Unlocking Java Reflection: Common Pitfalls to Avoid
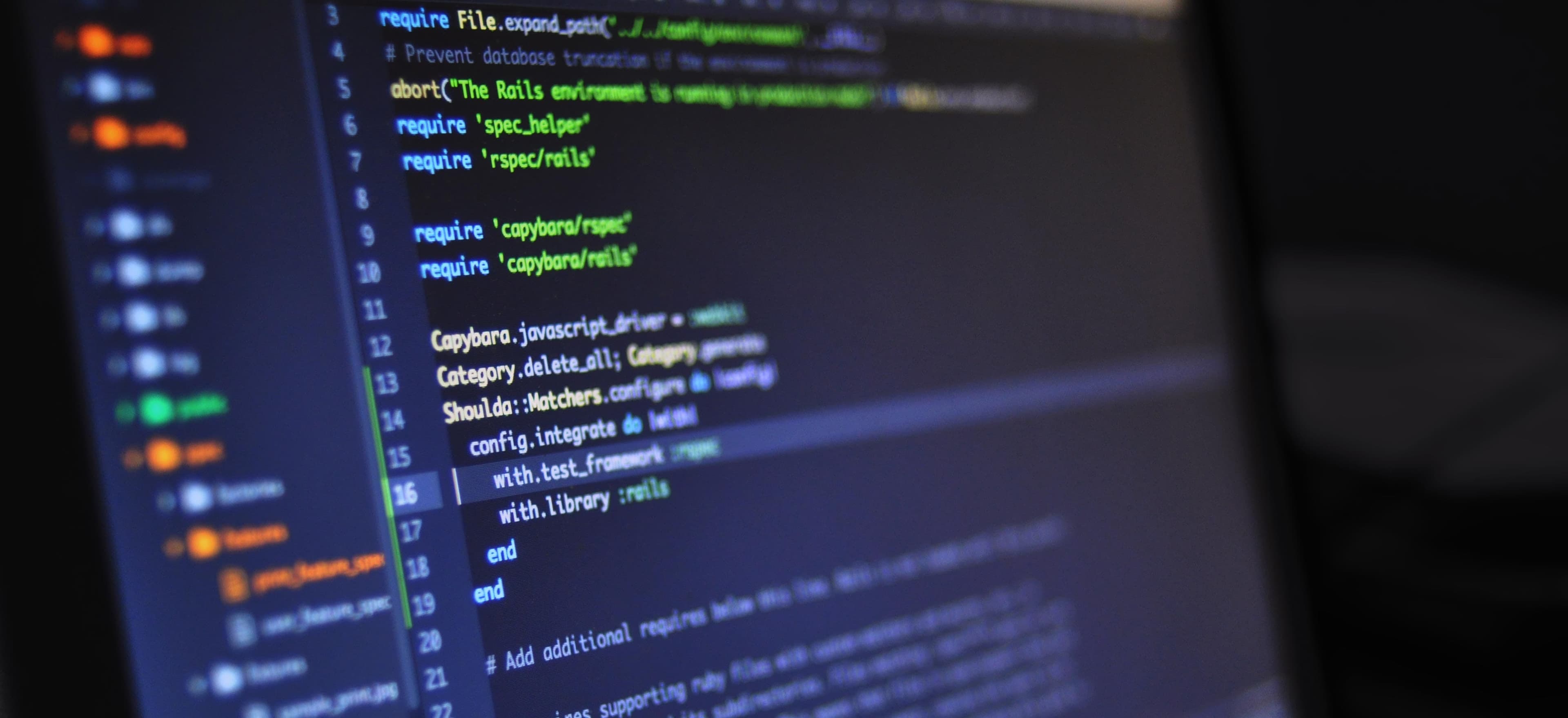
- Published on
Unlocking Java Reflection: Common Pitfalls to Avoid
Java reflection is a powerful feature that enables developers to inspect and manipulate classes, methods, fields, and more at runtime. While this flexibility can facilitate dynamic programming, it often leads to various pitfalls if developers are not cautious. In this blog post, we'll explore common pitfalls associated with Java reflection and provide some best practices to help you navigate this complex feature effectively.
Understanding Java Reflection
Before diving into the pitfalls, let’s quickly review what Java reflection entails. Reflection means examining or modifying the runtime behavior of applications. The reflective capabilities of Java include:
- Accessing private fields or methods.
- Creating instances of classes dynamically.
- Invoking methods at runtime.
- Retrieving metadata about classes (like annotations or interfaces).
