Mastering Database Schema Navigation in Java: Common Pitfalls
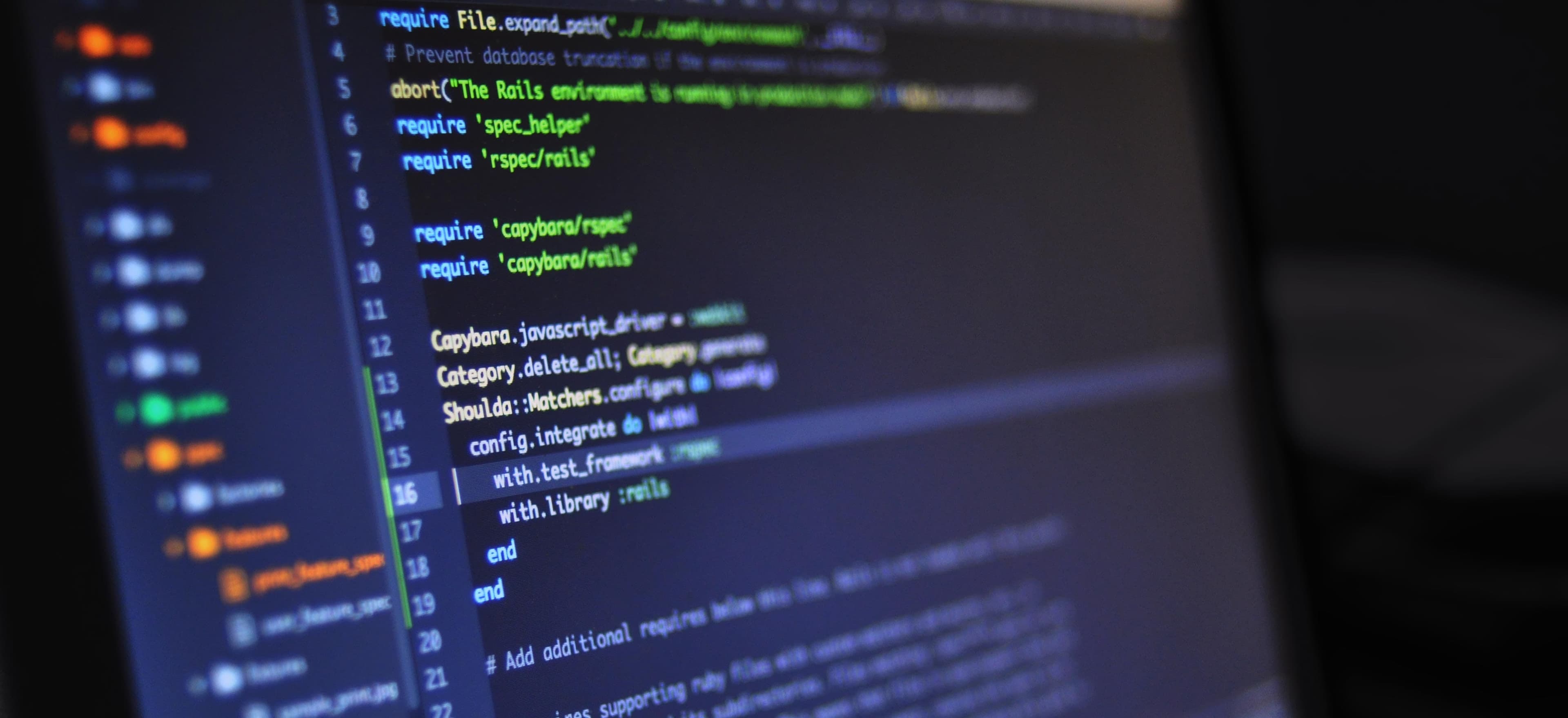
- Published on
Mastering Database Schema Navigation in Java: Common Pitfalls
Managing database schemas is a critical component of developing robust Java applications. This blog post aims to equip you with essential insights about navigating through different database schemas, while also illuminating common pitfalls encountered along the way.
Understanding Database Schemas
A database schema is essentially a blueprint of how data is organized and structured in a database. It encompasses the tables, relationships, views, and other elements that delineate the architecture of the database. In Java, working with database schemas efficiently requires both fundamental knowledge and practical experience.
Why Navigation Matters
Database navigation plays a pivotal role in:
- Performance: Inefficient schema navigation can result in slow queries and application performance issues.
- Data Integrity: Navigating schemas correctly helps prevent data inconsistency and potential loss.
- Maintainability: Well-structured code facilitates easier modifications and enhancements.
In this post, we will unveil some of the common pitfalls developers face when dealing with database schemas in Java and offer solutions to steer clear of them.
