Struggling with Selenium Test Maintenance? Here's the Solution!
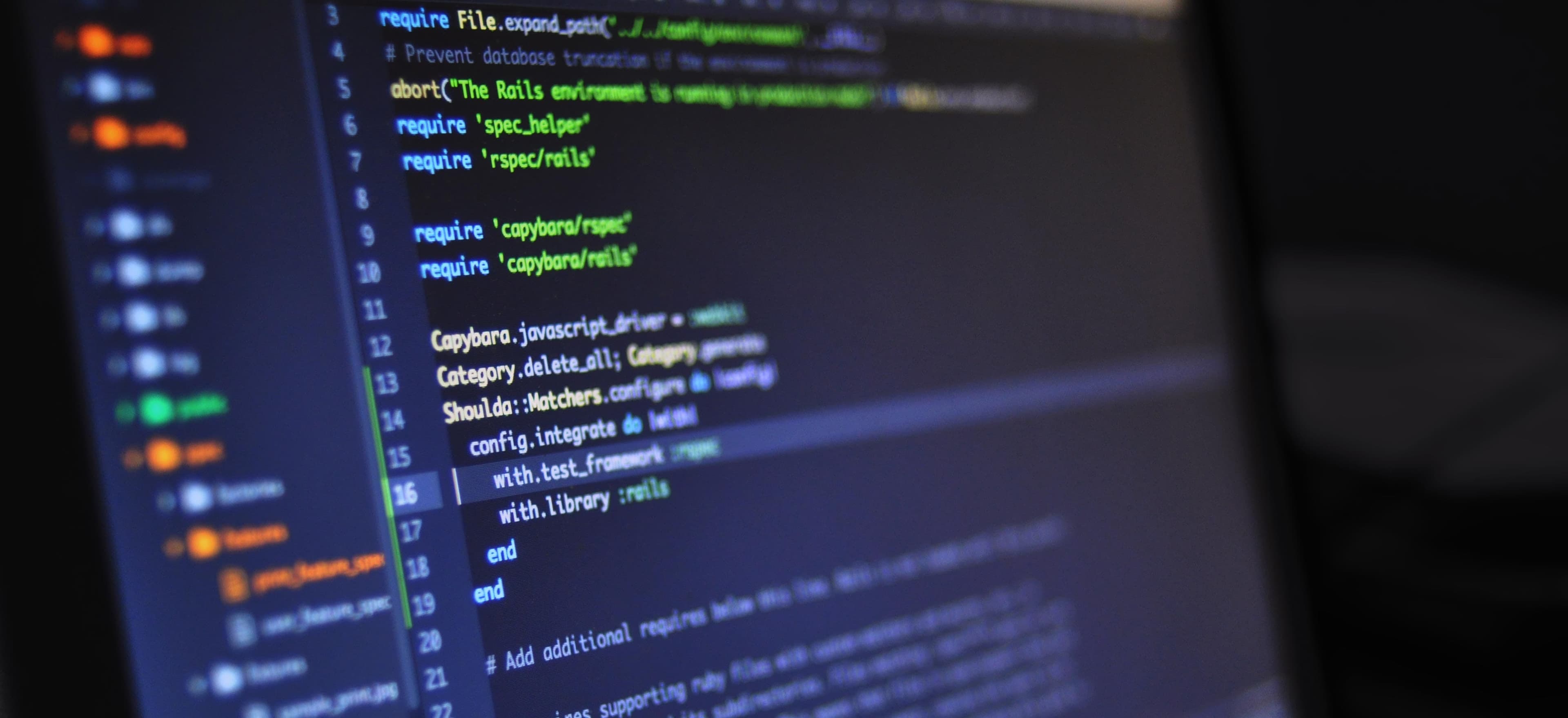
- Published on
Struggling with Selenium Test Maintenance? Here's the Solution!
As the demand for automation testing grows, the effectiveness of your Selenium tests can significantly influence the quality of your software products. However, many teams often grapple with the maintenance of these tests. This blog post will examine common challenges associated with Selenium test maintenance and provide actionable strategies to streamline your testing process.
The Challenge of Test Maintenance
Selenium is a powerful tool for automating web applications for testing purposes. Yet, maintaining a large suite of tests can be cumbersome. Here are a few challenges you may encounter:
- Frequent Changes in UI: Web applications undergo constant changes, which often leads to tests breaking due to element location changes.
- Test Instability: Flaky tests can increase maintenance overhead and reduce the trust in your test suite.
