Converting Short to Byte Array: Common Pitfalls to Avoid
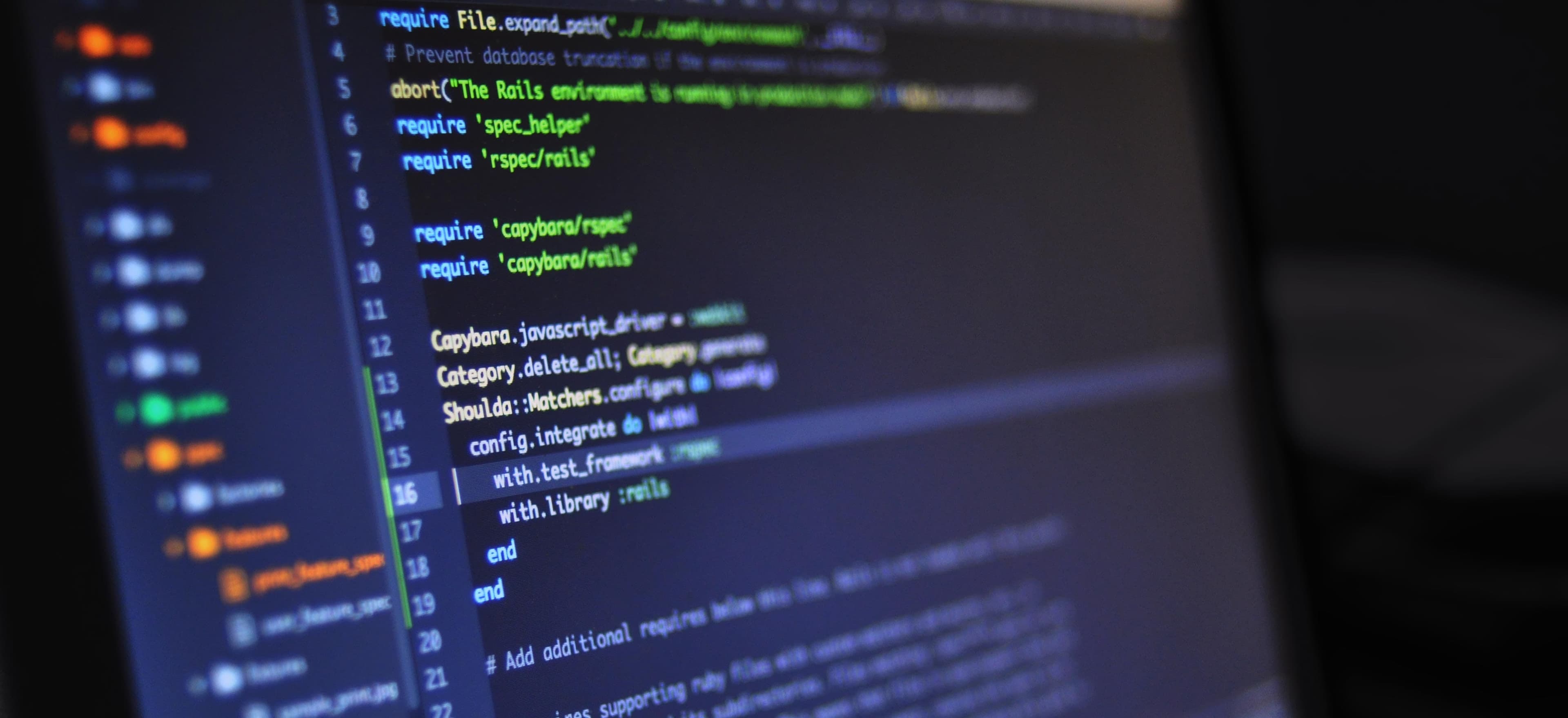
- Published on
Converting Short to Byte Array: Common Pitfalls to Avoid
When working with Java, one frequently encounters the need to convert various data types into byte arrays. This is particularly true for the short
data type, which requires careful handling to ensure data integrity and accuracy. In this blog post, we'll delve into the process of converting a short
to a byte array, discuss the common pitfalls developers may encounter, and provide practical, engaging examples.
