Constructors vs. Setters: When to Use Each in Java
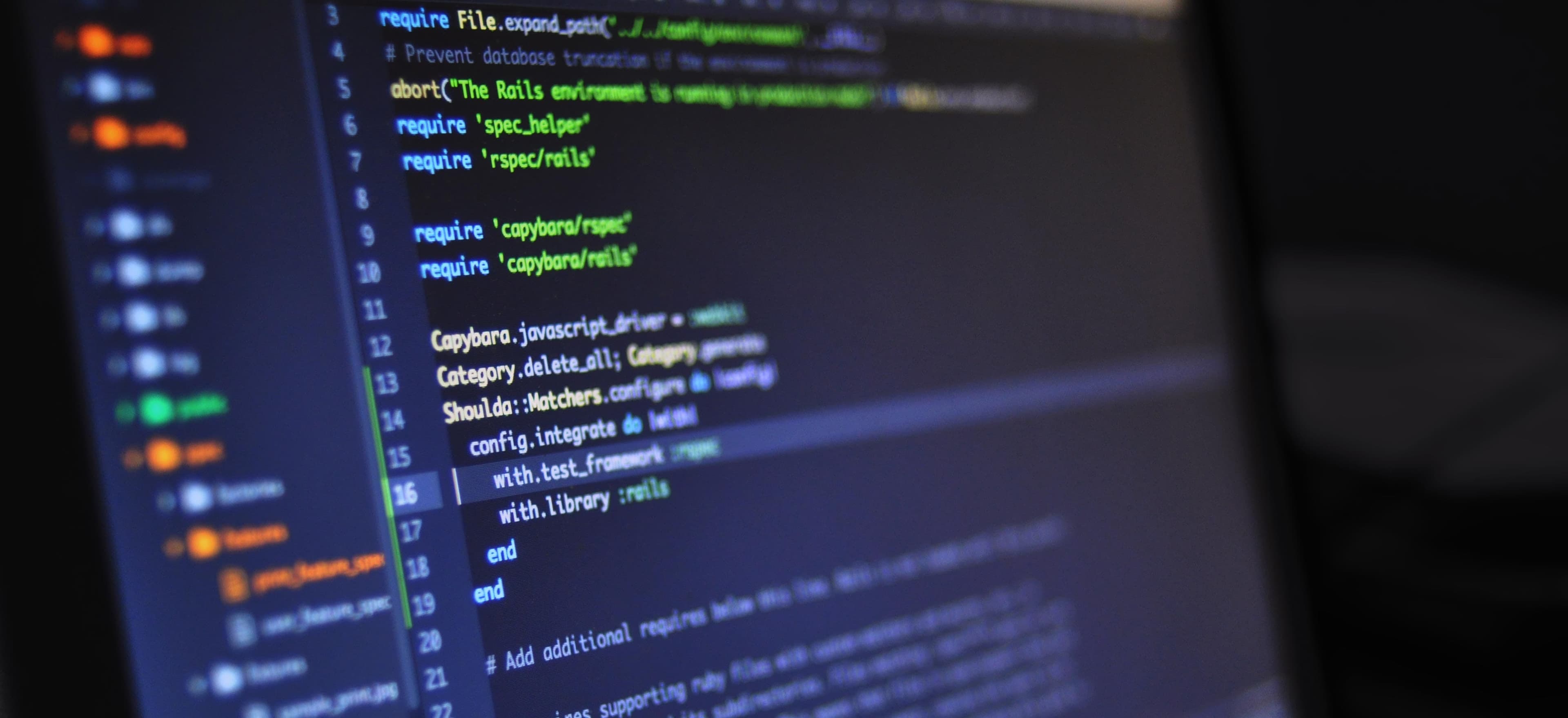
- Published on
Constructors vs. Setters: When to Use Each in Java
Java is an object-oriented programming language that heavily relies on concepts like encapsulation, abstraction, inheritance, and polymorphism. Among these concepts, the handling of object initialization is crucial. When creating an object, you essentially have two primary approaches for initializing its state: Constructors and Setters. Understanding when to use each is vital for writing clean, maintainable code.
What Are Constructors?
