Transforming Assertions: The Power of a Domain-Specific Language
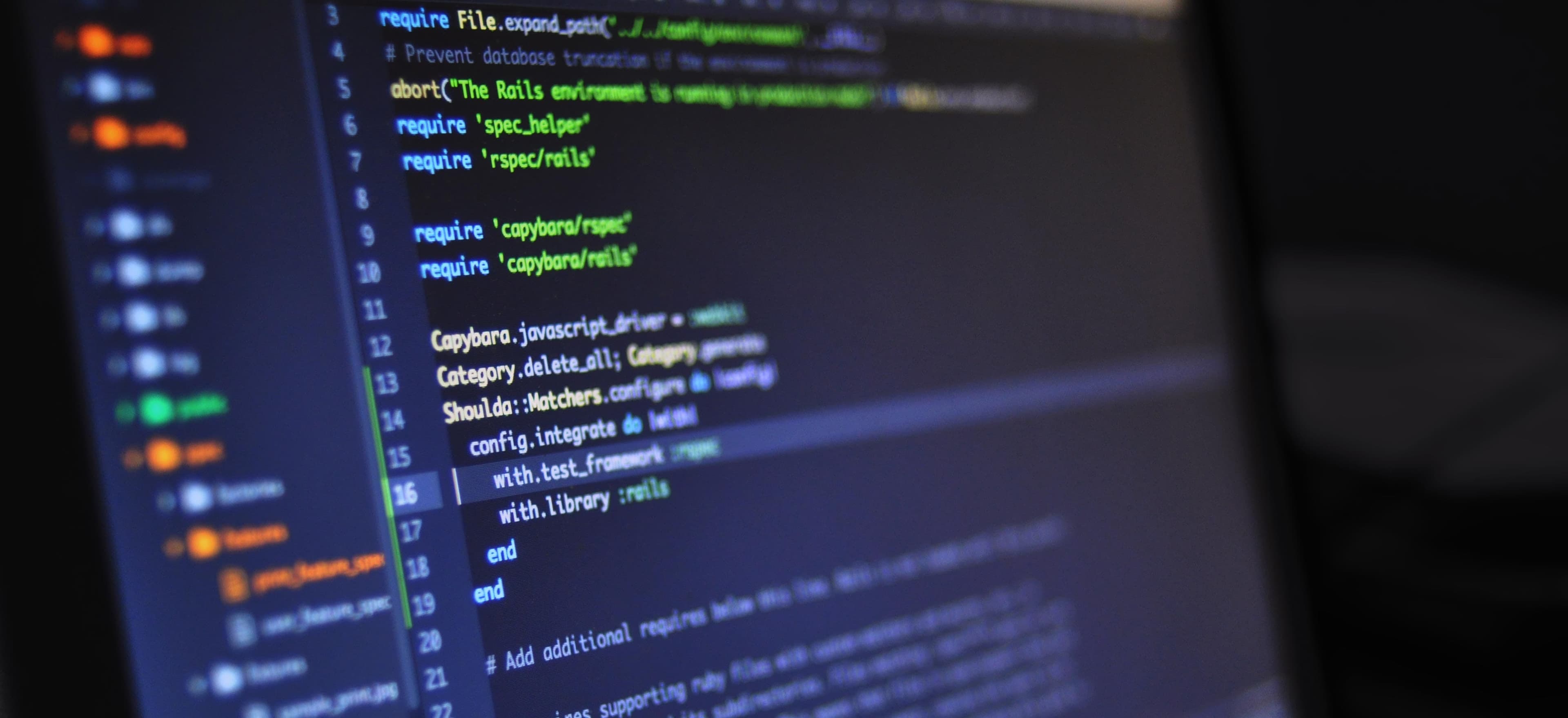
- Published on
Transforming Assertions: The Power of a Domain-Specific Language
Assertions are an integral part of software development, allowing developers to validate their assumptions and catch bugs early in the development cycle. However, the traditional assertion methods can sometimes be verbose, limiting their expressiveness and usability. This is where Domain-Specific Languages (DSLs) come into play. In this blog post, we will explore how transforming assertions into a DSL can streamline your development process, making your code clearer and more manageable.
What is a Domain-Specific Language?
A Domain-Specific Language is a programming language or specification language dedicated to a particular problem domain, a particular solution technique, or a particular technology. Unlike general-purpose programming languages like Java or Python, a DSL is tailored to express solutions in a specific context. This custom approach allows for a more intuitive and efficient expression of concepts relevant to that domain.
For further reading on DSLs, check out Martin Fowler’s article on DSLs.