Common Pitfalls
Pitfall 1: Inadequate Understanding of Schema Structure
One of the most serious missteps developers make is failing to understand the database schema structure thoroughly before initiating development. This can lead to poor design choices, incorrect relationships, and inefficient queries.
Example:
// Incorrect query due to lack of schema understanding
String query = "SELECT * FROM user WHERE id = ?";
If the developer did not understand that the user
table has a complex relationship with the roles
table, the query might not fetch adequate information.
Solution: Before coding, take time to visualize the schema. Use schema diagrams and tools like MySQL Workbench or pgAdmin. Understand all relationships and potential data you might need to navigate.
Pitfall 2: Ignoring Joins
Ignoring the significance of joins in SQL can lead to unnecessary applications of subqueries, which may degrade performance.
Example:
// Suboptimal performance due to ignoring joins
String query = "SELECT * FROM users WHERE id IN (SELECT userId FROM orders WHERE amount > 100)";
Here, leveraging joins would result in a more efficient query.
Solution:
Improve your SQL with JOIN
operations. Here’s how:
// Optimized approach with JOIN
String query = "SELECT u.name, o.amount FROM users u JOIN orders o ON u.id = o.userId WHERE o.amount > 100";
Pitfall 3: Hardcoding Schema Names
Hardcoding schema names within your application can lead to maintenance headaches. In case the schema changes or if your application needs to run on different environments, you will face compatibility issues.
Example:
// Hardcoded schema reference
String query = "SELECT * FROM public.users";
Should the schema name change, extensive refactoring will be required.
Solution:
Utilize configuration files or environment variables to store schema names. This ensures flexibility and helps preserve code cleanliness.
Properties properties = new Properties();
properties.load(new FileInputStream("config.properties"));
String schemaName = properties.getProperty("schema.name");
String query = "SELECT * FROM " + schemaName + ".users";
Pitfall 4: Lack of Parameterization
SQL injection remains a significant concern in web applications. Failing to use parameterized queries can expose your application to security vulnerabilities.
Example:
// Vulnerable to SQL injection
String userId = "' OR '1'='1";
String query = "SELECT * FROM users WHERE id = '" + userId + "'";
Solution:
Always use PreparedStatement for safe parameterization.
PreparedStatement statement = connection.prepareStatement("SELECT * FROM users WHERE id = ?");
statement.setString(1, userId);
ResultSet rs = statement.executeQuery();
Pitfall 5: Neglecting Transactions
Managing transactions improperly is another common oversight. Skipping transaction management can lead to data integrity issues due to partial updates in a multi-operation scenario.
Example:
connection.setAutoCommit(false);
try {
// multiple insert operations
statement1.executeUpdate();
statement2.executeUpdate();
} catch (SQLException e) {
connection.rollback(); // to avoid data inconsistency
}
Solution: Utilize try-catch-finally blocks effectively to always ensure that transactions are committed or rolled back appropriately.
Pitfall 6: Over-Reliance on ORM
While Object-Relational Mapping (ORM) tools like Hibernate expedite development, sole reliance on them can obscure how data is actually being accessed and manipulated.
Example:
List<User> users = session.createQuery("FROM User").list();
This is convenient but adds complexity when optimization is needed.
Solution: Understand the underlying SQL being generated and, when needed, write custom queries for performance-critical paths.
List<User> users = session.createNativeQuery("SELECT * FROM users WHERE age > :age", User.class)
.setParameter("age", 25)
.getResultList();
Tools and Libraries to Assist Database Schema Navigation
To further aid in database schema navigation, various libraries and tools could prove invaluable:
- Hibernate: Offers powerful ORM facilities that can ease schema navigation while being cautious about performance.
- Spring Data JPA: Helps with abstraction over data access layers in a Spring application.
- Postman (for REST APIs): If your application communicates with a database via REST APIs, Postman can be effective for testing and response validation.
For a comprehensive exploration of ORM and Spring Data JPA, check this guide.
Best Practices
- Documentation: Document the database schema clearly. This can be valuable for team members new to the project.
- Routine Schema Review: Regularly review the schema. Evolving application requirements may necessitate adjustments.
- Unit Tests: Integrate database unit tests to catch issues early on, ensuring that your data access layers are functioning as expected.
The Last Word
Mastering database schema navigation in Java involves recognizing common pitfalls and focusing on best practices. By cultivating an understanding of your database structure, optimizing queries, ensuring code flexibility, and managing data securely, you will be well on your way to building robust applications.
Taking the time to properly navigate schemas not only leads to enhanced performance but also ensures a comprehensive and secure application. Remember, preparation and continual learning are vital components of successful database management.
In case you are interested in learning more about handling database connections in Java, you can visit this tutorial for additional insights.
Feel free to share your own experiences or tips on database schema navigation in the comments below! Happy coding!