Mastering Default Methods in JDK 8: Common Pitfalls
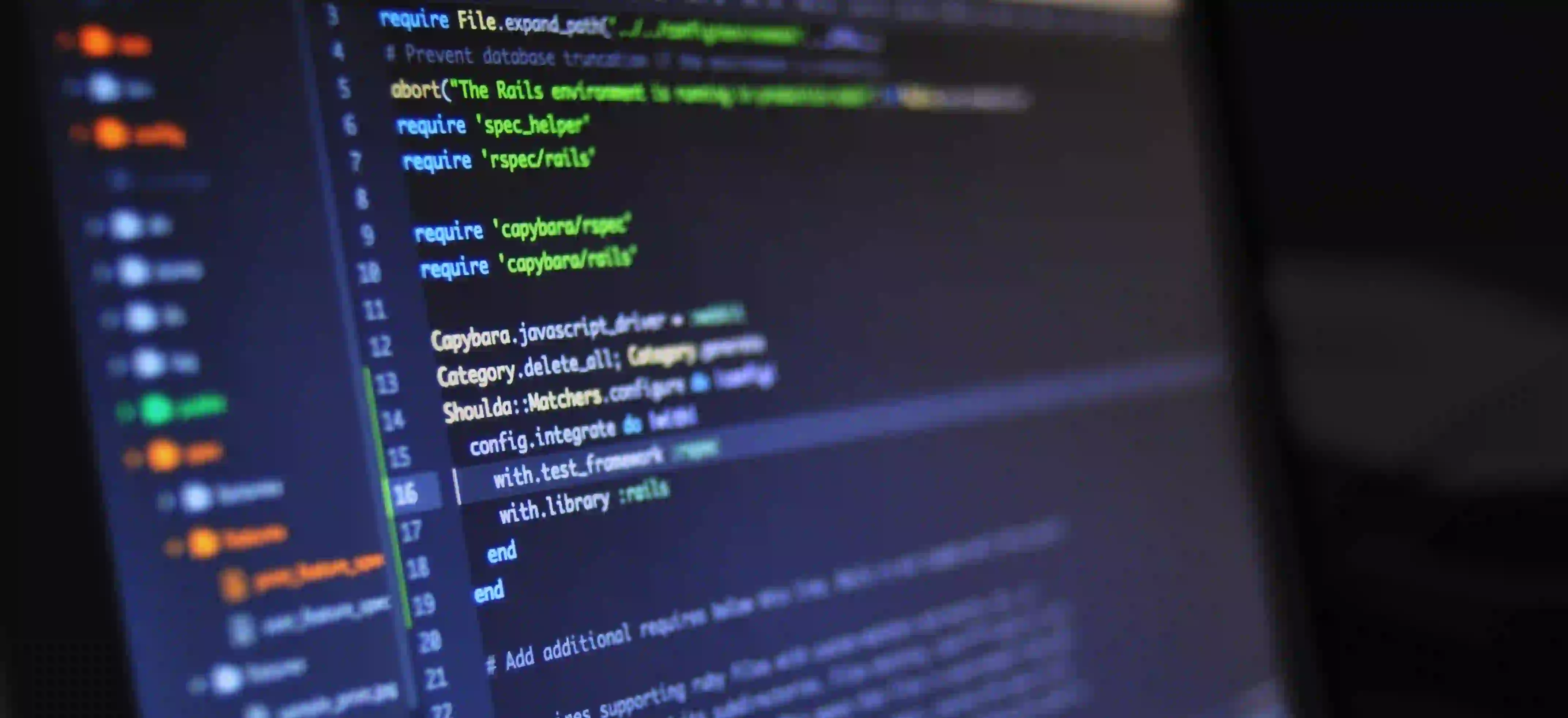
Mastering Default Methods in JDK 8: Common Pitfalls
Java has evolved significantly over the years, with many enhancements aimed at making the language more powerful and expressive. One of the most notable additions in Java 8 was the introduction of default methods in interfaces. Default methods offer a way to define behavior directly in interfaces, providing greater flexibility and reducing boilerplate code. However, with great power comes great responsibility. In this post, we will explore some common pitfalls associated with default methods and provide guidelines on how to avoid them.
