric8.kubernetes.api.model.ServiceBuilder;
// This Service will expose the deployment created earlier
ServiceBuilder service = new ServiceBuilder()
.withNewMetadata()
.withName("example-service")
.endMetadata()
.withNewSpec()
.addNewPort()
.withPort(80)
.withTargetPort(8080)
.endPort()
.withSelector(Map.of("app", "example")) // Selector to match Pods
.endSpec();
The embedded comments provide better context for future reference and aid collaborators who might work on the configuration later.
Wrapping Up
Navigating through the abyss of Kubernetes manifest DSLs may seem daunting, but being aware of these common pitfalls can help you streamline the process significantly. By avoiding over-complication, ensuring proper API versions, being cautious with dynamic features, validating manifests, and fostering a culture of documentation, your journey can become much smoother.
Further Reading
For additional information on Kubernetes resource definitions, check out the official Kubernetes Documentation and the Fabric8 Kubernetes Java Client.
By keeping these tips in mind, you can realize the full potential of your type-safe Kubernetes manifest DSL, leading to streamlined deployments and more maintainable configurations. Happy coding!