Common Pitfalls When Integrating Apache Kafka with Camel
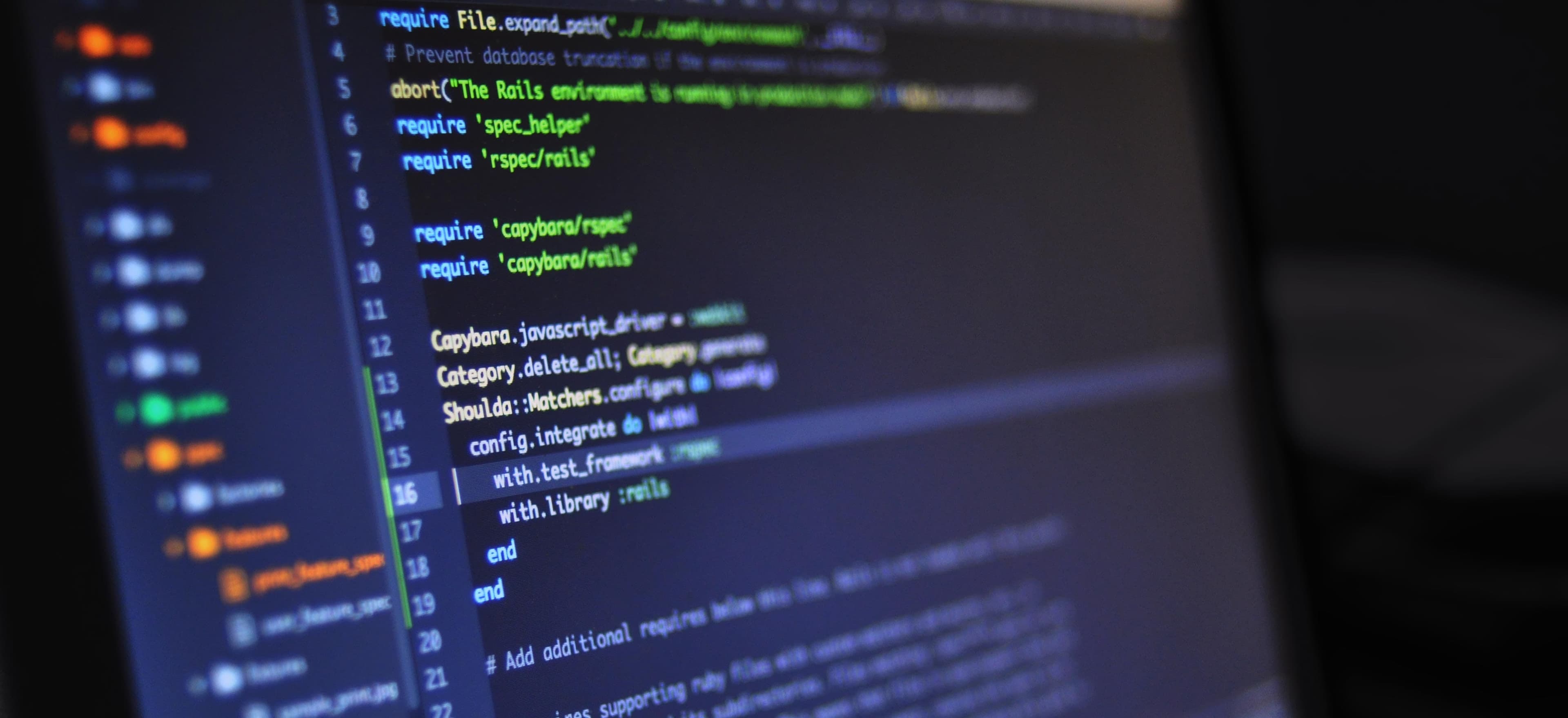
- Published on
Common Pitfalls When Integrating Apache Kafka with Camel
Integrating Apache Kafka with Apache Camel can unlock incredible capabilities for your applications. However, like any integration, it comes with common pitfalls that can affect performance and reliability. In this blog post, we will explore these pitfalls, offering insight and guidance on how to avoid them.
Understanding Apache Kafka and Apache Camel
What is Apache Kafka?
Apache Kafka is a distributed event streaming platform capable of handling trillions of events a day. It is designed to be fault-tolerant and highly scalable. This makes it a popular choice for real-time data pipelines and streaming applications.
What is Apache Camel?
Apache Camel is an open-source integration framework that allows for simple and efficient integration between various systems. It provides a wide variety of connectors (known as components), such as Kafka, to facilitate message routing and transformation.
Understanding how these two technologies work individually is critical before diving into how they can effectively work together.
