Troubleshooting MQTT Message Loss with Kafka Integration
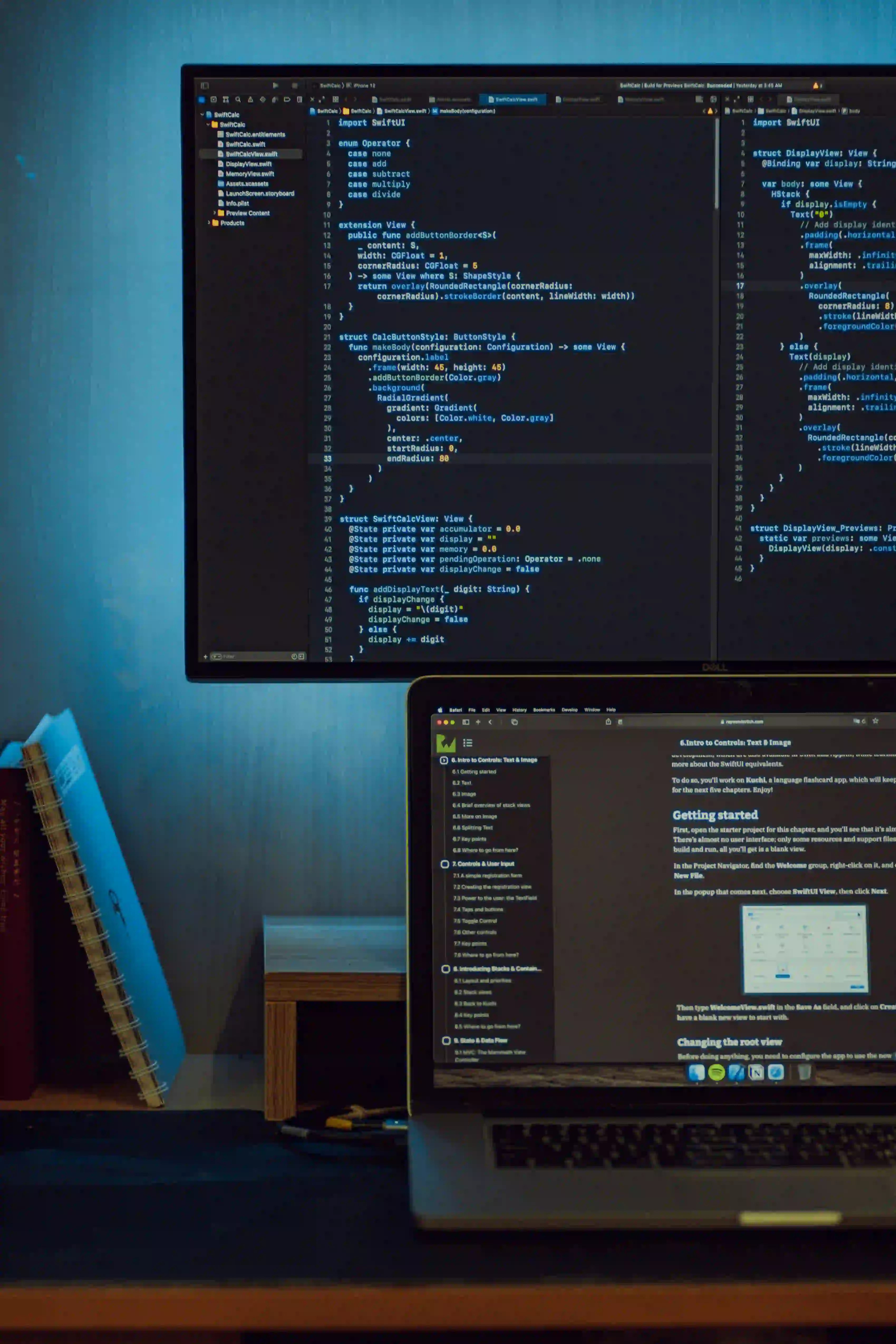
Troubleshooting MQTT Message Loss with Kafka Integration
In the modern world of IoT (Internet of Things), the integration of protocols like MQTT (Message Queuing Telemetry Transport) with systems like Apache Kafka can help facilitate real-time data processing and analytics. However, one of the significant challenges that developers face in this integrated environment is the potential for message loss. In this blog post, we will explore potential causes of MQTT message loss, how to troubleshoot it efficiently, and remedial strategies to ensure data integrity.
Understanding MQTT and Kafka Integration
What is MQTT?
MQTT is a lightweight messaging protocol designed for low-bandwidth, high-latency networks. Its publish-subscribe model allows devices to communicate efficiently. The key features of MQTT include:
- Lightweight: Minimal bandwidth usage makes it ideal for constrained environments.
- Quality of Service (QoS): MQTT offers three levels of QoS which help determine how messages are delivered:
- QoS 0: At most once.
- QoS 1: At least once.
- QoS 2: Exactly once.
What is Kafka?
Apache Kafka is a distributed streaming platform designed for building real-time data pipelines and streaming applications. It provides:
- Scalability: Kafka can handle high throughput.
- Durability: Messages can be retained for long periods.