What Are Default Methods?
Default methods, also known as defender methods, allow you to implement methods within an interface with the default
keyword. This means that when a class implements the interface, it can either use the default implementation or override it.
Syntax of Default Methods
public interface MyInterface {
default void display() {
System.out.println("Default Implementation");
}
}
In this example, any class that implements MyInterface
inherits the display
method unless it chooses to implement its version. This increases code reusability and flexibility but can also lead to complexities.
Common Pitfalls with Default Methods
While default methods can be incredibly useful, they also come with unique challenges. Here are several common pitfalls developers should be aware of:
1. Inheriting Multiple Default Methods
One of the more significant issues arises when a class implements multiple interfaces that have default methods with the same signature. This results in a diamond problem. Java requires that the class must explicitly override the method to resolve this ambiguity.
Example
interface InterfaceA {
default void show() {
System.out.println("InterfaceA show");
}
}
interface InterfaceB {
default void show() {
System.out.println("InterfaceB show");
}
}
class MyClass implements InterfaceA, InterfaceB {
// Compiler error unless overridden
@Override
public void show() {
InterfaceA.super.show(); // or InterfaceB.super.show();
}
}
In this code snippet, if MyClass
fails to provide an implementation for show
, it will lead to a compilation error. Therefore, always ensure to explicitly override methods when there’s a chance of ambiguity.
2. Overcomplicating Interfaces
With the advent of default methods, there is a tendency for developers to overload interfaces with too much behavior. Interfaces are intended to represent a contract, and too many default methods can clutter the interface, violating the single responsibility principle.
Best Practice
Maintain simplicity: Keep interfaces lean and focused. Use default methods sparingly, primarily for backward compatibility or to provide default behavior that is universally applicable across multiple implementations.
3. State within Default Methods
Default methods cannot maintain any state because they belong to the interface. Attempting to do so can lead to confusion and unexpected behavior.
Example of a Pitfall
interface Counter {
default void increment() {
count++; // This will not compile!
}
int count = 0; // Interface fields are implicitly public, static and final
}
In this scenario, the field count
is static and final, making it impossible to modify within the increment()
method. Therefore, avoid including any state management within default methods to prevent misunderstandings.
4. Not Taking Advantage of Virtual Extension
Default methods can be effective for code evolution. You can add new methods to existing interfaces without breaking the implementations. However, if developers do not take advantage of this feature, they may not fully utilize default methods.
Example of Evolution
interface MyNewInterface {
default void newMethod() {
System.out.println("New default method");
}
}
By adding newMethod
, you allow new implementations to benefit from this without forcing existing classes to modify their implementation. Embrace this aspect of default methods to ensure smooth evolution.
Best Practices for Using Default Methods
1. Use Default Methods for Default Behavior
Default methods should primarily serve the purpose of providing a common implementation when no specific behavior is required.
2. Clearly Document Default Methods
Maintaining documentation on what each default method does will significantly aid other developers (and your future self). Use Javadoc effectively to describe the behavior and any potential side effects.
3. Maintain Interface Cohesion
Keep related methods together, and avoid adding disparate functionalities into the same interface. A cohesive interface improves understandability.
4. Warn Against Overriding Default Methods Unnecessarily
Ensure that overriding default methods only occurs if there is a valid reason to change the behavior. Default methods should cover the majority of use cases before customization is necessary.
Bringing It All Together
Mastering default methods in JDK 8 is pivotal for writing effective, efficient, and clean Java code. However, like any powerful feature, they come with their set of common pitfalls. By understanding the potential complications and adhering to best practices, developers can reap the benefits while minimizing risks.
For further insight into the topic of Java interfaces and functional programming characteristics introduced in Java 8, check out the Java Tutorials by Oracle and Modern Java in Action to enrich your understanding.
Remember, Java continues to evolve. Always stay updated on the latest practices and be ready to adapt your code accordingly. Happy coding!