A constructor is a special method that is called when an object of a class is instantiated. The purpose of a constructor is to initialize the newly created object, often with specific values.
Key Features of Constructors
- Same Name as Class: A constructor must have the same name as the class.
- No Return Type: Unlike regular methods, constructors do not have a return type.
- Overloading: You can have multiple constructors in a class with different parameter lists.
Example of a Constructor
public class Person {
private String name;
private int age;
// Constructor
public Person(String name, int age) {
this.name = name;
this.age = age;
}
// Getters
public String getName() {
return name;
}
public int getAge() {
return age;
}
}
In the example above, the Person
class has a constructor that initializes the name
and age
. This is a clear way to ensure that every Person
object starts with defined values.
What Are Setters?
Setters (also known as mutator methods) are methods that allow you to set or update the values of an object's properties after it has been constructed. Setters offer flexibility for changing values without needing to instantiate a new object.
Key Features of Setters
- Change State: Setters can change the state of an object at any time.
- Validation: Setters can include validation to ensure that only appropriate values are set.
- Fluency: They can be used in a fluent interface for improved readability.
Example of a Setter
public class Employee {
private String name;
private double salary;
// Constructor
public Employee(String name) {
this.name = name;
}
// Setter for salary
public void setSalary(double salary) {
if (salary > 0) {
this.salary = salary;
} else {
System.out.println("Salary must be greater than zero.");
}
}
// Getter for salary
public double getSalary() {
return salary;
}
}
In this Employee
class, we have a constructor that initializes the name and a setter for salary that includes a validation check to ensure that the salary is a positive value.
When to Use Constructors
Choosing between a constructor and a setter often depends on the initialization requirement and the context of the object.
Use a Constructor When:
-
Immediate Initialization: If your object requires that certain properties are initialized at the time of instantiation, use constructors.
-
Immutability: Use a constructor if you want object state to remain unchanged after creation. By setting fields through a constructor and not providing setters, you can create immutable classes.
-
Mandatory Parameters: If certain parameter values are mandatory for an object to be in a valid state, you can enforce this by making them constructor parameters.
Example: Immediate Initialization
public class Book {
private String title;
private String author;
public Book(String title, String author) {
this.title = title;
this.author = author;
}
// Getters...
}
In the above Book
class, a title
and an author
are required for creation.
When to Use Setters
Setters allow for a more flexible approach to defining and updating property values.
Use a Setter When:
-
Optional Parameters: If certain properties can be set later, then using setters is appropriate.
-
Controlled Updates: Use setters for properties that require validation or complex processing on update.
-
Chaining Calls: Setters can be designed for chaining, making the code more readable.
Example: Optional Parameters
public class Car {
private String make;
private String model;
private int year;
public Car(String make, String model) {
this.make = make;
this.model = model;
}
public Car setYear(int year) {
this.year = year;
return this;
}
// Getters...
}
In this Car
class, we provide optional configuration for the year
using a setter, allowing for fluent use like:
Car myCar = new Car("Toyota", "Corolla").setYear(2022);
Best Practices
-
Parameter Validation: Use setters to perform validation. For instance, you might want to ensure that a person's age isn't negative.
-
Clarity and Readability: Choose an approach that enhances the clarity and readability of your code. Sometimes a mix of both is appropriate.
-
Consistency: Stick to a consistent approach throughout your codebase. If you start using constructors for one set of classes, continue that practice unless there's a compelling reason to switch.
-
Immutability: If you wish to create immutable objects, ensure the state can only be set during construction and not altered afterward through setters.
-
Documentation: Comment your code to explain why you’re using constructors or setters in certain cases, which will aid other developers in understanding your design choices.
My Closing Thoughts on the Matter
In conclusion, both constructors and setters play vital roles in Java programming. The choice between them is determined by context, the needed flexibility, and design concerns such as immutability and validation. By understanding when to use each, you can create classes that are easy to maintain, understand, and extend.
For further reading, consider exploring the concepts of encapsulation and immutability in Java. Resources like Oracle's Java Tutorials can provide additional insights into best practices within object-oriented design.
Using constructors and setters effectively can ultimately lead to a cleaner, more efficient codebase that enhances maintainability and scalability in your Java applications.