Common Selenium WebDriver Errors in Java and How to Fix Them
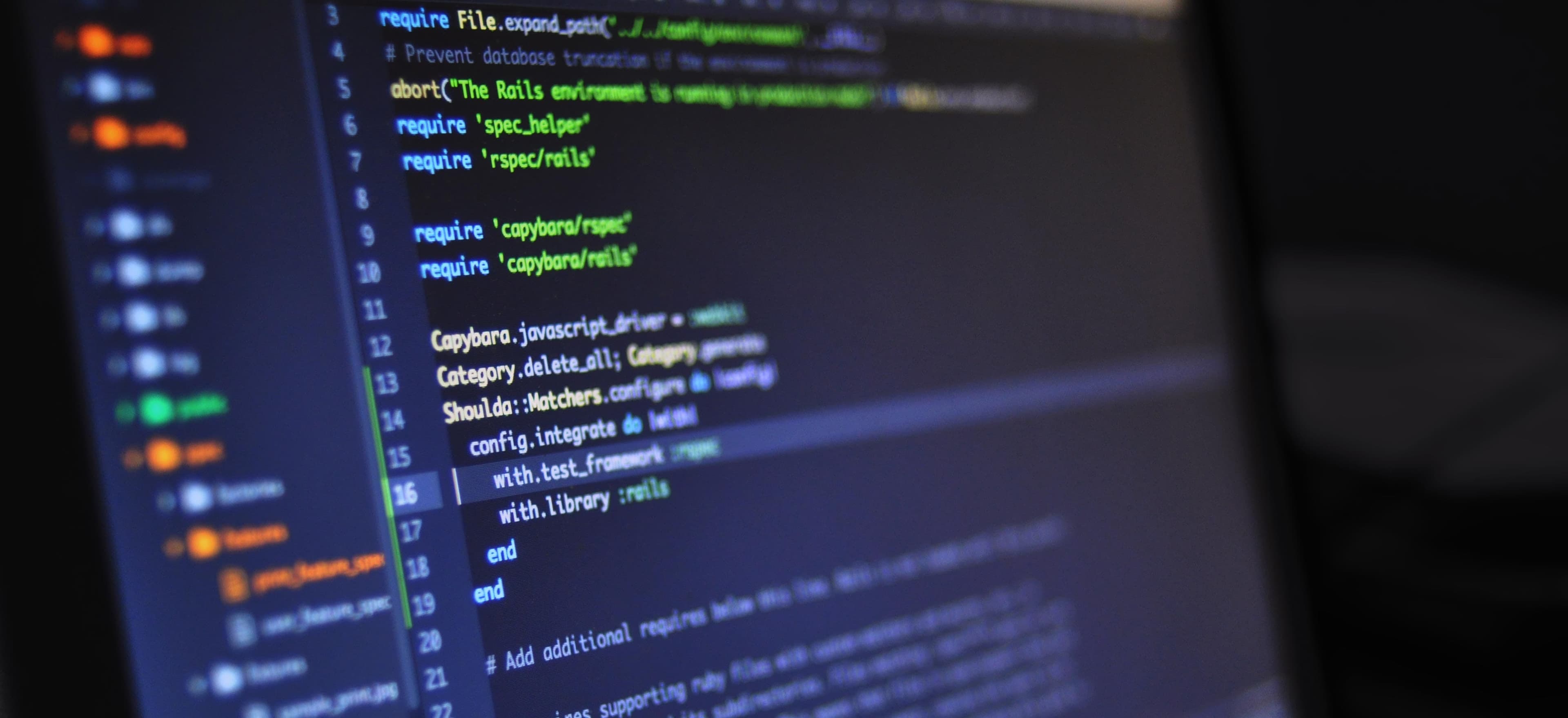
- Published on
Common Selenium WebDriver Errors in Java and How to Fix Them
Selenium WebDriver is a powerful tool for automating web application testing. However, like any other technology, it has its share of challenges. This blog post delves into common Selenium WebDriver errors experienced by Java developers, providing insights into their causes and offering practical solutions.
Table of Contents
Starting Off
Automated testing is integral to ensuring that web applications function as intended. Selenium WebDriver, a crucial component of Selenium, allows developers to write tests for web applications in various programming languages, including Java. Although powerful, the framework can be prone to some errors that developers encounter during their testing journeys.