Why Integrate Kafka with Camel?
Integrating Apache Kafka with Apache Camel provides:
- Seamless message routing: Camel's extensive collection of components simplifies routing logic.
- Higher throughput: Kafka's architecture allows for managing large volumes of data efficiently.
- Scalability: The integration allows for configuring scaling in both Kafka and Camel, increasing performance.
With these benefits in mind, let's explore common pitfalls developers encounter when integrating Apache Kafka with Apache Camel.
Common Pitfalls
1. Lack of Proper Configuration
Pitfall: One of the most common mistakes is overlooking necessary configurations in both Kafka and Camel.
Why it Matters: Correct configurations ensure that the message brokering operates efficiently and reliably.
Solution: Always refer to the Apache Camel documentation for detailed configuration options. Ensure that you configure:
- Bootstrap servers: Specify the Kafka broker endpoints.
- Consumer group ID: For managing delivery order and failures.
- Deserialization: Ensure messages are properly deserialized.
Example Configuration:
import org.apache.camel.builder.RouteBuilder;
public class KafkaRoute extends RouteBuilder {
@Override
public void configure() throws Exception {
from("kafka:myTopic?brokers=localhost:9092")
.to("log:received-message");
}
}
In the snippet above, we are defining a Camel route that consumes messages from a Kafka topic called "myTopic." Here, remember to set the brokers to your actual Kafka cluster.
2. Ignoring Error Handling
Pitfall: Failing to implement robust error handling can lead to message loss or duplication.
Why it Matters: Without corrective measures, your application may not behave as expected in case of exceptions.
Solution: Implement retry policies or use a dead-letter channel to handle failed messages effectively.
Example of Error Handling:
from("kafka:myTopic?brokers=localhost:9092")
.errorHandler(deadLetterChannel("activemq:queue:deadLetter"))
.to("log:received-message")
.onException(Exception.class)
.handled(true)
.to("log:error-logging");
In this code, we are sending any failed messages to a dead-letter queue while logging error messages, showcasing how Camel can facilitate error management.
3. Not Using the Right Serialization/Deserialization
Pitfall: Neglecting to select appropriate serializers can lead to serialization errors.
Why it Matters: Data integrity is paramount; if data cannot be serialized or deserialized correctly, the application will face issues.
Solution: Choose serialization and deserialization libraries wisely, preferably ones that are compatible with Kafka.
Example Configuration:
For JSON serialization, configure your Camel route as follows:
from("kafka:myTopic?brokers=localhost:9092&valueDeserializer=org.apache.kafka.common.serialization.StringDeserializer")
.unmarshal().json(MyDTO.class)
.to("log:processing-message");
Here, we specify the Kafka deserializer ensuring proper conversion for our messages.
4. Overlooking Performance Tuning
Pitfall: Many developers fail to optimize configurations for performance in high throughput systems.
Why it Matters: With improper tuning, you may encounter bottlenecks that can drag down overall performance.
Solution: Monitor and tune parameters, including:
- Batch size: Determines how many records to send in one batch for processing.
- Buffer size: Buffers set on the producer and consumer sides for tuning memory usage.
Example of Performance Tuning:
properties.put(ProducerConfig.BATCH_SIZE_CONFIG, 16384); // 16KB batch size
properties.put(ProducerConfig.LINGER_MS_CONFIG, 5); // Wait time before sending the batch
These settings can significantly improve throughput by enabling Kafka to send message batches rather than individual messages.
5. Failing to Monitor and Log
Pitfall: Not implementing proper logging and monitoring can lead to a lack of insights into potential problems.
Why it Matters: Without monitoring, it becomes challenging to diagnose issues, leading to increased downtime.
Solution: Use Camel's built-in logging capabilities and external monitoring tools.
Example of Monitoring:
from("kafka:myTopic?brokers=localhost:9092")
.to("log:received-message?level=INFO")
.process(exchange -> {
// Custom processing logic
});
This configuration allows you to log every message received, providing insights that will be beneficial for monitoring application performance.
A Final Look
Integrating Apache Kafka with Camel offers powerful avenues for building robust data pipelines. However, several common pitfalls can impede your application's full potential. By understanding proper configuration, implementing effective error handling, ensuring correct serialization methods, tuning performance settings, and maintaining vigilant monitoring, you can avoid these pitfalls effectively.
For more information about Camel's Kafka component, check the Apache Camel Kafka Documentation.
If you are looking for more hands-on integration and further reading, explore the Kafka's official documentation to understand how it fits into your overall architecture.
By being mindful of these considerations, you can maximize the potential of your integration efforts, leading to a more optimized and efficient application. Happy coding!