To illustrate the concept, here is a simple code snippet that uses reflection to call a private method:
import java.lang.reflect.Method;
public class ReflectionExample {
private void secretMethod() {
System.out.println("Secret method invoked!");
}
public void invokeSecret() throws Exception {
Method method = this.getClass().getDeclaredMethod("secretMethod");
method.setAccessible(true); // Bypass accessibility check
method.invoke(this); // Invoking the method
}
public static void main(String[] args) {
try {
new ReflectionExample().invokeSecret();
} catch (Exception e) {
e.printStackTrace();
}
}
}
In this code snippet, the invokeSecret
method uses reflection to access the private method secretMethod
. The call to setAccessible(true)
allows us to bypass the default access control checks.
While this feature is useful, it comes with a handful of pitfalls.
Pitfall 1: Performance Overhead
One of the significant downsides of using reflection is the performance hit it takes compared to normal method calls. Reflection involves dynamic type resolution and type checks at runtime, which can introduce overhead.
Why it Matters
If your application makes frequent reflective calls during critical performance paths, it can lead to noticeable slowdowns. For instance, consider a web application that dynamically invokes methods using reflection for every request. This practice can significantly impact response time.
Best Practice
Whenever possible, avoid reflection for performance-sensitive code. Instead, use standard Java method calls. If you still need to use reflection, consider caching method lookups to mitigate some performance impact.
Pitfall 2: Careless Use of setAccessible()
When you call setAccessible(true)
, you bypass Java's access control checks, which can be risky. Doing so can lead to maintenance headaches, especially if the actual access levels or contracts of your API are violated.
Why it Matters
Using reflection to alter the visibility of fields or methods can break encapsulation, making your codebase fragile and harder to maintain. If a method becomes private in a future version, calls made via reflection will still work, but this can cause unpredictable behavior in your code.
Best Practice
Use setAccessible(true)
sparingly and only when absolutely necessary. Ensure that you document these instances clearly to aid in code maintenance over time. If possible, reconsider whether the design could be refactored to avoid reflection.
Pitfall 3: Security Issues
Reflecting on certain classes may expose sensitive information or allow unauthorized access to objects, leading to security vulnerabilities.
Why it Matters
If used improperly, reflection can expose private data or methods that shouldn't be accessible. For example, when writing frameworks or libraries that leverage reflection, it is crucial to ensure secure access.
Best Practice
Whenever reflection is involved, consider security implications. Restrict the usage of reflection in sensitive portions of your application and always ensure that you validate the data being manipulated. This keeps your application more secure.
Pitfall 4: Difficult Debugging and Maintenance
Reflection can often make the code hard to understand, leading to challenging debugging experiences. Because the typical static type inspections are absent, IDE features like autocompletion or refactoring tools might not work properly.
Why it Matters
Code readability and maintainability suffer when reflection is overused. Future developers (and even you) will struggle to understand what is being done.
Best Practice
Limit the use of reflection to well-defined scenarios. It’s good practice to encapsulate reflective logic within a dedicated utility class. This way, you separate the reflection part from the business logic, improving maintainability.
Example of Reflection Utility
To improve code organization, you might choose to create a utility class that handles reflectively invoked methods:
import java.lang.reflect.Method;
public class ReflectionUtil {
public static Object invokeMethod(Object target, String methodName, Object... args) throws Exception {
Method method = target.getClass().getDeclaredMethod(methodName, getParameterTypes(args));
method.setAccessible(true);
return method.invoke(target, args);
}
private static Class<?>[] getParameterTypes(Object... args) {
return Arrays.stream(args)
.map(arg -> arg == null ? Object.class : arg.getClass())
.toArray(Class<?>[]::new);
}
}
This utility class contains a method invokeMethod
, which encapsulates the reflective logic, maintaining a cleaner separation of concerns.
Pitfall 5: Lack of Compile-Time Checks
Java reflection works at runtime, meaning the compiler cannot perform type checks, leading to potential ClassNotFoundException
or NoSuchMethodException
errors at runtime instead of compile-time.
Why it Matters
These exceptions can lead to runtime failures, which, depending on the environment (production, testing), can have a substantial impact.
Best Practice
Incorporate enough error handling to manage potential exceptions that can arise from reflection. Log these exceptions and provide fallback logic when necessary, which helps keep your application robust.
The Closing Argument
Java reflection is indeed a double-edged sword. Its capabilities can substantially ease certain programming scenarios, but with great power comes great responsibility. As developers, it's crucial to be aware of the pitfalls associated with reflection and to follow best practices to mitigate its downsides.
To deepen your understanding, consider diving into the official Java documentation on Java Reflection and Java Programming Conventions. With careful consideration and disciplined use, reflection can be a valuable asset in your Java toolbox while avoiding its common pitfalls.