Common Gradle Archetype Mistakes in Spring Projects
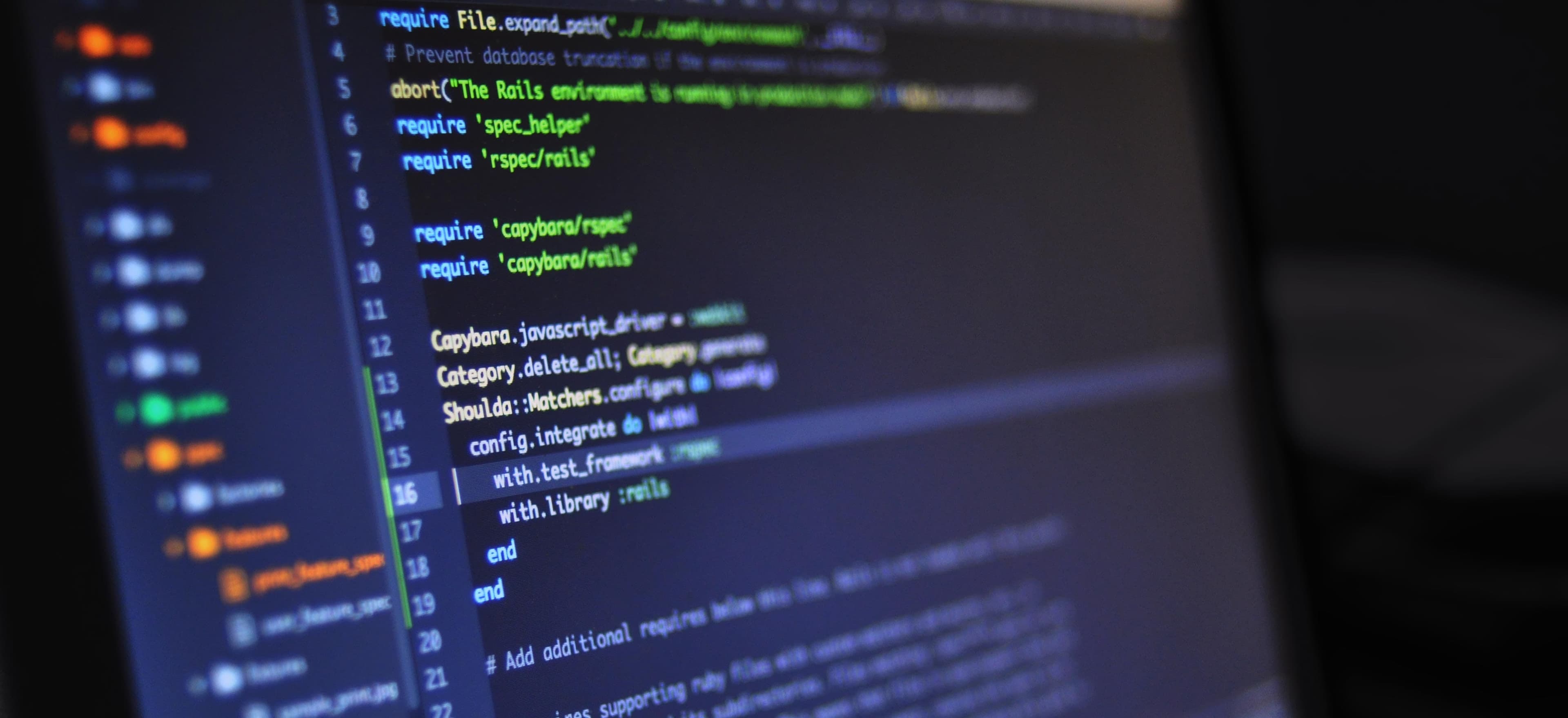
- Published on
Common Gradle Archetype Mistakes in Spring Projects
Gradle has become one of the most popular build tools in the Java ecosystem, especially for Spring projects. It streamlines project setup, dependency management, and build processes, making development faster and more efficient. However, new developers often encounter pitfalls when setting up Gradle in their Spring projects. In this blog post, we will discuss some common Gradle archetype mistakes that can lead to frustrating complications and how to avoid them.