- Fault Tolerance: It replicates data across nodes.
Integrating MQTT and Kafka can create a robust architecture for handling real-time data. However, developers sometimes encounter message loss issues. Let's delve into troubleshooting this problem.
Identifying Potential Causes of Message Loss
Before jumping to solutions, it’s crucial to understand the underlying causes of message loss between MQTT and Kafka:
-
Client Configuration Issues:
- Incorrect client settings for QoS levels can lead to loss. For instance, using QoS 0 and expecting delivery guarantees can be misleading.
-
Network Reliability:
- Network interruptions can cause message loss. MQTT operates over TCP, and if typically the network is unstable, it can impact message delivery.
-
Broker Configuration:
- If the MQTT broker is misconfigured (e.g., it doesn’t have persistence enabled), it can lose messages upon crash. Similarly, Kafka’s configuration limits can impact message retention.
-
Resource Constraints:
- Both MQTT brokers and Kafka brokers need sufficient resources (memory, CPU, disk I/O). Under heavy load, message loss might occur.
-
Serialization Issues:
- If messages are not serialized correctly when transitioning from MQTT to Kafka, they can become unreadable.
Diagnostic Steps to Troubleshoot Message Loss
Step 1: Assess Client Configuration
Ensure that the MQTT clients connected to the broker are set with an appropriate QoS level. For critical messages, use QoS 1 or 2 to ensure message delivery.
Example QoS Configuration
// MQTT client configuration example in Java
MqttConnectOptions options = new MqttConnectOptions();
options.setCleanSession(false);
options.setConnectionTimeout(10);
options.setKeepAlive(60);
options.setMqttVersion(MqttConnectOptions.MQTT_VERSION_3_1_1);
options.setUserName("username");
options.setPassword("password".toCharArray());
// Set QoS level
options.setWill("topic/will", "client disconnected".getBytes(), 2, true);
Why This Matters: Setting cleanSession
to false
retains messages while the client is disconnected.
Step 2: Monitor Network Conditions
Utilize network monitoring tools to assess the stability and throughput of the network infrastructure supporting your MQTT and Kafka integration. Tools like Wireshark or NetFlow can help in identifying potential issues.
Tip: Implement retries and exponential backoff strategies in your applications to handle transient network failures gracefully.
Step 3: Evaluate Broker Configurations
Examine both the MQTT and Kafka broker configurations. In the case of the Mosquitto MQTT broker, for example:
# mosquitto.conf example for persistent messaging
persistence true
persistence_location /mosquitto/data/
Why This Matters: Ensuring persistence can prevent message loss during broker restarts or crashes.
In Kafka, ensure that your topic retention settings are appropriately configured:
# Example Kafka topic configuration
kafka-topics --create --topic my_topic --bootstrap-server localhost:9092 --replication-factor 3 --partitions 1 --config retention.ms=604800000
Why This Matters: Retention settings control how long messages will be kept. Setting it too low can lead to premature deletions.
Step 4: Check System Resources
Perform a health check of the resources allocated to your brokers. High CPU usage or low available memory can lead to failure in message processing.
Example Command to Check Disk Usage on Linux:
df -h
Why This Matters: Insufficient disk space can prevent Kafka from writing new messages, leading to potential data loss.
Step 5: Monitor Serialization and Deserialization
Ensure all data serialized into MQTT messages is being deserialized correctly by the Kafka consumer. Incompatible formats can cause data to be lost or corrupted.
Example Serialization Code
// Using Jackson for serialization
ObjectMapper mapper = new ObjectMapper();
String jsonString = mapper.writeValueAsString(myDataObject);
Why This Matters: This code snippet ensures that your data object is transformed into a JSON string, which can be safely transported through MQTT and processed by Kafka.
Strategies for Ensuring Message Delivery
Having understood the potential causes and troubleshooting techniques, here are some strategies to ensure message delivery:
-
Use Higher QoS Levels: Opt for QoS 2 for critical messages that must be delivered exactly once.
-
Implement Acknowledgement Mechanisms: Design your system to require acknowledgments from Kafka upon receipt of messages.
-
Logging and Monitoring: Implement comprehensive logging and monitoring for both MQTT and Kafka to identify issues proactively.
-
Leverage Retained Messages: Use MQTT's retained message feature for the last known good value of data (like sensor readings) to ensure subscribers receive relevant information upon connecting.
-
Load Testing: Regularly perform load tests to ensure that both the MQTT and Kafka setups can handle the expected load without losing messages.
-
Consider Alternate Protocols: If message loss persists, evaluate whether other protocols better suit your use case, such as AMQP or HTTP/2.
Wrapping Up
Integrating MQTT and Kafka presents an excellent opportunity for real-time processing of IoT data. However, troubleshooting message loss in this environment requires a comprehensive understanding of both technologies and their configurations.
By following the diagnostic steps outlined above and implementing remedial strategies, you can effectively mitigate message loss and build a more reliable system. For further reading on best practices in MQTT and Kafka integration, consider checking out the official MQTT documentation and Kafka documentation for in-depth knowledge.
Happy coding!