Mastering Hexadecimal Formatting in JDK 17: Common Pitfalls
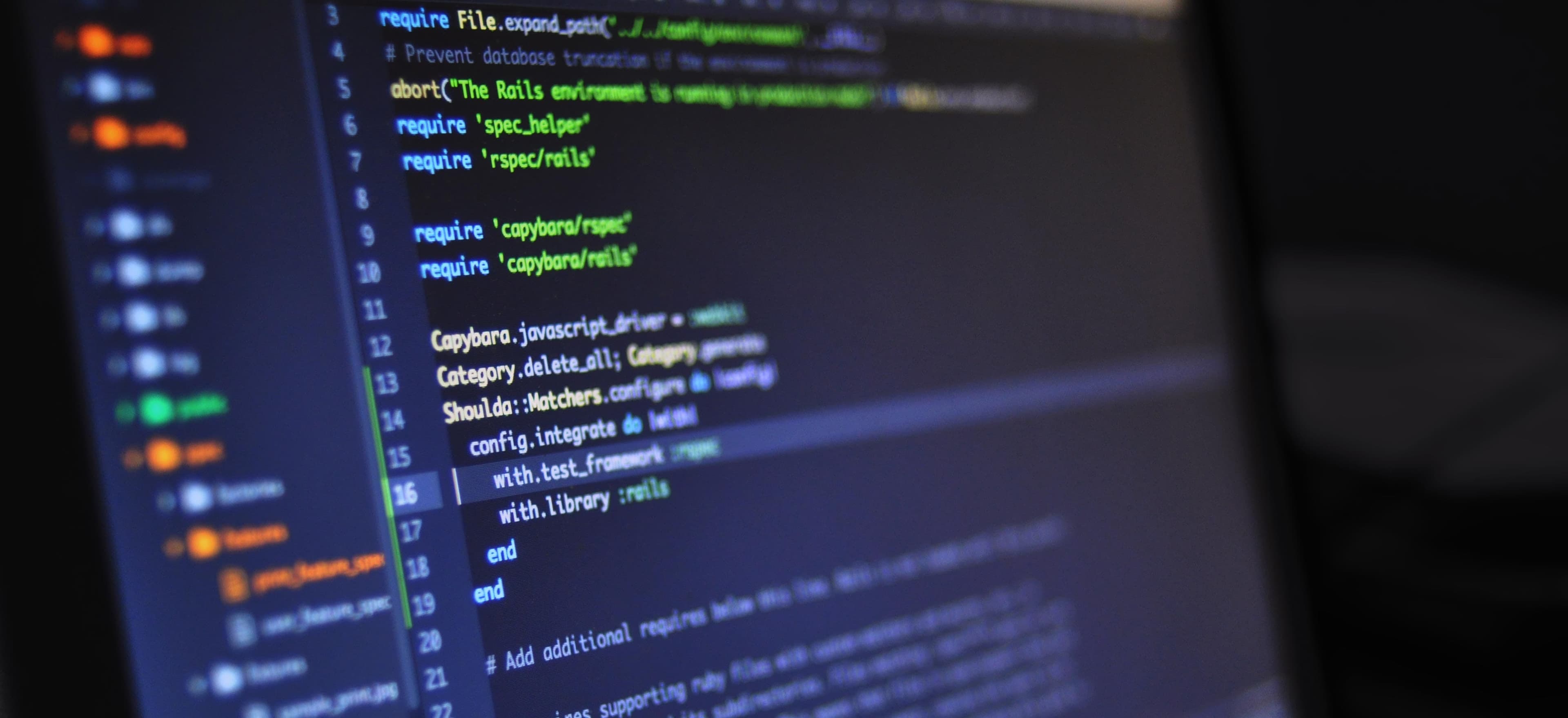
- Published on
Mastering Hexadecimal Formatting in JDK 17: Common Pitfalls
In today's programming world, understanding how to work with different numeral systems is essential. Java, with its vast array of built-in libraries and functions, makes manipulating various types of data straightforward. Specifically, hexadecimal formatting, which uses a base-16 numeral system, is critical for tasks ranging from color representation in graphics to low-level memory management. In JDK 17, mastering hexadecimal format is crucial, and this blog will highlight common pitfalls that developers face while working with it.
Why Hexadecimal?
Hexadecimal (or hex) simplifies binary representation by expressing every four bits as a single digit. Each digit can represent values from 0 to 15, using the symbols 0-9 and A-F. The benefits include:
- Conciseness: It is more compact than binary.