Why Use a DSL for Assertions?
1. Enhanced Readability
When assertions are written using a DSL, the code becomes easier to read and understand. By abstracting complex logic into simple, declarative statements, developers can focus on the "what" rather than the "how."
2. Improved Maintainability
A well-designed DSL allows developers to express complex business rules or assertions more succinctly, making it easier to maintain. Changes to business rules can often be made by updating the DSL definitions rather than rewriting extensive amounts of code.
3. Increased Expressiveness
A DSL can introduce constructs that are specific to the domain, allowing for more nuanced assertions. This linguistic expressiveness increases the range of possible assertions that can be made while reducing ambiguity.
4. Testing and Validation
When assertions are expressed in a DSL, it becomes easier to automate testing and validation. By defining assertions in a structured way, developers can programmatically verify assumptions within the application.
Designing Your Assertion DSL
Let’s explore how to design a simple assertion DSL in Java. We'll start by creating a DSL that allows us to assert that our objects meet certain conditions.
Lexical Structure
Consider a scenario where we want to assert that a user’s age meets certain conditions. We will define our DSL as follows:
- Assert: This will be the main entry point.
- Is: This will be a helper method to define conditions.
- Valid: This will confirm that the assertion is true.
Let’s implement this.
Code Example: Basic Structure of Assertion DSL
public class Assert {
private final boolean condition;
private final String message;
private Assert(boolean condition, String message) {
this.condition = condition;
this.message = message;
}
public static Assert is(boolean condition) {
return new Assert(condition, null);
}
public Assert valid() {
if (!condition) {
throw new AssertionError(message != null ? message : "Assertion failed");
}
return this;
}
public Assert withMessage(String message) {
this.message = message;
return this;
}
}
Commentary
In this initial code structure:
-
The Assert class: This class encapsulates the assertion logic. The constructor is private to prevent direct instantiation, enforcing the use of the static
is
method. -
Static method
is
: This initializes the assertion with a condition. -
Method
valid
: This method checks the assertion. If the condition is false, it throws anAssertionError
. -
Method
withMessage
: This allows adding a custom error message to the assertion.
Using the DSL
Now, let’s use our DSL in practice. We want to assert that a user’s age is greater than 18.
public class User {
private int age;
public User(int age) {
this.age = age;
}
public void validate() {
Assert.is(this.age > 18)
.withMessage("User must be over 18 years old")
.valid();
}
}
Commentary
In the validate
method of the User
class, we're using our DSL to assert that the user's age is greater than 18. If it’s not, a clear error message is thrown, making it immediately obvious why the assertion failed. This enhances both clarity and debugging ease.
Expanding the DSL: Composability
As the complexity of the application grows, you may find the need to compose multiple assertions. The good news is that DSLs can typically be designed for composability.
Enhancing the DSL with Composable Conditions
Let’s extend our DSL to support composable assertions. We can add static methods for common conditions like "greater than," "less than," etc.
public class AssertionBuilder {
public static Assert isGreaterThan(int value, int threshold) {
return Assert.is(value > threshold)
.withMessage(value + " must be greater than " + threshold);
}
public static Assert isLessThan(int value, int threshold) {
return Assert.is(value < threshold)
.withMessage(value + " must be less than " + threshold);
}
}
Using Composable Assertions
Leveraging the updated DSL, let's validate our user further:
public void validate() {
AssertionBuilder.isGreaterThan(this.age, 18).valid();
AssertionBuilder.isLessThan(this.age, 65).valid();
}
Commentary
Here, we've introduced two static methods that define common boundaries: isGreaterThan
and isLessThan
. This increases the expressiveness of our assertions and simplifies the validation logic within the User
class.
The Bottom Line
By leveraging a Domain-Specific Language tailored for assertions, you can significantly enhance the readability, maintainability, and expressiveness of your code. The assertion DSL we designed allows developers to write clearer, more structured code that is easily extensible.
Implementing a DSL requires an investment in time and effort, but the long-term benefits on large projects can be substantial. As your system grows, finding ways to simplify and clarify your code will pay off in reduced bugs and increased developer efficiency.
Further Reading
If you're interested in learning more about creating your own DSLs or deep diving into assertion frameworks, consider the following resources:
- Creating a Domain-Specific Language in Java
- A Beginner's Guide to Domain Specific Languages
- Martin Fowler's Domain-Specific Languages
As software development evolves, so too must our tools and methodologies. By embracing the power of DSLs, you are one step closer to writing code that not only works but resonates with clarity and purpose.