- Lack of Structure: Without a well-structured framework, tests can become difficult to read and maintain.
- Limited Reusability: If tests are not modular, reuse becomes a challenge, leading to code duplication.
Understanding these challenges is the first step toward easing your Selenium test maintenance burden.
Choosing the Right Framework
Selecting a robust test framework can alleviate many maintenance woes. Below is an excellent option you might consider.
JUnit
JUnit is a widely-used testing framework for Java applications. It integrates seamlessly with Selenium and offers clean syntax for structuring tests. A typical test case could look like this:
import org.junit.After;
import org.junit.Before;
import org.junit.Test;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class SampleTest {
private WebDriver driver;
@Before
public void setUp() {
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
driver = new ChromeDriver();
}
@Test
public void testGoogleSearch() {
driver.get("https://www.google.com");
// Test steps go here
}
@After
public void tearDown() {
driver.quit();
}
}
Why JUnit?
Using JUnit not only simplifies the structure of your tests but also provides features like annotations to help manage your setup and teardown processes effectively. This enhances readability and maintainability.
For a deeper dive into JUnit and its features, check out JUnit Official Documentation.
Implementing Page Object Model (POM)
The Page Object Model (POM) is a design pattern that enhances the maintainability of your Selenium tests. By encapsulating page elements and actions in separate classes, you can minimize code duplication.
Example of POM
Let's look at a simple implementation of the POM in a Selenium test:
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.By;
public class GoogleSearchPage {
private WebDriver driver;
public GoogleSearchPage(WebDriver driver) {
this.driver = driver;
}
public void enterSearchTerm(String searchTerm) {
WebElement searchBox = driver.findElement(By.name("q"));
searchBox.sendKeys(searchTerm);
searchBox.submit();
}
}
// Test case utilizing POM
@Test
public void testGoogleSearchWithPOM() {
GoogleSearchPage searchPage = new GoogleSearchPage(driver);
searchPage.enterSearchTerm("Selenium");
}
Why Use POM?
- Encapsulation: Each page is encapsulated in its own class, making it easier to locate and manage.
- Code Reusability: Reusing page objects across your tests minimizes redundancy and maintenance efforts.
- Improved Readability: Your tests will read more like actual user actions, making it easier for non-tech stakeholders to understand.
For more on POM, visit SeleniumHQ's POM Documentation.
Handle Dynamic Elements
Often, websites feature elements that load asynchronously or change IDs frequently. Stale elements can pose challenges for test stability. Here are some approaches to handle this:
Utilize Explicit Waits
Use explicit waits to ensure your tests wait for elements to be present before interacting with them. Here’s how:
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
public class WaitExample {
private WebDriver driver;
public WaitExample(WebDriver driver) {
this.driver = driver;
}
public void waitForElement() {
WebDriverWait wait = new WebDriverWait(driver, 10);
WebElement element = wait.until(ExpectedConditions.presenceOfElementLocated(By.id("dynamicElementId")));
// Now you can safely interact with the element
}
}
Why Use Explicit Waits?
Explicit waits help manage timing issues without making your tests dryer. They only wait as long as needed, improving test execution time.
Continuous Integration (CI)
Integrating your Selenium tests into a continuous integration pipeline can simplify maintenance. By running tests automatically upon each code commit, issues can be identified early. Tools like Jenkins or GitHub Actions can help streamline this process.
Sample GitHub Actions Configuration
Here’s how you could set up GitHub Actions to run your Java Selenium tests:
name: Java CI
on:
push:
branches: [ main ]
pull_request:
branches: [ main ]
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Set up JDK 11
uses: actions/setup-java@v1
with:
java-version: '11'
- name: Run tests
run: ./gradlew test
Why Use CI?
- Immediate Feedback: Get instant notifications about test failures.
- Stability: Regularly run tests to ensure stability among code changes.
- Reduced Manual Efforts: Automate the testing process to save valuable time.
The Closing Argument
Maintaining a suite of Selenium tests should not feel overwhelming. By implementing best practices like using a structured framework, adopting the Page Object Model, managing dynamic elements with explicit waits, and implementing continuous integration, you can drastically improve your Selenium test maintenance process.
Automating testing is a powerful asset for software development teams, and taking open steps to make your tests robust will yield long-term benefits, including fewer bugs and higher quality software products.
For more insights into leveraging the power of Selenium, check out SeleniumHQ's Official Documentation.
Through understanding and application, you can turn the struggle of test maintenance into a streamlined process that enhances productivity and software quality. Happy testing!
Checkout our other articles