Overcoming Java 9 Compatibility Issues in Your App Development
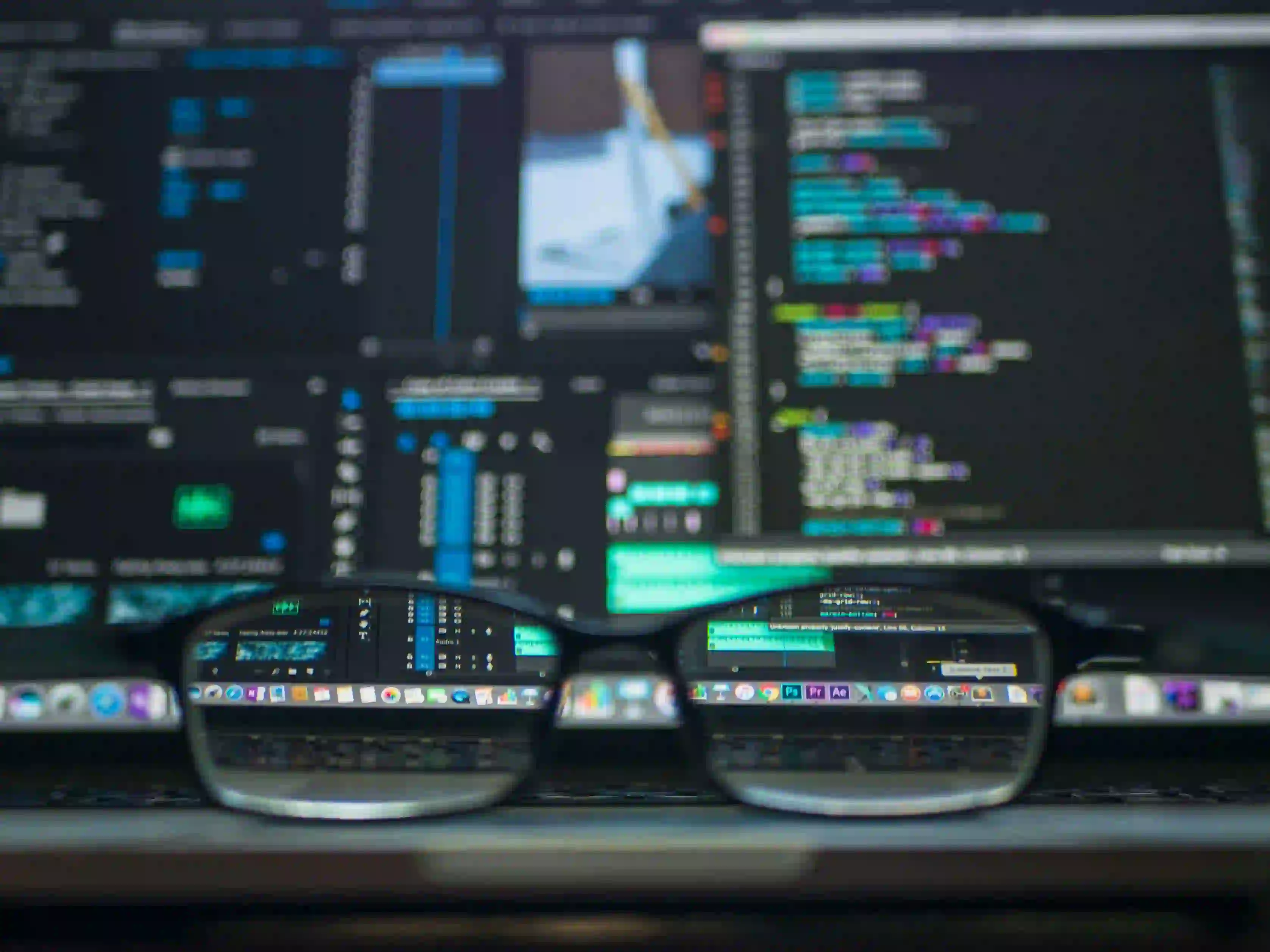
Overcoming Java 9 Compatibility Issues in Your App Development
Java has long been a staple programming language that developers rely upon, largely due to its object-oriented design and platform independence. However, with the introduction of Java 9, many changes and enhancements were made that could lead to compatibility issues in existing applications. This blog post aims to navigate through the hurdles you might encounter when upgrading to Java 9, alongside best practices for resolving them.