- Clarity: It is easier for humans to read and understand relative to binary equivalents.
- Direct Mapping: Hexadecimal maps directly to byte values, aiding in programming at the hardware level.
Understanding JDK 17's String.format
Java has a built-in method for formatting numbers, including hexadecimal values. Let's start with a basic example that demonstrates the formatting of integers into hexadecimal:
public class HexadecimalExample {
public static void main(String[] args) {
int number = 255;
String hex = String.format("%02X", number);
System.out.println("Hexadecimal representation: " + hex);
}
}
Code Commentary:
%02X
: This is a format specifier where:%
: Indicate the beginning of the format specifier.0
: Pads the number with leading zeros.2
: The minimum width of the output is 2 characters.X
: Converts the integer to an uppercase hexadecimal representation.
The output of the program will be: Hexadecimal representation: FF
.
Common Pitfalls to Avoid
-
Understanding Case Sensitivity:
When working with hex, it's essential to be mindful of the case (uppercase vs. lowercase). Java treatsx
andX
differently in format specifiers:int number = 255; String lowerHex = String.format("%02x", number); // lower case String upperHex = String.format("%02X", number); // upper case System.out.println(lowerHex); // ff System.out.println(upperHex); // FF
Choosing the correct case is important, especially when string comparison is performed later in the code.
-
Ignoring Data Types:
Developers sometimes misuse data types. For example, hexadecimal representation of a long value can lead to unexpected behavior if an integer is used inadvertently:long bigNumber = 4294967296L; // greater than Integer.MAX_VALUE String hexRepresentation = String.format("%X", bigNumber); System.out.println("Hexadecimal representation of long: " + hexRepresentation);
Always ensure the data type matches your needs, especially when performing operations that might involve overflows.
-
Confusing Hexadecimal with Other Bases:
Hexadecimal is not the same as octal or decimal. Developers mistakenly assume that format specifiers are interchangeable. Remember to stay consistent.int decimal = 10; String hex0 = String.format("%x", decimal); // 0a String octal = String.format("%o", decimal); // 12 System.out.println("Hexadecimal: " + hex0); System.out.println("Octal: " + octal);
-
Not Considering Negative Numbers:
Hexadecimal representations of negative numbers can throw developers off, especially with bitwise operations. The following example shows the correct way to display negative numbers:int negativeNumber = -255; String hexNegative = String.format("%08X", negativeNumber & 0xFFFFFFFFL); System.out.println("Hexadecimal representation: " + hexNegative);
Using the bitwise AND operator ensures the correct representation of negative values in hexadecimal.
-
Assuming Default Formatting:
The default format may not always be what you expect. Consider explicitly setting widths and prefixes if necessary. For example, if you need a prefix that indicates it's hexadecimal:int number = 255; String prefixedHex = "0x" + String.format("%X", number); System.out.println("Hexadecimal with prefix: " + prefixedHex);
-
Overlooking Locale Sensitivity:
Formatting can sometimes be affected by locale settings. While hexadecimal formatting is fairly standard, be vigilant if your application is locale-sensitive and requires specific formatting conventions.
Advanced Hexadecimal Manipulation
In addition to simple formatting, you may find yourself needing more advanced manipulation of hexadecimal values. For instance, converting between different numeric systems or performing bitwise operations. Here's how to convert a hexadecimal string back to an integer:
public class HexToInt {
public static void main(String[] args) {
String hexString = "FF";
int intValue = Integer.parseInt(hexString, 16); // Specifies base 16
System.out.println("Integer value of hex FF: " + intValue);
}
}
Code Commentary:
Integer.parseInt(hexString, 16)
: This method converts a hexadecimal string to its integer equivalent. The second argument specifies the base to be used, which is16
for hexadecimal.
The Closing Argument
Hexadecimal formatting is an essential skill for any Java developer working within JDK 17. It is critical not only for proper data representation but also for efficient debugging and performance tuning.
Remember the common pitfalls discussed: case sensitivity, data type mismatches, base confusion, negative representation, default formatting assumptions, and locale sensitivity. By adhering to these guidelines, you can avoid unnecessary problems and make your code more robust and maintainable.
For further reading on number formatting in Java, check out the official Java Documentation. Understanding how the underlying classes interact can significantly enhance your knowledge.
In conclusion, embrace hexadecimal formatting as a valuable tool in your coding arsenal. You'll find that it unlocks new possibilities and plays a vital role in effective data management. Happy coding!