Overcoming Challenges with Default Methods in Java Interfaces
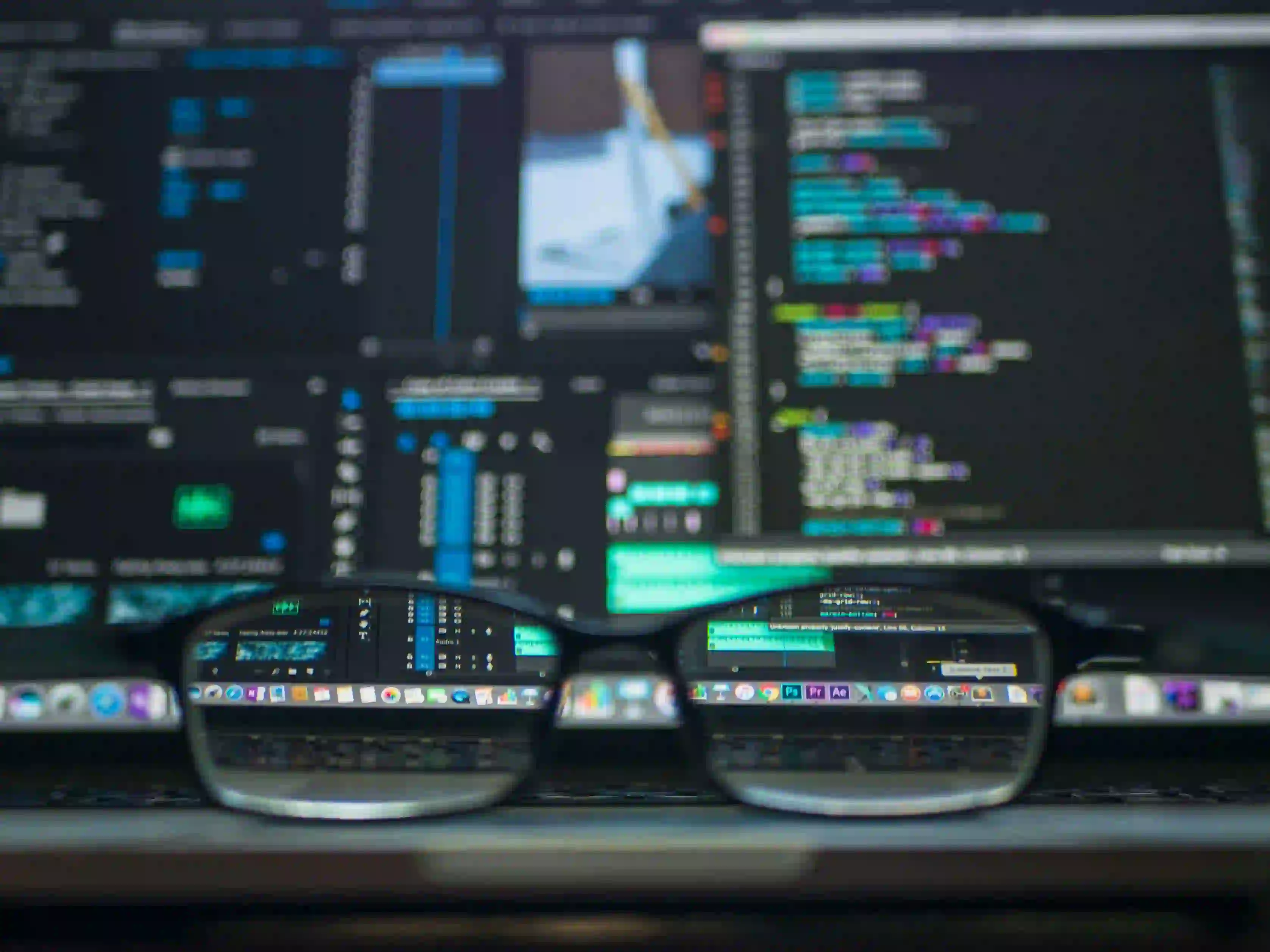
Overcoming Challenges with Default Methods in Java Interfaces
Java has evolved significantly since its inception, adapting to the needs of modern programming. One of the most impactful features introduced in Java 8 is default methods in interfaces. Default methods allow you to add new methods to interfaces without breaking existing implementations. However, along with this power come challenges. In this post, we will explore what default methods are, discuss their benefits, and learn how to overcome common challenges associated with them.