Understanding Short in Java
Before we dive into conversions, let's clarify what a short
is in Java. The short
type is a 16-bit signed two's complement integer. It ranges from -32,768 to 32,767. This means it takes up less memory than an int
, making it useful in memory-sensitive applications.
Why Convert Short to Byte Array?
Converting a short
to a byte array is vital for:
- Network Communication: Sending data over networks often requires byte-level manipulation.
- File Storage: Binary formats often dictate the need for converting integers into byte arrays.
- Data Serialization: When saving tool data structures in a serial manner, utilizing byte arrays becomes necessary.
Conversion Techniques
To convert a short
to a byte array in Java, you can use various methods. Let's discuss a common and efficient method using bitwise operations.
Using Bitwise Operations
Here’s a simple and effective way to perform the conversion using bitwise operations:
public static byte[] shortToByteArray(short value) {
return new byte[] {
(byte) (value >> 8),
(byte) value
};
}
Code Explanation:
- We define a method named
shortToByteArray
that takes ashort
parameter. - We create a byte array of size two because a
short
is represented by two bytes. - The first byte is determined by shifting the
short
value 8 bits to the right. - The second byte directly takes the
short
value.
Example Usage
To see our method in action, consider the following snippet:
public static void main(String[] args) {
short originalValue = 12345;
byte[] byteArray = shortToByteArray(originalValue);
System.out.println("Original short value: " + originalValue);
System.out.println("Byte array: " + Arrays.toString(byteArray));
}
Output:
Original short value: 12345
Byte array: [48, 57]
Here, the short
value is effectively converted into its corresponding byte representation.
Common Pitfalls to Avoid
-
Incorrect Byte Order: One frequent error developers encounter is assuming the byte order. The above code uses big-endian order (most significant byte first), which is a standard but not universal. Ensure your application’s byte order matches your data protocol.
-
Using Unsigned Conversion: Java does not have an unsigned
short
type. Attempting to convert a negativeshort
to a byte may yield unintended results. Always validate the input if negative values could be possible. -
Ignoring Data Type Limits: Ensure that the byte array can accommodate all possible values. While a
short
occupies 16 bits, Java bytes are 8 bits. This means using larger data types or handling overflow correctly is crucial.
Converting Byte Array back to Short
It is equally important to know how to reverse the process. Here’s how you convert a byte array back into a short
:
public static short byteArrayToShort(byte[] byteArray) {
if (byteArray.length != 2) {
throw new IllegalArgumentException("Byte array must be of length 2");
}
return (short) ((byteArray[0] << 8) | (byteArray[1] & 0xFF));
}
Explanation of Reverse Conversion
- Array Length Check: Before processing, we check that the provided byte array has the correct length.
- Combining Bytes: We shift the first byte 8 bits to the left and combine it with the second byte, masking the second byte to ensure no sign bits interfere (by using
& 0xFF
).
Example Usage of Reverse Conversion
public static void main(String[] args) {
byte[] byteArray = {48, 57}; // representation of short 12345
short convertedValue = byteArrayToShort(byteArray);
System.out.println("Converted back to short: " + convertedValue);
}
Output:
Converted back to short: 12345
The reverse conversion solidifies our understanding of both processes, confirming that data integrity is maintained.
When You Should Use Libraries
While manipulation of primitive data types can feel straightforward, using libraries can often streamline processes and reduce human errors. Libraries like Apache Commons Lang or Guava provide utilities that can ease array manipulations.
Utilizing these libraries tends to promote best practices, making the codebase cleaner and less error-prone.
The Last Word
Converting a short
to a byte array in Java may seem trivial, but it comes with its own set of challenges and best practices. By adhering to the techniques outlined in this post, and keeping the common pitfalls in mind, developers can effectively manage data type conversions with confidence.
With such foundational knowledge, you can open the door to more advanced programming concepts requiring byte manipulations. Stick to sound coding principles, and embrace libraries when needed. Your applications will be all the better for it!
Checkout our other articles