Table of Contents
Understanding Gradle and Spring
Before diving into common mistakes, it’s crucial to understand the symbiotic relationship between Gradle and Spring. Gradle provides the foundation for building Spring applications with ease. It enables dependency management through repositories, allows for concise build configurations, and integrates seamlessly with plugins like Spring Boot.
Key Features of Gradle
- Dependency Management: Transparent handling of project dependencies.
- Incremental Builds: Only rebuilds components that have changed.
- Easy Integration: Compatible with various tools and profiles.
Gradle can significantly enhance your Spring projects, but incorrect configurations can nullify these benefits. Let's explore common archetype mistakes that developers tend to make.
Common Gradle Archetype Mistakes
1. Ignoring Dependency Management
One of the biggest advantages of using Gradle is its ability to handle dependencies efficiently. New developers often forget to define their dependencies in a structured way, which may lead to unexpected behavior.
Example:
dependencies {
implementation 'org.springframework.boot:spring-boot-starter-web'
}
Why: The implementation
configuration only exposes the dependency to your project, not to consumers. This isolation helps to avoid dependency conflicts down the line.
Tip: Always scope your dependencies correctly. Using the proper configurations like api
, implementation
, runtimeOnly
, and testImplementation
can prevent version conflicts and clarify the structure of your project.
2. Improper Configuration of Build Files
Failing to configure build.gradle
appropriately is a common mistake. This can cause issues during compilation or runtime. New developers sometimes neglect vital configurations, such as Java compatibility.
Example:
java {
sourceCompatibility = '1.8'
targetCompatibility = '1.8'
}
Why: If you don’t set the Java version, Gradle might use the default version installed on your machine, which could differ from what your project requires. This can lead to NoClassDefFoundError
or ClassNotFoundException
.
Tip: Always specify the Java version that is consistent with your application. This ensures compatibility across different development environments.
3. Inconsistent Plugin Usage
Inconsistently applying plugins can cause confusion and inconsistency across various modules of your Spring project. This not only makes your project hard to maintain but can also result in missed features or vulnerabilities.
Example:
plugins {
id 'org.springframework.boot' version '2.5.4'
id 'io.spring.dependency-management' version '1.0.11.RELEASE'
id 'java'
}
Why: Using the Spring Boot plugin simplifies tasks such as creating executable JAR files and running the application, while the dependency management plugin helps you manage versions of your dependencies effectively.
Tip: Always apply necessary plugins at the project level to ensure consistency. Refer to the Spring Boot Plugin documentation for more insights.
4. Not Utilizing Gradle Wrapper
Another frequent misstep is not using the Gradle wrapper. Developers sometimes rely on system-installed Gradle versions, which can lead to compatibility issues when sharing the project.
Example:
To add the Gradle wrapper, run:
gradle wrapper --gradle-version=7.2
Why: The Gradle wrapper creates scripts that allow developers to build projects with a specific, pre-defined version of Gradle. This ensures consistency across different environments.
Tip: Always commit the generated gradlew
and gradlew.bat
files to your version control system. This way, team members can build the project without needing to install Gradle individually.
5. Neglecting Multi-Module Projects
In larger applications, it is common to split the project into multiple modules. However, many developers fail to set up multi-module structure properly, leading to tight coupling and poor maintainability.
Example Structure:
my-spring-project
├── settings.gradle
├── build.gradle
├── module-one
│ └── build.gradle
└── module-two
└── build.gradle
Why: Each module can have its own build.gradle
file, which keeps concerns separated and encourages modularization.
Tip: Use settings.gradle
to include modules:
include 'module-one', 'module-two'
Lessons Learned
Gradle is an excellent tool for managing Spring projects. However, awareness of common pitfalls can save you a lot of time and trouble.
By managing dependencies carefully, configuring build files correctly, applying plugins consistently, using the Gradle wrapper, and structuring multi-module projects sensibly, you will set a strong foundation for success.
Understanding these common archetype mistakes is essential to ensure your Gradle build runs smoothly. If you're looking to dive deeper into Gradle, check the official Gradle documentation for comprehensive insights and configurations.
Integrate these best practices into your workflow, and you’ll enhance not only your development efficiency but also the maintainability of your Spring projects! Happy coding!
Checkout our other articles