What Are Default Methods?
Before Java 8, interfaces could declare methods, but they couldn't provide implementations. With the introduction of default methods, Java allows interfaces to define methods with a default implementation. This makes it possible to add new functionality to interfaces while maintaining backward compatibility.
Here's an example of a default method:
public interface Vehicle {
void start();
default void honk() {
System.out.println("Beep! Beep!");
}
}
In this example, the Vehicle
interface has a start
method with no implementation and a honk
method with a default implementation. Any class that implements the Vehicle
interface will inherit the behavior of honk
, unless it provides its own implementation.
Benefits of Default Methods
1. Backward Compatibility
Default methods allow you to add new methods to interfaces without requiring changes in the classes that already implement them. This is massively beneficial in large systems with numerous interface implementations.
For example, imagine you have a Vehicle
interface implemented by various classes (like Car
and Bike
). If you need to add a new method to the interface, without default methods, you'd have to modify all those classes to implement this new method.
2. Code Reusability
Default methods promote code reusability by allowing the placement of common logic directly in the interface. This minimizes redundancy and encourages cleaner code architecture.
3. Enhanced Flexibility
They provide more flexibility in designing APIs. API designers can add features without worrying about breaking existing clients.
4. Multi-Interface Inheritance
Default methods enable classes to implement multiple interfaces with default methods without conflicts using method overriding.
Common Challenges with Default Methods
Despite their advantages, default methods come with their share of challenges. Let's explore these challenges and how to overcome them.
1. Method Conflict
If a class implements multiple interfaces that have a default method with the same name and parameters, the compiler will throw a compilation error due to ambiguity.
Example of Method Conflict
public interface A {
default void display() {
System.out.println("Display from A");
}
}
public interface B {
default void display() {
System.out.println("Display from B");
}
}
public class C implements A, B {
@Override
public void display() {
// must override the method to resolve conflict
A.super.display(); // or B.super.display();
}
}
How to Overcome
The solution is to explicitly override the method in the implementing class. You can specify which interface's default method to invoke using the syntax InterfaceName.super.methodName()
.
2. Limited to Non-Abstract Methods
Default methods can become problematic because they cannot be used to provide behavior for abstract methods declared in interfaces. If you try to override a method declared as abstract in an implementing class, you can lose the default behavior.
Example
public interface Shape {
void draw(); // Abstract method
default void resize() {
System.out.println("Resizing the shape");
}
}
If you implement the Shape
interface and provide an implementation for draw
, you cannot provide a default behavior for resize
within the interface.
How to Overcome
Ensure that you have thoughtful design considerations in place about which methods are required to be abstract and which can be default. Aim for clear separation in responsibilities between the abstract and default methods.
3. Inconsistent Object Behavior
Using default methods can sometimes lead to inconsistent behavior, as different implementing classes might provide different implementations for the same default method.
Example
public class Circle implements Shape {
@Override
public void draw() {
System.out.println("Drawing a Circle");
}
}
public class Square implements Shape {
@Override
public void draw() {
System.out.println("Drawing a Square");
}
// Inherits resize() from Shape
}
While the above classes share the resize()
method, the behavior when a circle is resized versus a square could differ based on how such classes are utilized.
How to Overcome
Establish a clear contract on how default methods should behave. Document the expected behavior and potentially use template methods or strategies to standardize functionality across implementations.
4. Increases Complexity
Introducing default methods might increase the complexity of your interfaces. Not only do they complicate the inheritance structure by adding multiple behaviors to interfaces, but they might also introduce unexpected behaviors.
How to Overcome
Maintain simplicity in interface design. Use default methods judiciously, and when introducing multiple behaviors, ensure they are well documented and logically structured.
The Bottom Line
Default methods in Java interfaces provide significant advantages, including backward compatibility and reusability. However, they also present several challenges, such as potential conflicts, inconsistent behavior, and increased complexity.
By recognizing these challenges and following best practices, developers can harness the power of default methods safely and effectively.
Here are some key takeaways:
- Always resolve method conflicts explicitly by overriding methods in the implementing class.
- Be cautious about which methods you designate as default to avoid confusing designs.
- Document your interfaces thoroughly to ensure maintainability and clarity regarding expected behaviors.
For further reading on Java's default methods and interface design, check Java Tutorials.
By mastering default methods in Java interfaces, you can incorporate flexibility and maintainability into your software design while avoiding common pitfalls. Happy coding!