This post aims to clarify some of the most common issues developers face while using Selenium WebDriver with Java, alongside practical solutions to overcome them.
Common Errors
1. NoSuchElementException
Description: This error occurs when Selenium cannot locate an element on the webpage that the code tries to interact with.
Example:
WebDriver driver = new ChromeDriver();
driver.get("http://example.com");
WebElement element = driver.findElement(By.id("non-existent-id"));
Fix: Ensure the element is present in the DOM before interacting with it. It’s essential to wait for the element to load completely. Utilize explicit waits as demonstrated below:
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
WebElement element = wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("existent-id")));
This approach helps in handling dynamic content loading issues and minimizes the chances of encountering NoSuchElementException.
2. TimeoutException
Description: A TimeoutException arises when a command does not complete in a specified timeframe, usually related toImplicit wait or explicit wait.
Example:
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(5));
WebElement element = wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("slow-loading-element")));
Fix: Increase the wait time if the element takes longer to load, or use a condition that fits your situation better.
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(20));
WebElement element = wait.until(ExpectedConditions.elementToBeClickable(By.id("slow-loading-element")));
Setting an appropriate timeout can greatly enhance your testing experience by allowing sufficient time for elements to appear.
3. StaleElementReferenceException
Description: This exception occurs when the element referenced in the WebDriver code no longer resides in the DOM. This usually happens when the page has been refreshed or changed since the element was found.
Example:
WebDriver driver = new ChromeDriver();
driver.get("http://example.com");
WebElement element = driver.findElement(By.id("fresh-element"));
// Simulate some action that might refresh the DOM
driver.navigate().refresh();
element.click(); // This will throw StaleElementReferenceException
Fix: Re-locate the element after the DOM changes. You might need to wrap the interaction in a try-catch block.
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
try {
WebElement element = driver.findElement(By.id("fresh-element"));
element.click();
} catch (StaleElementReferenceException e) {
WebElement element = driver.findElement(By.id("fresh-element")); // Re-find the element
element.click();
}
This method ensures that you're working with the latest reference of the web element.
4. ElementNotVisibleException
Description: This error indicates that the element you are trying to interact with is present in the DOM but is not visible to the user.
Example:
WebDriver driver = new ChromeDriver();
driver.get("http://example.com");
WebElement element = driver.findElement(By.id("hidden-element"));
element.click(); // Throws ElementNotVisibleException
Fix: Ensure that the element is visible before the interaction.
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
WebElement element = wait.until(ExpectedConditions.elementToBeClickable(By.id("visible-element")));
element.click();
Not only does this practice prevent unnecessary exceptions, but it also contributes to more reliable tests.
5. WebDriverException
Description: This is a generic error thrown when WebDriver fails to execute a command. The root cause can often be difficult to trace.
Example:
WebDriver driver = new ChromeDriver();
driver.get("http://example.com");
driver.quit(); // If you try to interact after quitting, it throws WebDriverException
Fix: Check the state of the WebDriver before executing any actions. Also, ensure that the WebDriver binaries are up to date.
if (driver != null) {
driver.quit();
}
Regularly updating your WebDriver binaries helps mitigate compatibility issues between your code and the browser.
Best Practices
To prevent the occurrence of errors, consider adopting the following practices:
- Use Explicit Waits: Waiting for elements to appear can significantly reduce the chance of encountering common exceptions.
- Handle Exceptions Gracefully: Utilize try-catch blocks to handle exceptions and allow your test to continue when possible.
- Re-Find Elements: If a DOM change is suspected, always retrieve the element again rather than assuming the old reference is valid.
- Keep WebDriver Updated: Stay on top of WebDriver updates to ensure compatibility with the browsers you are testing against.
- Adopt a Page Object Model: This design pattern helps in maintaining code organization and reusability, making the test scripts easier to manage and debug.
Final Considerations
Selenium WebDriver presents remarkable opportunities for automated testing in Java, but it also requires careful consideration of its common pitfalls. No one wants their test scripts to fail due to unanticipated errors, resulting in wasted time and resources.
By understanding these common Selenium WebDriver errors and implementing the provided solutions and best practices, you can bolster your testing strategy. Automating web applications efficiently becomes a straightforward task when armed with the right knowledge. For more information about Selenium best practices, check out SeleniumHQ Documentation.
Happy testing!
Checkout our other articles