Understanding Java 9 Changes
Before we delve into compatibility issues, it's important to understand some key changes in Java 9:
-
Module System (Project Jigsaw): Java 9 introduced a module system that encapsulates packages and resources into modules. This allows for better organizing large codebases.
-
JShell: A Read-Eval-Print Loop (REPL) tool that lets developers execute Java code snippets interactively.
-
Improved APIs: The addition of several APIs, such as the
jshell
package, thejava.util.stream
updates, and enhancements in thejava.math
library. -
New Garbage Collection (GC) Options: More options for GCs, improving performance.
Understanding these features will help you mitigate the compatibility issues associated with them.
Common Compatibility Issues
1. Java Module System
One of the most significant improvements with Java 9 is the introduction of the module system. If your existing applications are not modularized, or if you're using third-party libraries that have not yet been remodeled for the module system, you may encounter serious runtime errors.
Solution: Modularize Your Application
Moving to a modular structure may seem daunting, but it's beneficial for long-term maintainability. Start by decomposing your application into modules.
Here's how you can define a simple module:
// module-info.java
module com.example.mymodule {
exports com.example.mymodule.api;
requires com.example.dependency;
}
Why: The exports
directive makes the specified package available to other modules, while requires
specifies dependencies. By modularizing, you can avoid runtime errors and take advantage of improved maintainability.
2. Changes in the Standard API
Java 9 deprecated several methods and classes in its standard libraries. This can lead to compilation errors in applications that rely on these outdated features. An example would be the removal of Thread.stop()
.
Solution: Update Your Code
In order to resolve deprecations, examine the new alternatives or APIs provided by the Java 9 release notes. Here's how you could potentially handle a thread without using deprecated methods:
public class SafeRunnable implements Runnable {
private volatile boolean running = true;
public void run() {
while (running) {
// perform task
}
}
public void stop() {
running = false;
}
}
Why: Instead of calling Thread.stop()
, which is unsafe, we implement a volatile
boolean flag to safely control the thread execution. This approach adheres to best practices regarding thread management in Java.
3. Third-Party Libraries
If your application relies on third-party libraries, make sure they are compatible with Java 9. Some libraries may not support the module system, leading to NoClassDefFoundError
.
Solution: Update Libraries
Always check the official documentation for the libraries you are using. The library’s maintainers usually offer newer versions that have resolved existing issues. For instance, libraries like Apache Commons have updated their frameworks to be compatible with Java 9.
4. JShell and Applications
Though JShell is beneficial for interactive learning or testing, embedding JShell into your application can lead to convoluted issues, such as namespace conflicts.
Solution: Keep it Separate
JShell should be primarily used for testing purposes. If you must integrate it into an application, be cautious about namespace usage. Here’s a simple snippet to execute in JShell safely:
import java.util.stream.IntStream;
IntStream.range(1, 10)
.filter(n -> n % 2 == 0)
.forEach(System.out::println);
Why: JShell can offer real-time feedback for streaming operations, which can greatly enhance your coding experience without affecting your application.
Practical Steps to Transition to Java 9
-
Code Refactoring: Identify deprecated APIs and refactor your code. This requires thorough testing to ensure functional integrity.
-
Utilize Module System: Take time to understand the module system. Create a plan to transition your application to utilize Java modules effectively.
-
Testing: Rigorously test your application using JUnit or another testing framework to identify any unforeseen issues.
-
Documentation & Community Help: Refer to the official Java documentation for a detailed overview of changes. Engaging with community forums like Stack Overflow can provide additional insights and solutions.
Lessons Learned
Upgrading to Java 9 doesn’t have to be a painful endeavor. With proper planning, understanding of Java 9’s enhancements, and refactoring of existing code, developers can overcome compatibility issues and even take advantage of new features. By modularizing and updating third-party libraries, you set your application up for long-term success while adhering to best practices.
Feel free to share your experiences or questions about upgrading to Java 9 in the comments below! Happy coding!